umbrello/umbrello
#include <sqlwriter.h>
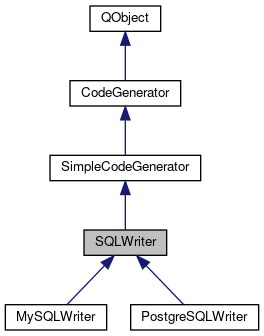
Public Member Functions | |
SQLWriter () | |
virtual | ~SQLWriter () |
virtual QStringList | defaultDatatypes () |
virtual Uml::ProgrammingLanguage::Enum | language () const |
virtual QStringList | reservedKeywords () const |
virtual void | writeClass (UMLClassifier *c) |
![]() | |
SimpleCodeGenerator (bool createDirHierarchyForPackages=true) | |
virtual | ~SimpleCodeGenerator () |
CodeDocument * | newClassifierCodeDocument (UMLClassifier *classifier) |
void | writeCodeToFile (UMLClassifierList &concepts) |
void | writeCodeToFile () |
![]() | |
CodeGenerator () | |
virtual | ~CodeGenerator () |
bool | addCodeDocument (CodeDocument *add_object) |
void | connect_newcodegen_slots () |
virtual void | createDefaultStereotypes () |
CodeDocument * | findCodeDocumentByClassifier (UMLClassifier *classifier) |
CodeDocument * | findCodeDocumentByID (const QString &id) |
QString | findFileName (CodeDocument *codeDocument) |
bool | forceDoc () const |
bool | forceSections () const |
CodeDocumentList * | getCodeDocumentList () |
virtual CodeViewerDialog * | getCodeViewerDialog (QWidget *parent, CodeDocument *doc, Settings::CodeViewerState state) |
virtual QString | getHeadingFile (const QString &file) |
QString | getUniqueID (CodeDocument *codeDoc) |
QString | headingFileDir () const |
bool | includeHeadings () const |
virtual bool | isReservedKeyword (const QString &keyword) |
virtual void | loadFromXMI (QDomElement &element) |
CodeGenerationPolicy::ModifyNamePolicy | modifyNamePolicy () const |
virtual CodeDocument * | newCodeDocument () |
bool | removeCodeDocument (CodeDocument *remove_object) |
virtual void | saveToXMI (QDomDocument &doc, QDomElement &root) |
void | setForceDoc (bool f) |
void | setForceSections (bool f) |
void | setHeadingFileDir (const QString &) |
void | setIncludeHeadings (bool i) |
void | setModifyNamePolicy (CodeGenerationPolicy::ModifyNamePolicy p) |
![]() | |
QObject (QObject *parent) | |
QObject (QObject *parent, const char *name) | |
virtual | ~QObject () |
bool | blockSignals (bool block) |
QObject * | child (const char *objName, const char *inheritsClass, bool recursiveSearch) const |
const QObjectList & | children () const |
const char * | className () const |
bool | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const QObject *receiver, const char *method) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) |
void | dumpObjectInfo () |
void | dumpObjectTree () |
QList< QByteArray > | dynamicPropertyNames () const |
virtual bool | event (QEvent *e) |
virtual bool | eventFilter (QObject *watched, QEvent *event) |
T | findChild (const QString &name) const |
QList< T > | findChildren (const QRegExp ®Exp) const |
QList< T > | findChildren (const QString &name) const |
bool | inherits (const char *className) const |
void | insertChild (QObject *object) |
void | installEventFilter (QObject *filterObj) |
bool | isA (const char *className) const |
bool | isWidgetType () const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const |
void | moveToThread (QThread *targetThread) |
const char * | name () const |
const char * | name (const char *defaultName) const |
QString | objectName () const |
QObject * | parent () const |
QVariant | property (const char *name) const |
void | removeChild (QObject *object) |
void | removeEventFilter (QObject *obj) |
void | setName (const char *name) |
void | setObjectName (const QString &name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | signalsBlocked () const |
int | startTimer (int interval) |
QThread * | thread () const |
Protected Attributes | |
UMLEntity * | m_pEntity |
![]() | |
QString | className_ |
QString | fileName_ |
bool | m_createDirHierarchyForPackages |
QString | m_endl |
QMap< UMLPackage *, QString > | m_fileMap |
QString | m_indentation |
int | m_indentLevel |
![]() | |
bool | m_applyToAllRemaining |
QHash< QString, CodeDocument * > | m_codeDocumentDictionary |
UMLDoc * | m_document |
Additional Inherited Members | |
![]() | |
void | syncCodeToDocument () |
![]() | |
virtual void | checkAddUMLObject (UMLObject *obj) |
virtual void | checkRemoveUMLObject (UMLObject *obj) |
virtual void | syncCodeToDocument () |
![]() | |
void | codeGenerated (UMLClassifier *concept, bool generated) |
void | showGeneratedFile (const QString &filename) |
![]() | |
static QString | cleanName (const QString &name) |
static void | findObjectsRelated (UMLClassifier *c, UMLPackageList &cList) |
static QString | formatDoc (const QString &text, const QString &linePrefix=QLatin1String(" *"), int lineWidth=80) |
static QString | formatSourceCode (const QString &code, const QString &indentation) |
![]() | |
bool | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
bool | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
QString | trUtf8 (const char *sourceText, const char *disambiguation, int n) |
![]() | |
QByteArray | normalizeSignalSlot (const char *signalSlot) |
![]() | |
objectName | |
Detailed Description
Class SQLWriter is a code generator for UMLClassifier objects.
Create an instance of this class, and feed it a UMLClassifier when calling writeClass and it will generate a sql source file for that concept.
Definition at line 29 of file sqlwriter.h.
Constructor & Destructor Documentation
SQLWriter::SQLWriter | ( | ) |
Definition at line 176 of file sqlwriter.cpp.
|
virtual |
Definition at line 180 of file sqlwriter.cpp.
Member Function Documentation
|
virtual |
Reimplement method from CodeGenerator.
Reimplemented from CodeGenerator.
Reimplemented in MySQLWriter, and PostgreSQLWriter.
Definition at line 313 of file sqlwriter.cpp.
|
virtual |
Returns "SQL".
Implements CodeGenerator.
Reimplemented in MySQLWriter, and PostgreSQLWriter.
Definition at line 305 of file sqlwriter.cpp.
|
protectedvirtual |
Handles AutoIncrements.
The derived classes provide the actual body.
- Parameters
-
sql The Stream we should print to entAttList The List of Entity Attributes that we want to auto increment
Reimplemented in MySQLWriter, and PostgreSQLWriter.
Definition at line 580 of file sqlwriter.cpp.
|
protectedvirtual |
Prints out Check Constraints as "ALTER TABLE" statements.
- Parameters
-
sql The stream we should print to constrList The checkConstraints to be printed
Reimplemented in MySQLWriter.
Definition at line 591 of file sqlwriter.cpp.
|
protectedvirtual |
Prints out attributes as columns in the table.
- Parameters
-
sql the stream we should print to entityAttributeList the attributes to be printed
Definition at line 364 of file sqlwriter.cpp.
|
protectedvirtual |
Prints out foreign key constraints as "ALTER TABLE" statements.
- Parameters
-
sql the stream we should print to constrList the foreignkey constraints to be printed
Reimplemented in MySQLWriter.
Definition at line 467 of file sqlwriter.cpp.
|
protectedvirtual |
Prints out Indexes as "CREATE INDEX " statements.
- Parameters
-
sql The Stream we should print to ent The Entity's attributes on which we want to create an Index entAttList The list of entityattributes to create an index upon
Definition at line 543 of file sqlwriter.cpp.
|
protectedvirtual |
Prints out unique constraints (including primary key) as "ALTER TABLE" statements.
- Parameters
-
sql the stream we should print to constrList the unique constraints to be printed
Definition at line 423 of file sqlwriter.cpp.
|
virtual |
Get list of reserved keywords.
Reimplemented from CodeGenerator.
Definition at line 346 of file sqlwriter.cpp.
|
virtual |
Call this method to generate sql code for a UMLClassifier.
- Parameters
-
c the class to generate code for
Implements SimpleCodeGenerator.
Definition at line 188 of file sqlwriter.cpp.
Member Data Documentation
|
protected |
Definition at line 47 of file sqlwriter.h.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2020 The KDE developers.
Generated on Mon Jun 22 2020 13:40:29 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.