KGameDifficulty
#include <KGameDifficulty>
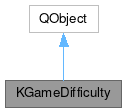
Properties | |
const KGameDifficultyLevel * | currentLevel |
bool | editable |
bool | gameRunning |
const KGameDifficultyLevel * | selectedLevel |
![]() | |
objectName | |
Signals | |
void | currentLevelChanged (const KGameDifficultyLevel *level) |
void | editableChanged (bool editable) |
void | gameRunningChanged (bool gameRunning) |
void | selectedLevelChanged (const KGameDifficultyLevel *level) |
Public Slots | |
void | select (const KGameDifficultyLevel *level) |
Public Member Functions | |
KGameDifficulty (QObject *parent=nullptr) | |
~KGameDifficulty () override | |
void | addLevel (KGameDifficultyLevel *level) |
void | addStandardLevel (KGameDifficultyLevel::StandardLevel level, bool isDefault=false) |
void | addStandardLevelRange (KGameDifficultyLevel::StandardLevel from, KGameDifficultyLevel::StandardLevel to) |
void | addStandardLevelRange (KGameDifficultyLevel::StandardLevel from, KGameDifficultyLevel::StandardLevel to, KGameDifficultyLevel::StandardLevel defaultLevel) |
const KGameDifficultyLevel * | currentLevel () const |
bool | isEditable () const |
bool | isGameRunning () const |
QList< const KGameDifficultyLevel * > | levels () const |
void | setEditable (bool editable) |
void | setGameRunning (bool running) |
![]() | |
QObject (QObject *parent) | |
QBindable< QString > | bindableObjectName () |
bool | blockSignals (bool block) |
const QObjectList & | children () const const |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) const const |
bool | disconnect (const QObject *receiver, const char *method) const const |
void | dumpObjectInfo () const const |
void | dumpObjectTree () const const |
QList< QByteArray > | dynamicPropertyNames () const const |
virtual bool | event (QEvent *e) |
virtual bool | eventFilter (QObject *watched, QEvent *event) |
T | findChild (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QRegularExpression &re, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (Qt::FindChildOptions options) const const |
bool | inherits (const char *className) const const |
void | installEventFilter (QObject *filterObj) |
bool | isQuickItemType () const const |
bool | isWidgetType () const const |
bool | isWindowType () const const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const const |
void | moveToThread (QThread *targetThread) |
QString | objectName () const const |
void | objectNameChanged (const QString &objectName) |
QObject * | parent () const const |
QVariant | property (const char *name) const const |
Q_CLASSINFO (Name, Value) | |
Q_EMIT Q_EMIT | |
Q_ENUM (...) | |
Q_ENUM_NS (...) | |
Q_ENUMS (...) | |
Q_FLAG (...) | |
Q_FLAG_NS (...) | |
Q_FLAGS (...) | |
Q_GADGET Q_GADGET | |
Q_GADGET_EXPORT (EXPORT_MACRO) | |
Q_INTERFACES (...) | |
Q_INVOKABLE Q_INVOKABLE | |
Q_MOC_INCLUDE Q_MOC_INCLUDE | |
Q_NAMESPACE Q_NAMESPACE | |
Q_NAMESPACE_EXPORT (EXPORT_MACRO) | |
Q_OBJECT Q_OBJECT | |
Q_PROPERTY (...) | |
Q_REVISION Q_REVISION | |
Q_SET_OBJECT_NAME (Object) | |
Q_SIGNAL Q_SIGNAL | |
Q_SIGNALS Q_SIGNALS | |
Q_SLOT Q_SLOT | |
Q_SLOTS Q_SLOTS | |
T | qobject_cast (const QObject *object) |
T | qobject_cast (QObject *object) |
QT_NO_NARROWING_CONVERSIONS_IN_CONNECT QT_NO_NARROWING_CONVERSIONS_IN_CONNECT | |
void | removeEventFilter (QObject *obj) |
void | setObjectName (const QString &name) |
void | setObjectName (QAnyStringView name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | setProperty (const char *name, QVariant &&value) |
bool | signalsBlocked () const const |
int | startTimer (int interval, Qt::TimerType timerType) |
int | startTimer (std::chrono::milliseconds interval, Qt::TimerType timerType) |
QThread * | thread () const const |
Static Public Member Functions | |
static KGameDifficulty * | global () |
static KGameDifficultyLevel::StandardLevel | globalLevel () |
![]() | |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *context, Functor functor, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, Functor functor) |
bool | disconnect (const QMetaObject::Connection &connection) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
bool | disconnect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
Additional Inherited Members | |
![]() | |
typedef | QObjectList |
![]() | |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const QMetaMethod &signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const QMetaMethod &signal) |
bool | isSignalConnected (const QMetaMethod &signal) const const |
int | receivers (const char *signal) const const |
QObject * | sender () const const |
int | senderSignalIndex () const const |
virtual void | timerEvent (QTimerEvent *event) |
Detailed Description
KGameDifficulty manages difficulty levels of a game in a standard way.
The difficulty can be a type of game (like in KMines: small or big field) or the AI skills (like in Bovo: how deep should the computer search to find the best move) or a combination of both of them. On the user point of view, it's not really different: either is the game easy or hard to play.
KGameDifficulty contains a list of KGameDifficultyLevel instances. One of these levels is selected; this selection will be recorded when the application is closed. A set of standard difficulty levels is provided by KGameDifficultyLevel, but custom levels can be defined at the same time.
Definition at line 88 of file kgamedifficulty.h.
Property Documentation
◆ currentLevel
|
readwrite |
Definition at line 93 of file kgamedifficulty.h.
◆ editable
|
readwrite |
Definition at line 95 of file kgamedifficulty.h.
◆ gameRunning
|
readwrite |
Definition at line 96 of file kgamedifficulty.h.
◆ selectedLevel
|
readwrite |
Definition at line 94 of file kgamedifficulty.h.
Constructor & Destructor Documentation
◆ KGameDifficulty()
|
explicit |
Definition at line 166 of file kgamedifficulty.cpp.
◆ ~KGameDifficulty()
|
override |
Destroys this instance and all DifficultyLevel instances in it.
Definition at line 174 of file kgamedifficulty.cpp.
Member Function Documentation
◆ addLevel()
void KGameDifficulty::addLevel | ( | KGameDifficultyLevel * | level | ) |
Adds a difficulty level to this instance.
This will not affect the currentLevel() if there is one.
Definition at line 181 of file kgamedifficulty.cpp.
◆ addStandardLevel()
void KGameDifficulty::addStandardLevel | ( | KGameDifficultyLevel::StandardLevel | level, |
bool | isDefault = false ) |
A shortcut for addLevel(new KGameDifficultyLevel(level)).
Definition at line 201 of file kgamedifficulty.cpp.
◆ addStandardLevelRange() [1/2]
void KGameDifficulty::addStandardLevelRange | ( | KGameDifficultyLevel::StandardLevel | from, |
KGameDifficultyLevel::StandardLevel | to ) |
This convenience method adds a range of standard levels to this instance (including the boundaries).
For example:
This adds the levels "Easy", "Medium", "Hard" and "Very hard".
Definition at line 206 of file kgamedifficulty.cpp.
◆ addStandardLevelRange() [2/2]
void KGameDifficulty::addStandardLevelRange | ( | KGameDifficultyLevel::StandardLevel | from, |
KGameDifficultyLevel::StandardLevel | to, | ||
KGameDifficultyLevel::StandardLevel | defaultLevel ) |
This is an overloaded member function, provided for convenience. It differs from the above function only in what argument(s) it accepts. This overload allows to specify a defaultLevel.
Definition at line 212 of file kgamedifficulty.cpp.
◆ currentLevel()
const KGameDifficultyLevel * KGameDifficulty::currentLevel | ( | ) | const |
- Returns
- the current difficulty level
After the KGameDifficulty object has been created, the current difficulty level will not be determined until this method is called for the first time. This allows the application developer to set up the difficulty levels before KGameDifficulty retrieves the last selected level from the configuration file.
Definition at line 243 of file kgamedifficulty.cpp.
◆ currentLevelChanged
|
signal |
Emitted when a new difficulty level has been selected.
◆ editableChanged
|
signal |
Emitted when the editability changes.
- See also
- setEditable
◆ gameRunningChanged
|
signal |
Emitted when a running game has been marked or unmarked.
- See also
- setGameRunning
◆ global()
|
static |
- Returns
- a singleton instance of KGameDifficulty
Definition at line 333 of file kgamedifficulty.cpp.
◆ globalLevel()
|
static |
A shortcut for KGameDifficulty::global()->currentLevel()->standardLevel().
Definition at line 338 of file kgamedifficulty.cpp.
◆ isEditable()
bool KGameDifficulty::isEditable | ( | ) | const |
- Returns
- whether the difficulty level selection may be edited
Definition at line 269 of file kgamedifficulty.cpp.
◆ isGameRunning()
bool KGameDifficulty::isGameRunning | ( | ) | const |
- Returns
- whether a running game has been marked
- See also
- setGameRunning
Definition at line 287 of file kgamedifficulty.cpp.
◆ levels()
QList< const KGameDifficultyLevel * > KGameDifficulty::levels | ( | ) | const |
- Returns
- a list of all difficulty levels, sorted by hardness
Definition at line 236 of file kgamedifficulty.cpp.
◆ select
|
slot |
Select a new difficulty level.
The given level must already have been added to this instance.
- Note
- This does nothing if isEditable() is false. If a game is running (according to setGameRunning()), the user will be asked for confirmation before the new difficulty level is selected.
Definition at line 305 of file kgamedifficulty.cpp.
◆ selectedLevelChanged
|
signal |
Emitted after every call to select(), even when the user has rejected the change.
This is useful to reset a difficulty level selection UI after a rejected change.
◆ setEditable()
void KGameDifficulty::setEditable | ( | bool | editable | ) |
Set whether the difficulty level selection may be edited.
The default value is true.
Definition at line 276 of file kgamedifficulty.cpp.
◆ setGameRunning()
void KGameDifficulty::setGameRunning | ( | bool | running | ) |
KGameDifficulty has optional protection against changing the difficulty level while a game is running.
If setGameRunning(true) has been called, and select() is called to select a new difficulty level, the user will be asked for confirmation.
Definition at line 294 of file kgamedifficulty.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2025 The KDE developers.
Generated on Fri May 2 2025 11:57:56 by doxygen 1.13.2 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.