KGameHighscore
#include <KGameHighscore>
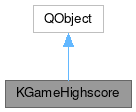
Public Member Functions | |
KGameHighscore (bool forceLocal=true, QObject *parent=nullptr) | |
~KGameHighscore () override | |
QStringList | groupList () const |
bool | hasEntry (int entry, const QString &key) const |
bool | hasTable () const |
QString | highscoreGroup () const |
bool | isLocked () const |
bool | lockForWriting (QWidget *widget=nullptr) |
void | readCurrentConfig () |
QString | readEntry (int entry, const QString &key, const QString &pDefault=QString()) const |
QStringList | readList (const QString &key, int lastEntry=20) const |
int | readNumEntry (int entry, const QString &key, int pDefault=-1) const |
QVariant | readPropertyEntry (int entry, const QString &key, const QVariant &pDefault) const |
void | setHighscoreGroup (const QString &groupname=QString()) |
void | writeAndUnlock () |
void | writeEntry (int entry, const QString &key, const QString &value) |
void | writeEntry (int entry, const QString &key, const QVariant &value) |
void | writeEntry (int entry, const QString &key, int value) |
void | writeList (const QString &key, const QStringList &list) |
![]() | |
QObject (QObject *parent) | |
QBindable< QString > | bindableObjectName () |
bool | blockSignals (bool block) |
const QObjectList & | children () const const |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) const const |
bool | disconnect (const QObject *receiver, const char *method) const const |
void | dumpObjectInfo () const const |
void | dumpObjectTree () const const |
QList< QByteArray > | dynamicPropertyNames () const const |
virtual bool | event (QEvent *e) |
virtual bool | eventFilter (QObject *watched, QEvent *event) |
T | findChild (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QRegularExpression &re, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (Qt::FindChildOptions options) const const |
bool | inherits (const char *className) const const |
void | installEventFilter (QObject *filterObj) |
bool | isQuickItemType () const const |
bool | isWidgetType () const const |
bool | isWindowType () const const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const const |
void | moveToThread (QThread *targetThread) |
QString | objectName () const const |
void | objectNameChanged (const QString &objectName) |
QObject * | parent () const const |
QVariant | property (const char *name) const const |
Q_CLASSINFO (Name, Value) | |
Q_EMIT Q_EMIT | |
Q_ENUM (...) | |
Q_ENUM_NS (...) | |
Q_ENUMS (...) | |
Q_FLAG (...) | |
Q_FLAG_NS (...) | |
Q_FLAGS (...) | |
Q_GADGET Q_GADGET | |
Q_GADGET_EXPORT (EXPORT_MACRO) | |
Q_INTERFACES (...) | |
Q_INVOKABLE Q_INVOKABLE | |
Q_MOC_INCLUDE Q_MOC_INCLUDE | |
Q_NAMESPACE Q_NAMESPACE | |
Q_NAMESPACE_EXPORT (EXPORT_MACRO) | |
Q_OBJECT Q_OBJECT | |
Q_PROPERTY (...) | |
Q_REVISION Q_REVISION | |
Q_SET_OBJECT_NAME (Object) | |
Q_SIGNAL Q_SIGNAL | |
Q_SIGNALS Q_SIGNALS | |
Q_SLOT Q_SLOT | |
Q_SLOTS Q_SLOTS | |
T | qobject_cast (const QObject *object) |
T | qobject_cast (QObject *object) |
QT_NO_NARROWING_CONVERSIONS_IN_CONNECT QT_NO_NARROWING_CONVERSIONS_IN_CONNECT | |
void | removeEventFilter (QObject *obj) |
void | setObjectName (const QString &name) |
void | setObjectName (QAnyStringView name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | setProperty (const char *name, QVariant &&value) |
bool | signalsBlocked () const const |
int | startTimer (int interval, Qt::TimerType timerType) |
int | startTimer (std::chrono::milliseconds interval, Qt::TimerType timerType) |
QThread * | thread () const const |
Static Public Member Functions | |
static void | init (const char *appname) |
![]() | |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *context, Functor functor, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, Functor functor) |
bool | disconnect (const QMetaObject::Connection &connection) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
bool | disconnect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
Protected Member Functions | |
KConfig * | config () const |
QString | group () const |
void | init (bool forceLocal) |
![]() | |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const QMetaMethod &signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const QMetaMethod &signal) |
bool | isSignalConnected (const QMetaMethod &signal) const const |
int | receivers (const char *signal) const const |
QObject * | sender () const const |
int | senderSignalIndex () const const |
virtual void | timerEvent (QTimerEvent *event) |
Additional Inherited Members | |
![]() | |
objectName | |
![]() | |
typedef | QObjectList |
Detailed Description
Class for managing highscore tables.
This is the KDE class for saving and reading highscore tables. It offers the possibility for system-wide highscore tables (cmake with e.g. -DHIGHSCORE_DIRECTORY=/var/games) and a theoretically unlimited number of entries.
You can specify different "keys" for an entry - just like the KConfig keys. But it will be prefixed with the number of the entry. For example you will probably use something like this to save the name of the player on the top of the list (ie the winner):
Note that it does not really matter if you use "0" or "1" as the first entry of the list as long as your program always uses the same for the first entry. I recommend to use "1", as several convenience methods use this.
You can also specify different groups using setHighscoreGroup. Just like the keys mentioned above the groups behave like groups in KConfig but are prefixed with "KHighscore_". The default group is just "KHighscore". You might use this e.g. to create different highscore tables like
As you can see above you can also use this to write the highscores of a single player, so the "best times" of a player. To write highscores for a specific player in a specific level you will have to use a more complex way:
Also note that you MUST NOT mark the key or the group for translation! I.e. don't use i18n() for the keys or groups! Here is the code to read the above written entry:
Easy, what?
Definition at line 73 of file kgamehighscore.h.
Constructor & Destructor Documentation
◆ KGameHighscore()
|
explicit |
Constructor.
- Parameters
-
forceLocal if true, the local highscore file is used even when the configuration has been set to use a system-wide file. This is convenient for converting highscores from legacy applications. parent parent widget for this widget
Definition at line 59 of file kgamehighscore.cpp.
◆ ~KGameHighscore()
|
override |
Destructor.
If necessary, write and unlock the highscore file.
Definition at line 191 of file kgamehighscore.cpp.
Member Function Documentation
◆ config()
|
protected |
- Returns
- A pointer to the KConfig object to be used. This is either KGlobal::config() (default) or a KSimpleConfig object for a system-wide highscore file.
Definition at line 196 of file kgamehighscore.cpp.
◆ group()
|
protected |
- Returns
- A groupname to be used in KConfig. Used internally to prefix the value from highscoreGroup() with "KHighscore_"
Definition at line 306 of file kgamehighscore.cpp.
◆ groupList()
QStringList KGameHighscore::groupList | ( | ) | const |
Returns a list of group names without the KHighscore_ prexix.
E.g, "KHighscore", "KHighscore_Easy", "KHighscore_Medium" will return "", "Easy", "Medium"
- Returns
- A list of highscore groups.
Definition at line 288 of file kgamehighscore.cpp.
◆ hasEntry()
bool KGameHighscore::hasEntry | ( | int | entry, |
const QString & | key ) const |
- Returns
- True if the highscore table contains the entry/key pair, otherwise false
Definition at line 248 of file kgamehighscore.cpp.
◆ hasTable()
bool KGameHighscore::hasTable | ( | ) | const |
You can use this function to indicate whether KGameHighscore created a highscore table before and - if not - read your old (non-KGameHighscore) table instead.
This way you can safely read an old table and save it using KGameHighscore without losing any data
- Returns
- Whether a highscore table exists.
Definition at line 315 of file kgamehighscore.cpp.
◆ highscoreGroup()
QString KGameHighscore::highscoreGroup | ( | ) | const |
- Returns
- The currently used group. This doesn't contain the prefix ("KHighscore_") but the same as setHighscoreGroup uses. The default is QString()
Definition at line 281 of file kgamehighscore.cpp.
◆ init() [1/2]
|
protected |
Definition at line 66 of file kgamehighscore.cpp.
◆ init() [2/2]
|
static |
This method open the system-wide highscore file using the effective group id of the game executable (which should be "games").
The effective group id is completely dropped afterwards.
Note: this method should be called in main() before creating a KApplication and doing anything else (KApplication checks that the program is not suid/sgid and will exit the program for security reason if it is the case).
Definition at line 99 of file kgamehighscore.cpp.
◆ isLocked()
bool KGameHighscore::isLocked | ( | ) | const |
- Returns
- true if the highscore file is locked or if a local file is used.
Definition at line 84 of file kgamehighscore.cpp.
◆ lockForWriting()
bool KGameHighscore::lockForWriting | ( | QWidget * | widget = nullptr | ) |
Lock the system-wide highscore file for writing (does nothing and return true if the local file is used).
You should perform writing without GUI interaction to avoid blocking and don't forget to unlock the file as soon as possible with writeAndUnlock().
If the config file cannot be locked, the method waits for 1 second and, if it failed again, displays a message box asking for retry or cancel.
- Parameters
-
widget used as the parent of the message box.
- Returns
- false on error or if the config file is locked by another process. In such case, the config stays read-only.
Definition at line 135 of file kgamehighscore.cpp.
◆ readCurrentConfig()
void KGameHighscore::readCurrentConfig | ( | ) |
Read the current state of the highscore file.
Remember that when it's not locked for writing, this file can change at any time. (This method is only useful for a system-wide highscore file).
Definition at line 91 of file kgamehighscore.cpp.
◆ readEntry()
QString KGameHighscore::readEntry | ( | int | entry, |
const QString & | key, | ||
const QString & | pDefault = QString() ) const |
Reads an entry from the highscore table.
- Parameters
-
entry The number of the entry / the placing to be read key The key of the entry. E.g. "name" for the name of the player. Nearly the same as the usual keys in KConfig - but they are prefixed with the entry number pDefault This will be used as default value if the key+pair entry can't be found.
- Returns
- The value of this entry+key pair or pDefault if the entry+key pair doesn't exist
Definition at line 234 of file kgamehighscore.cpp.
◆ readList()
QStringList KGameHighscore::readList | ( | const QString & | key, |
int | lastEntry = 20 ) const |
Reads a list of entries from the highscore table starting at 1 until lastEntry.
If an entry between those numbers doesn't exist the function aborts reading even if after the missing entry is an existing one. The first entry of the list is the first placing, the last on is the last placing.
- Returns
- A list of the entries of this key. You could also call readEntry(i, key) where i is from 1 to 20. Note that this function depends on "1" as the first entry!
- Parameters
-
key The key of the entry. E.g. "name" for the name of the player. Nearly the same as the usual keys in KConfig - but they are prefixed with the entry number lastEntry the last entry which will be includes into the list. 1 will include a list with maximal 1 entry - 20 a list with maximal 20 entries. If lastEntry is <= 0 then rading is only stopped when when an entry does not exist.
Definition at line 255 of file kgamehighscore.cpp.
◆ readNumEntry()
int KGameHighscore::readNumEntry | ( | int | entry, |
const QString & | key, | ||
int | pDefault = -1 ) const |
Read a numeric value.
- Parameters
-
entry The number of the entry / the placing to be read key The key of the entry. E.g. "name" for the name of the player. Nearly the same as the usual keys in KConfig - but they are prefixed with the entry number pDefault This will be used as default value if the key+pair entry can't be found.
- Returns
- The value of this entry+key pair or pDefault if the entry+key pair doesn't exist
Definition at line 241 of file kgamehighscore.cpp.
◆ readPropertyEntry()
QVariant KGameHighscore::readPropertyEntry | ( | int | entry, |
const QString & | key, | ||
const QVariant & | pDefault ) const |
Read a QVariant entry.
See KConfigBase documentation for allowed QVariant::Type.
- Returns
- the value of this entry+key pair or pDefault if the entry+key pair doesn't exist or
Definition at line 227 of file kgamehighscore.cpp.
◆ setHighscoreGroup()
Set the new highscore group.
The group is being prefixed with "KHighscore_" in the table.
- Parameters
-
groupname The new groupname. E.g. use "easy" for the easy level of your game. If you use QString() (the default) the default group is used.
Definition at line 274 of file kgamehighscore.cpp.
◆ writeAndUnlock()
void KGameHighscore::writeAndUnlock | ( | ) |
Effectively write and unlock the system-wide highscore file (.
- See also
- lockForWriting). If using a local highscore file, it will sync the config.
Definition at line 175 of file kgamehighscore.cpp.
◆ writeEntry() [1/3]
- Parameters
-
entry The number of the entry / the placing of the player key A key for this entry. E.g. "name" for the name of the player. Nearly the same as the usual keys in KConfig - but they are prefixed with the entry number value The value of this entry
Definition at line 219 of file kgamehighscore.cpp.
◆ writeEntry() [2/3]
This is an overloaded member function, provided for convenience.
It differs from the above function only in what argument(s) it accepts. See KConfigBase documentation for allowed QVariant::Type.
Definition at line 203 of file kgamehighscore.cpp.
◆ writeEntry() [3/3]
void KGameHighscore::writeEntry | ( | int | entry, |
const QString & | key, | ||
int | value ) |
This is an overloaded member function, provided for convenience.
It differs from the above function only in what argument(s) it accepts.
Definition at line 211 of file kgamehighscore.cpp.
◆ writeList()
void KGameHighscore::writeList | ( | const QString & | key, |
const QStringList & | list ) |
Writes a list of entries to the highscore table.
The first entry is prefixed with "1". Using this method is a short way of calling writeEntry(i, key, list[i]) from i = 1 to list.count()
- Parameters
-
key A key for the entry. E.g. "name" for the name of the player. Nearly the same as the usual keys in KConfig - but they are prefixed with the entry number list The list of values
Definition at line 267 of file kgamehighscore.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2025 The KDE developers.
Generated on Fri Mar 28 2025 11:56:01 by doxygen 1.13.2 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.