KGameRenderedGraphicsObject
#include <KGameRenderedGraphicsObject>
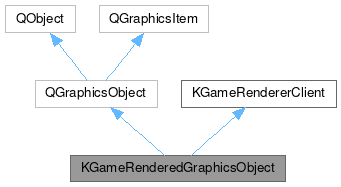
Properties | |
int | frame |
![]() | |
effect | |
enabled | |
opacity | |
parent | |
pos | |
rotation | |
scale | |
transformOriginPoint | |
visible | |
x | |
y | |
z | |
![]() | |
objectName | |
Public Member Functions | |
KGameRenderedGraphicsObject (KGameGraphicsViewRenderer *renderer, const QString &spriteKey, QGraphicsItem *parent=nullptr) | |
QRectF | boundingRect () const override |
bool | contains (const QPointF &point) const override |
QSizeF | fixedSize () const |
bool | isObscuredBy (const QGraphicsItem *item) const override |
QPointF | offset () const |
QPainterPath | opaqueArea () const override |
void | paint (QPainter *painter, const QStyleOptionGraphicsItem *option, QWidget *widget=nullptr) override |
QGraphicsView * | primaryView () const |
void | setFixedSize (QSizeF size) |
void | setOffset (QPointF offset) |
void | setOffset (qreal x, qreal y) |
void | setPrimaryView (QGraphicsView *view) |
QPainterPath | shape () const override |
![]() | |
QGraphicsObject (QGraphicsItem *parent) | |
void | enabledChanged () |
void | grabGesture (Qt::GestureType gesture, Qt::GestureFlags flags) |
void | opacityChanged () |
void | parentChanged () |
void | rotationChanged () |
void | scaleChanged () |
void | ungrabGesture (Qt::GestureType gesture) |
void | visibleChanged () |
void | xChanged () |
void | yChanged () |
void | zChanged () |
![]() | |
QObject (QObject *parent) | |
QBindable< QString > | bindableObjectName () |
bool | blockSignals (bool block) |
const QObjectList & | children () const const |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) const const |
bool | disconnect (const QObject *receiver, const char *method) const const |
void | dumpObjectInfo () const const |
void | dumpObjectTree () const const |
QList< QByteArray > | dynamicPropertyNames () const const |
virtual bool | eventFilter (QObject *watched, QEvent *event) |
T | findChild (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QRegularExpression &re, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (Qt::FindChildOptions options) const const |
bool | inherits (const char *className) const const |
void | installEventFilter (QObject *filterObj) |
bool | isQuickItemType () const const |
bool | isWidgetType () const const |
bool | isWindowType () const const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const const |
void | moveToThread (QThread *targetThread) |
QString | objectName () const const |
void | objectNameChanged (const QString &objectName) |
QObject * | parent () const const |
QVariant | property (const char *name) const const |
Q_CLASSINFO (Name, Value) | |
Q_EMIT Q_EMIT | |
Q_ENUM (...) | |
Q_ENUM_NS (...) | |
Q_ENUMS (...) | |
Q_FLAG (...) | |
Q_FLAG_NS (...) | |
Q_FLAGS (...) | |
Q_GADGET Q_GADGET | |
Q_GADGET_EXPORT (EXPORT_MACRO) | |
Q_INTERFACES (...) | |
Q_INVOKABLE Q_INVOKABLE | |
Q_MOC_INCLUDE Q_MOC_INCLUDE | |
Q_NAMESPACE Q_NAMESPACE | |
Q_NAMESPACE_EXPORT (EXPORT_MACRO) | |
Q_OBJECT Q_OBJECT | |
Q_PROPERTY (...) | |
Q_REVISION Q_REVISION | |
Q_SET_OBJECT_NAME (Object) | |
Q_SIGNAL Q_SIGNAL | |
Q_SIGNALS Q_SIGNALS | |
Q_SLOT Q_SLOT | |
Q_SLOTS Q_SLOTS | |
T | qobject_cast (const QObject *object) |
T | qobject_cast (QObject *object) |
QT_NO_NARROWING_CONVERSIONS_IN_CONNECT QT_NO_NARROWING_CONVERSIONS_IN_CONNECT | |
void | removeEventFilter (QObject *obj) |
void | setObjectName (const QString &name) |
void | setObjectName (QAnyStringView name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | setProperty (const char *name, QVariant &&value) |
bool | signalsBlocked () const const |
int | startTimer (int interval, Qt::TimerType timerType) |
int | startTimer (std::chrono::milliseconds interval, Qt::TimerType timerType) |
QThread * | thread () const const |
![]() | |
QGraphicsItem (QGraphicsItem *parent) | |
bool | acceptDrops () const const |
Qt::MouseButtons | acceptedMouseButtons () const const |
bool | acceptHoverEvents () const const |
bool | acceptTouchEvents () const const |
virtual void | advance (int phase) |
QRegion | boundingRegion (const QTransform &itemToDeviceTransform) const const |
qreal | boundingRegionGranularity () const const |
CacheMode | cacheMode () const const |
QList< QGraphicsItem * > | childItems () const const |
QRectF | childrenBoundingRect () const const |
void | clearFocus () |
QPainterPath | clipPath () const const |
virtual bool | collidesWithItem (const QGraphicsItem *other, Qt::ItemSelectionMode mode) const const |
virtual bool | collidesWithPath (const QPainterPath &path, Qt::ItemSelectionMode mode) const const |
QList< QGraphicsItem * > | collidingItems (Qt::ItemSelectionMode mode) const const |
QGraphicsItem * | commonAncestorItem (const QGraphicsItem *other) const const |
QCursor | cursor () const const |
QVariant | data (int key) const const |
QTransform | deviceTransform (const QTransform &viewportTransform) const const |
qreal | effectiveOpacity () const const |
void | ensureVisible (const QRectF &rect, int xmargin, int ymargin) |
void | ensureVisible (qreal x, qreal y, qreal w, qreal h, int xmargin, int ymargin) |
bool | filtersChildEvents () const const |
GraphicsItemFlags | flags () const const |
QGraphicsItem * | focusItem () const const |
QGraphicsItem * | focusProxy () const const |
void | grabKeyboard () |
void | grabMouse () |
QGraphicsEffect * | graphicsEffect () const const |
QGraphicsItemGroup * | group () const const |
bool | handlesChildEvents () const const |
bool | hasCursor () const const |
bool | hasFocus () const const |
void | hide () |
Qt::InputMethodHints | inputMethodHints () const const |
void | installSceneEventFilter (QGraphicsItem *filterItem) |
bool | isActive () const const |
bool | isAncestorOf (const QGraphicsItem *child) const const |
bool | isBlockedByModalPanel (QGraphicsItem **blockingPanel) const const |
bool | isClipped () const const |
bool | isEnabled () const const |
bool | isObscured (const QRectF &rect) const const |
bool | isObscured (qreal x, qreal y, qreal w, qreal h) const const |
bool | isPanel () const const |
bool | isSelected () const const |
bool | isUnderMouse () const const |
bool | isVisible () const const |
bool | isVisibleTo (const QGraphicsItem *parent) const const |
bool | isWidget () const const |
bool | isWindow () const const |
QTransform | itemTransform (const QGraphicsItem *other, bool *ok) const const |
QPainterPath | mapFromItem (const QGraphicsItem *item, const QPainterPath &path) const const |
QPointF | mapFromItem (const QGraphicsItem *item, const QPointF &point) const const |
QPolygonF | mapFromItem (const QGraphicsItem *item, const QPolygonF &polygon) const const |
QPolygonF | mapFromItem (const QGraphicsItem *item, const QRectF &rect) const const |
QPointF | mapFromItem (const QGraphicsItem *item, qreal x, qreal y) const const |
QPolygonF | mapFromItem (const QGraphicsItem *item, qreal x, qreal y, qreal w, qreal h) const const |
QPainterPath | mapFromParent (const QPainterPath &path) const const |
QPointF | mapFromParent (const QPointF &point) const const |
QPolygonF | mapFromParent (const QPolygonF &polygon) const const |
QPolygonF | mapFromParent (const QRectF &rect) const const |
QPointF | mapFromParent (qreal x, qreal y) const const |
QPolygonF | mapFromParent (qreal x, qreal y, qreal w, qreal h) const const |
QPainterPath | mapFromScene (const QPainterPath &path) const const |
QPointF | mapFromScene (const QPointF &point) const const |
QPolygonF | mapFromScene (const QPolygonF &polygon) const const |
QPolygonF | mapFromScene (const QRectF &rect) const const |
QPointF | mapFromScene (qreal x, qreal y) const const |
QPolygonF | mapFromScene (qreal x, qreal y, qreal w, qreal h) const const |
QRectF | mapRectFromItem (const QGraphicsItem *item, const QRectF &rect) const const |
QRectF | mapRectFromItem (const QGraphicsItem *item, qreal x, qreal y, qreal w, qreal h) const const |
QRectF | mapRectFromParent (const QRectF &rect) const const |
QRectF | mapRectFromParent (qreal x, qreal y, qreal w, qreal h) const const |
QRectF | mapRectFromScene (const QRectF &rect) const const |
QRectF | mapRectFromScene (qreal x, qreal y, qreal w, qreal h) const const |
QRectF | mapRectToItem (const QGraphicsItem *item, const QRectF &rect) const const |
QRectF | mapRectToItem (const QGraphicsItem *item, qreal x, qreal y, qreal w, qreal h) const const |
QRectF | mapRectToParent (const QRectF &rect) const const |
QRectF | mapRectToParent (qreal x, qreal y, qreal w, qreal h) const const |
QRectF | mapRectToScene (const QRectF &rect) const const |
QRectF | mapRectToScene (qreal x, qreal y, qreal w, qreal h) const const |
QPainterPath | mapToItem (const QGraphicsItem *item, const QPainterPath &path) const const |
QPointF | mapToItem (const QGraphicsItem *item, const QPointF &point) const const |
QPolygonF | mapToItem (const QGraphicsItem *item, const QPolygonF &polygon) const const |
QPolygonF | mapToItem (const QGraphicsItem *item, const QRectF &rect) const const |
QPointF | mapToItem (const QGraphicsItem *item, qreal x, qreal y) const const |
QPolygonF | mapToItem (const QGraphicsItem *item, qreal x, qreal y, qreal w, qreal h) const const |
QPainterPath | mapToParent (const QPainterPath &path) const const |
QPointF | mapToParent (const QPointF &point) const const |
QPolygonF | mapToParent (const QPolygonF &polygon) const const |
QPolygonF | mapToParent (const QRectF &rect) const const |
QPointF | mapToParent (qreal x, qreal y) const const |
QPolygonF | mapToParent (qreal x, qreal y, qreal w, qreal h) const const |
QPainterPath | mapToScene (const QPainterPath &path) const const |
QPointF | mapToScene (const QPointF &point) const const |
QPolygonF | mapToScene (const QPolygonF &polygon) const const |
QPolygonF | mapToScene (const QRectF &rect) const const |
QPointF | mapToScene (qreal x, qreal y) const const |
QPolygonF | mapToScene (qreal x, qreal y, qreal w, qreal h) const const |
void | moveBy (qreal dx, qreal dy) |
qreal | opacity () const const |
QGraphicsItem * | panel () const const |
PanelModality | panelModality () const const |
QGraphicsItem * | parentItem () const const |
QGraphicsObject * | parentObject () const const |
QGraphicsWidget * | parentWidget () const const |
QPointF | pos () const const |
T | qgraphicsitem_cast (QGraphicsItem *item) |
void | removeSceneEventFilter (QGraphicsItem *filterItem) |
void | resetTransform () |
qreal | rotation () const const |
qreal | scale () const const |
QGraphicsScene * | scene () const const |
QRectF | sceneBoundingRect () const const |
QPointF | scenePos () const const |
QTransform | sceneTransform () const const |
void | scroll (qreal dx, qreal dy, const QRectF &rect) |
void | setAcceptDrops (bool on) |
void | setAcceptedMouseButtons (Qt::MouseButtons buttons) |
void | setAcceptHoverEvents (bool enabled) |
void | setAcceptTouchEvents (bool enabled) |
void | setActive (bool active) |
void | setBoundingRegionGranularity (qreal granularity) |
void | setCacheMode (CacheMode mode, const QSize &logicalCacheSize) |
void | setCursor (const QCursor &cursor) |
void | setData (int key, const QVariant &value) |
void | setEnabled (bool enabled) |
void | setFiltersChildEvents (bool enabled) |
void | setFlag (GraphicsItemFlag flag, bool enabled) |
void | setFlags (GraphicsItemFlags flags) |
void | setFocus (Qt::FocusReason focusReason) |
void | setFocusProxy (QGraphicsItem *item) |
void | setGraphicsEffect (QGraphicsEffect *effect) |
void | setGroup (QGraphicsItemGroup *group) |
void | setHandlesChildEvents (bool enabled) |
void | setInputMethodHints (Qt::InputMethodHints hints) |
void | setOpacity (qreal opacity) |
void | setPanelModality (PanelModality panelModality) |
void | setParentItem (QGraphicsItem *newParent) |
void | setPos (const QPointF &pos) |
void | setPos (qreal x, qreal y) |
void | setRotation (qreal angle) |
void | setScale (qreal factor) |
void | setSelected (bool selected) |
void | setToolTip (const QString &toolTip) |
void | setTransform (const QTransform &matrix, bool combine) |
void | setTransformations (const QList< QGraphicsTransform * > &transformations) |
void | setTransformOriginPoint (const QPointF &origin) |
void | setTransformOriginPoint (qreal x, qreal y) |
void | setVisible (bool visible) |
void | setX (qreal x) |
void | setY (qreal y) |
void | setZValue (qreal z) |
void | show () |
void | stackBefore (const QGraphicsItem *sibling) |
QGraphicsObject * | toGraphicsObject () |
const QGraphicsObject * | toGraphicsObject () const const |
QString | toolTip () const const |
QGraphicsItem * | topLevelItem () const const |
QGraphicsWidget * | topLevelWidget () const const |
QTransform | transform () const const |
QList< QGraphicsTransform * > | transformations () const const |
QPointF | transformOriginPoint () const const |
virtual int | type () const const |
void | ungrabKeyboard () |
void | ungrabMouse () |
void | unsetCursor () |
void | update (const QRectF &rect) |
void | update (qreal x, qreal y, qreal width, qreal height) |
QGraphicsWidget * | window () const const |
qreal | x () const const |
qreal | y () const const |
qreal | zValue () const const |
![]() | |
KGameRendererClient (KGameRenderer *renderer, const QString &spriteKey) | |
QHash< QColor, QColor > | customColors () const |
int | frame () const |
int | frameCount () const |
KGameRenderer * | renderer () const |
QSize | renderSize () const |
void | setCustomColors (const QHash< QColor, QColor > &customColors) |
void | setFrame (int frame) |
void | setRenderSize (QSize renderSize) |
void | setSpriteKey (const QString &spriteKey) |
QString | spriteKey () const |
Detailed Description
A QGraphicsObject which displays pixmaps from a KGameRenderer.
This item displays a pixmap which is retrieved from a KGameRenderer, and is updated automatically when the KGameRenderer changes the theme.
The item has built-in handling for animated sprites (i.e. those with multiple frames). It is a QGraphicsObject and exposes a "frame" property, so you can easily run the animation by plugging in a QPropertyAnimation.
Modes of operation
By default, this item behaves just like a QGraphicsPixmapItem. The size of its bounding rect is equal to the size of the pixmap, i.e. the renderSize().
However, the KGameRenderedGraphicsObject has a second mode of operation, which is enabled by setting a "primary view". (This can be done automatically via KGameGraphicsViewRenderer::setDefaultPrimaryView.)
If such a primary view is set, the following happens:
- The renderSize of the pixmap is automatically determined from the painting requests received from the primary view (manual calls to setRenderSize() are unnecessary and need to be avoided).
- The size of the item's boundingRect() is independent of the renderSize(). The default fixedSize() is 1x1, which means that the item's bounding rect is the unit square (moved by the configured offset()).
- Since
- 4.6
Definition at line 52 of file kgamerenderedgraphicsobject.h.
Property Documentation
◆ frame
|
readwrite |
Definition at line 55 of file kgamerenderedgraphicsobject.h.
Constructor & Destructor Documentation
◆ KGameRenderedGraphicsObject()
KGameRenderedGraphicsObject::KGameRenderedGraphicsObject | ( | KGameGraphicsViewRenderer * | renderer, |
const QString & | spriteKey, | ||
QGraphicsItem * | parent = nullptr ) |
Creates a new KGameRenderedGraphicsObject which renders the sprite with the given spriteKey as provided by the given renderer.
Definition at line 84 of file kgamerenderedgraphicsobject.cpp.
Member Function Documentation
◆ boundingRect()
|
overridevirtual |
Implements QGraphicsItem.
Definition at line 187 of file kgamerenderedgraphicsobject.cpp.
◆ contains()
|
overridevirtual |
Reimplemented from QGraphicsItem.
Definition at line 194 of file kgamerenderedgraphicsobject.cpp.
◆ fixedSize()
QSizeF KGameRenderedGraphicsObject::fixedSize | ( | ) | const |
- Returns
- the fixed size of this item (or (-1, -1) if this item has no primary view)
Definition at line 117 of file kgamerenderedgraphicsobject.cpp.
◆ isObscuredBy()
|
overridevirtual |
Reimplemented from QGraphicsItem.
Definition at line 201 of file kgamerenderedgraphicsobject.cpp.
◆ offset()
QPointF KGameRenderedGraphicsObject::offset | ( | ) | const |
- Returns
- the item's offset, which defines the point of the top-left corner of the bounding rect, in local coordinates.
Definition at line 94 of file kgamerenderedgraphicsobject.cpp.
◆ opaqueArea()
|
overridevirtual |
Reimplemented from QGraphicsItem.
Definition at line 208 of file kgamerenderedgraphicsobject.cpp.
◆ paint()
|
overridevirtual |
Implements QGraphicsItem.
Definition at line 215 of file kgamerenderedgraphicsobject.cpp.
◆ primaryView()
QGraphicsView * KGameRenderedGraphicsObject::primaryView | ( | ) | const |
Returns a pointer to the current primary view, or 0 if no primary view has been set (which is the default).
- See also
- setPrimaryView()
Definition at line 134 of file kgamerenderedgraphicsobject.cpp.
◆ receivePixmap()
|
overrideprotectedvirtual |
This method is called when the KGameRenderer has provided a new pixmap for this client (esp.
after theme changes and after calls to setFrame(), setRenderSize() and setSpriteKey()).
Implements KGameRendererClient.
Definition at line 164 of file kgamerenderedgraphicsobject.cpp.
◆ setFixedSize()
void KGameRenderedGraphicsObject::setFixedSize | ( | QSizeF | size | ) |
Sets the fixed size of this item, i.e.
the guaranteed size of the item. This works only when a primary view has been set.
Definition at line 124 of file kgamerenderedgraphicsobject.cpp.
◆ setOffset() [1/2]
void KGameRenderedGraphicsObject::setOffset | ( | QPointF | offset | ) |
Sets the item's offset, which defines the point of the top-left corner of the bounding rect, in local coordinates.
Definition at line 101 of file kgamerenderedgraphicsobject.cpp.
◆ setOffset() [2/2]
void KGameRenderedGraphicsObject::setOffset | ( | qreal | x, |
qreal | y ) |
This is an overloaded member function, provided for convenience. It differs from the above function only in what argument(s) it accepts.
Definition at line 112 of file kgamerenderedgraphicsobject.cpp.
◆ setPrimaryView()
void KGameRenderedGraphicsObject::setPrimaryView | ( | QGraphicsView * | view | ) |
Sets the primary view of this item.
(See class documentation for what the primary view does.) Pass a null pointer to just disconnect from the current primary view. The fixed size is then reset to (-1, -1). If a primary view is set, the fixed size is initialized to (1, 1).
- Warning
- While a primary view is set, avoid any manual calls to setRenderSize().
- See also
- {Modes of operation}
Definition at line 141 of file kgamerenderedgraphicsobject.cpp.
◆ shape()
|
overridevirtual |
Reimplemented from QGraphicsItem.
Definition at line 222 of file kgamerenderedgraphicsobject.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2025 The KDE developers.
Generated on Fri May 2 2025 11:57:56 by doxygen 1.13.2 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.