KGameRenderer
#include <KGameRenderer>
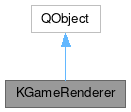
Public Types | |
typedef QFlags< Strategy > | Strategies |
enum | Strategy { UseDiskCache = 1 << 0 , UseRenderingThreads = 1 << 1 } |
![]() | |
typedef | QObjectList |
Properties | |
const KGameTheme * | theme |
KGameThemeProvider * | themeProvider |
![]() | |
objectName | |
Signals | |
void | readOnlyProperty () |
void | themeChanged (const KGameTheme *theme) |
Public Member Functions | |
KGameRenderer (KGameTheme *theme, unsigned cacheSize=0) | |
KGameRenderer (KGameThemeProvider *prov, unsigned cacheSize=0) | |
~KGameRenderer () override | |
QRectF | boundsOnSprite (const QString &key, int frame=-1) const |
int | frameBaseIndex () const |
int | frameCount (const QString &key) const |
QString | frameSuffix () const |
void | setFrameBaseIndex (int frameBaseIndex) |
void | setFrameSuffix (const QString &suffix) |
void | setStrategyEnabled (Strategy strategy, bool enabled=true) |
bool | spriteExists (const QString &key) const |
QPixmap | spritePixmap (const QString &key, QSize size, int frame=-1, const QHash< QColor, QColor > &customColors=(QHash< QColor, QColor >())) const |
Strategies | strategies () const |
const KGameTheme * | theme () const |
KGameThemeProvider * | themeProvider () const |
![]() | |
QObject (QObject *parent) | |
QBindable< QString > | bindableObjectName () |
bool | blockSignals (bool block) |
const QObjectList & | children () const const |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) const const |
bool | disconnect (const QObject *receiver, const char *method) const const |
void | dumpObjectInfo () const const |
void | dumpObjectTree () const const |
QList< QByteArray > | dynamicPropertyNames () const const |
virtual bool | event (QEvent *e) |
virtual bool | eventFilter (QObject *watched, QEvent *event) |
T | findChild (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QRegularExpression &re, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (Qt::FindChildOptions options) const const |
bool | inherits (const char *className) const const |
void | installEventFilter (QObject *filterObj) |
bool | isQuickItemType () const const |
bool | isWidgetType () const const |
bool | isWindowType () const const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const const |
void | moveToThread (QThread *targetThread) |
QString | objectName () const const |
void | objectNameChanged (const QString &objectName) |
QObject * | parent () const const |
QVariant | property (const char *name) const const |
Q_CLASSINFO (Name, Value) | |
Q_EMIT Q_EMIT | |
Q_ENUM (...) | |
Q_ENUM_NS (...) | |
Q_ENUMS (...) | |
Q_FLAG (...) | |
Q_FLAG_NS (...) | |
Q_FLAGS (...) | |
Q_GADGET Q_GADGET | |
Q_GADGET_EXPORT (EXPORT_MACRO) | |
Q_INTERFACES (...) | |
Q_INVOKABLE Q_INVOKABLE | |
Q_MOC_INCLUDE Q_MOC_INCLUDE | |
Q_NAMESPACE Q_NAMESPACE | |
Q_NAMESPACE_EXPORT (EXPORT_MACRO) | |
Q_OBJECT Q_OBJECT | |
Q_PROPERTY (...) | |
Q_REVISION Q_REVISION | |
Q_SET_OBJECT_NAME (Object) | |
Q_SIGNAL Q_SIGNAL | |
Q_SIGNALS Q_SIGNALS | |
Q_SLOT Q_SLOT | |
Q_SLOTS Q_SLOTS | |
T | qobject_cast (const QObject *object) |
T | qobject_cast (QObject *object) |
QT_NO_NARROWING_CONVERSIONS_IN_CONNECT QT_NO_NARROWING_CONVERSIONS_IN_CONNECT | |
void | removeEventFilter (QObject *obj) |
void | setObjectName (const QString &name) |
void | setObjectName (QAnyStringView name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | setProperty (const char *name, QVariant &&value) |
bool | signalsBlocked () const const |
int | startTimer (int interval, Qt::TimerType timerType) |
int | startTimer (std::chrono::milliseconds interval, Qt::TimerType timerType) |
QThread * | thread () const const |
Additional Inherited Members | |
![]() | |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *context, Functor functor, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, Functor functor) |
bool | disconnect (const QMetaObject::Connection &connection) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
bool | disconnect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
![]() | |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const QMetaMethod &signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const QMetaMethod &signal) |
bool | isSignalConnected (const QMetaMethod &signal) const const |
int | receivers (const char *signal) const const |
QObject * | sender () const const |
int | senderSignalIndex () const const |
virtual void | timerEvent (QTimerEvent *event) |
Detailed Description
Cache-enabled rendering of SVG themes.
KGameRenderer is a light-weight rendering framework for the rendering of SVG themes (as represented by KGameTheme) into pixmap caches.
Terminology
- Themes in the context of KGameRenderer are KGameTheme instances. The theme selection by a KGameRenderer can be managed by a KGameThemeProvider.
- A sprite is either a single pixmap ("non-animated sprites") or a sequence of pixmaps which are shown consecutively to produce an animation ("animated sprites"). Non-animated sprites correspond to a single element with the same key in the SVG theme file. The element keys for the pixmaps of an animated sprite are produced by appending the frameSuffix() to the sprite key.
Access to the pixmaps
Sprite pixmaps can be retrieved from KGameRenderer in the main thread using the synchronous KGameRenderer::spritePixmap() method. However, it is highly recommended to use the asynchronous interface provided by the interface class KGameRendererClient. A client corresponds to one pixmap and registers itself with the corresponding KGameRenderer instance to get notified when a new pixmap is available.
For QGraphicsView-based applications, the KGameRenderedItem class provides a QGraphicsPixmapItem which is a KGameRendererClient and displays the pixmap for a given sprite.
Rendering strategy
For each theme, KGameRenderer keeps two caches around: an in-process cache of QPixmaps, and a disk cache containing QImages (powered by KImageCache). You therefore will not need to implement any caching for the pixmaps provided by KGameRenderer.
When requests from a KGameRendererClient cannot be served immediately because the requested sprite is not in the caches, a rendering request is sent to a worker thread.
Support for legacy themes
When porting applications to KGameRenderer, you probably have to support the format of existing themes. KGameRenderer provides the frameBaseIndex() and frameSuffix() properties for this purpose. It is recommended not to change these properties in new applications.
- Since
- 4.6
Definition at line 83 of file kgamerenderer.h.
Member Typedef Documentation
◆ Strategies
Stores a combination of Strategy values.
Definition at line 107 of file kgamerenderer.h.
Member Enumeration Documentation
◆ Strategy
Describes the various strategies which KGameRenderer can use to speed up rendering.
- See also
- setStrategyEnabled
Enumerator | |
---|---|
UseDiskCache | If set, pixmaps will be cached in a shared disk cache (using KSharedDataCache). This is especially useful for complex SVG themes because KGameRenderer will not load the SVG if all needed pixmaps are available from the disk cache. |
UseRenderingThreads | If set, pixmap requests from KGameRendererClients will be handled asynchronously if possible. This is especially useful when many clients are requesting complex pixmaps at one time. |
Definition at line 93 of file kgamerenderer.h.
Property Documentation
◆ theme
|
read |
Definition at line 86 of file kgamerenderer.h.
◆ themeProvider
|
read |
Definition at line 87 of file kgamerenderer.h.
Constructor & Destructor Documentation
◆ KGameRenderer() [1/2]
|
explicit |
Constructs a new KGameRenderer that renders prov->currentTheme().
- Parameters
-
prov the theme provider cacheSize the cache size in megabytes (if not given, a sane default is used)
- Warning
- This constructor may only be called from the main thread.
Definition at line 49 of file kgamerenderer.cpp.
◆ KGameRenderer() [2/2]
|
explicit |
overload that allows to use KGameRenderer without a theme provider (useful when there is only one theme)
- Note
- Takes ownership of theme.
Definition at line 68 of file kgamerenderer.cpp.
◆ ~KGameRenderer()
|
override |
Deletes this KGameRenderer instance, as well as all clients using it.
Definition at line 73 of file kgamerenderer.cpp.
Member Function Documentation
◆ boundsOnSprite()
- Returns
- the bounding rectangle of the sprite with this key This is equal to QSvgRenderer::boundsOnElement() of the corresponding SVG element.
Definition at line 315 of file kgamerenderer.cpp.
◆ frameBaseIndex()
int KGameRenderer::frameBaseIndex | ( | ) | const |
- Returns
- the frame base index.
- See also
- setFrameBaseIndex()
Definition at line 86 of file kgamerenderer.cpp.
◆ frameCount()
int KGameRenderer::frameCount | ( | const QString & | key | ) | const |
- Returns
- the count of frames available for the sprite with this key If this sprite is not animated (i.e. there are no SVG elements for any frames), this method returns 0. If the sprite does not exist at all, -1 is returned.
If the sprite is animated, the method counts frames starting at zero (unless you change the frameBaseIndex()), and returns the number of frames for which corresponding elements exist in the SVG file.
For example, if the SVG contains the elements "foo_0", "foo_1" and "foo_3", frameCount("foo") returns 2 for the default frame suffix. (The element "foo_3" is ignored because "foo_2" is missing.)
Definition at line 266 of file kgamerenderer.cpp.
◆ frameSuffix()
QString KGameRenderer::frameSuffix | ( | ) | const |
◆ readOnlyProperty
|
signal |
This signal is never emitted.
It is provided because QML likes to complain about properties without NOTIFY signals, even readonly ones.
◆ setFrameBaseIndex()
void KGameRenderer::setFrameBaseIndex | ( | int | frameBaseIndex | ) |
Sets the frame base index, i.e.
the lowest frame index. Usually, frame numbering starts at zero, so the frame base index is zero.
For example, if you set the frame base index to 42, and use the default frame suffix, the 3 frames of an animated sprite "foo" are provided by the SVG elements "foo_42", "foo_43" and "foo_44".
It is recommended not to alter the frame base index unless you need to support legacy themes.
Definition at line 93 of file kgamerenderer.cpp.
◆ setFrameSuffix()
void KGameRenderer::setFrameSuffix | ( | const QString & | suffix | ) |
Sets the frame suffix.
This suffix will be added to a sprite key to create the corresponding SVG element key, after any occurrence of "%1" in the suffix has been replaced by the frame number.
- Note
- Giving a suffix which does not include "%1" will reset to the default suffix "_%1".
For example, if the frame suffix is set to "_%1" (the default), the SVG element key for the frame no. 23 of the sprite "foo" is "foo_23".
- Note
- Frame numbering starts at zero unless you setFrameBaseIndex().
Definition at line 107 of file kgamerenderer.cpp.
◆ setStrategyEnabled()
void KGameRenderer::setStrategyEnabled | ( | KGameRenderer::Strategy | strategy, |
bool | enabled = true ) |
Enables/disables an optimization strategy for this renderer.
By default, both the UseDiskCache and the UseRenderingThreads strategies are enabled. This is a sane default for 99% of all games. You might only want to disable optimizations if the graphics are so simple that the optimizations create an overhead in your special case.
If you disable UseDiskCache, you should do so before setTheme(), because changes to UseDiskCache cause a full theme reload.
Definition at line 121 of file kgamerenderer.cpp.
◆ spriteExists()
bool KGameRenderer::spriteExists | ( | const QString & | key | ) | const |
- Returns
- if the sprite with the given key exists This is the same as renderer.frameCount(key) >= 0
Definition at line 360 of file kgamerenderer.cpp.
◆ spritePixmap()
QPixmap KGameRenderer::spritePixmap | ( | const QString & | key, |
QSize | size, | ||
int | frame = -1, | ||
const QHash< QColor, QColor > & | customColors = (QHash<QColor, QColor>()) ) const |
- Returns
- a rendered pixmap
- Parameters
-
key the key of the sprite size the size of the resulting pixmap frame the number of the frame which you want customColors the custom color replacements for this client. That is, for each entry in this has, the key color will be replaced by its value if it is encountered in the sprite.
- Note
- For non-animated frames, set frame to -1 or omit it.
- Custom colors increase the rendering time considerably, so use this feature only if you really need its flexibility.
Definition at line 367 of file kgamerenderer.cpp.
◆ strategies()
KGameRenderer::Strategies KGameRenderer::strategies | ( | ) | const |
- Returns
- the optimization strategies used by this renderer
- See also
- setStrategyEnabled()
Definition at line 114 of file kgamerenderer.cpp.
◆ theme()
const KGameTheme * KGameRenderer::theme | ( | ) | const |
- Returns
- the KGameTheme instance used by this renderer
Definition at line 229 of file kgamerenderer.cpp.
◆ themeProvider()
KGameThemeProvider * KGameRenderer::themeProvider | ( | ) | const |
- Returns
- the KGameThemeProvider instance used by this renderer, or 0 if the renderer was created with a single static theme
Definition at line 240 of file kgamerenderer.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2025 The KDE developers.
Generated on Fri Jan 3 2025 11:46:49 by doxygen 1.12.0 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.