KGameThemeProvider
#include <KGameThemeProvider>
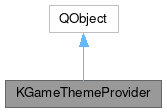
Properties | |
const KGameTheme * | currentTheme |
QString | currentThemeName |
QString | name |
![]() | |
objectName | |
Signals | |
void | currentThemeChanged (const KGameTheme *theme) |
void | currentThemeNameChanged (const QString &themeName) |
void | nameChanged (const QString &name) |
Public Slots | |
void | setCurrentTheme (const KGameTheme *theme) |
Public Member Functions | |
KGameThemeProvider (const QByteArray &configKey=QByteArrayLiteral("Theme"), QObject *parent=nullptr) | |
~KGameThemeProvider () override | |
void | addTheme (KGameTheme *theme) |
const KGameTheme * | currentTheme () const |
QString | currentThemeName () const |
const KGameTheme * | defaultTheme () const |
void | discoverThemes (const QString &directory, const QString &defaultThemeName=QStringLiteral("default"), const QMetaObject *themeClass=nullptr) |
virtual QPixmap | generatePreview (const KGameTheme *theme, QSize size) |
QString | name () const |
void | rediscoverThemes () |
void | setDeclarativeEngine (const QString &name, QQmlEngine *engine) |
void | setDefaultTheme (const KGameTheme *theme) |
QList< const KGameTheme * > | themes () const |
![]() | |
QObject (QObject *parent) | |
QBindable< QString > | bindableObjectName () |
bool | blockSignals (bool block) |
const QObjectList & | children () const const |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) const const |
bool | disconnect (const QObject *receiver, const char *method) const const |
void | dumpObjectInfo () const const |
void | dumpObjectTree () const const |
QList< QByteArray > | dynamicPropertyNames () const const |
virtual bool | event (QEvent *e) |
virtual bool | eventFilter (QObject *watched, QEvent *event) |
T | findChild (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QRegularExpression &re, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (Qt::FindChildOptions options) const const |
bool | inherits (const char *className) const const |
void | installEventFilter (QObject *filterObj) |
bool | isQuickItemType () const const |
bool | isWidgetType () const const |
bool | isWindowType () const const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const const |
void | moveToThread (QThread *targetThread) |
QString | objectName () const const |
void | objectNameChanged (const QString &objectName) |
QObject * | parent () const const |
QVariant | property (const char *name) const const |
Q_CLASSINFO (Name, Value) | |
Q_EMIT Q_EMIT | |
Q_ENUM (...) | |
Q_ENUM_NS (...) | |
Q_ENUMS (...) | |
Q_FLAG (...) | |
Q_FLAG_NS (...) | |
Q_FLAGS (...) | |
Q_GADGET Q_GADGET | |
Q_GADGET_EXPORT (EXPORT_MACRO) | |
Q_INTERFACES (...) | |
Q_INVOKABLE Q_INVOKABLE | |
Q_MOC_INCLUDE Q_MOC_INCLUDE | |
Q_NAMESPACE Q_NAMESPACE | |
Q_NAMESPACE_EXPORT (EXPORT_MACRO) | |
Q_OBJECT Q_OBJECT | |
Q_PROPERTY (...) | |
Q_REVISION Q_REVISION | |
Q_SET_OBJECT_NAME (Object) | |
Q_SIGNAL Q_SIGNAL | |
Q_SIGNALS Q_SIGNALS | |
Q_SLOT Q_SLOT | |
Q_SLOTS Q_SLOTS | |
T | qobject_cast (const QObject *object) |
T | qobject_cast (QObject *object) |
QT_NO_NARROWING_CONVERSIONS_IN_CONNECT QT_NO_NARROWING_CONVERSIONS_IN_CONNECT | |
void | removeEventFilter (QObject *obj) |
void | setObjectName (const QString &name) |
void | setObjectName (QAnyStringView name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | setProperty (const char *name, QVariant &&value) |
bool | signalsBlocked () const const |
int | startTimer (int interval, Qt::TimerType timerType) |
int | startTimer (std::chrono::milliseconds interval, Qt::TimerType timerType) |
QThread * | thread () const const |
Additional Inherited Members | |
![]() | |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *context, Functor functor, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, Functor functor) |
bool | disconnect (const QMetaObject::Connection &connection) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
bool | disconnect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
![]() | |
typedef | QObjectList |
![]() | |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const QMetaMethod &signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const QMetaMethod &signal) |
bool | isSignalConnected (const QMetaMethod &signal) const const |
int | receivers (const char *signal) const const |
QObject * | sender () const const |
int | senderSignalIndex () const const |
virtual void | timerEvent (QTimerEvent *event) |
Detailed Description
A theme provider manages KGameTheme instances, and maintains a selection of the currentTheme().
It can automatically coordinate its selection with a KGameRenderer instance.
- Note
- KGameThemeProvider instances store selections in the application config, in the group [KgTheme]. This is documented here because this information is relevant for kconfig_
Definition at line 30 of file kgamethemeprovider.h.
Property Documentation
◆ currentTheme
|
readwrite |
Definition at line 33 of file kgamethemeprovider.h.
◆ currentThemeName
|
read |
Definition at line 35 of file kgamethemeprovider.h.
◆ name
|
read |
Definition at line 34 of file kgamethemeprovider.h.
Constructor & Destructor Documentation
◆ KGameThemeProvider()
|
explicit |
Constructor.
If you don't want KGameThemeProvider to store the current theme selection in the application config file automatically, set configKey to an empty QByteArray.
If there are multiple KGameThemeProvider instances, make sure they use different config keys to avoid collisions.
Definition at line 55 of file kgamethemeprovider.cpp.
◆ ~KGameThemeProvider()
|
override |
Destructor.
Definition at line 67 of file kgamethemeprovider.cpp.
Member Function Documentation
◆ addTheme()
void KGameThemeProvider::addTheme | ( | KGameTheme * | theme | ) |
Adds a theme to this instance.
The theme provider takes ownership of theme.
Definition at line 102 of file kgamethemeprovider.cpp.
◆ currentTheme()
const KGameTheme * KGameThemeProvider::currentTheme | ( | ) | const |
- Returns
- the currently selected theme, or 0 if the provider does not contain any themes
After the KGameThemeProvider instance has been created, the current theme will not be determined until this method is called for the first time. This allows the application developer to set up the theme provider before it restores the theme selection from the configuration file.
Definition at line 136 of file kgamethemeprovider.cpp.
◆ currentThemeChanged
|
signal |
Emitted when the current theme changes.
- See also
- setCurrentTheme
◆ currentThemeName()
QString KGameThemeProvider::currentThemeName | ( | ) | const |
- Returns
- the name of the current theme
- Since
- 4.11
Definition at line 170 of file kgamethemeprovider.cpp.
◆ currentThemeNameChanged
|
signal |
Emitted when the name of the current theme changes.
- Since
- 4.11
◆ defaultTheme()
const KGameTheme * KGameThemeProvider::defaultTheme | ( | ) | const |
- Returns
- the default theme, or 0 if the provider does not contain any themes
Definition at line 116 of file kgamethemeprovider.cpp.
◆ discoverThemes()
void KGameThemeProvider::discoverThemes | ( | const QString & | directory, |
const QString & | defaultThemeName = QStringLiteral("default"), | ||
const QMetaObject * | themeClass = nullptr ) |
This method reads theme description files from a standard location.
The directory
argument is passed to QStandardPaths like this:
The typical usage is to install theme description files in
and then call:
If a themeClass's
QMetaObject is given, the created themes will be instances of this KGameTheme subclass. The themeClass
must export (with the Q_INVOKABLE marker) a constructor with the same signature as the KGameTheme constructor.
- Since
- 7.4
Definition at line 177 of file kgamethemeprovider.cpp.
◆ generatePreview()
|
virtual |
Generate a preview pixmap for the given theme.
The application will typically want to reimplement this to load the given theme into a KGameRenderer and then arrange some sprites into a preview.
size is the maximal allowed size.
The default implementation tries to load a preview image from KGameTheme::previewPath(), and resizes the result to fit in size.
Definition at line 330 of file kgamethemeprovider.cpp.
◆ name()
QString KGameThemeProvider::name | ( | ) | const |
- Returns
- the name of the KGameThemeProvider object. This name can be used as QML element ID to reference the object inside QML.
- Since
- 4.11
Definition at line 88 of file kgamethemeprovider.cpp.
◆ nameChanged
|
signal |
Emitted when the name of the provider changes.
- Since
- 4.11
◆ rediscoverThemes()
void KGameThemeProvider::rediscoverThemes | ( | ) |
After this provider has been set up with discoverThemes(), this method may be used to read additional themes which were added since the discoverThemes() call.
This is esp. useful for KNewStuff integration.
Definition at line 206 of file kgamethemeprovider.cpp.
◆ setCurrentTheme
|
slot |
Select a new theme.
The given theme must already have been added to this instance.
Definition at line 159 of file kgamethemeprovider.cpp.
◆ setDeclarativeEngine()
void KGameThemeProvider::setDeclarativeEngine | ( | const QString & | name, |
QQmlEngine * | engine ) |
Registers this KGameThemeProvider with engine's root context with ID name and constructs a KGameImageProvider corresponding to this KGameThemeProvider and adds it to the QML engine, also with name, which will receive sprite requests.
- Since
- 4.11
Definition at line 338 of file kgamethemeprovider.cpp.
◆ setDefaultTheme()
void KGameThemeProvider::setDefaultTheme | ( | const KGameTheme * | theme | ) |
- See also
- defaultTheme()
Usually this will be set automatically by discoverThemes(). Call this before the first call to currentTheme(), it won't have any effect afterwards. theme must already have been added to this instance.
Definition at line 123 of file kgamethemeprovider.cpp.
◆ themes()
QList< const KGameTheme * > KGameThemeProvider::themes | ( | ) | const |
- Returns
- the themes in this provider
Definition at line 95 of file kgamethemeprovider.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2025 The KDE developers.
Generated on Fri Mar 28 2025 11:56:01 by doxygen 1.13.2 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.