KMessageClient
#include <KGame/KMessageClient>
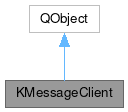
Signals | |
void | aboutToDisconnect (quint32 id) |
void | adminStatusChanged (bool isAdmin) |
void | broadcastReceived (const QByteArray &msg, quint32 senderID) |
void | connectionBroken () |
void | eventClientConnected (quint32 clientID) |
void | eventClientDisconnected (quint32 clientID, bool broken) |
void | forwardReceived (const QByteArray &msg, quint32 senderID, const QList< quint32 > &receivers) |
void | serverMessageReceived (const QByteArray &msg, bool &unknown) |
Public Member Functions | |
KMessageClient (QObject *parent=nullptr) | |
~KMessageClient () override | |
quint32 | adminId () const |
QList< quint32 > | clientList () const |
unsigned int | delayedMessageCount () const |
void | disconnect () |
quint32 | id () const |
bool | isAdmin () const |
bool | isConnected () const |
bool | isNetwork () const |
void | lock () |
QString | peerName () const |
quint16 | peerPort () const |
void | sendBroadcast (const QByteArray &msg) |
void | sendForward (const QByteArray &msg, const QList< quint32 > &clients) |
void | sendForward (const QByteArray &msg, quint32 client) |
void | sendServerMessage (const QByteArray &msg) |
void | setServer (const QString &host, quint16 port) |
virtual void | setServer (KMessageIO *connection) |
void | setServer (KMessageServer *server) |
void | unlock () |
![]() | |
QObject (QObject *parent) | |
QBindable< QString > | bindableObjectName () |
bool | blockSignals (bool block) |
const QObjectList & | children () const const |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) const const |
bool | disconnect (const QObject *receiver, const char *method) const const |
void | dumpObjectInfo () const const |
void | dumpObjectTree () const const |
QList< QByteArray > | dynamicPropertyNames () const const |
virtual bool | event (QEvent *e) |
virtual bool | eventFilter (QObject *watched, QEvent *event) |
T | findChild (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QRegularExpression &re, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (Qt::FindChildOptions options) const const |
bool | inherits (const char *className) const const |
void | installEventFilter (QObject *filterObj) |
bool | isQuickItemType () const const |
bool | isWidgetType () const const |
bool | isWindowType () const const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const const |
void | moveToThread (QThread *targetThread) |
QString | objectName () const const |
void | objectNameChanged (const QString &objectName) |
QObject * | parent () const const |
QVariant | property (const char *name) const const |
Q_CLASSINFO (Name, Value) | |
Q_EMIT Q_EMIT | |
Q_ENUM (...) | |
Q_ENUM_NS (...) | |
Q_ENUMS (...) | |
Q_FLAG (...) | |
Q_FLAG_NS (...) | |
Q_FLAGS (...) | |
Q_GADGET Q_GADGET | |
Q_GADGET_EXPORT (EXPORT_MACRO) | |
Q_INTERFACES (...) | |
Q_INVOKABLE Q_INVOKABLE | |
Q_MOC_INCLUDE Q_MOC_INCLUDE | |
Q_NAMESPACE Q_NAMESPACE | |
Q_NAMESPACE_EXPORT (EXPORT_MACRO) | |
Q_OBJECT Q_OBJECT | |
Q_PROPERTY (...) | |
Q_REVISION Q_REVISION | |
Q_SET_OBJECT_NAME (Object) | |
Q_SIGNAL Q_SIGNAL | |
Q_SIGNALS Q_SIGNALS | |
Q_SLOT Q_SLOT | |
Q_SLOTS Q_SLOTS | |
T | qobject_cast (const QObject *object) |
T | qobject_cast (QObject *object) |
QT_NO_NARROWING_CONVERSIONS_IN_CONNECT QT_NO_NARROWING_CONVERSIONS_IN_CONNECT | |
void | removeEventFilter (QObject *obj) |
void | setObjectName (const QString &name) |
void | setObjectName (QAnyStringView name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | setProperty (const char *name, QVariant &&value) |
bool | signalsBlocked () const const |
int | startTimer (int interval, Qt::TimerType timerType) |
int | startTimer (std::chrono::milliseconds interval, Qt::TimerType timerType) |
QThread * | thread () const const |
Protected Slots | |
void | processFirstMessage () |
virtual void | processIncomingMessage (const QByteArray &msg) |
virtual void | removeBrokenConnection () |
void | removeBrokenConnection2 () |
Protected Member Functions | |
virtual void | processMessage (const QByteArray &msg) |
![]() | |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const QMetaMethod &signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const QMetaMethod &signal) |
bool | isSignalConnected (const QMetaMethod &signal) const const |
int | receivers (const char *signal) const const |
QObject * | sender () const const |
int | senderSignalIndex () const const |
virtual void | timerEvent (QTimerEvent *event) |
Additional Inherited Members | |
![]() | |
typedef | QObjectList |
![]() | |
objectName | |
![]() | |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *context, Functor functor, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, Functor functor) |
bool | disconnect (const QMetaObject::Connection &connection) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
bool | disconnect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
Detailed Description
A client to connect to a KMessageServer.
This class implements a client that can connect to a KMessageServer object. It can be used to exchange messages between clients.
Usually you will connect the signals broadcastReceived and forwardReceived to some specific slots. In these slot methods you can analyze the messages that are sent to you from other clients.
To send messages to other clients, use the methods sendBroadcast() (to send to all clients) or sendForward() (to send to a list of selected clients).
If you want to communicate with the KMessageServer object directly (on a more low level base), use the method sendServerMessage to send a command to the server and connect to the signal serverMessageReceived to see all the incoming messages. In that case the messages must be of the format specified in KMessageServer.
Definition at line 44 of file kmessageclient.h.
Constructor & Destructor Documentation
◆ KMessageClient()
|
explicit |
Constructor.
Creates an unconnected KMessageClient object. Use setServer() later to connect to a KMessageServer object.
Definition at line 41 of file kmessageclient.cpp.
◆ ~KMessageClient()
|
override |
Destructor.
Disconnects from the server, if any connection was established.
Definition at line 47 of file kmessageclient.cpp.
Member Function Documentation
◆ aboutToDisconnect
|
signal |
This signal is emitted right before the client disconnects.
It can be used to this store the id of the client which is about to be lost.
◆ adminId()
quint32 KMessageClient::adminId | ( | ) | const |
- Returns
- The ID of the admin client on the message server.
Definition at line 92 of file kmessageclient.cpp.
◆ adminStatusChanged
|
signal |
This signal is emitted when this client becomes the admin client or when it loses the admin client status.
Connect to this signal if you have to do any initialization or cleanup.
- Parameters
-
isAdmin Whether we are now admin or not
◆ broadcastReceived
|
signal |
This signal is emitted when the client receives a broadcast message from the KMessageServer, sent by another client.
Connect to this signal to analyze the received message and do the right reaction.
senderID contains the ID of the client that sent the broadcast message. You can use this e.g. to send a reply message to only that client. Or you can use it to ignore broadcast messages that were sent by yourself:
- Parameters
-
msg The message that has been sent to us senderID The ID of the client which sent the message
◆ clientList()
QList< quint32 > KMessageClient::clientList | ( | ) | const |
- Returns
- The list of the IDs of all the message clients connected to the message server.
Definition at line 97 of file kmessageclient.cpp.
◆ connectionBroken
|
signal |
This signal is emitted when the connection to the KMessageServer is broken.
Reasons for this can be: a network error, a server breakdown, or you were just kicked from the server.
When this signal is sent, the connection is already lost and the client is unconnected. You can connect to another server by calling setServer() afterwards. But keep in mind that some important messages might have vanished.
◆ delayedMessageCount()
unsigned int KMessageClient::delayedMessageCount | ( | ) | const |
- Returns
- The number of messages that got delayed since lock() was called
Definition at line 326 of file kmessageclient.cpp.
◆ disconnect()
void KMessageClient::disconnect | ( | ) |
Corresponds to setServer(0); but also emits the connectionBroken signal.
Definition at line 301 of file kmessageclient.cpp.
◆ eventClientConnected
|
signal |
This signal is emitted when another client has connected to the server.
Connect to this method if that clients needs initialization. This should usually only be done in one client, e.g. the admin client.
- Parameters
-
clientID The ID of the client that has newly connected.
◆ eventClientDisconnected
|
signal |
This signal is emitted when the server has lost the connection to one of the clients (This could be because of a bad internet connection or because the client disconnected on purpose).
- Parameters
-
clientID The ID of the client that has disconnected broken true if it was disconnected because of a network error
◆ forwardReceived
|
signal |
This signal is emitted when the client receives a forward message from the KMessageServer, sent by another client.
Connect to this signal to analyze the received message and do the right reaction.
senderID contains the ID of the client that sent the broadcast message. You can use this e.g. to send a reply message to only that client.
receivers contains the list of the clients that got the message. (If this list only contains one number, this will be your client ID, and it was exclusively sent to you.)
If you don't want to distinguish between broadcast and forward messages and treat them the same, you can connect forwardReceived signal to the broadcastReceived signal. (Yes, that's possible! You can connect a Qt signal to a Qt signal, and the second one can have less parameters.)
Then connect the broadcast signal to your slot that analyzes the message.
- Parameters
-
msg The message that has been sent to us senderID The ID of the client which sent the message receivers All clients which receive this message
◆ id()
quint32 KMessageClient::id | ( | ) | const |
- Returns
- The client ID of this client. Every client that is connected to a KMessageServer has a unique ID number.
NOTE: As long as the object is not yet connected to the server, and as long as the server hasn't sent the client ID, this method returns 0.
Definition at line 82 of file kmessageclient.cpp.
◆ isAdmin()
bool KMessageClient::isAdmin | ( | ) | const |
- Returns
- Whether or not this client is the server admin. One of the clients connected to the server is the admin and can administrate the server (set maximum number of clients, remove clients, ...).
If you use admin commands without being the admin, these commands are simply ignored by the server.
NOTE: As long as you are not connected to a server, this method returns false.
Definition at line 87 of file kmessageclient.cpp.
◆ isConnected()
bool KMessageClient::isConnected | ( | ) | const |
- Returns
- True, if a connection to a KMessageServer has been started, and if the connection is ready for transferring data. (It will return false e.g. as long as a socket connection hasn't been established, and it will also return false after a socket connection is broken.)
Definition at line 102 of file kmessageclient.cpp.
◆ isNetwork()
bool KMessageClient::isNetwork | ( | ) | const |
- Returns
- TRUE if isConnected() is true AND this is not a local (like KMessageDirect) connection.
Definition at line 107 of file kmessageclient.cpp.
◆ lock()
void KMessageClient::lock | ( | ) |
Once this function is called no message will be received anymore.
processIncomingMessage() gets delayed until unlock() is called.
Note that all messages are still received, but their delivery (like broadcastReceived()) get delayed only.
Definition at line 313 of file kmessageclient.cpp.
◆ peerName()
QString KMessageClient::peerName | ( | ) | const |
- Returns
- "localhost" if isConnected() is FALSE, otherwise the hostname this client is connected to. See also KMessageIO::peerName() and QSocket::peerName().
Definition at line 117 of file kmessageclient.cpp.
◆ peerPort()
quint16 KMessageClient::peerPort | ( | ) | const |
- Returns
- 0 if isConnected() is FALSE, otherwise the port number this client is connected to. See also KMessageIO::peerPort and QSocket::peerPort.
Definition at line 112 of file kmessageclient.cpp.
◆ processFirstMessage
|
protectedslot |
Called from unlock() (using QTimer::singleShot) until all delayed messages are delivered.
Definition at line 267 of file kmessageclient.cpp.
◆ processIncomingMessage
|
protectedvirtualslot |
This slot is called from the signal KMessageIO::received whenever a message from the KMessageServer arrives.
It processes the message and analyzes it. If it is a broadcast or a forward message from another client, it emits the signal processBroadcast or processForward accordingly.
If you want to treat additional server messages, you can overwrite this method. Don't forget to call processIncomingMessage() of your superclass!
At the moment, the following server messages are interpreted:
MSG_BROADCAST, MSG_FORWARD, ANS_CLIENT_ID, ANS_ADMIN_ID, ANS_CLIENT_LIST
- Parameters
-
msg The incoming message
Definition at line 164 of file kmessageclient.cpp.
◆ processMessage()
|
protectedvirtual |
This slot is called from processIncomingMessage or processFirstMessage, depending on whether the client is locked or a delayed message is still here (see lock)
It processes the message and analyzes it. If it is a broadcast or a forward message from another client, it emits the signal processBroadcast or processForward accordingly.
If you want to treat additional server messages, you can overwrite this method. Don't forget to call processIncomingMessage of your superclass!
At the moment, the following server messages are interpreted:
MSG_BROADCAST, MSG_FORWARD, ANS_CLIENT_ID, ANS_ADMIN_ID, ANS_CLIENT_LIST
- Parameters
-
msg The incoming message
Definition at line 180 of file kmessageclient.cpp.
◆ removeBrokenConnection
|
protectedvirtualslot |
This slot is called from the signal KMessageIO::connectionBroken.
It deletes the internal KMessageIO object, and resets the client to default values. To connect again to another server, use setServer.
Definition at line 281 of file kmessageclient.cpp.
◆ removeBrokenConnection2
|
protectedslot |
Definition at line 289 of file kmessageclient.cpp.
◆ sendBroadcast()
void KMessageClient::sendBroadcast | ( | const QByteArray & | msg | ) |
Sends a message to all the clients connected to the server, including ourself.
The message consists of an arbitrary block of data with arbitrary length.
All the clients will receive an exact copy of this block of data, which will be processed in their processBroadcast() method.
- Parameters
-
msg The message to be sent to the clients
Definition at line 133 of file kmessageclient.cpp.
◆ sendForward() [1/2]
void KMessageClient::sendForward | ( | const QByteArray & | msg, |
const QList< quint32 > & | clients ) |
Sends a message to all the clients in a list.
The message consists of an arbitrary block of data with arbitrary length.
All clients will receive an exact copy of this block of data, which will be processed in their processForward() method.
If the list contains client IDs that are not defined, they are ignored. If it contains an ID several times, that client will receive the message several times.
To send a message to the admin of the KMessageServer, you can use 0 as clientID, instead of using the real client ID.
- Parameters
-
msg The message to be sent to the clients clients A list of clients the message should be sent to
Definition at line 145 of file kmessageclient.cpp.
◆ sendForward() [2/2]
void KMessageClient::sendForward | ( | const QByteArray & | msg, |
quint32 | client ) |
Sends a message to a single client.
This is a convenience method. It calls sendForward (const QByteArray &msg, const QValueList <quint32> &clients) with a list containing only one client ID.
To send a message to the admin of the KMessageServer, you can use 0 as clientID, instead of using the real client ID.
- Parameters
-
msg The message to be sent to the client client The id of the client the message shall be sent to
Definition at line 157 of file kmessageclient.cpp.
◆ sendServerMessage()
void KMessageClient::sendServerMessage | ( | const QByteArray & | msg | ) |
Sends a message to the KMessageServer.
If we are not yet connected to one, nothing happens.
Use this method to send a low level command to the server. It has to be in the format specified in KMessageServer.
If you want to send messages to other clients, you should use sendBroadcast() and sendForward().
- Parameters
-
msg The message to be sent to the server. Must be in the format specified in KMessageServer.
Definition at line 124 of file kmessageclient.cpp.
◆ serverMessageReceived
|
signal |
This signal is emitted on every message that came from the server.
You can connect to this signal to see the messages directly. They are in the format specified in KMessageServer.
- Parameters
-
msg The message that has been sent to us unknown True when KMessageClient didn't recognize the message, i.e. it contained an unknown message ID. If you want to add additional message types to the client, connect to this signal, and if unknown is true, analyze the message by yourself. If you recognized the message, set unknown to false (Otherwise a debug message will be printed).
◆ setServer() [1/3]
void KMessageClient::setServer | ( | const QString & | host, |
quint16 | port ) |
Connects the client to (another) server.
Tries to connect via a TCP/IP socket to a KMessageServer object on the given host, listening on the specified port.
If we were already connected, the old connection is closed.
- Parameters
-
host The name of the host to connect to. Must be either a hostname which can be resolved to an IP or just an IP port The port to connect to
Definition at line 54 of file kmessageclient.cpp.
◆ setServer() [2/3]
|
virtual |
Connects the client to (another) server.
To use this method, you have to create a KMessageIO object with new (indeed you must create an instance of a subclass of KMessageIO, e.g. KMessageSocket or KMessageDirect). This object must already be connected to the new server.
Calling this method disconnects any earlier connection, and uses the new KMessageIO object instead. This object gets owned by the KMessageClient object, so don't delete or manipulate it afterwards.
With this method it is possible to change the server on the fly. But be careful that there are no important messages from the old server not yet delivered.
NOTE: It is very likely that we will have another client ID on the new server. The value returned by clientID may be a little outdated until the new server tells us our new ID.
NOTE: The two other setServer methods are for convenience. If you use them, you don't have to create a KMessageIO object yourself.
Definition at line 66 of file kmessageclient.cpp.
◆ setServer() [3/3]
void KMessageClient::setServer | ( | KMessageServer * | server | ) |
Connects the client to (another) server.
Connects to the given server, using KMessageDirect. (The server object has to be in the same process.)
If we were already connected, the old connection is closed.
- Parameters
-
server The KMessageServer to connect to
Definition at line 59 of file kmessageclient.cpp.
◆ unlock()
void KMessageClient::unlock | ( | ) |
Deliver every message that was delayed by lock() and actually deliver all messages that get received from now on.
Definition at line 318 of file kmessageclient.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2024 The KDE developers.
Generated on Fri Dec 20 2024 11:50:50 by doxygen 1.12.0 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.