KMessageServer
#include <KGame/KMessageServer>
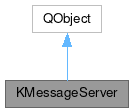
Public Types | |
enum | { REQ_BROADCAST = 1 , REQ_FORWARD , REQ_CLIENT_ID , REQ_ADMIN_ID , REQ_ADMIN_CHANGE , REQ_REMOVE_CLIENT , REQ_MAX_NUM_CLIENTS , REQ_CLIENT_LIST , REQ_MAX_REQ = 0xffff } |
enum | { MSG_BROADCAST = 101 , MSG_FORWARD , ANS_CLIENT_ID , ANS_ADMIN_ID , ANS_CLIENT_LIST , EVNT_CLIENT_CONNECTED , EVNT_CLIENT_DISCONNECTED , EVNT_MAX_EVNT = 0xffff } |
![]() | |
typedef | QObjectList |
Signals | |
void | clientConnected (KMessageIO *client) |
void | connectionLost (KMessageIO *client) |
void | messageReceived (const QByteArray &data, quint32 clientID, bool &unknown) |
Public Slots | |
void | addClient (KMessageIO *) |
void | deleteClients () |
void | removeClient (KMessageIO *io, bool broken) |
Public Member Functions | |
KMessageServer (quint16 cookie=42, QObject *parent=nullptr) | |
quint32 | adminID () const |
virtual void | broadcastMessage (const QByteArray &msg) |
int | clientCount () const |
QList< quint32 > | clientIDs () const |
virtual void | Debug () |
KMessageIO * | findClient (quint32 no) const |
bool | initNetwork (quint16 port=0) |
bool | isOfferingConnections () const |
int | maxClients () const |
virtual void | sendMessage (const QList< quint32 > &ids, const QByteArray &msg) |
virtual void | sendMessage (quint32 id, const QByteArray &msg) |
quint16 | serverPort () const |
void | setAdmin (quint32 adminID) |
void | setMaxClients (int maxnumber) |
void | stopNetwork () |
![]() | |
QObject (QObject *parent) | |
QBindable< QString > | bindableObjectName () |
bool | blockSignals (bool block) |
const QObjectList & | children () const const |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) const const |
bool | disconnect (const QObject *receiver, const char *method) const const |
void | dumpObjectInfo () const const |
void | dumpObjectTree () const const |
QList< QByteArray > | dynamicPropertyNames () const const |
virtual bool | event (QEvent *e) |
virtual bool | eventFilter (QObject *watched, QEvent *event) |
T | findChild (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QRegularExpression &re, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (Qt::FindChildOptions options) const const |
bool | inherits (const char *className) const const |
void | installEventFilter (QObject *filterObj) |
bool | isQuickItemType () const const |
bool | isWidgetType () const const |
bool | isWindowType () const const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const const |
void | moveToThread (QThread *targetThread) |
QString | objectName () const const |
void | objectNameChanged (const QString &objectName) |
QObject * | parent () const const |
QVariant | property (const char *name) const const |
Q_CLASSINFO (Name, Value) | |
Q_EMIT Q_EMIT | |
Q_ENUM (...) | |
Q_ENUM_NS (...) | |
Q_ENUMS (...) | |
Q_FLAG (...) | |
Q_FLAG_NS (...) | |
Q_FLAGS (...) | |
Q_GADGET Q_GADGET | |
Q_GADGET_EXPORT (EXPORT_MACRO) | |
Q_INTERFACES (...) | |
Q_INVOKABLE Q_INVOKABLE | |
Q_MOC_INCLUDE Q_MOC_INCLUDE | |
Q_NAMESPACE Q_NAMESPACE | |
Q_NAMESPACE_EXPORT (EXPORT_MACRO) | |
Q_OBJECT Q_OBJECT | |
Q_PROPERTY (...) | |
Q_REVISION Q_REVISION | |
Q_SET_OBJECT_NAME (Object) | |
Q_SIGNAL Q_SIGNAL | |
Q_SIGNALS Q_SIGNALS | |
Q_SLOT Q_SLOT | |
Q_SLOTS Q_SLOTS | |
T | qobject_cast (const QObject *object) |
T | qobject_cast (QObject *object) |
QT_NO_NARROWING_CONVERSIONS_IN_CONNECT QT_NO_NARROWING_CONVERSIONS_IN_CONNECT | |
void | removeEventFilter (QObject *obj) |
void | setObjectName (const QString &name) |
void | setObjectName (QAnyStringView name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | setProperty (const char *name, QVariant &&value) |
bool | signalsBlocked () const const |
int | startTimer (int interval, Qt::TimerType timerType) |
int | startTimer (std::chrono::milliseconds interval, Qt::TimerType timerType) |
QThread * | thread () const const |
Protected Slots | |
virtual void | getReceivedMessage (const QByteArray &msg) |
virtual void | processOneMessage () |
Protected Member Functions | |
quint32 | uniqueClientNumber () const |
![]() | |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const QMetaMethod &signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const QMetaMethod &signal) |
bool | isSignalConnected (const QMetaMethod &signal) const const |
int | receivers (const char *signal) const const |
QObject * | sender () const const |
int | senderSignalIndex () const const |
virtual void | timerEvent (QTimerEvent *event) |
Additional Inherited Members | |
![]() | |
objectName | |
![]() | |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *context, Functor functor, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, Functor functor) |
bool | disconnect (const QMetaObject::Connection &connection) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
bool | disconnect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
Detailed Description
A server for message sending and broadcasting, using TCP/IP connections.
An object of this class listens for incoming connections via TCP/IP sockets and creates KMessageSocket objects for every established connection. It receives messages from the "clients", analyzes them and processes an appropriate reaction.
You can also use other KMessageIO objects with KMessageServer, not only TCP/IP socket based ones. Use addClient to connect via an object of any KMessageIO subclass. (For clients within the same process, you can e.g. use KMessageDirect.) This object already has to be connected.
The messages are always packages of an arbitrary length. The format of the messages is given below. All the data is stored and received with QDataStream, to be platform independent.
Setting up a KMessageServer can be done like this:
Usually that is everything you will do. There are a lot of public methods to administrate the object (maximum number of clients, finding clients, removing clients, setting the admin client, ...), but this functionality can also be done by messages from the clients. So you can administrate the object completely on remote.
If you want to extend the Server for your own needs (e.g. additional message types), you can either create a subclass and overwrite the method processOneMessage. (But don't forget to call the method of the superclass!) Or you can connect to the signal messageReceived, and analyze the messages there.
Every client has a unique ID, so that messages can be sent to another dedicated client or a list of clients.
One of the clients (the admin) has a special administration right. Some of the administration messages can only be used with him. The admin can give the admin status to another client. You can send a message to the admin by using clientID 0. This is always interpreted as the admin client, of its real clientID.
Here is a list of the messages the KMessageServer understands: << means, the value is inserted into the QByteArray using QDataStream. The messageIDs (REQ_BROADCAST, ...) are of type quint32.
QByteArray << static_cast<quint32>( REQ_BROADCAST ) << raw_data
When the server receives this message, it sends the following message to ALL connected clients (a broadcast), where the raw_data is left unchanged: QByteArray << static_cast <quint32>( MSG_BROADCAST ) << clientID << raw_data quint32 clientID; // the ID of the client that sent the broadcast request
QByteArray << static_cast<quint32>( REQ_FORWARD ) << client_list << raw_data QValueList <quint32> client_list; // list of receivers
When the server receives this message, it sends the following message to the clients in client_list: QByteArray << static_cast<quint32>( MSG_FORWARD ) << senderID << client_list << raw_data quint32 senderID; // the sender of the forward request QValueList <quint32> client_list; // a copy of the receiver list
Note: Every client receives the message as many times as he is in the client_list. Note: Since the client_list is sent to all the clients, every client can see who else got the message. If you want to prevent this, send a single REQ_FORWARD message for every receiver.
QByteArray << static_cast<quint32>( REQ_CLIENT_ID )
When the server receives this message, it sends the following message to the asking client: QByteArray << static_cast<quint32>( ANS_CLIENT_ID ) << clientID quint32 clientID; // The ID of the client who asked for it
Note: This answer is also automatically sent to a new connected client, so that he can store his ID. The ID of a client doesn't change during his lifetime, and is unique for this KMessageServer.
QByteArray << static_cast<quint32>( REQ_ADMIN_ID )
When the server receives this message, it sends the following message to the asking client: QByteArray << ANS_ADMIN_ID << adminID quint32 adminID; // The ID of the admin
Note: This answer is also automatically sent to a new connected client, so that he can see if he is the admin or not. It will also be sent to all connected clients when a new admin is set (see REQ_ADMIN_CHANGE).
QByteArray << static_cast<quint32>( REQ_ADMIN_CHANGE ) << new_admin quint32 new_admin; // the ID of the new admin, or 0 for no admin
When the server receives this message, it sets the admin to the new ID. If no client with that ID exists, nothing happens. With new_admin == 0 no client is a admin. ONLY THE ADMIN ITSELF CAN USE THIS MESSAGE!
Note: The server sends a ANS_ADMIN_ID message to every connected client.
QByteArray << static_cast<quint32>( REQ_REMOVE_CLIENT ) << client_list QValueList <quint32> client_list; // The list of clients to be removed
When the server receives this message, it removes the clients with the ids stored in client_list, disconnecting the connection to them. ONLY THE ADMIN CAN USE THIS MESSAGE!
Note: If one of the clients is the admin himself, he will also be deleted. Another client (if any left) will become the new admin.
QByteArray << static_cast<quint32>( REQ_MAX_NUM_CLIENTS ) << maximum_clients qint32 maximum_clients; // The maximum of clients connected, or infinite if -1
When the server receives this message, it limits the number of clients to the number given, or sets it unlimited for maximum_clients == -1. ONLY THE ADMIN CAN USE THIS MESSAGE!
Note: If there are already more clients, they are not affected. It only prevents new Clients to be added. To assure this limit, remove clients afterwards (REQ_REMOVE_CLIENT)
QByteArray << static_cast<quint32>( REQ_CLIENT_LIST )
When the server receives this message, it answers by sending a list of IDs of all the clients that are connected at the moment. So it sends the following message to the asking client: QByteArray << static_cast<quint32>( ANS_CLIENT_LIST ) << clientList QValueList <quint32> clientList; // The IDs of the connected clients
Note: This message is also sent to every new connected client, so that he knows the other clients.
There are two more messages that are sent from the server to the every client automatically when a new client connects or a connection to a client is lost:
QByteArray << static_cast<quint32>( EVNT_CLIENT_CONNECTED ) << clientID; quint32 clientID; // the ID of the new connected client QByteArray << static_cast<quint32>( EVNT_CLIENT_DISCONNECTED ) << clientID; quint32 clientID; // the ID of the client that lost the connection quint8 broken; // 1 if the network connection was closed, 0 if it was disconnected
on purpose
Definition at line 166 of file kmessageserver.h.
Member Enumeration Documentation
◆ anonymous enum
anonymous enum |
MessageIDs for messages from a client to the message server.
Definition at line 174 of file kmessageserver.h.
◆ anonymous enum
anonymous enum |
MessageIDs for messages from the message server to a client.
Definition at line 189 of file kmessageserver.h.
Constructor & Destructor Documentation
◆ KMessageServer()
|
explicit |
Create a KGameNetwork object.
Definition at line 89 of file kmessageserver.cpp.
◆ ~KMessageServer()
|
override |
Definition at line 98 of file kmessageserver.cpp.
Member Function Documentation
◆ addClient
|
slot |
Adds a new KMessageIO object to the communication server.
This "client" gets a unique ID.
This slot method is automatically called for any incoming TCP/IP connection. You can use it to add other types of connections, e.g. local connections (KMessageDirect) to the server manually.
NOTE: The KMessageIO object gets owned by the KMessageServer, so don't delete or manipulate it afterwards. It is automatically deleted when the connection is broken or the communication server is deleted. So, add a KMessageIO object to just ONE KMessageServer.
Definition at line 156 of file kmessageserver.cpp.
◆ adminID()
quint32 KMessageServer::adminID | ( | ) | const |
Returns the clientID of the admin, if there is a admin, 0 otherwise.
NOTE: Most often you don't need to know that id, since you can use clientID 0 to specify the admin.
Definition at line 281 of file kmessageserver.cpp.
◆ broadcastMessage()
|
virtual |
Sends a message to all connected clients.
The message is NOT translated in any way. This method calls KMessageIO::send for every client added.
Definition at line 315 of file kmessageserver.cpp.
◆ clientConnected
|
signal |
A new client connected to the game.
- Parameters
-
client the client object that connected
◆ clientCount()
int KMessageServer::clientCount | ( | ) | const |
returns the current number of connected clients.
- Returns
- the number of clients
Definition at line 253 of file kmessageserver.cpp.
◆ clientIDs()
QList< quint32 > KMessageServer::clientIDs | ( | ) | const |
returns a list of the unique IDs of all clients.
Definition at line 258 of file kmessageserver.cpp.
◆ connectionLost
|
signal |
A network connection got broken.
Note that the client will automatically get deleted after this signal is emitted. The signal is not emitted when the client was removed regularly.
- Parameters
-
client the client which left the game
◆ Debug()
|
virtual |
Gives debug output of the game status.
Definition at line 467 of file kmessageserver.cpp.
◆ deleteClients
|
slot |
Deletes all connections to the clients.
Definition at line 224 of file kmessageserver.cpp.
◆ findClient()
KMessageIO * KMessageServer::findClient | ( | quint32 | no | ) | const |
Find the KMessageIO object to the given client number.
- Parameters
-
no the client number to look for, or 0 to look for the admin
- Returns
- address of the client, or 0 if no client with that number exists
Definition at line 267 of file kmessageserver.cpp.
◆ getReceivedMessage
|
protectedvirtualslot |
This slot receives all the messages from the KMessageIO::received signals.
It stores the messages in a queue. The messages are later taken out of the queue by getReceivedMessage.
NOTE: It is important that this slot may only be called from the signal KMessageIO::received, since the sender() object is used to find out the client that sent the message!
Definition at line 335 of file kmessageserver.cpp.
◆ initNetwork()
bool KMessageServer::initNetwork | ( | quint16 | port = 0 | ) |
Starts the Communication server to listen for incoming TCP/IP connections.
- Parameters
-
port The port on which the service is offered, or 0 to let the system pick a free port
- Returns
- true if it worked
Definition at line 109 of file kmessageserver.cpp.
◆ isOfferingConnections()
bool KMessageServer::isOfferingConnections | ( | ) | const |
Are we still offer offering server connections?
- Returns
- true, if we are still listening to connections requests
Definition at line 149 of file kmessageserver.cpp.
◆ maxClients()
int KMessageServer::maxClients | ( | ) | const |
returns the maximum number of clients
- Returns
- the number of clients
Definition at line 248 of file kmessageserver.cpp.
◆ messageReceived
|
signal |
This signal is always emitted when a message from a client is received.
You can use this signal to extend the communication server without subclassing. Just connect to this signal and analyze the message, if unknown is true. If you recognize a message and process it, set unknown to false, otherwise a warning message is printed.
- Parameters
-
data the message data clientID the ID of the KMessageIO object that received the message unknown true, if the message type is not known by the KMessageServer
◆ processOneMessage
|
protectedvirtualslot |
This slot is called whenever there are elements in the message queue.
This queue is filled by getReceivedMessage. This slot takes one message out of the queue and analyzes processes it, if it recognizes it. (See message types in the description of the class.) After that, the signal messageReceived is emitted. Connect to that signal if you want to process other types of messages.
Definition at line 354 of file kmessageserver.cpp.
◆ removeClient
|
slot |
Removes the KMessageIO object from the client list and deletes it.
This destroys the connection, if it already was up. Does NOT emit connectionLost. Sends an info message to the other clients, that contains the ID of the removed client and the value of the parameter broken.
- Parameters
-
io the object to delete and to remove from the client list broken true if the client has lost connection Mostly used internally. You will probably not need this.
Definition at line 202 of file kmessageserver.cpp.
◆ sendMessage() [1/2]
|
virtual |
Sends a message to a list of clients.
Their ID is given in ids. If a client id is given more than once in the list, the message is also sent several times to that client. This is just a convenience method. You could also iterate over the list of IDs.
Definition at line 328 of file kmessageserver.cpp.
◆ sendMessage() [2/2]
|
virtual |
Sends a message to a single client with the given ID.
The message is NOT translated in any way. If no client with the given id exists, nothing is done. This is just a convenience method. You could also call findClient (id)->send(msg) manually, but this method checks for errors.
Definition at line 321 of file kmessageserver.cpp.
◆ serverPort()
quint16 KMessageServer::serverPort | ( | ) | const |
Returns the TCP/IP port number we are listening to for incoming connections.
(This has to be known by other clients so that they can connect to us. It's especially necessary if you used 0 as port number in initNetwork().
- Returns
- the port number
Definition at line 133 of file kmessageserver.cpp.
◆ setAdmin()
void KMessageServer::setAdmin | ( | quint32 | adminID | ) |
Sets the admin to a new client with the given ID.
The old admin (if existed) and the new admin will get the ANS_ADMIN message. If you use 0 as new adminID, no client will be admin.
Definition at line 286 of file kmessageserver.cpp.
◆ setMaxClients()
void KMessageServer::setMaxClients | ( | int | maxnumber | ) |
sets the maximum number of clients which can connect.
If this number is reached, no more clients can be added. Setting this number to -1 means unlimited number of clients.
NOTE: Existing connections are not affected. So, clientCount > maxClients is possible, if there were already more clients than allowed before reducing this value.
- Parameters
-
maxnumber the number of clients
Definition at line 243 of file kmessageserver.cpp.
◆ stopNetwork()
void KMessageServer::stopNetwork | ( | ) |
Stops listening for connections.
The already running connections are not affected. To listen for connections again call initNetwork again.
Definition at line 141 of file kmessageserver.cpp.
◆ uniqueClientNumber()
|
protected |
- Returns
- A unique number which can be used as the id of a KMessageIO. It is incremented after every call so if you need the id twice you have to save it anywhere. It's currently used to initialize newly connected clients only.
Definition at line 308 of file kmessageserver.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2024 The KDE developers.
Generated on Fri Nov 29 2024 11:56:07 by doxygen 1.12.0 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.