Marble::GeoDataLineStyle
#include <GeoDataLineStyle.h>
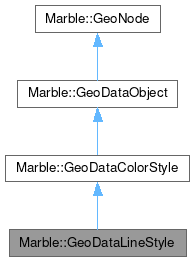
Additional Inherited Members | |
![]() | |
enum | ColorMode { Normal , Random } |
![]() | |
static QString | contrastColor (const QColor &color) |
![]() | |
virtual bool | equals (const GeoDataObject &other) const |
Detailed Description
specifies the style how lines are drawn
A GeoDataLineStyle specifies how the name of a GeoDataFeature is drawn in the viewer. A custom color, color mode (both inherited from GeoDataColorStyle) and width for the width of the line.
Definition at line 28 of file GeoDataLineStyle.h.
Constructor & Destructor Documentation
◆ GeoDataLineStyle() [1/3]
Marble::GeoDataLineStyle::GeoDataLineStyle | ( | ) |
Construct a new GeoDataLineStyle.
Definition at line 39 of file GeoDataLineStyle.cpp.
◆ GeoDataLineStyle() [2/3]
Marble::GeoDataLineStyle::GeoDataLineStyle | ( | const GeoDataLineStyle & | other | ) |
Definition at line 44 of file GeoDataLineStyle.cpp.
◆ GeoDataLineStyle() [3/3]
|
explicit |
Construct a new GeoDataLineStyle.
- Parameters
-
color the color to use when showing the name
- See also
- GeoDataColorStyle
Definition at line 50 of file GeoDataLineStyle.cpp.
◆ ~GeoDataLineStyle()
|
override |
Definition at line 56 of file GeoDataLineStyle.cpp.
Member Function Documentation
◆ background()
bool Marble::GeoDataLineStyle::background | ( | ) | const |
◆ capStyle()
Qt::PenCapStyle Marble::GeoDataLineStyle::capStyle | ( | ) | const |
Return the current pen cap style.
- Returns
- the current pen cap style
Definition at line 118 of file GeoDataLineStyle.cpp.
◆ cosmeticOutline()
bool Marble::GeoDataLineStyle::cosmeticOutline | ( | ) | const |
Return whether the line has a cosmetic 1 pixel outline.
Definition at line 108 of file GeoDataLineStyle.cpp.
◆ dashPattern()
QList< qreal > Marble::GeoDataLineStyle::dashPattern | ( | ) | const |
Return the current dash pattern.
- Returns
- the current dash pattern
Definition at line 148 of file GeoDataLineStyle.cpp.
◆ nodeType()
|
overridevirtual |
Provides type information for downcasting a GeoData.
Reimplemented from Marble::GeoDataColorStyle.
Definition at line 83 of file GeoDataLineStyle.cpp.
◆ operator!=()
bool Marble::GeoDataLineStyle::operator!= | ( | const GeoDataLineStyle & | other | ) | const |
Definition at line 78 of file GeoDataLineStyle.cpp.
◆ operator=()
GeoDataLineStyle & Marble::GeoDataLineStyle::operator= | ( | const GeoDataLineStyle & | other | ) |
assignment operator
Definition at line 61 of file GeoDataLineStyle.cpp.
◆ operator==()
bool Marble::GeoDataLineStyle::operator== | ( | const GeoDataLineStyle & | other | ) | const |
Definition at line 68 of file GeoDataLineStyle.cpp.
◆ pack()
|
override |
Serialize the style to a stream.
- Parameters
-
stream the stream
Definition at line 158 of file GeoDataLineStyle.cpp.
◆ penStyle()
Qt::PenStyle Marble::GeoDataLineStyle::penStyle | ( | ) | const |
Return the current pen cap style.
- Returns
- the current pen cap style
Definition at line 128 of file GeoDataLineStyle.cpp.
◆ physicalWidth()
float Marble::GeoDataLineStyle::physicalWidth | ( | ) | const |
Return the current physical width of the line.
- Returns
- the current width
Definition at line 98 of file GeoDataLineStyle.cpp.
◆ setBackground()
void Marble::GeoDataLineStyle::setBackground | ( | bool | background | ) |
Set whether to draw the solid background.
- Parameters
-
background true
if the background should be solid
Definition at line 143 of file GeoDataLineStyle.cpp.
◆ setCapStyle()
void Marble::GeoDataLineStyle::setCapStyle | ( | Qt::PenCapStyle | style | ) |
◆ setCosmeticOutline()
void Marble::GeoDataLineStyle::setCosmeticOutline | ( | bool | enabled | ) |
Set whether the line has a cosmetic 1 pixel outline.
Definition at line 113 of file GeoDataLineStyle.cpp.
◆ setDashPattern()
void Marble::GeoDataLineStyle::setDashPattern | ( | const QList< qreal > & | pattern | ) |
Sets the dash pattern.
- Parameters
-
pattern dash pattern
Definition at line 153 of file GeoDataLineStyle.cpp.
◆ setPenStyle()
void Marble::GeoDataLineStyle::setPenStyle | ( | Qt::PenStyle | style | ) |
◆ setPhysicalWidth()
void Marble::GeoDataLineStyle::setPhysicalWidth | ( | float | realWidth | ) |
Set the physical width of the line (in meters)
- Parameters
-
realWidth the new width
Definition at line 103 of file GeoDataLineStyle.cpp.
◆ setWidth()
void Marble::GeoDataLineStyle::setWidth | ( | float | width | ) |
Set the width of the line.
- Parameters
-
width the new width
Definition at line 88 of file GeoDataLineStyle.cpp.
◆ unpack()
|
override |
Unserialize the style from a stream.
- Parameters
-
stream the stream
Definition at line 169 of file GeoDataLineStyle.cpp.
◆ width()
float Marble::GeoDataLineStyle::width | ( | ) | const |
Return the current width of the line.
- Returns
- the current width
Definition at line 93 of file GeoDataLineStyle.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2025 The KDE developers.
Generated on Fri May 2 2025 12:01:36 by doxygen 1.13.2 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.