Marble::GeoDataFeature
#include <GeoDataFeature.h>
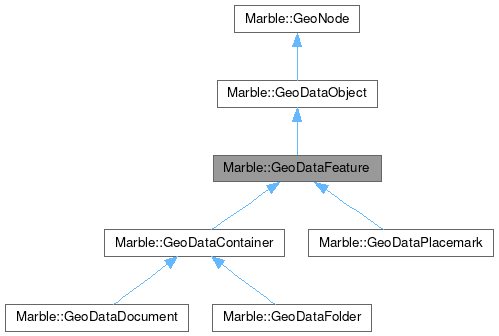
Public Member Functions | |
GeoDataFeature (const GeoDataFeature &other) | |
GeoDataFeature (const QString &name) | |
GeoDataAbstractView * | abstractView () |
const GeoDataAbstractView * | abstractView () const |
QString | address () const |
virtual GeoDataFeature * | clone () const =0 |
QSharedPointer< const GeoDataStyle > | customStyle () const |
QString | description () const |
bool | descriptionIsCDATA () const |
GeoDataExtendedData & | extendedData () |
const GeoDataExtendedData & | extendedData () const |
EnumFeatureId | featureId () const |
bool | isGloballyVisible () const |
bool | isVisible () const |
QString | name () const |
bool | operator!= (const GeoDataFeature &other) const |
GeoDataFeature & | operator= (const GeoDataFeature &other) |
bool | operator== (const GeoDataFeature &other) const |
void | pack (QDataStream &stream) const override |
QString | phoneNumber () const |
qint64 | popularity () const |
GeoDataRegion & | region () |
const GeoDataRegion & | region () const |
const QString | role () const |
void | setAbstractView (GeoDataAbstractView *abstractView) |
void | setAddress (const QString &value) |
void | setDescription (const QString &value) |
void | setDescriptionCDATA (bool cdata) |
void | setExtendedData (const GeoDataExtendedData &extendedData) |
void | setName (const QString &value) |
void | setPhoneNumber (const QString &value) |
void | setPopularity (qint64 popularity) |
void | setRegion (const GeoDataRegion ®ion) |
void | setRole (const QString &role) |
void | setSnippet (const GeoDataSnippet &value) |
void | setStyle (const QSharedPointer< GeoDataStyle > &style) |
void | setStyleMap (const GeoDataStyleMap *map) |
void | setStyleUrl (const QString &value) |
void | setTimeSpan (const GeoDataTimeSpan &timeSpan) |
void | setTimeStamp (const GeoDataTimeStamp &timeStamp) |
void | setVisible (bool value) |
void | setZoomLevel (int index) |
GeoDataSnippet | snippet () const |
QSharedPointer< const GeoDataStyle > | style () const |
const GeoDataStyleMap * | styleMap () const |
QString | styleUrl () const |
GeoDataTimeSpan & | timeSpan () |
const GeoDataTimeSpan & | timeSpan () const |
GeoDataTimeStamp & | timeStamp () |
const GeoDataTimeStamp & | timeStamp () const |
void | unpack (QDataStream &stream) override |
int | zoomLevel () const |
![]() | |
GeoDataObject (const GeoDataObject &) | |
QString | id () const |
GeoDataObject & | operator= (const GeoDataObject &) |
void | pack (QDataStream &stream) const override |
GeoDataObject * | parent () |
const GeoDataObject * | parent () const |
QString | resolvePath (const QString &relativePath) const |
void | setId (const QString &value) |
void | setParent (GeoDataObject *parent) |
void | setTargetId (const QString &value) |
QString | targetId () const |
void | unpack (QDataStream &steam) override |
![]() | |
virtual const char * | nodeType () const =0 |
Protected Member Functions | |
GeoDataFeature (const GeoDataFeature &other, GeoDataFeaturePrivate *dd) | |
GeoDataFeature (GeoDataFeaturePrivate *dd) | |
bool | equals (const GeoDataFeature &other) const |
virtual bool | equals (const GeoDataObject &other) const |
![]() |
Protected Attributes | |
GeoDataFeaturePrivate *const | d_ptr |
Detailed Description
A base class for all geodata features.
GeoDataFeature is the base class for most geodata classes that correspond to places on a map. It is never instantiated by itself, but is always used as part of a derived class.
- See also
- GeoDataPlacemark
- GeoDataContainer
Definition at line 43 of file GeoDataFeature.h.
Constructor & Destructor Documentation
◆ GeoDataFeature() [1/5]
Marble::GeoDataFeature::GeoDataFeature | ( | ) |
Definition at line 35 of file GeoDataFeature.cpp.
◆ GeoDataFeature() [2/5]
|
explicit |
Create a new GeoDataFeature with name
as its name.
Definition at line 46 of file GeoDataFeature.cpp.
◆ GeoDataFeature() [3/5]
Marble::GeoDataFeature::GeoDataFeature | ( | const GeoDataFeature & | other | ) |
Definition at line 40 of file GeoDataFeature.cpp.
◆ ~GeoDataFeature()
|
override |
Definition at line 66 of file GeoDataFeature.cpp.
◆ GeoDataFeature() [4/5]
|
explicitprotected |
Definition at line 52 of file GeoDataFeature.cpp.
◆ GeoDataFeature() [5/5]
|
protected |
Definition at line 58 of file GeoDataFeature.cpp.
Member Function Documentation
◆ abstractView() [1/2]
GeoDataAbstractView * Marble::GeoDataFeature::abstractView | ( | ) |
Definition at line 283 of file GeoDataFeature.cpp.
◆ abstractView() [2/2]
const GeoDataAbstractView * Marble::GeoDataFeature::abstractView | ( | ) | const |
Get the Abstract view of the feature.
Definition at line 273 of file GeoDataFeature.cpp.
◆ address()
QString Marble::GeoDataFeature::address | ( | ) | const |
Return the address of the feature.
Definition at line 197 of file GeoDataFeature.cpp.
◆ clone()
|
pure virtual |
Duplicate into another equal instance.
Implemented in Marble::GeoDataDocument, Marble::GeoDataFolder, and Marble::GeoDataPlacemark.
◆ customStyle()
GeoDataStyle::ConstPtr Marble::GeoDataFeature::customStyle | ( | ) | const |
Return the style assigned to the placemark with setStyle (can be 0)
Definition at line 413 of file GeoDataFeature.cpp.
◆ description()
QString Marble::GeoDataFeature::description | ( | ) | const |
Return the text description of the feature.
Definition at line 237 of file GeoDataFeature.cpp.
◆ descriptionIsCDATA()
bool Marble::GeoDataFeature::descriptionIsCDATA | ( | ) | const |
test if the description is CDATA or not CDATA allows for special characters to be included in XML and also allows for other XML formats to be embedded in the XML without interfering with parser namespace.
- Returns
true
if the description should be treated as CDATAfalse
if the description is a plain string
Definition at line 257 of file GeoDataFeature.cpp.
◆ equals() [1/2]
|
protected |
Definition at line 136 of file GeoDataFeature.cpp.
◆ equals() [2/2]
|
protectedvirtual |
Compares the value of id and targetId of the two objects.
- Returns
- true if they these values are equal or false otherwise
Reimplemented from Marble::GeoDataObject.
Definition at line 92 of file GeoDataObject.cpp.
◆ extendedData() [1/2]
GeoDataExtendedData & Marble::GeoDataFeature::extendedData | ( | ) |
Return the ExtendedData assigned to the feature.
Definition at line 427 of file GeoDataFeature.cpp.
◆ extendedData() [2/2]
const GeoDataExtendedData & Marble::GeoDataFeature::extendedData | ( | ) | const |
Definition at line 396 of file GeoDataFeature.cpp.
◆ featureId()
EnumFeatureId Marble::GeoDataFeature::featureId | ( | ) | const |
Definition at line 167 of file GeoDataFeature.cpp.
◆ isGloballyVisible()
bool Marble::GeoDataFeature::isGloballyVisible | ( | ) | const |
Return whether this feature is visible or not in the context of its parenting.
Definition at line 350 of file GeoDataFeature.cpp.
◆ isVisible()
bool Marble::GeoDataFeature::isVisible | ( | ) | const |
Return whether this feature is visible or not.
Definition at line 338 of file GeoDataFeature.cpp.
◆ name()
QString Marble::GeoDataFeature::name | ( | ) | const |
The name of the feature.
The name of the feature should be a short string. It is often shown directly on the map and need therefore not take up much space.
- Returns
- The name of this feature
Definition at line 173 of file GeoDataFeature.cpp.
◆ operator!=()
|
inline |
Definition at line 57 of file GeoDataFeature.h.
◆ operator=()
GeoDataFeature & Marble::GeoDataFeature::operator= | ( | const GeoDataFeature & | other | ) |
Definition at line 71 of file GeoDataFeature.cpp.
◆ operator==()
bool Marble::GeoDataFeature::operator== | ( | const GeoDataFeature & | other | ) | const |
Definition at line 80 of file GeoDataFeature.cpp.
◆ pack()
|
override |
Serialize the contents of the feature to stream
.
Definition at line 505 of file GeoDataFeature.cpp.
◆ phoneNumber()
QString Marble::GeoDataFeature::phoneNumber | ( | ) | const |
Return the phone number of the feature.
Definition at line 217 of file GeoDataFeature.cpp.
◆ popularity()
qint64 Marble::GeoDataFeature::popularity | ( | ) | const |
Return the popularity of the feature.
Definition at line 493 of file GeoDataFeature.cpp.
◆ region() [1/2]
GeoDataRegion & Marble::GeoDataFeature::region | ( | ) |
Definition at line 445 of file GeoDataFeature.cpp.
◆ region() [2/2]
const GeoDataRegion & Marble::GeoDataFeature::region | ( | ) | const |
Return the region assigned to the placemark.
Definition at line 439 of file GeoDataFeature.cpp.
◆ role()
const QString Marble::GeoDataFeature::role | ( | ) | const |
Return the role of the placemark.
FIXME: describe roles here!
Definition at line 457 of file GeoDataFeature.cpp.
◆ setAbstractView()
void Marble::GeoDataFeature::setAbstractView | ( | GeoDataAbstractView * | abstractView | ) |
Set the abstract view of the feature.
Definition at line 293 of file GeoDataFeature.cpp.
◆ setAddress()
void Marble::GeoDataFeature::setAddress | ( | const QString & | value | ) |
Set the address of this feature to value
.
Definition at line 207 of file GeoDataFeature.cpp.
◆ setDescription()
void Marble::GeoDataFeature::setDescription | ( | const QString & | value | ) |
Set the description of this feature to value
.
Definition at line 247 of file GeoDataFeature.cpp.
◆ setDescriptionCDATA()
void Marble::GeoDataFeature::setDescriptionCDATA | ( | bool | cdata | ) |
Set the description to be CDATA See:
- See also
- descriptionIsCDATA()
Definition at line 267 of file GeoDataFeature.cpp.
◆ setExtendedData()
void Marble::GeoDataFeature::setExtendedData | ( | const GeoDataExtendedData & | extendedData | ) |
Sets the ExtendedData of the feature.
- Parameters
-
extendedData the new ExtendedData to be used.
Definition at line 433 of file GeoDataFeature.cpp.
◆ setName()
void Marble::GeoDataFeature::setName | ( | const QString & | value | ) |
Set a new name for this feature.
- Parameters
-
value the new name
Definition at line 179 of file GeoDataFeature.cpp.
◆ setPhoneNumber()
void Marble::GeoDataFeature::setPhoneNumber | ( | const QString & | value | ) |
Set the phone number of this feature to value
.
Definition at line 227 of file GeoDataFeature.cpp.
◆ setPopularity()
void Marble::GeoDataFeature::setPopularity | ( | qint64 | popularity | ) |
Sets the popularity
of the feature.
- Parameters
-
popularity the new popularity value
Definition at line 499 of file GeoDataFeature.cpp.
◆ setRegion()
void Marble::GeoDataFeature::setRegion | ( | const GeoDataRegion & | region | ) |
Sets the region of the placemark.
- Parameters
-
region new value for the region
The feature is only shown when the region if active.
Definition at line 451 of file GeoDataFeature.cpp.
◆ setRole()
void Marble::GeoDataFeature::setRole | ( | const QString & | role | ) |
Sets the role of the placemark.
- Parameters
-
role the new role to be used.
Definition at line 463 of file GeoDataFeature.cpp.
◆ setSnippet()
void Marble::GeoDataFeature::setSnippet | ( | const GeoDataSnippet & | value | ) |
Set a new name for this feature.
- Parameters
-
value the new name
Definition at line 191 of file GeoDataFeature.cpp.
◆ setStyle()
void Marble::GeoDataFeature::setStyle | ( | const QSharedPointer< GeoDataStyle > & | style | ) |
Sets the style of the placemark.
- Parameters
-
style the new style to be used.
Definition at line 419 of file GeoDataFeature.cpp.
◆ setStyleMap()
void Marble::GeoDataFeature::setStyleMap | ( | const GeoDataStyleMap * | map | ) |
Sets the styleMap of the feature.
Definition at line 475 of file GeoDataFeature.cpp.
◆ setStyleUrl()
void Marble::GeoDataFeature::setStyleUrl | ( | const QString & | value | ) |
Set the styleUrl of this feature to value
.
Definition at line 309 of file GeoDataFeature.cpp.
◆ setTimeSpan()
void Marble::GeoDataFeature::setTimeSpan | ( | const GeoDataTimeSpan & | timeSpan | ) |
Set the timespan of the feature.
- Parameters
-
timeSpan new of timespan.
Definition at line 372 of file GeoDataFeature.cpp.
◆ setTimeStamp()
void Marble::GeoDataFeature::setTimeStamp | ( | const GeoDataTimeStamp & | timeStamp | ) |
Set the timestamp of the feature.
- Parameters
-
timeStamp new of the timestamp.
Definition at line 390 of file GeoDataFeature.cpp.
◆ setVisible()
void Marble::GeoDataFeature::setVisible | ( | bool | value | ) |
Set a new value for visibility.
- Parameters
-
value new value for the visibility
This function sets the visibility, i.e. whether this feature should be shown or not. This can be changed either from a GUI or through some action of the program.
Definition at line 344 of file GeoDataFeature.cpp.
◆ setZoomLevel()
void Marble::GeoDataFeature::setZoomLevel | ( | int | index | ) |
Sets the popularity index
of the placemark.
- Parameters
-
index the new index to be used.
Definition at line 487 of file GeoDataFeature.cpp.
◆ snippet()
GeoDataSnippet Marble::GeoDataFeature::snippet | ( | ) | const |
A short description of the feature.
HTML markup is not supported.
- Todo
- When the Snippet is not supplied, the first lines of description should be used.
- Returns
- The name of this feature
Definition at line 185 of file GeoDataFeature.cpp.
◆ style()
GeoDataStyle::ConstPtr Marble::GeoDataFeature::style | ( | ) | const |
Return the style assigned to the placemark, or a default style if none has been set.
Definition at line 402 of file GeoDataFeature.cpp.
◆ styleMap()
const GeoDataStyleMap * Marble::GeoDataFeature::styleMap | ( | ) | const |
Return a pointer to a GeoDataStyleMap object which represents the styleMap of this feature.
A styleMap is simply a QMap<QString,QString> which can connect two styles with a keyword. This can be used to have a highlighted and a normal style.
- See also
- GeoDataStyleMap
Definition at line 469 of file GeoDataFeature.cpp.
◆ styleUrl()
QString Marble::GeoDataFeature::styleUrl | ( | ) | const |
Return the styleUrl of the feature.
Definition at line 303 of file GeoDataFeature.cpp.
◆ timeSpan() [1/2]
GeoDataTimeSpan & Marble::GeoDataFeature::timeSpan | ( | ) |
Definition at line 366 of file GeoDataFeature.cpp.
◆ timeSpan() [2/2]
const GeoDataTimeSpan & Marble::GeoDataFeature::timeSpan | ( | ) | const |
Return the timespan of the feature.
Definition at line 360 of file GeoDataFeature.cpp.
◆ timeStamp() [1/2]
GeoDataTimeStamp & Marble::GeoDataFeature::timeStamp | ( | ) |
Definition at line 384 of file GeoDataFeature.cpp.
◆ timeStamp() [2/2]
const GeoDataTimeStamp & Marble::GeoDataFeature::timeStamp | ( | ) | const |
Return the timestamp of the feature.
Definition at line 378 of file GeoDataFeature.cpp.
◆ unpack()
|
override |
Unserialize the contents of the feature from stream
.
Definition at line 522 of file GeoDataFeature.cpp.
◆ zoomLevel()
int Marble::GeoDataFeature::zoomLevel | ( | ) | const |
Return the popularity index of the placemark.
The popularity index is a value which describes at which zoom level the placemark will be shown.
Definition at line 481 of file GeoDataFeature.cpp.
Member Data Documentation
◆ d_ptr
|
protected |
Definition at line 269 of file GeoDataFeature.h.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2024 The KDE developers.
Generated on Fri Dec 27 2024 11:51:00 by doxygen 1.12.0 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.