Marble::GeoDataContainer
#include <GeoDataContainer.h>
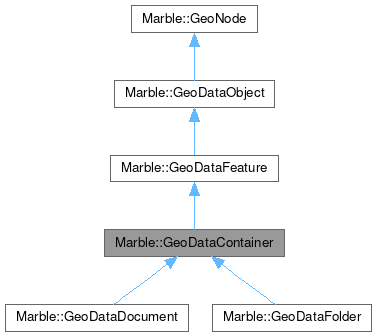
Public Member Functions | |
GeoDataContainer () | |
GeoDataContainer (const GeoDataContainer &other) | |
~GeoDataContainer () override | |
void | append (GeoDataFeature *other) |
GeoDataFeature & | at (int pos) |
const GeoDataFeature & | at (int pos) const |
QVector< GeoDataFeature * >::Iterator | begin () |
GeoDataFeature * | child (int) |
const GeoDataFeature * | child (int) const |
int | childPosition (const GeoDataFeature *child) const |
void | clear () |
QVector< GeoDataFeature * >::ConstIterator | constBegin () const |
QVector< GeoDataFeature * >::ConstIterator | constEnd () const |
QVector< GeoDataFeature * >::Iterator | end () |
QVector< GeoDataFeature * > | featureList () const |
GeoDataFeature & | first () |
const GeoDataFeature & | first () const |
QVector< GeoDataFolder * > | folderList () const |
GEODATA_DEPRECATED void | insert (GeoDataFeature *other, int index) |
void | insert (int index, GeoDataFeature *feature) |
bool | isEmpty () const |
GeoDataFeature & | last () |
const GeoDataFeature & | last () const |
GeoDataLatLonAltBox | latLonAltBox () const |
GeoDataContainer & | operator= (const GeoDataContainer &other) |
void | pack (QDataStream &stream) const override |
QVector< GeoDataPlacemark * > | placemarkList () const |
void | remove (int index) |
void | remove (int index, int count) |
int | removeAll (GeoDataFeature *feature) |
void | removeAt (int index) |
void | removeFirst () |
void | removeLast () |
bool | removeOne (GeoDataFeature *feature) |
int | size () const |
void | unpack (QDataStream &stream) override |
![]() | |
GeoDataFeature (const GeoDataFeature &other) | |
GeoDataFeature (const QString &name) | |
GeoDataAbstractView * | abstractView () |
const GeoDataAbstractView * | abstractView () const |
QString | address () const |
virtual GeoDataFeature * | clone () const =0 |
QSharedPointer< const GeoDataStyle > | customStyle () const |
QString | description () const |
bool | descriptionIsCDATA () const |
GeoDataExtendedData & | extendedData () |
const GeoDataExtendedData & | extendedData () const |
EnumFeatureId | featureId () const |
bool | isGloballyVisible () const |
bool | isVisible () const |
QString | name () const |
bool | operator!= (const GeoDataFeature &other) const |
GeoDataFeature & | operator= (const GeoDataFeature &other) |
bool | operator== (const GeoDataFeature &other) const |
void | pack (QDataStream &stream) const override |
QString | phoneNumber () const |
qint64 | popularity () const |
GeoDataRegion & | region () |
const GeoDataRegion & | region () const |
const QString | role () const |
void | setAbstractView (GeoDataAbstractView *abstractView) |
void | setAddress (const QString &value) |
void | setDescription (const QString &value) |
void | setDescriptionCDATA (bool cdata) |
void | setExtendedData (const GeoDataExtendedData &extendedData) |
void | setName (const QString &value) |
void | setPhoneNumber (const QString &value) |
void | setPopularity (qint64 popularity) |
void | setRegion (const GeoDataRegion ®ion) |
void | setRole (const QString &role) |
void | setSnippet (const GeoDataSnippet &value) |
void | setStyle (const QSharedPointer< GeoDataStyle > &style) |
void | setStyleMap (const GeoDataStyleMap *map) |
void | setStyleUrl (const QString &value) |
void | setTimeSpan (const GeoDataTimeSpan &timeSpan) |
void | setTimeStamp (const GeoDataTimeStamp &timeStamp) |
void | setVisible (bool value) |
void | setZoomLevel (int index) |
GeoDataSnippet | snippet () const |
QSharedPointer< const GeoDataStyle > | style () const |
const GeoDataStyleMap * | styleMap () const |
QString | styleUrl () const |
GeoDataTimeSpan & | timeSpan () |
const GeoDataTimeSpan & | timeSpan () const |
GeoDataTimeStamp & | timeStamp () |
const GeoDataTimeStamp & | timeStamp () const |
void | unpack (QDataStream &stream) override |
int | zoomLevel () const |
![]() | |
GeoDataObject (const GeoDataObject &) | |
QString | id () const |
GeoDataObject & | operator= (const GeoDataObject &) |
void | pack (QDataStream &stream) const override |
GeoDataObject * | parent () |
const GeoDataObject * | parent () const |
QString | resolvePath (const QString &relativePath) const |
void | setId (const QString &value) |
void | setParent (GeoDataObject *parent) |
void | setTargetId (const QString &value) |
QString | targetId () const |
void | unpack (QDataStream &steam) override |
![]() | |
virtual const char * | nodeType () const =0 |
Protected Member Functions | |
GeoDataContainer (const GeoDataContainer &other, GeoDataContainerPrivate *priv) | |
GeoDataContainer (GeoDataContainerPrivate *priv) | |
bool | equals (const GeoDataContainer &other) const |
bool | equals (const GeoDataFeature &other) const |
![]() | |
GeoDataFeature (const GeoDataFeature &other, GeoDataFeaturePrivate *dd) | |
GeoDataFeature (GeoDataFeaturePrivate *dd) | |
bool | equals (const GeoDataFeature &other) const |
virtual bool | equals (const GeoDataObject &other) const |
Additional Inherited Members | |
![]() | |
GeoDataFeaturePrivate *const | d_ptr |
Detailed Description
A base class that can hold GeoDataFeatures.
GeoDataContainer is the base class for the GeoData container classes GeoDataFolder and GeoDataDocument. It is never instantiated by itself, but is always used as part of a derived class.
It is based on GeoDataFeature, and it only adds a QVector<GeodataFeature *> to it, making it a Feature that can hold other Features.
- See also
- GeoDataFolder
- GeoDataDocument
Definition at line 42 of file GeoDataContainer.h.
Constructor & Destructor Documentation
◆ GeoDataContainer() [1/4]
Marble::GeoDataContainer::GeoDataContainer | ( | ) |
Default constructor.
Definition at line 31 of file GeoDataContainer.cpp.
◆ GeoDataContainer() [2/4]
Marble::GeoDataContainer::GeoDataContainer | ( | const GeoDataContainer & | other | ) |
Definition at line 50 of file GeoDataContainer.cpp.
◆ ~GeoDataContainer()
|
override |
Destruct the GeoDataContainer.
Definition at line 57 of file GeoDataContainer.cpp.
◆ GeoDataContainer() [3/4]
|
explicitprotected |
Definition at line 36 of file GeoDataContainer.cpp.
◆ GeoDataContainer() [4/4]
|
protected |
Definition at line 43 of file GeoDataContainer.cpp.
Member Function Documentation
◆ append()
void Marble::GeoDataContainer::append | ( | GeoDataFeature * | other | ) |
add an element
Definition at line 205 of file GeoDataContainer.cpp.
◆ at() [1/2]
GeoDataFeature & Marble::GeoDataContainer::at | ( | int | pos | ) |
return the reference of the element at a specific position
Definition at line 266 of file GeoDataContainer.cpp.
◆ at() [2/2]
const GeoDataFeature & Marble::GeoDataContainer::at | ( | int | pos | ) | const |
Definition at line 272 of file GeoDataContainer.cpp.
◆ begin()
QVector< GeoDataFeature * >::Iterator Marble::GeoDataContainer::begin | ( | ) |
Definition at line 309 of file GeoDataContainer.cpp.
◆ child() [1/2]
GeoDataFeature * Marble::GeoDataContainer::child | ( | int | i | ) |
returns the requested child item
Definition at line 166 of file GeoDataContainer.cpp.
◆ child() [2/2]
const GeoDataFeature * Marble::GeoDataContainer::child | ( | int | i | ) | const |
returns the requested child item
Definition at line 172 of file GeoDataContainer.cpp.
◆ childPosition()
int Marble::GeoDataContainer::childPosition | ( | const GeoDataFeature * | child | ) | const |
returns the position of an item in the list
Definition at line 181 of file GeoDataContainer.cpp.
◆ clear()
void Marble::GeoDataContainer::clear | ( | ) |
Definition at line 302 of file GeoDataContainer.cpp.
◆ constBegin()
QVector< GeoDataFeature * >::ConstIterator Marble::GeoDataContainer::constBegin | ( | ) | const |
Definition at line 321 of file GeoDataContainer.cpp.
◆ constEnd()
QVector< GeoDataFeature * >::ConstIterator Marble::GeoDataContainer::constEnd | ( | ) | const |
Definition at line 327 of file GeoDataContainer.cpp.
◆ end()
QVector< GeoDataFeature * >::Iterator Marble::GeoDataContainer::end | ( | ) |
Definition at line 315 of file GeoDataContainer.cpp.
◆ equals() [1/2]
|
protected |
Definition at line 71 of file GeoDataContainer.cpp.
◆ equals() [2/2]
|
protected |
Definition at line 272 of file GeoDataFeature.cpp.
◆ featureList()
QVector< GeoDataFeature * > Marble::GeoDataContainer::featureList | ( | ) | const |
A convenience function that returns all features in this container.
- Returns
- A QVector of GeoDataFeature
- See also
- GeoDataFeature
Definition at line 157 of file GeoDataContainer.cpp.
◆ first() [1/2]
GeoDataFeature & Marble::GeoDataContainer::first | ( | ) |
return the reference of the last element for convenience
Definition at line 290 of file GeoDataContainer.cpp.
◆ first() [2/2]
const GeoDataFeature & Marble::GeoDataContainer::first | ( | ) | const |
Definition at line 296 of file GeoDataContainer.cpp.
◆ folderList()
QVector< GeoDataFolder * > Marble::GeoDataContainer::folderList | ( | ) | const |
A convenience function that returns all folders in this container.
- Returns
- A QVector of GeoDataFolder
- See also
- GeoDataFolder
Definition at line 127 of file GeoDataContainer.cpp.
◆ insert() [1/2]
void Marble::GeoDataContainer::insert | ( | GeoDataFeature * | other, |
int | index ) |
Definition at line 193 of file GeoDataContainer.cpp.
◆ insert() [2/2]
void Marble::GeoDataContainer::insert | ( | int | index, |
GeoDataFeature * | feature ) |
inserts feature
at position index
in the container
Definition at line 198 of file GeoDataContainer.cpp.
◆ isEmpty()
bool Marble::GeoDataContainer::isEmpty | ( | ) | const |
Returns true if the container has size 0; otherwise returns false.
Definition at line 261 of file GeoDataContainer.cpp.
◆ last() [1/2]
GeoDataFeature & Marble::GeoDataContainer::last | ( | ) |
return the reference of the last element for convenience
Definition at line 278 of file GeoDataContainer.cpp.
◆ last() [2/2]
const GeoDataFeature & Marble::GeoDataContainer::last | ( | ) | const |
Definition at line 284 of file GeoDataContainer.cpp.
◆ latLonAltBox()
GeoDataLatLonAltBox Marble::GeoDataContainer::latLonAltBox | ( | ) | const |
A convenience function that returns the LatLonAltBox of all placemarks in this container.
- Returns
- The GeoDataLatLonAltBox
- See also
- GeoDataLatLonAltBox
Definition at line 93 of file GeoDataContainer.cpp.
◆ operator=()
GeoDataContainer & Marble::GeoDataContainer::operator= | ( | const GeoDataContainer & | other | ) |
Definition at line 61 of file GeoDataContainer.cpp.
◆ pack()
|
override |
Serialize the container to a stream.
- Parameters
-
stream the stream
Definition at line 333 of file GeoDataContainer.cpp.
◆ placemarkList()
QVector< GeoDataPlacemark * > Marble::GeoDataContainer::placemarkList | ( | ) | const |
A convenience function that returns all placemarks in this container.
- Returns
- A QVector of GeoDataPlacemark
- See also
- GeoDataPlacemark
Definition at line 145 of file GeoDataContainer.cpp.
◆ remove() [1/2]
void Marble::GeoDataContainer::remove | ( | int | index | ) |
Definition at line 213 of file GeoDataContainer.cpp.
◆ remove() [2/2]
void Marble::GeoDataContainer::remove | ( | int | index, |
int | count ) |
Definition at line 219 of file GeoDataContainer.cpp.
◆ removeAll()
int Marble::GeoDataContainer::removeAll | ( | GeoDataFeature * | feature | ) |
Definition at line 225 of file GeoDataContainer.cpp.
◆ removeAt()
void Marble::GeoDataContainer::removeAt | ( | int | index | ) |
Definition at line 231 of file GeoDataContainer.cpp.
◆ removeFirst()
void Marble::GeoDataContainer::removeFirst | ( | ) |
Definition at line 237 of file GeoDataContainer.cpp.
◆ removeLast()
void Marble::GeoDataContainer::removeLast | ( | ) |
Definition at line 243 of file GeoDataContainer.cpp.
◆ removeOne()
bool Marble::GeoDataContainer::removeOne | ( | GeoDataFeature * | feature | ) |
Definition at line 249 of file GeoDataContainer.cpp.
◆ size()
int Marble::GeoDataContainer::size | ( | ) | const |
size of the container
Definition at line 255 of file GeoDataContainer.cpp.
◆ unpack()
|
override |
Unserialize the container from a stream.
- Parameters
-
stream the stream
Definition at line 350 of file GeoDataContainer.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2024 The KDE developers.
Generated on Fri Jul 26 2024 11:57:58 by doxygen 1.11.0 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.