Marble::GeoDataLatLonAltBox
#include <GeoDataLatLonAltBox.h>
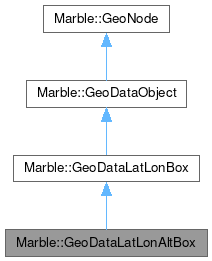
Public Member Functions | |
GeoDataLatLonAltBox (const GeoDataCoordinates &coordinates) | |
GeoDataLatLonAltBox (const GeoDataLatLonAltBox &other) | |
GeoDataLatLonAltBox (const GeoDataLatLonBox &other, qreal minAltitude, qreal maxAltitude) | |
AltitudeMode | altitudeMode () const |
GeoDataCoordinates | center () const override |
void | clear () override |
bool | contains (const GeoDataCoordinates &) const override |
bool | contains (const GeoDataLatLonAltBox &) const |
virtual bool | intersects (const GeoDataLatLonAltBox &) const |
virtual bool | intersects (const GeoDataLatLonBox &) const |
bool | isNull () const override |
qreal | maxAltitude () const |
qreal | minAltitude () const |
const char * | nodeType () const override |
GeoDataLatLonAltBox & | operator= (const GeoDataCoordinates &other) |
GeoDataLatLonAltBox & | operator= (const GeoDataLatLonAltBox &other) |
void | pack (QDataStream &stream) const override |
uint | qHash (const GeoDataLatLonAltBox &) |
void | setAltitudeMode (const AltitudeMode altitudeMode) |
void | setMaxAltitude (const qreal maxAltitude) |
void | setMinAltitude (const qreal minAltitude) |
void | unpack (QDataStream &stream) override |
![]() | |
GeoDataLatLonBox (const GeoDataLatLonBox &) | |
GeoDataLatLonBox (qreal north, qreal south, qreal east, qreal west, GeoDataCoordinates::Unit unit=GeoDataCoordinates::Radian) | |
void | boundaries (qreal &north, qreal &south, qreal &east, qreal &west, GeoDataCoordinates::Unit unit=GeoDataCoordinates::Radian) const |
bool | contains (const GeoDataLatLonBox &) const |
bool | contains (qreal lon, qreal lat) const |
bool | containsPole (Pole pole=AnyPole) const |
bool | crossesDateLine () const |
qreal | east (GeoDataCoordinates::Unit unit=GeoDataCoordinates::Radian) const |
qreal | height (GeoDataCoordinates::Unit unit=GeoDataCoordinates::Radian) const |
virtual bool | isEmpty () const |
const char * | nodeType () const override |
qreal | north (GeoDataCoordinates::Unit unit=GeoDataCoordinates::Radian) const |
GeoDataLatLonBox & | operator= (const GeoDataLatLonBox &other) |
GeoDataLatLonBox | operator| (const GeoDataLatLonBox &other) const |
GeoDataLatLonBox & | operator|= (const GeoDataLatLonBox &other) |
void | pack (QDataStream &stream) const override |
qreal | rotation (GeoDataCoordinates::Unit unit=GeoDataCoordinates::Radian) const |
void | scale (qreal verticalFactor, qreal horizontalFactor) const |
GeoDataLatLonBox | scaled (qreal verticalFactor, qreal horizontalFactor) const |
void | setBoundaries (qreal north, qreal south, qreal east, qreal west, GeoDataCoordinates::Unit unit=GeoDataCoordinates::Radian) |
void | setEast (const qreal east, GeoDataCoordinates::Unit unit=GeoDataCoordinates::Radian) |
void | setNorth (const qreal north, GeoDataCoordinates::Unit unit=GeoDataCoordinates::Radian) |
void | setRotation (const qreal rotation, GeoDataCoordinates::Unit unit=GeoDataCoordinates::Radian) |
void | setSouth (const qreal south, GeoDataCoordinates::Unit unit=GeoDataCoordinates::Radian) |
void | setWest (const qreal west, GeoDataCoordinates::Unit unit=GeoDataCoordinates::Radian) |
qreal | south (GeoDataCoordinates::Unit unit=GeoDataCoordinates::Radian) const |
GeoDataLatLonBox | toCircumscribedRectangle () const |
GeoDataLatLonBox | united (const GeoDataLatLonBox &other) const |
void | unpack (QDataStream &stream) override |
qreal | west (GeoDataCoordinates::Unit unit=GeoDataCoordinates::Radian) const |
qreal | width (GeoDataCoordinates::Unit unit=GeoDataCoordinates::Radian) const |
![]() | |
GeoDataObject (const GeoDataObject &) | |
QString | id () const |
GeoDataObject & | operator= (const GeoDataObject &) |
void | pack (QDataStream &stream) const override |
GeoDataObject * | parent () |
const GeoDataObject * | parent () const |
QString | resolvePath (const QString &relativePath) const |
void | setId (const QString &value) |
void | setParent (GeoDataObject *parent) |
void | setTargetId (const QString &value) |
QString | targetId () const |
void | unpack (QDataStream &steam) override |
Static Public Member Functions | |
static GeoDataLatLonAltBox | fromLineString (const GeoDataLineString &lineString) |
![]() | |
static bool | crossesDateLine (qreal east, qreal west) |
static GeoDataLatLonBox | fromLineString (const GeoDataLineString &lineString) |
static bool | fuzzyCompare (const GeoDataLatLonBox &lhs, const GeoDataLatLonBox &rhs, const qreal factor=0.01) |
static qreal | height (qreal north, qreal south, GeoDataCoordinates::Unit unit=GeoDataCoordinates::Radian) |
static qreal | width (qreal east, qreal west, GeoDataCoordinates::Unit unit=GeoDataCoordinates::Radian) |
Additional Inherited Members | |
![]() | |
virtual bool | equals (const GeoDataObject &other) const |
Detailed Description
A class that defines a 3D bounding box for geographic data.
GeoDataLatLonAltBox is a 3D bounding box that describes a geographic area in terms of latitude, longitude and altitude.
The bounding box gets described by assigning the northern, southern, eastern and western boundary. So usually the value of the eastern boundary is bigger than the value of the western boundary. Only if the bounding box crosses the date line then the eastern boundary has got a smaller value than the western one.
Definition at line 39 of file GeoDataLatLonAltBox.h.
Constructor & Destructor Documentation
◆ GeoDataLatLonAltBox() [1/4]
Marble::GeoDataLatLonAltBox::GeoDataLatLonAltBox | ( | ) |
Definition at line 60 of file GeoDataLatLonAltBox.cpp.
◆ GeoDataLatLonAltBox() [2/4]
Marble::GeoDataLatLonAltBox::GeoDataLatLonAltBox | ( | const GeoDataLatLonAltBox & | other | ) |
Definition at line 66 of file GeoDataLatLonAltBox.cpp.
◆ GeoDataLatLonAltBox() [3/4]
Marble::GeoDataLatLonAltBox::GeoDataLatLonAltBox | ( | const GeoDataLatLonBox & | other, |
qreal | minAltitude, | ||
qreal | maxAltitude ) |
Definition at line 72 of file GeoDataLatLonAltBox.cpp.
◆ GeoDataLatLonAltBox() [4/4]
|
explicit |
A LatLonAltBox with the data from a GeoDataCoordinate This way of creating a GeoDataLatLonAltBox sets the north and south values of this box to the Latitude value in the GeoDataCoordinate, resulting in a Box that has a 0 Area.
This is useful for building LatLonAltBoxes from GeoDataCoordinates.
Definition at line 86 of file GeoDataLatLonAltBox.cpp.
◆ ~GeoDataLatLonAltBox()
|
override |
Definition at line 99 of file GeoDataLatLonAltBox.cpp.
Member Function Documentation
◆ altitudeMode()
AltitudeMode Marble::GeoDataLatLonAltBox::altitudeMode | ( | ) | const |
Get the reference system for the altitude.
- Returns
- the point of reference which marks the origin for measuring the altitude.
Definition at line 129 of file GeoDataLatLonAltBox.cpp.
◆ center()
|
overridevirtual |
returns the center of this box
- Returns
- a coordinate, body-center of the box
Reimplemented from Marble::GeoDataLatLonBox.
Definition at line 134 of file GeoDataLatLonAltBox.cpp.
◆ clear()
|
overridevirtual |
Resets the bounding box to its uninitialised state (and thus contains nothing).
Reimplemented from Marble::GeoDataLatLonBox.
Definition at line 240 of file GeoDataLatLonAltBox.cpp.
◆ contains() [1/2]
|
overridevirtual |
Reimplemented from Marble::GeoDataLatLonBox.
Definition at line 151 of file GeoDataLatLonAltBox.cpp.
◆ contains() [2/2]
bool Marble::GeoDataLatLonAltBox::contains | ( | const GeoDataLatLonAltBox & | other | ) | const |
Definition at line 163 of file GeoDataLatLonAltBox.cpp.
◆ fromLineString()
|
static |
Create the smallest bounding box from a line string.
- Returns
- the smallest bounding box that contains the linestring.
Definition at line 194 of file GeoDataLatLonAltBox.cpp.
◆ intersects() [1/2]
|
virtual |
Check if this GeoDataLatLonAltBox intersects with the given one.
Definition at line 177 of file GeoDataLatLonAltBox.cpp.
◆ intersects() [2/2]
|
virtual |
Reimplemented from Marble::GeoDataLatLonBox.
Definition at line 170 of file GeoDataLatLonBox.cpp.
◆ isNull()
|
overridevirtual |
Indicates whether the bounding box only contains a single 2D point ("singularity").
- Returns
- Return value is true if the height and the width of the bounding box equal zero.
Reimplemented from Marble::GeoDataLatLonBox.
Definition at line 235 of file GeoDataLatLonAltBox.cpp.
◆ maxAltitude()
qreal Marble::GeoDataLatLonAltBox::maxAltitude | ( | ) | const |
Get the upper altitude boundary of the bounding box.
- Returns
- the height of the upper altitude boundary in meters.
Definition at line 119 of file GeoDataLatLonAltBox.cpp.
◆ minAltitude()
qreal Marble::GeoDataLatLonAltBox::minAltitude | ( | ) | const |
Get the lower altitude boundary of the bounding box.
- Returns
- the height of the lower altitude boundary in meters.
Definition at line 109 of file GeoDataLatLonAltBox.cpp.
◆ nodeType()
|
overridevirtual |
Provides type information for downcasting a GeoData.
Implements Marble::GeoNode.
Definition at line 104 of file GeoDataLatLonAltBox.cpp.
◆ operator=() [1/2]
GeoDataLatLonAltBox & Marble::GeoDataLatLonAltBox::operator= | ( | const GeoDataCoordinates & | other | ) |
Definition at line 49 of file GeoDataLatLonAltBox.cpp.
◆ operator=() [2/2]
GeoDataLatLonAltBox & Marble::GeoDataLatLonAltBox::operator= | ( | const GeoDataLatLonAltBox & | other | ) |
Definition at line 41 of file GeoDataLatLonAltBox.cpp.
◆ pack()
|
override |
Serialize the contents of the feature to stream
.
Definition at line 248 of file GeoDataLatLonAltBox.cpp.
◆ qHash()
uint Marble::GeoDataLatLonAltBox::qHash | ( | const GeoDataLatLonAltBox & | ) |
qHash, for using GeoDataLatLonAltBox in a QCache as Key
- Returns
- the hash of the GeoDataLatLonAltBox
◆ setAltitudeMode()
void Marble::GeoDataLatLonAltBox::setAltitudeMode | ( | const AltitudeMode | altitudeMode | ) |
Definition at line 146 of file GeoDataLatLonAltBox.cpp.
◆ setMaxAltitude()
void Marble::GeoDataLatLonAltBox::setMaxAltitude | ( | const qreal | maxAltitude | ) |
Definition at line 124 of file GeoDataLatLonAltBox.cpp.
◆ setMinAltitude()
void Marble::GeoDataLatLonAltBox::setMinAltitude | ( | const qreal | minAltitude | ) |
Definition at line 114 of file GeoDataLatLonAltBox.cpp.
◆ unpack()
|
override |
Unserialize the contents of the feature from stream
.
Definition at line 256 of file GeoDataLatLonAltBox.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2025 The KDE developers.
Generated on Fri Apr 11 2025 11:47:55 by doxygen 1.13.2 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.