Marble::GeoDataLineString
#include <GeoDataLineString.h>
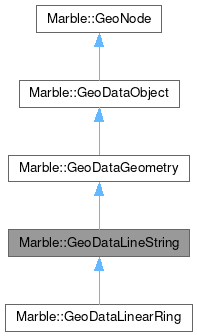
Public Types | |
using | ConstIterator = QList<GeoDataCoordinates>::ConstIterator |
using | Iterator = QList<GeoDataCoordinates>::Iterator |
Public Member Functions | |
GeoDataLineString (const GeoDataGeometry &other) | |
GeoDataLineString (TessellationFlags f=NoTessellation) | |
~GeoDataLineString () override | |
void | append (const GeoDataCoordinates &value) |
void | append (const QList< GeoDataCoordinates > &values) |
GeoDataCoordinates & | at (int pos) |
const GeoDataCoordinates & | at (int pos) const |
QList< GeoDataCoordinates >::Iterator | begin () |
QList< GeoDataCoordinates >::ConstIterator | begin () const |
void | clear () |
QList< GeoDataCoordinates >::ConstIterator | constBegin () const |
QList< GeoDataCoordinates >::ConstIterator | constEnd () const |
GeoDataGeometry * | copy () const override |
QList< GeoDataCoordinates >::Iterator | end () |
QList< GeoDataCoordinates >::ConstIterator | end () const |
QList< GeoDataCoordinates >::Iterator | erase (const QList< GeoDataCoordinates >::Iterator &begin, const QList< GeoDataCoordinates >::Iterator &end) |
QList< GeoDataCoordinates >::Iterator | erase (const QList< GeoDataCoordinates >::Iterator &position) |
GeoDataCoordinates & | first () |
const GeoDataCoordinates & | first () const |
EnumGeometryId | geometryId () const override |
void | insert (int index, const GeoDataCoordinates &value) |
virtual bool | isClosed () const |
bool | isEmpty () const |
GeoDataCoordinates & | last () |
const GeoDataCoordinates & | last () const |
const GeoDataLatLonAltBox & | latLonAltBox () const override |
virtual qreal | length (qreal planetRadius, int offset=0) const |
GeoDataLineString | mid (int pos, int length=-1) const |
const char * | nodeType () const override |
bool | operator!= (const GeoDataLineString &other) const |
GeoDataLineString & | operator<< (const GeoDataCoordinates &value) |
GeoDataLineString & | operator<< (const GeoDataLineString &lineString) |
bool | operator== (const GeoDataLineString &other) const |
GeoDataCoordinates & | operator[] (int pos) |
const GeoDataCoordinates & | operator[] (int pos) const |
GeoDataLineString | optimized () const |
void | pack (QDataStream &stream) const override |
void | remove (int i) |
void | reserve (int size) |
void | reverse () |
void | setTessellate (bool tessellate) |
void | setTessellationFlags (TessellationFlags f) |
int | size () const |
bool | tessellate () const |
TessellationFlags | tessellationFlags () const |
virtual QList< GeoDataLineString * > | toDateLineCorrected () const |
virtual GeoDataLineString | toNormalized () const |
virtual GeoDataLineString | toPoleCorrected () const |
virtual GeoDataLineString | toRangeCorrected () const |
QVariantList | toVariantList () const |
void | unpack (QDataStream &stream) override |
![]() | |
AltitudeMode | altitudeMode () const |
void | detach () |
bool | extrude () const |
bool | operator!= (const GeoDataGeometry &other) const |
bool | operator== (const GeoDataGeometry &other) const |
void | pack (QDataStream &stream) const override |
void | setAltitudeMode (const AltitudeMode altitudeMode) |
void | setExtrude (bool extrude) |
void | unpack (QDataStream &stream) override |
![]() | |
GeoDataObject (const GeoDataObject &) | |
QString | id () const |
GeoDataObject & | operator= (const GeoDataObject &) |
void | pack (QDataStream &stream) const override |
GeoDataObject * | parent () |
const GeoDataObject * | parent () const |
QString | resolvePath (const QString &relativePath) const |
void | setId (const QString &value) |
void | setParent (GeoDataObject *parent) |
void | setTargetId (const QString &value) |
QString | targetId () const |
void | unpack (QDataStream &steam) override |
![]() |
Protected Member Functions | |
GeoDataLineString (GeoDataLineStringPrivate *priv) | |
![]() | |
GeoDataGeometry (const GeoDataGeometry &other) | |
GeoDataGeometry (GeoDataGeometryPrivate *priv) | |
bool | equals (const GeoDataGeometry &other) const |
virtual bool | equals (const GeoDataObject &other) const |
GeoDataGeometry & | operator= (const GeoDataGeometry &other) |
![]() |
Additional Inherited Members | |
![]() | |
GeoDataGeometryPrivate * | d_ptr |
Detailed Description
A LineString that allows to store a contiguous set of line segments.
GeoDataLineString is a tool class that implements the LineString tag/class of the Open Geospatial Consortium standard KML 2.2.
GeoDataLineString extends GeoDataGeometry to store and edit LineStrings.
In the QPainter API "pure" LineStrings are also referred to as "polylines". As such they are similar to the outline of a non-closed QPolygon.
Whenever a LineString is painted GeoDataLineStyle should be used to assign a color and line width.
A GeoDataLineString consists of several (geodetic) nodes which are each connected through line segments. The nodes are stored as GeoDataCoordinates objects.
The API which provides access to the nodes is similar to the API of QList.
GeoDataLineString allows LineStrings to be tessellated in order to make them follow the terrain and the curvature of the earth. The tessellation options allow for different ways of visualization:
- Not tessellated: A LineString that connects each two nodes directly and straight in screen coordinate space.
- A tessellated line: Each line segment is bent so that the LineString follows the curvature of the earth and its terrain. A tessellated line segment connects two nodes at the shortest possible distance ("along great circles").
- A tessellated line that follows latitude circles whenever possible: In this case Latitude circles are followed as soon as two subsequent nodes have exactly the same amount of latitude. In all other places the line segments follow great circles.
Some convenience methods have been added that allow to calculate the geodesic bounding box or the length of a LineString.
Definition at line 65 of file GeoDataLineString.h.
Member Typedef Documentation
◆ ConstIterator
using Marble::GeoDataLineString::ConstIterator = QList<GeoDataCoordinates>::ConstIterator |
Definition at line 69 of file GeoDataLineString.h.
◆ Iterator
using Marble::GeoDataLineString::Iterator = QList<GeoDataCoordinates>::Iterator |
Definition at line 68 of file GeoDataLineString.h.
Constructor & Destructor Documentation
◆ GeoDataLineString() [1/3]
|
explicit |
Creates a new LineString.
Definition at line 20 of file GeoDataLineString.cpp.
◆ GeoDataLineString() [2/3]
|
explicit |
Creates a LineString from an existing geometry object.
Definition at line 32 of file GeoDataLineString.cpp.
◆ ~GeoDataLineString()
|
override |
Destroys a LineString.
Definition at line 38 of file GeoDataLineString.cpp.
◆ GeoDataLineString() [3/3]
|
explicitprotected |
Definition at line 26 of file GeoDataLineString.cpp.
Member Function Documentation
◆ append() [1/2]
void Marble::GeoDataLineString::append | ( | const GeoDataCoordinates & | value | ) |
Appends a given geodesic position as a new node to the LineString.
Definition at line 406 of file GeoDataLineString.cpp.
◆ append() [2/2]
void Marble::GeoDataLineString::append | ( | const QList< GeoDataCoordinates > & | values | ) |
Appends a given geodesic position as new nodes to the LineString.
Definition at line 424 of file GeoDataLineString.cpp.
◆ at() [1/2]
GeoDataCoordinates & Marble::GeoDataLineString::at | ( | int | pos | ) |
Returns a reference to the coordinates of a node at a given position. This method detaches the returned coordinate object from the line string.
Definition at line 280 of file GeoDataLineString.cpp.
◆ at() [2/2]
const GeoDataCoordinates & Marble::GeoDataLineString::at | ( | int | pos | ) | const |
Returns a reference to the coordinates of a node at a given position. This method does not detach the returned coordinate object from the line string.
Definition at line 290 of file GeoDataLineString.cpp.
◆ begin() [1/2]
QList< GeoDataCoordinates >::Iterator Marble::GeoDataLineString::begin | ( | ) |
Returns an iterator that points to the begin of the LineString.
Definition at line 354 of file GeoDataLineString.cpp.
◆ begin() [2/2]
QList< GeoDataCoordinates >::ConstIterator Marble::GeoDataLineString::begin | ( | ) | const |
Definition at line 362 of file GeoDataLineString.cpp.
◆ clear()
void Marble::GeoDataLineString::clear | ( | ) |
Destroys all nodes in a LineString.
Definition at line 500 of file GeoDataLineString.cpp.
◆ constBegin()
QList< GeoDataCoordinates >::ConstIterator Marble::GeoDataLineString::constBegin | ( | ) | const |
Returns a const iterator that points to the begin of the LineString.
Definition at line 382 of file GeoDataLineString.cpp.
◆ constEnd()
QList< GeoDataCoordinates >::ConstIterator Marble::GeoDataLineString::constEnd | ( | ) | const |
Returns a const iterator that points to the end of the LineString.
Definition at line 388 of file GeoDataLineString.cpp.
◆ copy()
|
overridevirtual |
Implements Marble::GeoDataGeometry.
Definition at line 55 of file GeoDataLineString.cpp.
◆ end() [1/2]
QList< GeoDataCoordinates >::Iterator Marble::GeoDataLineString::end | ( | ) |
Returns an iterator that points to the end of the LineString.
Definition at line 368 of file GeoDataLineString.cpp.
◆ end() [2/2]
QList< GeoDataCoordinates >::ConstIterator Marble::GeoDataLineString::end | ( | ) | const |
Definition at line 376 of file GeoDataLineString.cpp.
◆ erase() [1/2]
QList< GeoDataCoordinates >::Iterator Marble::GeoDataLineString::erase | ( | const QList< GeoDataCoordinates >::Iterator & | begin, |
const QList< GeoDataCoordinates >::Iterator & | end ) |
Removes the nodes within the given range and returns them.
Definition at line 832 of file GeoDataLineString.cpp.
◆ erase() [2/2]
QList< GeoDataCoordinates >::Iterator Marble::GeoDataLineString::erase | ( | const QList< GeoDataCoordinates >::Iterator & | position | ) |
Removes the node at the given position and returns it.
Definition at line 820 of file GeoDataLineString.cpp.
◆ first() [1/2]
GeoDataCoordinates & Marble::GeoDataLineString::first | ( | ) |
Returns a reference to the first node in the LineString. This method detaches the returned coordinate object from the line string.
Definition at line 334 of file GeoDataLineString.cpp.
◆ first() [2/2]
const GeoDataCoordinates & Marble::GeoDataLineString::first | ( | ) | const |
Returns a reference to the first node in the LineString. This method does not detach the returned coordinate object from the line string.
Definition at line 348 of file GeoDataLineString.cpp.
◆ geometryId()
|
overridevirtual |
Implements Marble::GeoDataGeometry.
Definition at line 50 of file GeoDataLineString.cpp.
◆ insert()
void Marble::GeoDataLineString::insert | ( | int | index, |
const GeoDataCoordinates & | value ) |
Inserts a new node at the given index.
Definition at line 394 of file GeoDataLineString.cpp.
◆ isClosed()
|
virtual |
Returns whether a LineString is a closed polygon.
- Returns
false
if the LineString is not a LinearRing.
Reimplemented in Marble::GeoDataLinearRing.
Definition at line 513 of file GeoDataLineString.cpp.
◆ isEmpty()
bool Marble::GeoDataLineString::isEmpty | ( | ) | const |
Returns whether the LineString has no nodes at all.
- Returns
true
if there are no nodes inside the line string.
Definition at line 268 of file GeoDataLineString.cpp.
◆ last() [1/2]
GeoDataCoordinates & Marble::GeoDataLineString::last | ( | ) |
Returns a reference to the last node in the LineString. This method detaches the returned coordinate object from the line string.
Definition at line 324 of file GeoDataLineString.cpp.
◆ last() [2/2]
const GeoDataCoordinates & Marble::GeoDataLineString::last | ( | ) | const |
Returns a reference to the last node in the LineString. This method does not detach the returned coordinate object from the line string.
Definition at line 342 of file GeoDataLineString.cpp.
◆ latLonAltBox()
|
overridevirtual |
Returns the smallest latLonAltBox that contains the LineString.
- See also
- GeoDataLatLonAltBox
Reimplemented from Marble::GeoDataGeometry.
Definition at line 786 of file GeoDataLineString.cpp.
◆ length()
|
virtual |
Returns the length of LineString across a sphere starting from a coordinate in LineString This method can be used as an approximation for distances along LineStrings.
The unit used for the resulting length matches the unit of the planet radius.
- Parameters
-
planetRadius radius of the sphere offset position of coordinate within LineString
Reimplemented in Marble::GeoDataLinearRing.
Definition at line 802 of file GeoDataLineString.cpp.
◆ mid()
GeoDataLineString Marble::GeoDataLineString::mid | ( | int | pos, |
int | length = -1 ) const |
Returns a sub-string which contains elements from this vector, starting at position pos.
If length is -1 (the default), all elements after pos are included; otherwise length elements (or all remaining elements if there are less than length elements) are included.
Definition at line 306 of file GeoDataLineString.cpp.
◆ nodeType()
|
overridevirtual |
Provides type information for downcasting a GeoNode.
Implements Marble::GeoNode.
Definition at line 45 of file GeoDataLineString.cpp.
◆ operator!=()
bool Marble::GeoDataLineString::operator!= | ( | const GeoDataLineString & | other | ) | const |
Definition at line 495 of file GeoDataLineString.cpp.
◆ operator<<() [1/2]
GeoDataLineString & Marble::GeoDataLineString::operator<< | ( | const GeoDataCoordinates & | value | ) |
Appends a given geodesic position as a new node to the LineString.
Definition at line 437 of file GeoDataLineString.cpp.
◆ operator<<() [2/2]
GeoDataLineString & Marble::GeoDataLineString::operator<< | ( | const GeoDataLineString & | lineString | ) |
Appends a given LineString to the end of the LineString.
Definition at line 450 of file GeoDataLineString.cpp.
◆ operator==()
bool Marble::GeoDataLineString::operator== | ( | const GeoDataLineString & | other | ) | const |
Returns true/false depending on whether this and other are/are not equal.
Definition at line 471 of file GeoDataLineString.cpp.
◆ operator[]() [1/2]
GeoDataCoordinates & Marble::GeoDataLineString::operator[] | ( | int | pos | ) |
Returns a reference to the coordinates of a node at a given position. This method detaches the returned coordinate object from the line string.
Definition at line 296 of file GeoDataLineString.cpp.
◆ operator[]() [2/2]
const GeoDataCoordinates & Marble::GeoDataLineString::operator[] | ( | int | pos | ) | const |
Returns a reference to the coordinates of a node at a given position. This method does not detach the returned coordinate object from the line string.
Definition at line 318 of file GeoDataLineString.cpp.
◆ optimized()
GeoDataLineString Marble::GeoDataLineString::optimized | ( | ) | const |
Returns a linestring with detail values assigned to each node.
Definition at line 854 of file GeoDataLineString.cpp.
◆ pack()
|
override |
Serialize the LineString to a stream.
- Parameters
-
stream the stream.
Definition at line 893 of file GeoDataLineString.cpp.
◆ remove()
void Marble::GeoDataLineString::remove | ( | int | i | ) |
Removes the node at the given position and destroys it.
Definition at line 844 of file GeoDataLineString.cpp.
◆ reserve()
void Marble::GeoDataLineString::reserve | ( | int | size | ) |
Attempts to allocate memory for at least size coordinates.
Definition at line 418 of file GeoDataLineString.cpp.
◆ reverse()
void Marble::GeoDataLineString::reverse | ( | ) |
◆ setTessellate()
void Marble::GeoDataLineString::setTessellate | ( | bool | tessellate | ) |
Sets the tessellation property for the LineString.
If tessellate is true
then the LineString's line segments are bent and follow the earth's surface and terrain along great circles. If tessellate is false
then the LineString's line segments are rendered as straight lines in screen coordinate space.
Definition at line 524 of file GeoDataLineString.cpp.
◆ setTessellationFlags()
void Marble::GeoDataLineString::setTessellationFlags | ( | TessellationFlags | f | ) |
Sets the given tessellation flags for a LineString.
Definition at line 547 of file GeoDataLineString.cpp.
◆ size()
int Marble::GeoDataLineString::size | ( | ) | const |
Returns the number of nodes in a LineString.
Definition at line 274 of file GeoDataLineString.cpp.
◆ tessellate()
bool Marble::GeoDataLineString::tessellate | ( | ) | const |
Returns whether the LineString follows the earth's surface.
- Returns
true
if the LineString's line segments follow the earth's surface and terrain along great circles.
Definition at line 518 of file GeoDataLineString.cpp.
◆ tessellationFlags()
TessellationFlags Marble::GeoDataLineString::tessellationFlags | ( | ) | const |
Returns the tessellation flags for a LineString.
Definition at line 541 of file GeoDataLineString.cpp.
◆ toDateLineCorrected()
|
virtual |
The line string corrected for date line crossing.
- Returns
- A set of LineStrings that don't cross the dateline and which resemble the original linestring.
Deprecation Warning: This method will likely be removed from the public API.
Definition at line 612 of file GeoDataLineString.cpp.
◆ toNormalized()
|
virtual |
The line string with nodes that have proper longitude/latitude ranges.
- Returns
- A LineString that resembles the original linestring with nodes that have longitude values between -180 and +180 deg and that feature latitude values between -90 and +90 deg.
Deprecation Warning: This method will likely be removed from the public API.
Definition at line 567 of file GeoDataLineString.cpp.
◆ toPoleCorrected()
|
virtual |
The line string with more generic pole values.
- Returns
- A LineString that resembles the original linestring. Nodes that represent one of the poles are duplicated to allow for a better visualization of flat projections.
Deprecation Warning: This method will likely be removed from the public API.
Definition at line 623 of file GeoDataLineString.cpp.
◆ toRangeCorrected()
|
virtual |
Provides a more generic representation of the LineString.
The LineString is normalized, and pole corrected.
Deprecation Warning: This method will likely be removed from the public API.
Definition at line 594 of file GeoDataLineString.cpp.
◆ toVariantList()
QVariantList Marble::GeoDataLineString::toVariantList | ( | ) | const |
Returns a javascript-style list (that can be used e.g. with the QML GeoPolyline element).
Definition at line 869 of file GeoDataLineString.cpp.
◆ unpack()
|
override |
Unserialize the LineString from a stream.
- Parameters
-
stream the stream.
Definition at line 909 of file GeoDataLineString.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2024 The KDE developers.
Generated on Fri Dec 20 2024 11:52:13 by doxygen 1.12.0 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.