Marble::GeoDataLinearRing
#include <GeoDataLinearRing.h>
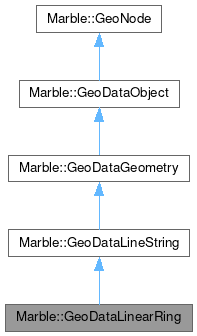
Public Member Functions | |
GeoDataLinearRing (const GeoDataGeometry &other) | |
GeoDataLinearRing (TessellationFlags f=NoTessellation) | |
~GeoDataLinearRing () override | |
virtual bool | contains (const GeoDataCoordinates &coordinates) const |
GeoDataGeometry * | copy () const override |
EnumGeometryId | geometryId () const override |
virtual bool | isClockwise () const |
bool | isClosed () const override |
qreal | length (qreal planetRadius, int offset=0) const override |
const char * | nodeType () const override |
bool | operator!= (const GeoDataLinearRing &other) const |
bool | operator== (const GeoDataLinearRing &other) const |
![]() | |
GeoDataLineString (const GeoDataGeometry &other) | |
GeoDataLineString (TessellationFlags f=NoTessellation) | |
~GeoDataLineString () override | |
void | append (const GeoDataCoordinates &value) |
void | append (const QVector< GeoDataCoordinates > &values) |
GeoDataCoordinates & | at (int pos) |
const GeoDataCoordinates & | at (int pos) const |
QVector< GeoDataCoordinates >::Iterator | begin () |
QVector< GeoDataCoordinates >::ConstIterator | begin () const |
void | clear () |
QVector< GeoDataCoordinates >::ConstIterator | constBegin () const |
QVector< GeoDataCoordinates >::ConstIterator | constEnd () const |
GeoDataGeometry * | copy () const override |
QVector< GeoDataCoordinates >::Iterator | end () |
QVector< GeoDataCoordinates >::ConstIterator | end () const |
QVector< GeoDataCoordinates >::Iterator | erase (const QVector< GeoDataCoordinates >::Iterator &begin, const QVector< GeoDataCoordinates >::Iterator &end) |
QVector< GeoDataCoordinates >::Iterator | erase (const QVector< GeoDataCoordinates >::Iterator &position) |
GeoDataCoordinates & | first () |
const GeoDataCoordinates & | first () const |
EnumGeometryId | geometryId () const override |
void | insert (int index, const GeoDataCoordinates &value) |
bool | isEmpty () const |
GeoDataCoordinates & | last () |
const GeoDataCoordinates & | last () const |
const GeoDataLatLonAltBox & | latLonAltBox () const override |
GeoDataLineString | mid (int pos, int length=-1) const |
const char * | nodeType () const override |
bool | operator!= (const GeoDataLineString &other) const |
GeoDataLineString & | operator<< (const GeoDataCoordinates &value) |
GeoDataLineString & | operator<< (const GeoDataLineString &lineString) |
bool | operator== (const GeoDataLineString &other) const |
GeoDataCoordinates & | operator[] (int pos) |
const GeoDataCoordinates & | operator[] (int pos) const |
GeoDataLineString | optimized () const |
void | pack (QDataStream &stream) const override |
void | remove (int i) |
void | reserve (int size) |
void | reverse () |
void | setTessellate (bool tessellate) |
void | setTessellationFlags (TessellationFlags f) |
int | size () const |
bool | tessellate () const |
TessellationFlags | tessellationFlags () const |
virtual QVector< GeoDataLineString * > | toDateLineCorrected () const |
virtual GeoDataLineString | toNormalized () const |
virtual GeoDataLineString | toPoleCorrected () const |
virtual GeoDataLineString | toRangeCorrected () const |
QVariantList | toVariantList () const |
void | unpack (QDataStream &stream) override |
![]() | |
AltitudeMode | altitudeMode () const |
void | detach () |
bool | extrude () const |
bool | operator!= (const GeoDataGeometry &other) const |
bool | operator== (const GeoDataGeometry &other) const |
void | pack (QDataStream &stream) const override |
void | setAltitudeMode (const AltitudeMode altitudeMode) |
void | setExtrude (bool extrude) |
void | unpack (QDataStream &stream) override |
![]() | |
GeoDataObject (const GeoDataObject &) | |
QString | id () const |
GeoDataObject & | operator= (const GeoDataObject &) |
void | pack (QDataStream &stream) const override |
GeoDataObject * | parent () |
const GeoDataObject * | parent () const |
QString | resolvePath (const QString &relativePath) const |
void | setId (const QString &value) |
void | setParent (GeoDataObject *parent) |
void | setTargetId (const QString &value) |
QString | targetId () const |
void | unpack (QDataStream &steam) override |
Additional Inherited Members | |
![]() | |
using | ConstIterator = QVector<GeoDataCoordinates>::ConstIterator |
using | Iterator = QVector<GeoDataCoordinates>::Iterator |
![]() | |
GeoDataLineString (GeoDataLineStringPrivate *priv) | |
![]() | |
GeoDataGeometry (const GeoDataGeometry &other) | |
GeoDataGeometry (GeoDataGeometryPrivate *priv) | |
bool | equals (const GeoDataGeometry &other) const |
virtual bool | equals (const GeoDataObject &other) const |
GeoDataGeometry & | operator= (const GeoDataGeometry &other) |
![]() | |
GeoDataGeometryPrivate * | d_ptr |
Detailed Description
A LinearRing that allows to store a closed, contiguous set of line segments.
GeoDataLinearRing is a tool class that implements the LinearRing tag/class of the Open Geospatial Consortium standard KML 2.2.
Unlike suggested in the KML spec GeoDataLinearRing extends GeoDataLineString to store a closed LineString (the KML specification suggests to inherit from the Geometry class directly).
In the QPainter API LinearRings are also referred to as "polygons". As such they are similar to QPolygons.
Whenever a LinearRing is painted GeoDataLineStyle should be used to assign a color and line width.
A GeoDataLinearRing consists of several (geodetic) nodes which are each connected through line segments. The nodes are stored as GeoDataCoordinates objects.
The API which provides access to the nodes is similar to the API of QVector.
GeoDataLinearRing allows LinearRings to be tessellated in order to make them follow the terrain and the curvature of the earth. The tessellation options allow for different ways of visualization:
- Not tessellated: A LinearRing that connects each two nodes directly and straight in screen coordinate space.
- A tessellated line: Each line segment is bent so that the LinearRing follows the curvature of the earth and its terrain. A tessellated line segment connects two nodes at the shortest possible distance ("along great circles").
- A tessellated line that follows latitude circles whenever possible: In this case Latitude circles are followed as soon as two subsequent nodes have exactly the same amount of latitude. In all other places the line segments follow great circles.
Some convenience methods have been added that allow to calculate the geodesic bounding box or the length of a LinearRing.
Definition at line 62 of file GeoDataLinearRing.h.
Constructor & Destructor Documentation
◆ GeoDataLinearRing() [1/2]
|
explicit |
Creates a new LinearRing.
Definition at line 16 of file GeoDataLinearRing.cpp.
◆ GeoDataLinearRing() [2/2]
|
explicit |
Creates a LinearRing from an existing geometry object.
Definition at line 21 of file GeoDataLinearRing.cpp.
◆ ~GeoDataLinearRing()
|
override |
Destroys a LinearRing.
Definition at line 26 of file GeoDataLinearRing.cpp.
Member Function Documentation
◆ contains()
|
virtual |
Returns whether the given coordinates lie within the polygon.
- Returns
true
if the coordinates lie within the polygon, false otherwise.
Definition at line 68 of file GeoDataLinearRing.cpp.
◆ copy()
|
overridevirtual |
Implements Marble::GeoDataGeometry.
Definition at line 40 of file GeoDataLinearRing.cpp.
◆ geometryId()
|
overridevirtual |
Implements Marble::GeoDataGeometry.
Definition at line 35 of file GeoDataLinearRing.cpp.
◆ isClockwise()
|
virtual |
Returns whether the orientaion of ring is coloskwise or not.
- Returns
- Return value is true if ring is clockwise orientated
Definition at line 96 of file GeoDataLinearRing.cpp.
◆ isClosed()
|
overridevirtual |
Returns whether a LinearRing is a closed polygon.
- Returns
true
for a LinearRing.
Reimplemented from Marble::GeoDataLineString.
Definition at line 56 of file GeoDataLinearRing.cpp.
◆ length()
|
overridevirtual |
Returns the length of the LinearRing across a sphere.
As a parameter the planetRadius needs to be passed.
- Returns
- The return value is the length of the LinearRing. The unit used for the resulting length matches the unit of the planet radius.
This method can be used as an approximation for the circumference of a LinearRing.
Reimplemented from Marble::GeoDataLineString.
Definition at line 61 of file GeoDataLinearRing.cpp.
◆ nodeType()
|
overridevirtual |
Provides type information for downcasting a GeoNode.
Implements Marble::GeoNode.
Definition at line 30 of file GeoDataLinearRing.cpp.
◆ operator!=()
bool Marble::GeoDataLinearRing::operator!= | ( | const GeoDataLinearRing & | other | ) | const |
Definition at line 51 of file GeoDataLinearRing.cpp.
◆ operator==()
bool Marble::GeoDataLinearRing::operator== | ( | const GeoDataLinearRing & | other | ) | const |
Returns true/false depending on whether this and other are/are not equal.
Definition at line 45 of file GeoDataLinearRing.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2024 The KDE developers.
Generated on Fri Jul 26 2024 11:57:58 by doxygen 1.11.0 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.