Marble::GeoDataFolder
#include <GeoDataFolder.h>
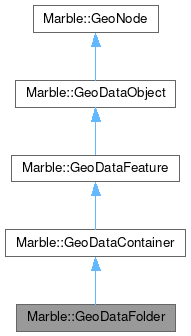
Additional Inherited Members | |
![]() | |
GeoDataContainer (const GeoDataContainer &other, GeoDataContainerPrivate *priv) | |
GeoDataContainer (GeoDataContainerPrivate *priv) | |
bool | equals (const GeoDataContainer &other) const |
bool | equals (const GeoDataFeature &other) const |
![]() | |
GeoDataFeature (const GeoDataFeature &other, GeoDataFeaturePrivate *dd) | |
GeoDataFeature (GeoDataFeaturePrivate *dd) | |
bool | equals (const GeoDataFeature &other) const |
virtual bool | equals (const GeoDataObject &other) const |
![]() | |
GeoDataFeaturePrivate *const | d_ptr |
Detailed Description
A container that is used to arrange other GeoDataFeatures.
A GeoDataFolder is used to arrange other GeoDataFeatures hierarchically (Folders, Placemarks, NetworkLinks, or Overlays). A GeoDataFeature is visible only if it and all its ancestors are visible.
- See also
- GeoDataFeature
- GeoDataContainer
Definition at line 32 of file GeoDataFolder.h.
Constructor & Destructor Documentation
◆ GeoDataFolder() [1/2]
Marble::GeoDataFolder::GeoDataFolder | ( | ) |
Definition at line 21 of file GeoDataFolder.cpp.
◆ GeoDataFolder() [2/2]
Marble::GeoDataFolder::GeoDataFolder | ( | const GeoDataFolder & | other | ) |
Definition at line 26 of file GeoDataFolder.cpp.
Member Function Documentation
◆ clone()
|
overridevirtual |
Duplicate into another equal instance.
Implements Marble::GeoDataFeature.
Definition at line 58 of file GeoDataFolder.cpp.
◆ nodeType()
|
overridevirtual |
Provides type information for downcasting a GeoNode.
Implements Marble::GeoNode.
Definition at line 53 of file GeoDataFolder.cpp.
◆ operator!=()
bool Marble::GeoDataFolder::operator!= | ( | const GeoDataFolder & | other | ) | const |
Definition at line 48 of file GeoDataFolder.cpp.
◆ operator=()
GeoDataFolder & Marble::GeoDataFolder::operator= | ( | const GeoDataFolder & | other | ) |
Definition at line 33 of file GeoDataFolder.cpp.
◆ operator==()
bool Marble::GeoDataFolder::operator== | ( | const GeoDataFolder & | other | ) | const |
Definition at line 43 of file GeoDataFolder.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2025 The KDE developers.
Generated on Fri May 2 2025 12:01:36 by doxygen 1.13.2 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.