Marble::GeoDataStyle
#include <GeoDataStyle.h>
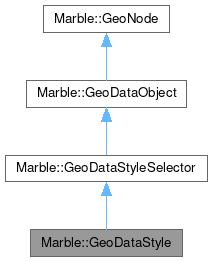
Public Types | |
using | ConstPtr = QSharedPointer<const GeoDataStyle> |
using | Ptr = QSharedPointer<GeoDataStyle> |
Public Member Functions | |
GeoDataStyle () | |
GeoDataStyle (const GeoDataStyle &other) | |
GeoDataStyle (const QString &iconPath, const QFont &font, const QColor &color) | |
GeoDataBalloonStyle & | balloonStyle () |
const GeoDataBalloonStyle & | balloonStyle () const |
GeoDataIconStyle & | iconStyle () |
const GeoDataIconStyle & | iconStyle () const |
GeoDataLabelStyle & | labelStyle () |
const GeoDataLabelStyle & | labelStyle () const |
GeoDataLineStyle & | lineStyle () |
const GeoDataLineStyle & | lineStyle () const |
GeoDataListStyle & | listStyle () |
const GeoDataListStyle & | listStyle () const |
const char * | nodeType () const override |
bool | operator!= (const GeoDataStyle &other) const |
GeoDataStyle & | operator= (const GeoDataStyle &other) |
bool | operator== (const GeoDataStyle &other) const |
void | pack (QDataStream &stream) const override |
GeoDataPolyStyle & | polyStyle () |
const GeoDataPolyStyle & | polyStyle () const |
void | setBalloonStyle (const GeoDataBalloonStyle &style) |
void | setIconStyle (const GeoDataIconStyle &style) |
void | setLabelStyle (const GeoDataLabelStyle &style) |
void | setLineStyle (const GeoDataLineStyle &style) |
void | setListStyle (const GeoDataListStyle &style) |
void | setPolyStyle (const GeoDataPolyStyle &style) |
void | unpack (QDataStream &stream) override |
![]() | |
bool | operator!= (const GeoDataStyleSelector &other) const |
GeoDataStyleSelector & | operator= (const GeoDataStyleSelector &other) |
bool | operator== (const GeoDataStyleSelector &other) const |
void | pack (QDataStream &stream) const override |
void | unpack (QDataStream &stream) override |
![]() | |
GeoDataObject (const GeoDataObject &) | |
QString | id () const |
GeoDataObject & | operator= (const GeoDataObject &) |
void | pack (QDataStream &stream) const override |
GeoDataObject * | parent () |
const GeoDataObject * | parent () const |
QString | resolvePath (const QString &relativePath) const |
void | setId (const QString &value) |
void | setParent (GeoDataObject *parent) |
void | setTargetId (const QString &value) |
QString | targetId () const |
void | unpack (QDataStream &steam) override |
Additional Inherited Members | |
![]() | |
GeoDataStyleSelector (const GeoDataStyleSelector &other) | |
![]() | |
virtual bool | equals (const GeoDataObject &other) const |
Detailed Description
an addressable style group
A GeoDataStyle defines an addressable style group that can be referenced by GeoDataStyleMaps and GeoDataFeatures. GeoDataStyles affect how Geometry is presented in the 3D viewer (not yet implemented) and how Features appear. Shared styles are collected in a GeoDataDocument and must have an id defined for them so that they can be referenced by the individual Features that use them.
- See also
- GeoDataIconStyle
- GeoDataLabelStyle
- GeoDataLineStyle
- GeoDataPolyStyle
- GeoDataBalloonStyle
- GeoDataListStyle
Definition at line 48 of file GeoDataStyle.h.
Member Typedef Documentation
◆ ConstPtr
using Marble::GeoDataStyle::ConstPtr = QSharedPointer<const GeoDataStyle> |
Definition at line 52 of file GeoDataStyle.h.
◆ Ptr
Definition at line 51 of file GeoDataStyle.h.
Constructor & Destructor Documentation
◆ GeoDataStyle() [1/3]
Marble::GeoDataStyle::GeoDataStyle | ( | ) |
Construct a default style.
Definition at line 41 of file GeoDataStyle.cpp.
◆ GeoDataStyle() [2/3]
Marble::GeoDataStyle::GeoDataStyle | ( | const GeoDataStyle & | other | ) |
Definition at line 46 of file GeoDataStyle.cpp.
◆ GeoDataStyle() [3/3]
Marble::GeoDataStyle::GeoDataStyle | ( | const QString & | iconPath, |
const QFont & | font, | ||
const QColor & | color ) |
Construct a new style.
- Parameters
-
iconPath used to construct the icon style font used to construct the label styles color used to construct the label styles
Definition at line 52 of file GeoDataStyle.cpp.
◆ ~GeoDataStyle()
|
override |
Definition at line 57 of file GeoDataStyle.cpp.
Member Function Documentation
◆ balloonStyle() [1/2]
GeoDataBalloonStyle & Marble::GeoDataStyle::balloonStyle | ( | ) |
Return the balloon style of this style.
Definition at line 161 of file GeoDataStyle.cpp.
◆ balloonStyle() [2/2]
const GeoDataBalloonStyle & Marble::GeoDataStyle::balloonStyle | ( | ) | const |
Definition at line 166 of file GeoDataStyle.cpp.
◆ iconStyle() [1/2]
GeoDataIconStyle & Marble::GeoDataStyle::iconStyle | ( | ) |
Return the icon style of this style.
Definition at line 121 of file GeoDataStyle.cpp.
◆ iconStyle() [2/2]
const GeoDataIconStyle & Marble::GeoDataStyle::iconStyle | ( | ) | const |
Definition at line 126 of file GeoDataStyle.cpp.
◆ labelStyle() [1/2]
GeoDataLabelStyle & Marble::GeoDataStyle::labelStyle | ( | ) |
Return the label style of this style.
Definition at line 151 of file GeoDataStyle.cpp.
◆ labelStyle() [2/2]
const GeoDataLabelStyle & Marble::GeoDataStyle::labelStyle | ( | ) | const |
Definition at line 156 of file GeoDataStyle.cpp.
◆ lineStyle() [1/2]
GeoDataLineStyle & Marble::GeoDataStyle::lineStyle | ( | ) |
Return the label style of this style.
Definition at line 131 of file GeoDataStyle.cpp.
◆ lineStyle() [2/2]
const GeoDataLineStyle & Marble::GeoDataStyle::lineStyle | ( | ) | const |
Definition at line 136 of file GeoDataStyle.cpp.
◆ listStyle() [1/2]
GeoDataListStyle & Marble::GeoDataStyle::listStyle | ( | ) |
Return the list style of this style.
Definition at line 171 of file GeoDataStyle.cpp.
◆ listStyle() [2/2]
const GeoDataListStyle & Marble::GeoDataStyle::listStyle | ( | ) | const |
Definition at line 176 of file GeoDataStyle.cpp.
◆ nodeType()
|
overridevirtual |
Provides type information for downcasting a GeoNode.
Implements Marble::GeoNode.
Definition at line 84 of file GeoDataStyle.cpp.
◆ operator!=()
bool Marble::GeoDataStyle::operator!= | ( | const GeoDataStyle & | other | ) | const |
Definition at line 79 of file GeoDataStyle.cpp.
◆ operator=()
GeoDataStyle & Marble::GeoDataStyle::operator= | ( | const GeoDataStyle & | other | ) |
assignment operator
- Parameters
-
other the GeoDataStyle that gets duplicated
Definition at line 62 of file GeoDataStyle.cpp.
◆ operator==()
bool Marble::GeoDataStyle::operator== | ( | const GeoDataStyle & | other | ) | const |
Definition at line 69 of file GeoDataStyle.cpp.
◆ pack()
|
override |
Serialize the style to a stream.
- Parameters
-
stream the stream
Definition at line 181 of file GeoDataStyle.cpp.
◆ polyStyle() [1/2]
GeoDataPolyStyle & Marble::GeoDataStyle::polyStyle | ( | ) |
Return the label style of this style.
Definition at line 141 of file GeoDataStyle.cpp.
◆ polyStyle() [2/2]
const GeoDataPolyStyle & Marble::GeoDataStyle::polyStyle | ( | ) | const |
Definition at line 146 of file GeoDataStyle.cpp.
◆ setBalloonStyle()
void Marble::GeoDataStyle::setBalloonStyle | ( | const GeoDataBalloonStyle & | style | ) |
set the balloon style
Definition at line 110 of file GeoDataStyle.cpp.
◆ setIconStyle()
void Marble::GeoDataStyle::setIconStyle | ( | const GeoDataIconStyle & | style | ) |
set the icon style
Definition at line 89 of file GeoDataStyle.cpp.
◆ setLabelStyle()
void Marble::GeoDataStyle::setLabelStyle | ( | const GeoDataLabelStyle & | style | ) |
set the label style
Definition at line 100 of file GeoDataStyle.cpp.
◆ setLineStyle()
void Marble::GeoDataStyle::setLineStyle | ( | const GeoDataLineStyle & | style | ) |
set the line style
Definition at line 95 of file GeoDataStyle.cpp.
◆ setListStyle()
void Marble::GeoDataStyle::setListStyle | ( | const GeoDataListStyle & | style | ) |
set the list style
Definition at line 115 of file GeoDataStyle.cpp.
◆ setPolyStyle()
void Marble::GeoDataStyle::setPolyStyle | ( | const GeoDataPolyStyle & | style | ) |
set the poly style
Definition at line 105 of file GeoDataStyle.cpp.
◆ unpack()
|
override |
Unserialize the style from a stream.
- Parameters
-
stream the stream
Definition at line 193 of file GeoDataStyle.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2025 The KDE developers.
Generated on Fri May 2 2025 12:01:36 by doxygen 1.13.2 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.