Marble::GeoDataPolyStyle
#include <GeoDataPolyStyle.h>
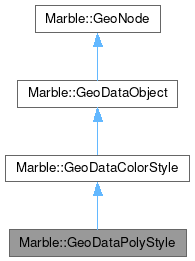
Additional Inherited Members | |
![]() | |
enum | ColorMode { Normal , Random } |
![]() | |
static QString | contrastColor (const QColor &color) |
![]() | |
virtual bool | equals (const GeoDataObject &other) const |
Detailed Description
specifies the style how polygons are drawn
A GeoDataPolyStyle specifies how Polygons are drawn in the viewer. A custom color, color mode (both inherited from GeoDataColorStyle) and two boolean values whether to fill and whether to draw the outline.
Definition at line 25 of file GeoDataPolyStyle.h.
Constructor & Destructor Documentation
◆ GeoDataPolyStyle() [1/3]
Marble::GeoDataPolyStyle::GeoDataPolyStyle | ( | ) |
Construct a new GeoDataPolyStyle.
Definition at line 38 of file GeoDataPolyStyle.cpp.
◆ GeoDataPolyStyle() [2/3]
Marble::GeoDataPolyStyle::GeoDataPolyStyle | ( | const GeoDataPolyStyle & | other | ) |
Definition at line 43 of file GeoDataPolyStyle.cpp.
◆ GeoDataPolyStyle() [3/3]
|
explicit |
Construct a new GeoDataPolyStyle.
- Parameters
-
color the color to use when showing the name
- See also
- GeoDataColorStyle
Definition at line 49 of file GeoDataPolyStyle.cpp.
◆ ~GeoDataPolyStyle()
|
override |
Definition at line 55 of file GeoDataPolyStyle.cpp.
Member Function Documentation
◆ brushStyle()
Qt::BrushStyle Marble::GeoDataPolyStyle::brushStyle | ( | ) | const |
◆ colorIndex()
quint8 Marble::GeoDataPolyStyle::colorIndex | ( | ) | const |
Return the value of color index.
- Returns
- Color index
Definition at line 121 of file GeoDataPolyStyle.cpp.
◆ fill()
bool Marble::GeoDataPolyStyle::fill | ( | ) | const |
Return true if polygons get filled.
- Returns
- whether to fill
Definition at line 91 of file GeoDataPolyStyle.cpp.
◆ nodeType()
|
overridevirtual |
Provides type information for downcasting a GeoNode.
Reimplemented from Marble::GeoDataColorStyle.
Definition at line 81 of file GeoDataPolyStyle.cpp.
◆ operator!=()
bool Marble::GeoDataPolyStyle::operator!= | ( | const GeoDataPolyStyle & | other | ) | const |
Definition at line 76 of file GeoDataPolyStyle.cpp.
◆ operator=()
GeoDataPolyStyle & Marble::GeoDataPolyStyle::operator= | ( | const GeoDataPolyStyle & | other | ) |
assignment operator
Definition at line 60 of file GeoDataPolyStyle.cpp.
◆ operator==()
bool Marble::GeoDataPolyStyle::operator== | ( | const GeoDataPolyStyle & | other | ) | const |
Definition at line 67 of file GeoDataPolyStyle.cpp.
◆ outline()
bool Marble::GeoDataPolyStyle::outline | ( | ) | const |
Return true if outlines of polygons get drawn.
- Returns
- whether outline is drawn
Definition at line 101 of file GeoDataPolyStyle.cpp.
◆ pack()
|
override |
Serialize the style to a stream.
- Parameters
-
stream the stream
Definition at line 148 of file GeoDataPolyStyle.cpp.
◆ setBrushStyle()
void Marble::GeoDataPolyStyle::setBrushStyle | ( | const Qt::BrushStyle | style | ) |
◆ setColorIndex()
void Marble::GeoDataPolyStyle::setColorIndex | ( | quint8 | colorIndex | ) |
Set the color index which will be used to assign color to brush.
- Parameters
-
colorIndex The value of color index
Definition at line 116 of file GeoDataPolyStyle.cpp.
◆ setFill()
void Marble::GeoDataPolyStyle::setFill | ( | bool | fill | ) |
Set whether to fill the polygon.
- Parameters
-
fill
Definition at line 86 of file GeoDataPolyStyle.cpp.
◆ setOutline()
void Marble::GeoDataPolyStyle::setOutline | ( | bool | outline | ) |
Set whether to draw the outline.
- Parameters
-
outline
Definition at line 96 of file GeoDataPolyStyle.cpp.
◆ setTexturePath()
void Marble::GeoDataPolyStyle::setTexturePath | ( | const QString & | path | ) |
Definition at line 126 of file GeoDataPolyStyle.cpp.
◆ textureImage()
QImage Marble::GeoDataPolyStyle::textureImage | ( | ) | const |
Definition at line 137 of file GeoDataPolyStyle.cpp.
◆ texturePath()
QString Marble::GeoDataPolyStyle::texturePath | ( | ) | const |
Definition at line 132 of file GeoDataPolyStyle.cpp.
◆ unpack()
|
override |
Unserialize the style from a stream.
- Parameters
-
stream the stream
Definition at line 157 of file GeoDataPolyStyle.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2025 The KDE developers.
Generated on Fri May 2 2025 12:01:36 by doxygen 1.13.2 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.