Marble::MapThemeManager
#include <MapThemeManager.h>
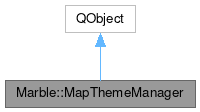
Signals | |
void | themesChanged () |
Additional Inherited Members | |
![]() | |
objectName | |
![]() | |
typedef | QObjectList |
![]() | |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const QMetaMethod &signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const QMetaMethod &signal) |
bool | isSignalConnected (const QMetaMethod &signal) const const |
int | receivers (const char *signal) const const |
QObject * | sender () const const |
int | senderSignalIndex () const const |
virtual void | timerEvent (QTimerEvent *event) |
Detailed Description
The class that handles map themes that are locally available .
This class which is able to check for maps that are locally available. After parsing the data it only stores the name, description and path into a QStandardItemModel.
The MapThemeManager is not owned by the MarbleWidget/Map itself. Instead it is owned by the widget or application that contains MarbleWidget/Map ( usually: the ControlView convenience class )
For convenience MarbleThemeManager provides a static helper class that loads the properties of a map theme into a GeoSceneDocument object.
- See also
- GeoSceneDocument
Definition at line 42 of file MapThemeManager.h.
Constructor & Destructor Documentation
◆ MapThemeManager()
Definition at line 128 of file MapThemeManager.cpp.
◆ ~MapThemeManager()
|
override |
Definition at line 139 of file MapThemeManager.cpp.
Member Function Documentation
◆ celestialBodiesModel()
QStandardItemModel * MapThemeManager::celestialBodiesModel | ( | ) |
Provides a model of all installed planets.
Definition at line 336 of file MapThemeManager.cpp.
◆ createMapThemeFromOverlay()
|
static |
Returns a map as a GeoSceneDocument object created from a GeoDataPhotoOverlay.
Definition at line 530 of file MapThemeManager.cpp.
◆ deleteMapTheme()
Deletes the map theme with the specified map theme ID.
- Parameters
-
mapThemeId ID of the map theme to be deleted
Deletion will only succeed for local map themes, that is, if the map theme's directory structure resides in the user's home directory.
Definition at line 171 of file MapThemeManager.cpp.
◆ loadMapTheme()
|
static |
Returns the map theme as a GeoSceneDocument object.
- Parameters
-
mapThemeStringID the string ID that refers to the map theme
This helper method should only get used by MarbleModel to load the current theme into memory or by the MapThemeManager.
Definition at line 163 of file MapThemeManager.cpp.
◆ mapThemeIds()
QStringList MapThemeManager::mapThemeIds | ( | ) | const |
Returns a list of all locally available map theme IDs.
Definition at line 144 of file MapThemeManager.cpp.
◆ mapThemeModel()
QStandardItemModel * MapThemeManager::mapThemeModel | ( | ) |
Provides a model of the locally existing themes.
This method provides a QStandardItemModel of all themes that are available via MarbleDirs.
Definition at line 327 of file MapThemeManager.cpp.
◆ themesChanged
|
signal |
This signal will be emitted, when the themes change.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2024 The KDE developers.
Generated on Sat Apr 27 2024 22:12:41 by doxygen 1.10.0 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.