Marble::MarbleModel
#include <MarbleModel.h>
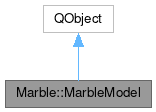
Properties | |
QString | mapThemeId |
bool | workOffline |
![]() | |
objectName | |
Signals | |
void | creatingTilesStart (TileCreator *, const QString &name, const QString &description) |
void | homeChanged (const GeoDataCoordinates &newHomePoint) |
void | themeChanged (const QString &mapTheme) |
void | trackedPlacemarkChanged (const GeoDataPlacemark *placemark) |
void | workOfflineChanged () |
Public Slots | |
void | clearPersistentTileCache () |
void | setPersistentTileCacheLimit (quint64 kiloBytes) |
void | setTrackedPlacemark (const GeoDataPlacemark *placemark) |
void | updateProperty (const QString &property, bool value) |
Public Member Functions | |
MarbleModel (QObject *parent=nullptr) | |
void | addGeoDataFile (const QString &filename) |
void | addGeoDataString (const QString &data, const QString &key=QLatin1String("data")) |
BookmarkManager * | bookmarkManager () |
MarbleClock * | clock () |
const MarbleClock * | clock () const |
QDateTime | clockDateTime () const |
int | clockSpeed () const |
int | clockTimezone () const |
HttpDownloadManager * | downloadManager () |
const HttpDownloadManager * | downloadManager () const |
ElevationModel * | elevationModel () |
const ElevationModel * | elevationModel () const |
FileManager * | fileManager () |
QAbstractItemModel * | groundOverlayModel () |
const QAbstractItemModel * | groundOverlayModel () const |
void | home (qreal &lon, qreal &lat, int &zoom) const |
QTextDocument * | legend () |
GeoSceneDocument * | mapTheme () |
const GeoSceneDocument * | mapTheme () const |
QString | mapThemeId () const |
quint64 | persistentTileCacheLimit () const |
QAbstractItemModel * | placemarkModel () |
const QAbstractItemModel * | placemarkModel () const |
QItemSelectionModel * | placemarkSelectionModel () |
const Planet * | planet () const |
QString | planetId () const |
QString | planetName () const |
qreal | planetRadius () const |
PluginManager * | pluginManager () |
const PluginManager * | pluginManager () const |
PositionTracking * | positionTracking () const |
void | removeGeoData (const QString &key) |
RoutingManager * | routingManager () |
const RoutingManager * | routingManager () const |
void | setClockDateTime (const QDateTime &datetime) |
void | setClockSpeed (int speed) |
void | setClockTimezone (int timeInSec) |
void | setHome (const GeoDataCoordinates &homePoint, int zoom=1050) |
void | setHome (qreal lon, qreal lat, int zoom=1050) |
void | setLegend (QTextDocument *document) |
void | setMapTheme (GeoSceneDocument *document) |
void | setMapThemeId (const QString &mapThemeId) |
void | setWorkOffline (bool workOffline) |
SunLocator * | sunLocator () |
const SunLocator * | sunLocator () const |
const GeoDataPlacemark * | trackedPlacemark () const |
GeoDataTreeModel * | treeModel () |
const GeoDataTreeModel * | treeModel () const |
quint64 | volatileTileCacheLimit () const |
bool | workOffline () const |
![]() | |
QObject (QObject *parent) | |
QBindable< QString > | bindableObjectName () |
bool | blockSignals (bool block) |
const QObjectList & | children () const const |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) const const |
bool | disconnect (const QObject *receiver, const char *method) const const |
void | dumpObjectInfo () const const |
void | dumpObjectTree () const const |
QList< QByteArray > | dynamicPropertyNames () const const |
virtual bool | event (QEvent *e) |
virtual bool | eventFilter (QObject *watched, QEvent *event) |
T | findChild (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QRegularExpression &re, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (Qt::FindChildOptions options) const const |
bool | inherits (const char *className) const const |
void | installEventFilter (QObject *filterObj) |
bool | isQuickItemType () const const |
bool | isWidgetType () const const |
bool | isWindowType () const const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const const |
void | moveToThread (QThread *targetThread) |
QString | objectName () const const |
void | objectNameChanged (const QString &objectName) |
QObject * | parent () const const |
QVariant | property (const char *name) const const |
Q_CLASSINFO (Name, Value) | |
Q_EMIT Q_EMIT | |
Q_ENUM (...) | |
Q_ENUM_NS (...) | |
Q_ENUMS (...) | |
Q_FLAG (...) | |
Q_FLAG_NS (...) | |
Q_FLAGS (...) | |
Q_GADGET Q_GADGET | |
Q_GADGET_EXPORT (EXPORT_MACRO) | |
Q_INTERFACES (...) | |
Q_INVOKABLE Q_INVOKABLE | |
Q_MOC_INCLUDE Q_MOC_INCLUDE | |
Q_NAMESPACE Q_NAMESPACE | |
Q_NAMESPACE_EXPORT (EXPORT_MACRO) | |
Q_OBJECT Q_OBJECT | |
Q_PROPERTY (...) | |
Q_REVISION Q_REVISION | |
Q_SET_OBJECT_NAME (Object) | |
Q_SIGNAL Q_SIGNAL | |
Q_SIGNALS Q_SIGNALS | |
Q_SLOT Q_SLOT | |
Q_SLOTS Q_SLOTS | |
T | qobject_cast (const QObject *object) |
T | qobject_cast (QObject *object) |
QT_NO_NARROWING_CONVERSIONS_IN_CONNECT QT_NO_NARROWING_CONVERSIONS_IN_CONNECT | |
void | removeEventFilter (QObject *obj) |
void | setObjectName (const QString &name) |
void | setObjectName (QAnyStringView name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | setProperty (const char *name, QVariant &&value) |
bool | signalsBlocked () const const |
int | startTimer (int interval, Qt::TimerType timerType) |
int | startTimer (std::chrono::milliseconds interval, Qt::TimerType timerType) |
QThread * | thread () const const |
Additional Inherited Members | |
![]() | |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *context, Functor functor, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, Functor functor) |
bool | disconnect (const QMetaObject::Connection &connection) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
bool | disconnect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
![]() | |
typedef | QObjectList |
![]() | |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const QMetaMethod &signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const QMetaMethod &signal) |
bool | isSignalConnected (const QMetaMethod &signal) const const |
int | receivers (const char *signal) const const |
QObject * | sender () const const |
int | senderSignalIndex () const const |
virtual void | timerEvent (QTimerEvent *event) |
Detailed Description
The data model (not based on QAbstractModel) for a MarbleWidget.
This class provides a data storage and indexer that can be displayed in a MarbleWidget. It contains 3 different datatypes: tiles which provide the background, vectors which provide things like country borders and coastlines and placemarks which can show points of interest, such as cities, mountain tops or the poles.
The tiles provide the background of the image and can be for instance height and depth fields, magnetic strength, topographic data or anything else that is area based.
The vectors provide things like country borders and coastlines. They are stored in separate files and can be added or removed at anytime.
The placemarks contain points of interest, such as cities, mountain tops or the poles. These are sorted by size (for cities) and category (capitals, other important cities, less important cities, etc) and are displayed with different color or shape like square or round.
- See also
- MarbleWidget
Definition at line 83 of file MarbleModel.h.
Property Documentation
◆ mapThemeId
|
readwrite |
Definition at line 89 of file MarbleModel.h.
◆ workOffline
|
readwrite |
Definition at line 90 of file MarbleModel.h.
Constructor & Destructor Documentation
◆ MarbleModel()
|
explicit |
Construct a new MarbleModel.
- Parameters
-
parent the parent widget
Definition at line 174 of file MarbleModel.cpp.
◆ ~MarbleModel()
|
override |
Definition at line 192 of file MarbleModel.cpp.
Member Function Documentation
◆ addGeoDataFile()
void Marble::MarbleModel::addGeoDataFile | ( | const QString & | filename | ) |
Handle file loading into the treeModel.
- Parameters
-
filename the file to load
Definition at line 698 of file MarbleModel.cpp.
◆ addGeoDataString()
void Marble::MarbleModel::addGeoDataString | ( | const QString & | data, |
const QString & | key = QLatin1String("data") ) |
Handle raw data loading into the treeModel.
- Parameters
-
data the raw data to load key the name to remove this raw data later
Definition at line 703 of file MarbleModel.cpp.
◆ bookmarkManager()
BookmarkManager * Marble::MarbleModel::bookmarkManager | ( | ) |
return instance of BookmarkManager
Definition at line 199 of file MarbleModel.cpp.
◆ clearPersistentTileCache
|
slot |
Definition at line 545 of file MarbleModel.cpp.
◆ clock() [1/2]
MarbleClock * Marble::MarbleModel::clock | ( | ) |
Definition at line 520 of file MarbleModel.cpp.
◆ clock() [2/2]
const MarbleClock * Marble::MarbleModel::clock | ( | ) | const |
Definition at line 525 of file MarbleModel.cpp.
◆ clockDateTime()
QDateTime Marble::MarbleModel::clockDateTime | ( | ) | const |
Definition at line 662 of file MarbleModel.cpp.
◆ clockSpeed()
int Marble::MarbleModel::clockSpeed | ( | ) | const |
Definition at line 667 of file MarbleModel.cpp.
◆ clockTimezone()
int Marble::MarbleModel::clockTimezone | ( | ) | const |
Definition at line 682 of file MarbleModel.cpp.
◆ creatingTilesStart
|
signal |
Signal that the MarbleModel has started to create a new set of tiles.
- Parameters
-
name name of the set description the set description
◆ downloadManager() [1/2]
HttpDownloadManager * Marble::MarbleModel::downloadManager | ( | ) |
Return the downloadmanager to load missing tiles.
- Returns
- the HttpDownloadManager instance.
Definition at line 450 of file MarbleModel.cpp.
◆ downloadManager() [2/2]
const HttpDownloadManager * Marble::MarbleModel::downloadManager | ( | ) | const |
Definition at line 455 of file MarbleModel.cpp.
◆ elevationModel() [1/2]
ElevationModel * Marble::MarbleModel::elevationModel | ( | ) |
Definition at line 842 of file MarbleModel.cpp.
◆ elevationModel() [2/2]
const ElevationModel * Marble::MarbleModel::elevationModel | ( | ) | const |
Definition at line 847 of file MarbleModel.cpp.
◆ fileManager()
FileManager * Marble::MarbleModel::fileManager | ( | ) |
Definition at line 500 of file MarbleModel.cpp.
◆ groundOverlayModel() [1/2]
QAbstractItemModel * Marble::MarbleModel::groundOverlayModel | ( | ) |
Definition at line 480 of file MarbleModel.cpp.
◆ groundOverlayModel() [2/2]
const QAbstractItemModel * Marble::MarbleModel::groundOverlayModel | ( | ) | const |
Definition at line 485 of file MarbleModel.cpp.
◆ home()
void Marble::MarbleModel::home | ( | qreal & | lon, |
qreal & | lat, | ||
int & | zoom ) const |
get the home point
- Parameters
-
lon the longitude of the home point. lat the latitude of the home point. zoom the default zoom level of the home point.
Definition at line 430 of file MarbleModel.cpp.
◆ homeChanged
|
signal |
◆ legend()
QTextDocument * Marble::MarbleModel::legend | ( | ) |
Definition at line 687 of file MarbleModel.cpp.
◆ mapTheme() [1/2]
GeoSceneDocument * Marble::MarbleModel::mapTheme | ( | ) |
Definition at line 214 of file MarbleModel.cpp.
◆ mapTheme() [2/2]
const GeoSceneDocument * Marble::MarbleModel::mapTheme | ( | ) | const |
Definition at line 219 of file MarbleModel.cpp.
◆ mapThemeId()
QString Marble::MarbleModel::mapThemeId | ( | ) | const |
Return the name of the current map theme.
- Returns
- the identifier of the current MapTheme. To ensure that a unique identifier is being used the theme does NOT get represented by its name but the by relative location of the file that specifies the theme:
Example: maptheme = "earth/bluemarble/bluemarble.dgml"
Definition at line 204 of file MarbleModel.cpp.
◆ persistentTileCacheLimit()
quint64 Marble::MarbleModel::persistentTileCacheLimit | ( | ) | const |
Returns the limit in kilobytes of the persistent (on hard disc) tile cache.
- Returns
- the limit of persistent tile cache in kilobytes.
Definition at line 540 of file MarbleModel.cpp.
◆ placemarkModel() [1/2]
QAbstractItemModel * Marble::MarbleModel::placemarkModel | ( | ) |
Definition at line 470 of file MarbleModel.cpp.
◆ placemarkModel() [2/2]
const QAbstractItemModel * Marble::MarbleModel::placemarkModel | ( | ) | const |
Definition at line 475 of file MarbleModel.cpp.
◆ placemarkSelectionModel()
QItemSelectionModel * Marble::MarbleModel::placemarkSelectionModel | ( | ) |
Definition at line 490 of file MarbleModel.cpp.
◆ planet()
const Planet * Marble::MarbleModel::planet | ( | ) | const |
Returns the planet object for the current map.
- Returns
- the planet object for the current map
Definition at line 616 of file MarbleModel.cpp.
◆ planetId()
QString Marble::MarbleModel::planetId | ( | ) | const |
Definition at line 515 of file MarbleModel.cpp.
◆ planetName()
QString Marble::MarbleModel::planetName | ( | ) | const |
Definition at line 510 of file MarbleModel.cpp.
◆ planetRadius()
qreal Marble::MarbleModel::planetRadius | ( | ) | const |
Definition at line 505 of file MarbleModel.cpp.
◆ pluginManager() [1/2]
PluginManager * Marble::MarbleModel::pluginManager | ( | ) |
Definition at line 611 of file MarbleModel.cpp.
◆ pluginManager() [2/2]
const PluginManager * Marble::MarbleModel::pluginManager | ( | ) | const |
Definition at line 606 of file MarbleModel.cpp.
◆ positionTracking()
PositionTracking * Marble::MarbleModel::positionTracking | ( | ) | const |
Definition at line 495 of file MarbleModel.cpp.
◆ removeGeoData()
void Marble::MarbleModel::removeGeoData | ( | const QString & | key | ) |
Remove the file or raw data from the treeModel.
- Parameters
-
key either the file name or the key for raw data
Definition at line 708 of file MarbleModel.cpp.
◆ routingManager() [1/2]
RoutingManager * Marble::MarbleModel::routingManager | ( | ) |
Definition at line 647 of file MarbleModel.cpp.
◆ routingManager() [2/2]
const RoutingManager * Marble::MarbleModel::routingManager | ( | ) | const |
Definition at line 652 of file MarbleModel.cpp.
◆ setClockDateTime()
void Marble::MarbleModel::setClockDateTime | ( | const QDateTime & | datetime | ) |
Definition at line 657 of file MarbleModel.cpp.
◆ setClockSpeed()
void Marble::MarbleModel::setClockSpeed | ( | int | speed | ) |
Definition at line 672 of file MarbleModel.cpp.
◆ setClockTimezone()
void Marble::MarbleModel::setClockTimezone | ( | int | timeInSec | ) |
Definition at line 677 of file MarbleModel.cpp.
◆ setHome() [1/2]
void Marble::MarbleModel::setHome | ( | const GeoDataCoordinates & | homePoint, |
int | zoom = 1050 ) |
Set the home point.
- Parameters
-
homePoint the new home point. zoom the default zoom level for the new home point.
Definition at line 443 of file MarbleModel.cpp.
◆ setHome() [2/2]
void Marble::MarbleModel::setHome | ( | qreal | lon, |
qreal | lat, | ||
int | zoom = 1050 ) |
Set the home point.
- Parameters
-
lon the longitude of the new home point. lat the latitude of the new home point. zoom the default zoom level for the new home point.
Definition at line 436 of file MarbleModel.cpp.
◆ setLegend()
void Marble::MarbleModel::setLegend | ( | QTextDocument * | document | ) |
Uses the given text document as the new content of the legend Any previous legend content is overwritten.
MarbleModel takes ownership of the passed document.
Definition at line 692 of file MarbleModel.cpp.
◆ setMapTheme()
void Marble::MarbleModel::setMapTheme | ( | GeoSceneDocument * | document | ) |
Definition at line 242 of file MarbleModel.cpp.
◆ setMapThemeId()
void Marble::MarbleModel::setMapThemeId | ( | const QString & | mapThemeId | ) |
Set a new map theme to use.
- Parameters
-
mapThemeId the identifier of the new map theme
This function sets the map theme, i.e. combination of tile set and color scheme to use. If the map theme is not previously used, some basic tiles are created and a progress dialog is shown.
The ID of the new maptheme. To ensure that a unique identifier is being used the theme does NOT get represented by its name but the by relative location of the file that specifies the theme:
Example: maptheme = "earth/bluemarble/bluemarble.dgml"
Definition at line 233 of file MarbleModel.cpp.
◆ setPersistentTileCacheLimit
|
slot |
Set the limit of the persistent (on hard disc) tile cache.
- Parameters
-
kiloBytes The limit in kilobytes, 0 means no limit.
Definition at line 582 of file MarbleModel.cpp.
◆ setTrackedPlacemark
|
slot |
Change the placemark tracked by this model.
Definition at line 595 of file MarbleModel.cpp.
◆ setWorkOffline()
void Marble::MarbleModel::setWorkOffline | ( | bool | workOffline | ) |
Definition at line 832 of file MarbleModel.cpp.
◆ sunLocator() [1/2]
SunLocator * Marble::MarbleModel::sunLocator | ( | ) |
Definition at line 530 of file MarbleModel.cpp.
◆ sunLocator() [2/2]
const SunLocator * Marble::MarbleModel::sunLocator | ( | ) | const |
Definition at line 535 of file MarbleModel.cpp.
◆ themeChanged
|
signal |
Signal that the map theme has changed, and to which theme.
- Parameters
-
mapTheme the identifier of the new map theme.
- See also
- mapTheme
- setMapTheme
◆ trackedPlacemark()
const GeoDataPlacemark * Marble::MarbleModel::trackedPlacemark | ( | ) | const |
Returns the placemark being tracked by this model or 0 if no placemark is currently tracked.
Definition at line 601 of file MarbleModel.cpp.
◆ trackedPlacemarkChanged
|
signal |
Emitted when the placemark tracked by this model has changed.
- See also
- setTrackedPlacemark(), trackedPlacemark()
◆ treeModel() [1/2]
GeoDataTreeModel * Marble::MarbleModel::treeModel | ( | ) |
Return the list of Placemarks as a QAbstractItemModel *.
- Returns
- a list of all Placemarks in the MarbleModel.
Definition at line 460 of file MarbleModel.cpp.
◆ treeModel() [2/2]
const GeoDataTreeModel * Marble::MarbleModel::treeModel | ( | ) | const |
Definition at line 465 of file MarbleModel.cpp.
◆ updateProperty
|
slot |
Definition at line 713 of file MarbleModel.cpp.
◆ volatileTileCacheLimit()
quint64 Marble::MarbleModel::volatileTileCacheLimit | ( | ) | const |
Returns the limit of the volatile (in RAM) tile cache.
- Returns
- the cache limit in kilobytes
◆ workOffline()
bool Marble::MarbleModel::workOffline | ( | ) | const |
Definition at line 827 of file MarbleModel.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2025 The KDE developers.
Generated on Fri May 2 2025 12:01:36 by doxygen 1.13.2 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.