Marble::MarbleWidget
#include <MarbleWidget.h>
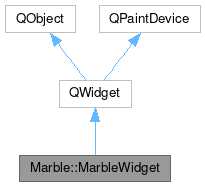
Public Member Functions | |
MarbleWidget (QWidget *parent=nullptr) | |
Access to helper objects | |
MarbleModel * | model () |
const MarbleModel * | model () const |
ViewportParams * | viewport () |
const ViewportParams * | viewport () const |
MarbleWidgetPopupMenu * | popupMenu () |
MarbleWidgetInputHandler * | inputHandler () const |
void | setInputHandler (MarbleWidgetInputHandler *handler) |
QList< RenderPlugin * > | renderPlugins () const |
QList< AbstractFloatItem * > | floatItems () const |
AbstractFloatItem * | floatItem (const QString &nameId) const |
void | readPluginSettings (QSettings &settings) |
void | writePluginSettings (QSettings &settings) const |
ViewContext | viewContext () const |
GeoSceneDocument * | mapTheme () const |
QList< AbstractDataPluginItem * > | whichItemAt (const QPoint &curpos) const |
void | addLayer (LayerInterface *layer) |
void | removeLayer (LayerInterface *layer) |
RoutingLayer * | routingLayer () |
PopupLayer * | popupLayer () |
const StyleBuilder * | styleBuilder () const |
Projection | projection () const |
Visible map area | |
QString | mapThemeId () const |
QRegion | mapRegion () const |
int | radius () const |
int | zoom () const |
int | tileZoomLevel () const |
qreal | distance () const |
QString | distanceString () const |
int | minimumZoom () const |
int | maximumZoom () const |
Position management | |
bool | screenCoordinates (qreal lon, qreal lat, qreal &x, qreal &y) const |
bool | geoCoordinates (int x, int y, qreal &lon, qreal &lat, GeoDataCoordinates::Unit=GeoDataCoordinates::Degree) const |
qreal | centerLongitude () const |
qreal | centerLatitude () const |
qreal | heading () const |
qreal | moveStep () const |
GeoDataLookAt | lookAt () const |
GeoDataCoordinates | focusPoint () const |
void | setFocusPoint (const GeoDataCoordinates &focusPoint) |
void | resetFocusPoint () |
qreal | radiusFromDistance (qreal distance) const |
qreal | distanceFromRadius (qreal radius) const |
qreal | zoomFromDistance (qreal distance) const |
qreal | distanceFromZoom (qreal zoom) const |
Placemark management | |
QList< const GeoDataFeature * > | whichFeatureAt (const QPoint &) const |
Float items and map appearance | |
bool | showOverviewMap () const |
bool | showScaleBar () const |
bool | showCompass () const |
bool | showClouds () const |
bool | showSunShading () const |
bool | showCityLights () const |
bool | isLockedToSubSolarPoint () const |
bool | isSubSolarPointIconVisible () const |
bool | showAtmosphere () const |
bool | showCrosshairs () const |
bool | showGrid () const |
bool | showPlaces () const |
bool | showCities () const |
bool | showTerrain () const |
bool | showOtherPlaces () const |
bool | showRelief () const |
bool | showIceLayer () const |
bool | showBorders () const |
bool | showRivers () const |
bool | showLakes () const |
bool | showFrameRate () const |
bool | showBackground () const |
MapQuality | mapQuality (ViewContext=Still) const |
bool | animationsEnabled () const |
AngleUnit | defaultAngleUnit () const |
void | setDefaultAngleUnit (AngleUnit angleUnit) |
QFont | defaultFont () const |
void | setDefaultFont (const QFont &font) |
Tile management | |
quint64 | volatileTileCacheLimit () const |
Miscellaneous | |
QPixmap | mapScreenShot () |
RenderStatus | renderStatus () const |
RenderState | renderState () const |
void | setHighlightEnabled (bool enabled) |
![]() | |
QWidget (QWidget *parent, Qt::WindowFlags f) | |
bool | acceptDrops () const const |
QString | accessibleDescription () const const |
QString | accessibleName () const const |
QList< QAction * > | actions () const const |
void | activateWindow () |
QAction * | addAction (const QIcon &icon, const QString &text) |
QAction * | addAction (const QIcon &icon, const QString &text, Args &&... args) |
QAction * | addAction (const QIcon &icon, const QString &text, const QKeySequence &shortcut) |
QAction * | addAction (const QIcon &icon, const QString &text, const QKeySequence &shortcut, Args &&... args) |
QAction * | addAction (const QIcon &icon, const QString &text, const QKeySequence &shortcut, const QObject *receiver, const char *member, Qt::ConnectionType type) |
QAction * | addAction (const QIcon &icon, const QString &text, const QObject *receiver, const char *member, Qt::ConnectionType type) |
QAction * | addAction (const QString &text) |
QAction * | addAction (const QString &text, Args &&... args) |
QAction * | addAction (const QString &text, const QKeySequence &shortcut) |
QAction * | addAction (const QString &text, const QKeySequence &shortcut, Args &&... args) |
QAction * | addAction (const QString &text, const QKeySequence &shortcut, const QObject *receiver, const char *member, Qt::ConnectionType type) |
QAction * | addAction (const QString &text, const QObject *receiver, const char *member, Qt::ConnectionType type) |
void | addAction (QAction *action) |
void | addActions (const QList< QAction * > &actions) |
void | adjustSize () |
bool | autoFillBackground () const const |
QPalette::ColorRole | backgroundRole () const const |
QBackingStore * | backingStore () const const |
QSize | baseSize () const const |
QWidget * | childAt (const QPoint &p) const const |
QWidget * | childAt (int x, int y) const const |
QRect | childrenRect () const const |
QRegion | childrenRegion () const const |
void | clearFocus () |
void | clearMask () |
bool | close () |
QMargins | contentsMargins () const const |
QRect | contentsRect () const const |
Qt::ContextMenuPolicy | contextMenuPolicy () const const |
QCursor | cursor () const const |
void | customContextMenuRequested (const QPoint &pos) |
WId | effectiveWinId () const const |
void | ensurePolished () const const |
Qt::FocusPolicy | focusPolicy () const const |
QWidget * | focusProxy () const const |
QWidget * | focusWidget () const const |
const QFont & | font () const const |
QFontInfo | fontInfo () const const |
QFontMetrics | fontMetrics () const const |
QPalette::ColorRole | foregroundRole () const const |
QRect | frameGeometry () const const |
QSize | frameSize () const const |
const QRect & | geometry () const const |
QPixmap | grab (const QRect &rectangle) |
void | grabGesture (Qt::GestureType gesture, Qt::GestureFlags flags) |
void | grabKeyboard () |
void | grabMouse () |
void | grabMouse (const QCursor &cursor) |
int | grabShortcut (const QKeySequence &key, Qt::ShortcutContext context) |
QGraphicsEffect * | graphicsEffect () const const |
QGraphicsProxyWidget * | graphicsProxyWidget () const const |
bool | hasEditFocus () const const |
bool | hasFocus () const const |
virtual bool | hasHeightForWidth () const const |
bool | hasMouseTracking () const const |
bool | hasTabletTracking () const const |
int | height () const const |
virtual int | heightForWidth (int w) const const |
void | hide () |
Qt::InputMethodHints | inputMethodHints () const const |
virtual QVariant | inputMethodQuery (Qt::InputMethodQuery query) const const |
void | insertAction (QAction *before, QAction *action) |
void | insertActions (QAction *before, const QList< QAction * > &actions) |
bool | isActiveWindow () const const |
bool | isAncestorOf (const QWidget *child) const const |
bool | isEnabled () const const |
bool | isEnabledTo (const QWidget *ancestor) const const |
bool | isFullScreen () const const |
bool | isHidden () const const |
bool | isMaximized () const const |
bool | isMinimized () const const |
bool | isModal () const const |
bool | isTopLevel () const const |
bool | isVisible () const const |
bool | isVisibleTo (const QWidget *ancestor) const const |
bool | isWindow () const const |
bool | isWindowModified () const const |
QLayout * | layout () const const |
Qt::LayoutDirection | layoutDirection () const const |
QLocale | locale () const const |
void | lower () |
QPoint | mapFrom (const QWidget *parent, const QPoint &pos) const const |
QPointF | mapFrom (const QWidget *parent, const QPointF &pos) const const |
QPoint | mapFromGlobal (const QPoint &pos) const const |
QPointF | mapFromGlobal (const QPointF &pos) const const |
QPoint | mapFromParent (const QPoint &pos) const const |
QPointF | mapFromParent (const QPointF &pos) const const |
QPoint | mapTo (const QWidget *parent, const QPoint &pos) const const |
QPointF | mapTo (const QWidget *parent, const QPointF &pos) const const |
QPoint | mapToGlobal (const QPoint &pos) const const |
QPointF | mapToGlobal (const QPointF &pos) const const |
QPoint | mapToParent (const QPoint &pos) const const |
QPointF | mapToParent (const QPointF &pos) const const |
QRegion | mask () const const |
int | maximumHeight () const const |
QSize | maximumSize () const const |
int | maximumWidth () const const |
int | minimumHeight () const const |
QSize | minimumSize () const const |
virtual QSize | minimumSizeHint () const const |
int | minimumWidth () const const |
void | move (const QPoint &) |
void | move (int x, int y) |
QWidget * | nativeParentWidget () const const |
QWidget * | nextInFocusChain () const const |
QRect | normalGeometry () const const |
void | overrideWindowFlags (Qt::WindowFlags flags) |
virtual QPaintEngine * | paintEngine () const const override |
const QPalette & | palette () const const |
QWidget * | parentWidget () const const |
QPoint | pos () const const |
QWidget * | previousInFocusChain () const const |
QWIDGETSIZE_MAX QWIDGETSIZE_MAX | |
void | raise () |
QRect | rect () const const |
void | releaseKeyboard () |
void | releaseMouse () |
void | releaseShortcut (int id) |
void | removeAction (QAction *action) |
void | render (QPaintDevice *target, const QPoint &targetOffset, const QRegion &sourceRegion, RenderFlags renderFlags) |
void | render (QPainter *painter, const QPoint &targetOffset, const QRegion &sourceRegion, RenderFlags renderFlags) |
void | repaint () |
void | repaint (const QRect &rect) |
void | repaint (const QRegion &rgn) |
void | repaint (int x, int y, int w, int h) |
void | resize (const QSize &) |
void | resize (int w, int h) |
bool | restoreGeometry (const QByteArray &geometry) |
QByteArray | saveGeometry () const const |
QScreen * | screen () const const |
void | scroll (int dx, int dy) |
void | scroll (int dx, int dy, const QRect &r) |
void | setAcceptDrops (bool on) |
void | setAccessibleDescription (const QString &description) |
void | setAccessibleName (const QString &name) |
void | setAttribute (Qt::WidgetAttribute attribute, bool on) |
void | setAutoFillBackground (bool enabled) |
void | setBackgroundRole (QPalette::ColorRole role) |
void | setBaseSize (const QSize &) |
void | setBaseSize (int basew, int baseh) |
void | setContentsMargins (const QMargins &margins) |
void | setContentsMargins (int left, int top, int right, int bottom) |
void | setContextMenuPolicy (Qt::ContextMenuPolicy policy) |
void | setCursor (const QCursor &) |
void | setDisabled (bool disable) |
void | setEditFocus (bool enable) |
void | setEnabled (bool) |
void | setFixedHeight (int h) |
void | setFixedSize (const QSize &s) |
void | setFixedSize (int w, int h) |
void | setFixedWidth (int w) |
void | setFocus () |
void | setFocus (Qt::FocusReason reason) |
void | setFocusPolicy (Qt::FocusPolicy policy) |
void | setFocusProxy (QWidget *w) |
void | setFont (const QFont &) |
void | setForegroundRole (QPalette::ColorRole role) |
void | setGeometry (const QRect &) |
void | setGeometry (int x, int y, int w, int h) |
void | setGraphicsEffect (QGraphicsEffect *effect) |
void | setHidden (bool hidden) |
void | setInputMethodHints (Qt::InputMethodHints hints) |
void | setLayout (QLayout *layout) |
void | setLayoutDirection (Qt::LayoutDirection direction) |
void | setLocale (const QLocale &locale) |
void | setMask (const QBitmap &bitmap) |
void | setMask (const QRegion ®ion) |
void | setMaximumHeight (int maxh) |
void | setMaximumSize (const QSize &) |
void | setMaximumSize (int maxw, int maxh) |
void | setMaximumWidth (int maxw) |
void | setMinimumHeight (int minh) |
void | setMinimumSize (const QSize &) |
void | setMinimumSize (int minw, int minh) |
void | setMinimumWidth (int minw) |
void | setMouseTracking (bool enable) |
void | setPalette (const QPalette &) |
void | setParent (QWidget *parent) |
void | setParent (QWidget *parent, Qt::WindowFlags f) |
void | setScreen (QScreen *screen) |
void | setShortcutAutoRepeat (int id, bool enable) |
void | setShortcutEnabled (int id, bool enable) |
void | setSizeIncrement (const QSize &) |
void | setSizeIncrement (int w, int h) |
void | setSizePolicy (QSizePolicy) |
void | setSizePolicy (QSizePolicy::Policy horizontal, QSizePolicy::Policy vertical) |
void | setStatusTip (const QString &) |
void | setStyle (QStyle *style) |
void | setStyleSheet (const QString &styleSheet) |
void | setTabletTracking (bool enable) |
void | setToolTip (const QString &) |
void | setToolTipDuration (int msec) |
void | setUpdatesEnabled (bool enable) |
void | setupUi (QWidget *widget) |
virtual void | setVisible (bool visible) |
void | setWhatsThis (const QString &) |
void | setWindowFilePath (const QString &filePath) |
void | setWindowFlag (Qt::WindowType flag, bool on) |
void | setWindowFlags (Qt::WindowFlags type) |
void | setWindowIcon (const QIcon &icon) |
void | setWindowIconText (const QString &) |
void | setWindowModality (Qt::WindowModality windowModality) |
void | setWindowModified (bool) |
void | setWindowOpacity (qreal level) |
void | setWindowRole (const QString &role) |
void | setWindowState (Qt::WindowStates windowState) |
void | setWindowTitle (const QString &) |
void | show () |
void | showFullScreen () |
void | showMaximized () |
void | showMinimized () |
void | showNormal () |
QSize | size () const const |
virtual QSize | sizeHint () const const |
QSize | sizeIncrement () const const |
QSizePolicy | sizePolicy () const const |
void | stackUnder (QWidget *w) |
QString | statusTip () const const |
QStyle * | style () const const |
QString | styleSheet () const const |
bool | testAttribute (Qt::WidgetAttribute attribute) const const |
QString | toolTip () const const |
int | toolTipDuration () const const |
QWidget * | topLevelWidget () const const |
bool | underMouse () const const |
void | ungrabGesture (Qt::GestureType gesture) |
void | unsetCursor () |
void | unsetLayoutDirection () |
void | unsetLocale () |
void | update () |
void | update (const QRect &rect) |
void | update (const QRegion &rgn) |
void | update (int x, int y, int w, int h) |
void | updateGeometry () |
bool | updatesEnabled () const const |
QRegion | visibleRegion () const const |
QString | whatsThis () const const |
int | width () const const |
QWidget * | window () const const |
QString | windowFilePath () const const |
Qt::WindowFlags | windowFlags () const const |
QWindow * | windowHandle () const const |
QIcon | windowIcon () const const |
void | windowIconChanged (const QIcon &icon) |
QString | windowIconText () const const |
void | windowIconTextChanged (const QString &iconText) |
Qt::WindowModality | windowModality () const const |
qreal | windowOpacity () const const |
QString | windowRole () const const |
Qt::WindowStates | windowState () const const |
QString | windowTitle () const const |
void | windowTitleChanged (const QString &title) |
Qt::WindowType | windowType () const const |
WId | winId () const const |
int | x () const const |
int | y () const const |
![]() | |
QObject (QObject *parent) | |
QBindable< QString > | bindableObjectName () |
bool | blockSignals (bool block) |
const QObjectList & | children () const const |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) const const |
bool | disconnect (const QObject *receiver, const char *method) const const |
void | dumpObjectInfo () const const |
void | dumpObjectTree () const const |
QList< QByteArray > | dynamicPropertyNames () const const |
virtual bool | eventFilter (QObject *watched, QEvent *event) |
T | findChild (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QRegularExpression &re, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (Qt::FindChildOptions options) const const |
bool | inherits (const char *className) const const |
void | installEventFilter (QObject *filterObj) |
bool | isQuickItemType () const const |
bool | isWidgetType () const const |
bool | isWindowType () const const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const const |
void | moveToThread (QThread *targetThread) |
QString | objectName () const const |
void | objectNameChanged (const QString &objectName) |
QObject * | parent () const const |
QVariant | property (const char *name) const const |
Q_CLASSINFO (Name, Value) | |
Q_EMIT Q_EMIT | |
Q_ENUM (...) | |
Q_ENUM_NS (...) | |
Q_ENUMS (...) | |
Q_FLAG (...) | |
Q_FLAG_NS (...) | |
Q_FLAGS (...) | |
Q_GADGET Q_GADGET | |
Q_GADGET_EXPORT (EXPORT_MACRO) | |
Q_INTERFACES (...) | |
Q_INVOKABLE Q_INVOKABLE | |
Q_MOC_INCLUDE Q_MOC_INCLUDE | |
Q_NAMESPACE Q_NAMESPACE | |
Q_NAMESPACE_EXPORT (EXPORT_MACRO) | |
Q_OBJECT Q_OBJECT | |
Q_PROPERTY (...) | |
Q_REVISION Q_REVISION | |
Q_SET_OBJECT_NAME (Object) | |
Q_SIGNAL Q_SIGNAL | |
Q_SIGNALS Q_SIGNALS | |
Q_SLOT Q_SLOT | |
Q_SLOTS Q_SLOTS | |
T | qobject_cast (const QObject *object) |
T | qobject_cast (QObject *object) |
QT_NO_NARROWING_CONVERSIONS_IN_CONNECT QT_NO_NARROWING_CONVERSIONS_IN_CONNECT | |
void | removeEventFilter (QObject *obj) |
void | setObjectName (const QString &name) |
void | setObjectName (QAnyStringView name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | setProperty (const char *name, QVariant &&value) |
bool | signalsBlocked () const const |
int | startTimer (int interval, Qt::TimerType timerType) |
int | startTimer (std::chrono::milliseconds interval, Qt::TimerType timerType) |
QThread * | thread () const const |
![]() | |
int | colorCount () const const |
int | depth () const const |
qreal | devicePixelRatio () const const |
qreal | devicePixelRatioF () const const |
int | height () const const |
int | heightMM () const const |
int | logicalDpiX () const const |
int | logicalDpiY () const const |
bool | paintingActive () const const |
int | physicalDpiX () const const |
int | physicalDpiY () const const |
int | width () const const |
int | widthMM () const const |
Miscellaneous slots | |
void | notifyMouseClick (int x, int y) |
void | setSelection (const QRect ®ion) |
void | setInputEnabled (bool) |
TextureLayer * | textureLayer () const |
VectorTileLayer * | vectorTileLayer () const |
void | zoomChanged (int zoom) |
void | distanceChanged (const QString &distanceString) |
void | tileLevelChanged (int level) |
void | viewContextChanged (ViewContext newViewContext) |
void | themeChanged (const QString &theme) |
void | projectionChanged (Projection) |
void | mouseMoveGeoPosition (const QString &) |
void | mouseClickGeoPosition (qreal lon, qreal lat, GeoDataCoordinates::Unit) |
void | framesPerSecond (qreal fps) |
void | regionSelected (const GeoDataLatLonBox &boundingBox) |
void | pluginSettingsChanged () |
void | renderPluginInitialized (RenderPlugin *renderPlugin) |
void | visibleLatLonAltBoxChanged (const GeoDataLatLonAltBox &visibleLatLonAltBox) |
void | renderStatusChanged (RenderStatus status) |
void | renderStateChanged (const RenderState &state) |
void | highlightedPlacemarksChanged (qreal lon, qreal lat, GeoDataCoordinates::Unit unit) |
void | propertyValueChanged (const QString &name, bool value) |
void | leaveEvent (QEvent *event) override |
void | paintEvent (QPaintEvent *event) override |
void | resizeEvent (QResizeEvent *event) override |
void | connectNotify (const QMetaMethod &signal) override |
void | disconnectNotify (const QMetaMethod &signal) override |
void | changeEvent (QEvent *event) override |
virtual void | customPaint (GeoPainter *painter) |
Additional Inherited Members | |
![]() | |
enum | RenderFlag |
![]() | |
enum | PaintDeviceMetric |
![]() | |
QWidget * | createWindowContainer (QWindow *window, QWidget *parent, Qt::WindowFlags flags) |
QWidget * | find (WId id) |
QWidget * | keyboardGrabber () |
QWidget * | mouseGrabber () |
void | setTabOrder (QWidget *first, QWidget *second) |
void | setTabOrder (std::initializer_list< QWidget * > widgets) |
![]() | |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *context, Functor functor, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, Functor functor) |
bool | disconnect (const QMetaObject::Connection &connection) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
bool | disconnect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
![]() | |
DrawChildren | |
DrawWindowBackground | |
IgnoreMask | |
typedef | RenderFlags |
![]() | |
typedef | QObjectList |
![]() | |
PdmDepth | |
PdmDevicePixelRatio | |
PdmDevicePixelRatioScaled | |
PdmDpiX | |
PdmDpiY | |
PdmHeight | |
PdmHeightMM | |
PdmNumColors | |
PdmPhysicalDpiX | |
PdmPhysicalDpiY | |
PdmWidth | |
PdmWidthMM | |
![]() | |
virtual void | actionEvent (QActionEvent *event) |
virtual void | closeEvent (QCloseEvent *event) |
virtual void | contextMenuEvent (QContextMenuEvent *event) |
void | create (WId window, bool initializeWindow, bool destroyOldWindow) |
void | destroy (bool destroyWindow, bool destroySubWindows) |
virtual void | dragEnterEvent (QDragEnterEvent *event) |
virtual void | dragLeaveEvent (QDragLeaveEvent *event) |
virtual void | dragMoveEvent (QDragMoveEvent *event) |
virtual void | dropEvent (QDropEvent *event) |
virtual void | enterEvent (QEnterEvent *event) |
virtual bool | event (QEvent *event) override |
virtual void | focusInEvent (QFocusEvent *event) |
bool | focusNextChild () |
virtual bool | focusNextPrevChild (bool next) |
virtual void | focusOutEvent (QFocusEvent *event) |
bool | focusPreviousChild () |
virtual void | hideEvent (QHideEvent *event) |
virtual void | initPainter (QPainter *painter) const const override |
virtual void | inputMethodEvent (QInputMethodEvent *event) |
virtual void | keyPressEvent (QKeyEvent *event) |
virtual void | keyReleaseEvent (QKeyEvent *event) |
virtual int | metric (PaintDeviceMetric m) const const override |
virtual void | mouseDoubleClickEvent (QMouseEvent *event) |
virtual void | mouseMoveEvent (QMouseEvent *event) |
virtual void | mousePressEvent (QMouseEvent *event) |
virtual void | mouseReleaseEvent (QMouseEvent *event) |
virtual void | moveEvent (QMoveEvent *event) |
virtual bool | nativeEvent (const QByteArray &eventType, void *message, qintptr *result) |
virtual void | showEvent (QShowEvent *event) |
virtual void | tabletEvent (QTabletEvent *event) |
void | updateMicroFocus (Qt::InputMethodQuery query) |
virtual void | wheelEvent (QWheelEvent *event) |
![]() | |
virtual void | childEvent (QChildEvent *event) |
virtual void | customEvent (QEvent *event) |
bool | isSignalConnected (const QMetaMethod &signal) const const |
int | receivers (const char *signal) const const |
QObject * | sender () const const |
int | senderSignalIndex () const const |
virtual void | timerEvent (QTimerEvent *event) |
Detailed Description
A widget class that displays a view of the earth.
This widget displays a view of the earth or any other globe, depending on which dataset is used. The user can navigate the globe using either a control widget, e.g. the MarbleNavigator, or the mouse. The mouse and keyboard control is done through a MarbleWidgetInputHandler. Only some aspects of the widget can be controlled by the mouse and/or keyboard.
By clicking on the globe and moving the mouse, the position can be changed. The user can also zoom by using the scroll wheel of the mouse in the widget. The zoom value is not tied to any units, but is an abstract value without any physical meaning. A value around 1000 shows the full globe in a normal-sized window. Higher zoom values give a more zoomed-in view.
The MarbleWidget owns a data model to work. This model is contained in the MarbleModel class, and it is painted by using a MarbleMap. The widget takes care of creating the map and model. A MarbleModel contains several datatypes, among them tiles which provide the background, vectors which provide things like country borders and coastlines and placemarks which can show points of interest, such as cities, mountain tops or the poles.
In addition to navigating with the mouse, you can also use it to get information about items on the map. You can either click on a placemark with the left mouse button or with the right mouse button anywhere on the map.
The left mouse button opens up a menu with all the placemarks within a certain distance from the mouse pointer. When you choose one item from the menu, Marble will open up a dialog window with some information about the placemark and also try to connect to Wikipedia to retrieve an article about it. If there is such an article, you will get a mini-browser window with the article in a tab.
- See also
- MarbleNavigator
- MarbleMap
- MarbleModel
Definition at line 97 of file MarbleWidget.h.
Property Documentation
◆ isLockedToSubSolarPoint
|
readwrite |
Definition at line 120 of file MarbleWidget.h.
◆ isSubSolarPointIconVisible
|
readwrite |
Definition at line 121 of file MarbleWidget.h.
◆ latitude
|
readwrite |
Definition at line 110 of file MarbleWidget.h.
◆ longitude
|
readwrite |
Definition at line 109 of file MarbleWidget.h.
◆ mapThemeId
|
readwrite |
Definition at line 106 of file MarbleWidget.h.
◆ projection
|
readwrite |
Definition at line 107 of file MarbleWidget.h.
◆ renderStatus
|
read |
Definition at line 139 of file MarbleWidget.h.
◆ showAtmosphere
|
readwrite |
Definition at line 122 of file MarbleWidget.h.
◆ showBorders
|
readwrite |
Definition at line 133 of file MarbleWidget.h.
◆ showCities
|
readwrite |
Definition at line 126 of file MarbleWidget.h.
◆ showCityLights
|
readwrite |
Definition at line 119 of file MarbleWidget.h.
◆ showClouds
|
readwrite |
Definition at line 117 of file MarbleWidget.h.
◆ showCompass
|
readwrite |
Definition at line 114 of file MarbleWidget.h.
◆ showCrosshairs
|
readwrite |
Definition at line 123 of file MarbleWidget.h.
◆ showGrid
|
readwrite |
Definition at line 115 of file MarbleWidget.h.
◆ showIceLayer
|
readwrite |
Definition at line 132 of file MarbleWidget.h.
◆ showLakes
|
readwrite |
Definition at line 135 of file MarbleWidget.h.
◆ showOtherPlaces
|
readwrite |
Definition at line 128 of file MarbleWidget.h.
◆ showOverviewMap
|
readwrite |
Definition at line 112 of file MarbleWidget.h.
◆ showPlaces
|
readwrite |
Definition at line 125 of file MarbleWidget.h.
◆ showRelief
|
readwrite |
Definition at line 130 of file MarbleWidget.h.
◆ showRivers
|
readwrite |
Definition at line 134 of file MarbleWidget.h.
◆ showScaleBar
|
readwrite |
Definition at line 113 of file MarbleWidget.h.
◆ showSunShading
|
readwrite |
Definition at line 118 of file MarbleWidget.h.
◆ showTerrain
|
readwrite |
Definition at line 127 of file MarbleWidget.h.
◆ viewContext
|
readwrite |
Definition at line 137 of file MarbleWidget.h.
◆ volatileTileCacheLimit
|
readwrite |
Definition at line 141 of file MarbleWidget.h.
◆ zoom
|
readwrite |
Definition at line 104 of file MarbleWidget.h.
Constructor & Destructor Documentation
◆ MarbleWidget()
|
explicit |
Construct a new MarbleWidget.
- Parameters
-
parent the parent widget
This constructor should be used when you will only use one MarbleWidget. The widget will create its own MarbleModel when created.
Definition at line 151 of file MarbleWidget.cpp.
◆ ~MarbleWidget()
|
override |
Definition at line 159 of file MarbleWidget.cpp.
Member Function Documentation
◆ addLayer()
void Marble::MarbleWidget::addLayer | ( | LayerInterface * | layer | ) |
Add a layer to be included in rendering.
Definition at line 356 of file MarbleWidget.cpp.
◆ animationsEnabled()
bool Marble::MarbleWidget::animationsEnabled | ( | ) | const |
Retrieve whether travels to a point should get animated.
Definition at line 1025 of file MarbleWidget.cpp.
◆ centerLatitude()
qreal Marble::MarbleWidget::centerLatitude | ( | ) | const |
Return the latitude of the center point.
- Returns
- The latitude of the center point in degree.
Definition at line 671 of file MarbleWidget.cpp.
◆ centerLongitude()
qreal Marble::MarbleWidget::centerLongitude | ( | ) | const |
Return the longitude of the center point.
- Returns
- The longitude of the center point in degree.
Definition at line 676 of file MarbleWidget.cpp.
◆ centerOn [1/4]
|
slot |
Center the view on a point This method centers the Marble map on the point described by the latitude and longitude in the GeoDataCoordinate parameter point
.
It also zooms the map to be at the elevation described by the altitude. If this is not the desired functionality or you do not have an accurate altitude then use
- See also
- centerOn(qreal, qreal, bool)
- Parameters
-
point the point in 3 dimensions above the globe to move the view to. It will always be looking vertically down. animated whether to use animation
Definition at line 564 of file MarbleWidget.cpp.
◆ centerOn [2/4]
|
slot |
Center the view on a bounding box so that it completely fills the viewport This method not only centers on the center of the GeoDataLatLon box but it also adjusts the zoom of the marble widget so that the LatLon box provided fills the viewport.
- Parameters
-
box The GeoDataLatLonBox to zoom and move the MarbleWidget to. animated whether to use animation.
Definition at line 570 of file MarbleWidget.cpp.
◆ centerOn [3/4]
|
slot |
Center the view on a placemark according to the following logic:
- if the placemark has a lookAt, zoom and center on that lookAt
- otherwise use the placemark geometry's latLonAltBox
- Parameters
-
placemark The GeoDataPlacemark to zoom and move the MarbleWidget to. animated Whether the centering is animated.
Definition at line 576 of file MarbleWidget.cpp.
◆ centerOn [4/4]
|
slot |
Center the view on a geographical point.
- Parameters
-
lat an angle in degrees parallel to the latitude lines +90(N) - -90(S) lon an angle in degrees parallel to the longitude lines +180(W) - -180(E) animated whether to use animation
Definition at line 558 of file MarbleWidget.cpp.
◆ changeEvent()
|
overrideprotectedvirtual |
Reimplementation of the changeEvent() function in QWidget to react to changes of the enabled state.
Reimplemented from QWidget.
Definition at line 1143 of file MarbleWidget.cpp.
◆ clearVolatileTileCache
|
slot |
Definition at line 977 of file MarbleWidget.cpp.
◆ connectNotify()
|
overrideprotectedvirtual |
Reimplemented from QObject.
Definition at line 647 of file MarbleWidget.cpp.
◆ creatingTilesStart
|
slot |
A slot that is called when the model starts to create new tiles.
- Parameters
-
creator the tile creator object. name the name of the created theme. description a descriptive text that can be shown in a dialog.
- See also
- creatingTilesProgress
This function is connected to the models signal with the same name. When the model needs to create a cache of tiles in several different resolutions, it will emit creatingTilesStart once with a name of the theme and a descriptive text. The widget can then pop up a dialog to explain why there is a delay. The model will then call creatingTilesProgress several times until the parameter reaches 100 (100%), after which the creation process is finished. After this there will be no more calls to creatingTilesProgress, and the poup dialog can then be closed.
Definition at line 990 of file MarbleWidget.cpp.
◆ customPaint()
|
protectedvirtual |
Enables custom drawing onto the MarbleWidget straight after.
the globe and before all other layers has been rendered.
- Parameters
-
painter
- Deprecated
- implement LayerInterface and add it using
addLayer()
Definition at line 733 of file MarbleWidget.cpp.
◆ debugLevelTags
|
slot |
Definition at line 955 of file MarbleWidget.cpp.
◆ defaultAngleUnit()
AngleUnit Marble::MarbleWidget::defaultAngleUnit | ( | ) | const |
Definition at line 1035 of file MarbleWidget.cpp.
◆ defaultFont()
QFont Marble::MarbleWidget::defaultFont | ( | ) | const |
Definition at line 1045 of file MarbleWidget.cpp.
◆ disconnectNotify()
|
overrideprotectedvirtual |
Reimplemented from QObject.
Definition at line 654 of file MarbleWidget.cpp.
◆ distance()
qreal Marble::MarbleWidget::distance | ( | ) | const |
Return the current distance.
Definition at line 1060 of file MarbleWidget.cpp.
◆ distanceFromRadius()
qreal Marble::MarbleWidget::distanceFromRadius | ( | qreal | radius | ) | const |
Return the distance (km) at the given globe radius (pixel)
Definition at line 1193 of file MarbleWidget.cpp.
◆ distanceFromZoom()
qreal Marble::MarbleWidget::distanceFromZoom | ( | qreal | zoom | ) | const |
Returns the distance (km) corresponding to the given zoom value.
Definition at line 1203 of file MarbleWidget.cpp.
◆ distanceString()
QString Marble::MarbleWidget::distanceString | ( | ) | const |
Return the current distance string.
Definition at line 1070 of file MarbleWidget.cpp.
◆ downloadRegion
|
slot |
Definition at line 1163 of file MarbleWidget.cpp.
◆ floatItem()
AbstractFloatItem * Marble::MarbleWidget::floatItem | ( | const QString & | nameId | ) | const |
Returns the FloatItem with the given id.
- Returns
- The pointer to the requested floatItem,
If no item is found the null pointer is returned.
Definition at line 1138 of file MarbleWidget.cpp.
◆ floatItems()
QList< AbstractFloatItem * > Marble::MarbleWidget::floatItems | ( | ) | const |
Returns a list of all FloatItems on the widget.
- Returns
- the list of the floatItems
Definition at line 1133 of file MarbleWidget.cpp.
◆ flyTo
Change the camera position to the given position.
- Parameters
-
lookAt New camera position. Changing the camera position means that both the current center position as well as the zoom value may change mode Interpolation type for intermediate camera positions. Automatic (default) chooses a suitable interpolation among Instant, Lenar and Jump. Instant will directly set the new zoom and position values, while Linear results in a linear interpolation of intermediate center coordinates along the sphere and a linear interpolation of changes in the camera distance to the ground. Finally, Jump will behave the same as Linear with regard to the center position interpolation, but use a parabolic height increase towards the middle point of the intermediate positions. This appears like a jump of the camera.
Definition at line 1152 of file MarbleWidget.cpp.
◆ focusPoint()
GeoDataCoordinates Marble::MarbleWidget::focusPoint | ( | ) | const |
- Returns
- The current point of focus, e.g. the point that is not moved when changing the zoom level. If not set, it defaults to the center point.
Definition at line 1173 of file MarbleWidget.cpp.
◆ geoCoordinates()
bool Marble::MarbleWidget::geoCoordinates | ( | int | x, |
int | y, | ||
qreal & | lon, | ||
qreal & | lat, | ||
GeoDataCoordinates::Unit | unit = GeoDataCoordinates::Degree ) const |
Get the earth coordinates corresponding to a pixel in the widget.
- Parameters
-
x the x coordinate of the pixel y the y coordinate of the pixel lon the longitude angle is returned through this parameter lat the latitude angle is returned through this parameter unit the angle unit
- Returns
true
if the pixel (x, y) is within the globefalse
if the pixel (x, y) is outside the globe, i.e. in space.
Definition at line 666 of file MarbleWidget.cpp.
◆ goHome
Center the view on the default start point with the default zoom.
Definition at line 739 of file MarbleWidget.cpp.
◆ heading()
qreal Marble::MarbleWidget::heading | ( | ) | const |
Definition at line 1228 of file MarbleWidget.cpp.
◆ inputHandler()
MarbleWidgetInputHandler * Marble::MarbleWidget::inputHandler | ( | ) | const |
Returns the current input handler.
Definition at line 306 of file MarbleWidget.cpp.
◆ isLockedToSubSolarPoint()
bool Marble::MarbleWidget::isLockedToSubSolarPoint | ( | ) | const |
Return whether the globe is locked to the sub solar point.
- Returns
- if globe is locked to sub solar point
Definition at line 437 of file MarbleWidget.cpp.
◆ isSubSolarPointIconVisible()
bool Marble::MarbleWidget::isSubSolarPointIconVisible | ( | ) | const |
Return whether the sun icon is shown in the sub solar point.
- Returns
- visibility of the sun icon in the sub solar point
Definition at line 442 of file MarbleWidget.cpp.
◆ leaveEvent()
|
overrideprotectedvirtual |
Reimplementation of the leaveEvent() function in QWidget.
Reimplemented from QWidget.
Definition at line 633 of file MarbleWidget.cpp.
◆ levelToDebug
|
slot |
Definition at line 1238 of file MarbleWidget.cpp.
◆ lookAt()
GeoDataLookAt Marble::MarbleWidget::lookAt | ( | ) | const |
Return the lookAt.
Definition at line 1168 of file MarbleWidget.cpp.
◆ mapQuality()
MapQuality Marble::MarbleWidget::mapQuality | ( | ViewContext | viewContext = Still | ) | const |
Retrieve the map quality depending on the view context.
Definition at line 998 of file MarbleWidget.cpp.
◆ mapRegion()
QRegion Marble::MarbleWidget::mapRegion | ( | ) | const |
Return the projected region which describes the (shape of the) projected surface.
Definition at line 681 of file MarbleWidget.cpp.
◆ mapScreenShot()
QPixmap Marble::MarbleWidget::mapScreenShot | ( | ) |
Return a QPixmap with the current contents of the widget.
Definition at line 376 of file MarbleWidget.cpp.
◆ mapTheme()
GeoSceneDocument * Marble::MarbleWidget::mapTheme | ( | ) | const |
Get the GeoSceneDocument object of the current map theme.
Definition at line 773 of file MarbleWidget.cpp.
◆ mapThemeId()
QString Marble::MarbleWidget::mapThemeId | ( | ) | const |
Get the ID of the current map theme To ensure that a unique identifier is being used the theme does NOT get represented by its name but the by relative location of the file that specifies the theme:
Example: mapThemeId = "earth/bluemarble/bluemarble.dgml"
Definition at line 745 of file MarbleWidget.cpp.
◆ maximumZoom()
int Marble::MarbleWidget::maximumZoom | ( | ) | const |
Return the minimum zoom value for the current map theme.
Definition at line 341 of file MarbleWidget.cpp.
◆ minimumZoom()
int Marble::MarbleWidget::minimumZoom | ( | ) | const |
Return the minimum zoom value for the current map theme.
Definition at line 336 of file MarbleWidget.cpp.
◆ model() [1/2]
MarbleModel * Marble::MarbleWidget::model | ( | ) |
Return the model that this view shows.
Definition at line 276 of file MarbleWidget.cpp.
◆ model() [2/2]
const MarbleModel * Marble::MarbleWidget::model | ( | ) | const |
Definition at line 281 of file MarbleWidget.cpp.
◆ moveDown
Move down by the moveStep.
Definition at line 627 of file MarbleWidget.cpp.
◆ moveLeft
Move left by the moveStep.
Definition at line 609 of file MarbleWidget.cpp.
◆ moveRight
Move right by the moveStep.
Definition at line 615 of file MarbleWidget.cpp.
◆ moveStep()
qreal Marble::MarbleWidget::moveStep | ( | ) | const |
Return how much the map will move if one of the move slots are called.
- Returns
- The move step.
Definition at line 321 of file MarbleWidget.cpp.
◆ moveUp
Move up by the moveStep.
Definition at line 621 of file MarbleWidget.cpp.
◆ notifyMouseClick
|
slot |
Used to notify about the position of the mouse click.
Definition at line 965 of file MarbleWidget.cpp.
◆ paintEvent()
|
overrideprotectedvirtual |
Reimplementation of the paintEvent() function in QWidget.
Reimplemented from QWidget.
Definition at line 686 of file MarbleWidget.cpp.
◆ pluginSettingsChanged
|
signal |
This signal is emit when the settings of a plugin changed.
◆ popupLayer()
PopupLayer * Marble::MarbleWidget::popupLayer | ( | ) |
Definition at line 1213 of file MarbleWidget.cpp.
◆ popupMenu()
MarbleWidgetPopupMenu * Marble::MarbleWidget::popupMenu | ( | ) |
Definition at line 296 of file MarbleWidget.cpp.
◆ projection()
Projection Marble::MarbleWidget::projection | ( | ) | const |
Get the Projection used for the map.
- Returns
Spherical
a Globe-
Equirectangular
a flat map -
Mercator
another flat map
Definition at line 594 of file MarbleWidget.cpp.
◆ radius()
int Marble::MarbleWidget::radius | ( | ) | const |
Return the radius of the globe in pixels.
Definition at line 311 of file MarbleWidget.cpp.
◆ radiusFromDistance()
qreal Marble::MarbleWidget::radiusFromDistance | ( | qreal | distance | ) | const |
Return the globe radius (pixel) for the given distance (km)
Definition at line 1188 of file MarbleWidget.cpp.
◆ readPluginSettings()
void Marble::MarbleWidget::readPluginSettings | ( | QSettings & | settings | ) |
Reads the plugin settings from the passed QSettings.
You shouldn't use this in a KDE application as these use KConfig. Here you could use MarblePart which is handling this automatically.
- Parameters
-
settings The QSettings object to be used.
Definition at line 1099 of file MarbleWidget.cpp.
◆ regionSelected
|
signal |
This signal is emit when a new rectangle region is selected over the map.
- Parameters
-
boundingBox The geographical coordinates of the selected region
◆ reloadMap
|
slot |
Re-download all visible tiles.
Definition at line 1158 of file MarbleWidget.cpp.
◆ removeLayer()
void Marble::MarbleWidget::removeLayer | ( | LayerInterface * | layer | ) |
Remove a layer from being included in rendering.
Definition at line 361 of file MarbleWidget.cpp.
◆ renderPluginInitialized
|
signal |
Signal that a render item has been initialized.
◆ renderPlugins()
QList< RenderPlugin * > Marble::MarbleWidget::renderPlugins | ( | ) | const |
Returns a list of all RenderPlugins on the widget, this includes float items.
- Returns
- the list of RenderPlugins
Definition at line 1094 of file MarbleWidget.cpp.
◆ renderState()
RenderState Marble::MarbleWidget::renderState | ( | ) | const |
Detailed render status of the current map view.
Definition at line 386 of file MarbleWidget.cpp.
◆ renderStatus()
RenderStatus Marble::MarbleWidget::renderStatus | ( | ) | const |
Summarized render status of the current map view.
- Todo
- Enable this instead of the zoomView slot below for proper deprecation warnings around Marble 1.8
- See also
- renderState
Definition at line 381 of file MarbleWidget.cpp.
◆ renderStatusChanged
|
signal |
Emitted when the layer rendering status has changed.
- Parameters
-
status New render status
◆ resetFocusPoint()
void Marble::MarbleWidget::resetFocusPoint | ( | ) |
Invalidate any focus point set with setFocusPoint.
- See also
- focusPoint setFocusPoint
Definition at line 1183 of file MarbleWidget.cpp.
◆ resizeEvent()
|
overrideprotectedvirtual |
Reimplementation of the resizeEvent() function in QWidget.
Reimplemented from QWidget.
Definition at line 638 of file MarbleWidget.cpp.
◆ rotateBy
|
slot |
Rotate the view by the two angles phi and theta.
- Parameters
-
deltaLon an angle that specifies the change in terms of longitude deltaLat an angle that specifies the change in terms of latitude mode the FlyToMode that will be used
This function rotates the view by two angles, deltaLon ("theta") and deltaLat ("phi"). If we start at (0, 0), the result will be the exact equivalent of (lon, lat), otherwise the resulting angle will be the sum of the previous position and the two offsets.
Definition at line 552 of file MarbleWidget.cpp.
◆ routingLayer()
RoutingLayer * Marble::MarbleWidget::routingLayer | ( | ) |
Definition at line 1208 of file MarbleWidget.cpp.
◆ screenCoordinates()
bool Marble::MarbleWidget::screenCoordinates | ( | qreal | lon, |
qreal | lat, | ||
qreal & | x, | ||
qreal & | y ) const |
Get the screen coordinates corresponding to geographical coordinates in the widget.
- Parameters
-
lon the lon coordinate of the requested pixel position lat the lat coordinate of the requested pixel position x the x coordinate of the pixel is returned through this parameter y the y coordinate of the pixel is returned through this parameter
- Returns
true
if the geographical coordinates are visible on the screenfalse
if the geographical coordinates are not visible on the screen
Definition at line 661 of file MarbleWidget.cpp.
◆ setAnimationsEnabled
|
slot |
Set whether travels to a point should get animated.
Definition at line 1030 of file MarbleWidget.cpp.
◆ setCenterLatitude
Set the latitude for the center point.
- Parameters
-
lat the new value for the latitude in degree. mode the FlyToMode that will be used.
Definition at line 582 of file MarbleWidget.cpp.
◆ setCenterLongitude
Set the longitude for the center point.
- Parameters
-
lon the new value for the longitude in degree. mode the FlyToMode that will be used.
Definition at line 588 of file MarbleWidget.cpp.
◆ setDebugLevelTags
|
slot |
Set whether to render according to OSM indoor level tags.
- Parameters
-
visible visibility of entities (placemarks, buildings etc.) level-wise
Definition at line 950 of file MarbleWidget.cpp.
◆ setDefaultAngleUnit()
void Marble::MarbleWidget::setDefaultAngleUnit | ( | AngleUnit | angleUnit | ) |
Definition at line 1040 of file MarbleWidget.cpp.
◆ setDefaultFont()
void Marble::MarbleWidget::setDefaultFont | ( | const QFont & | font | ) |
Definition at line 1050 of file MarbleWidget.cpp.
◆ setDistance
|
slot |
Set the distance of the observer to the globe in km.
- Parameters
-
distance The new distance in km.
Definition at line 1065 of file MarbleWidget.cpp.
◆ setFocusPoint()
void Marble::MarbleWidget::setFocusPoint | ( | const GeoDataCoordinates & | focusPoint | ) |
Change the point of focus, overridding any previously set focus point.
- Parameters
-
focusPoint New focus point
- See also
- focusPoint resetFocusPoint
Definition at line 1178 of file MarbleWidget.cpp.
◆ setHeading
|
slot |
Definition at line 1223 of file MarbleWidget.cpp.
◆ setHighlightEnabled()
void Marble::MarbleWidget::setHighlightEnabled | ( | bool | enabled | ) |
Toggle whether regions are highlighted when user selects them.
Definition at line 391 of file MarbleWidget.cpp.
◆ setInputEnabled
|
slot |
Definition at line 1075 of file MarbleWidget.cpp.
◆ setInputHandler()
void Marble::MarbleWidget::setInputHandler | ( | MarbleWidgetInputHandler * | handler | ) |
Set the input handler.
Definition at line 301 of file MarbleWidget.cpp.
◆ setLevelToDebug
|
slot |
Set the level to debug.
- Parameters
-
level the level to debug
Definition at line 1233 of file MarbleWidget.cpp.
◆ setLockToSubSolarPoint
|
slot |
Set the globe locked to the sub solar point.
- Parameters
-
visible if globe is locked to the sub solar point
Definition at line 814 of file MarbleWidget.cpp.
◆ setMapQualityForViewContext
|
slot |
Set the map quality for the specified view context.
- Parameters
-
quality map quality for the specified view context viewContext view context whose map quality should be set
Definition at line 1003 of file MarbleWidget.cpp.
◆ setMapThemeId
|
slot |
Set a new map theme.
- Parameters
-
maptheme The ID of the new maptheme. To ensure that a unique identifier is being used the theme does NOT get represented by its name but the by relative location of the file that specifies the theme:
Example: maptheme = "earth/bluemarble/bluemarble.dgml"
Definition at line 750 of file MarbleWidget.cpp.
◆ setProjection [1/2]
|
slot |
Set the Projection used for the map.
- Parameters
-
projection projection type (e.g. Spherical, Equirectangular, Mercator)
Definition at line 604 of file MarbleWidget.cpp.
◆ setProjection [2/2]
|
slot |
Definition at line 599 of file MarbleWidget.cpp.
◆ setPropertyValue
|
slot |
Sets the value of a map theme property.
- Parameters
-
name name of the property value value of the property (usually: visibility)
Later on we might add a setPropertyType and a QVariant if needed.
Definition at line 778 of file MarbleWidget.cpp.
◆ setRadius
|
slot |
Set the radius of the globe in pixels.
- Parameters
-
radius The new globe radius value in pixels.
Definition at line 316 of file MarbleWidget.cpp.
◆ setSelection
|
slot |
Definition at line 1055 of file MarbleWidget.cpp.
◆ setShowAtmosphere
|
slot |
Set whether the atmospheric glow is visible.
- Parameters
-
visible visibility of the atmospheric glow
Definition at line 838 of file MarbleWidget.cpp.
◆ setShowBackground
|
slot |
Definition at line 905 of file MarbleWidget.cpp.
◆ setShowBorders
|
slot |
Set whether the borders visible.
- Parameters
-
visible visibility of the borders
Definition at line 883 of file MarbleWidget.cpp.
◆ setShowCities
|
slot |
Set whether the city place mark overlay is visible.
- Parameters
-
visible visibility of the city place marks
Definition at line 858 of file MarbleWidget.cpp.
◆ setShowCityLights
|
slot |
Set whether city lights instead of night shadow are visible.
- Parameters
-
visible visibility of city lights
Definition at line 809 of file MarbleWidget.cpp.
◆ setShowClouds
|
slot |
Set whether the cloud cover is visible.
- Parameters
-
visible visibility of the cloud cover
Definition at line 799 of file MarbleWidget.cpp.
◆ setShowCompass
|
slot |
Set whether the compass overlay is visible.
- Parameters
-
visible visibility of the compass
Definition at line 794 of file MarbleWidget.cpp.
◆ setShowCrosshairs
|
slot |
Set whether the crosshairs are visible.
- Parameters
-
visible visibility of the crosshairs
Definition at line 843 of file MarbleWidget.cpp.
◆ setShowDebugBatchRender
|
slot |
Set whether to enter the debug mode for batch rendering.
- Parameters
-
visible visibility of the batch rendering
Definition at line 930 of file MarbleWidget.cpp.
◆ setShowDebugPlacemarks
|
slot |
Set whether to enter the debug mode for placemark drawing.
- Parameters
-
visible visibility of the node debug mode
Definition at line 940 of file MarbleWidget.cpp.
◆ setShowDebugPolygons
|
slot |
Set whether to enter the debug mode for polygon node drawing.
- Parameters
-
visible visibility of the node debug mode
Definition at line 920 of file MarbleWidget.cpp.
◆ setShowFrameRate
|
slot |
Set whether the frame rate gets shown.
- Parameters
-
visible visibility of the frame rate
Definition at line 898 of file MarbleWidget.cpp.
◆ setShowGrid
|
slot |
Set whether the coordinate grid overlay is visible.
- Parameters
-
visible visibility of the coordinate grid
Definition at line 848 of file MarbleWidget.cpp.
◆ setShowIceLayer
|
slot |
Set whether the ice layer is visible.
- Parameters
-
visible visibility of the ice layer
Definition at line 878 of file MarbleWidget.cpp.
◆ setShowLakes
|
slot |
Set whether the lakes are visible.
- Parameters
-
visible visibility of the lakes
Definition at line 893 of file MarbleWidget.cpp.
◆ setShowOtherPlaces
|
slot |
Set whether the other places overlay is visible.
- Parameters
-
visible visibility of other places
Definition at line 868 of file MarbleWidget.cpp.
◆ setShowOverviewMap
|
slot |
Set whether the overview map overlay is visible.
- Parameters
-
visible visibility of the overview map
Definition at line 784 of file MarbleWidget.cpp.
◆ setShowPlaces
|
slot |
Set whether the place mark overlay is visible.
- Parameters
-
visible visibility of the place marks
Definition at line 853 of file MarbleWidget.cpp.
◆ setShowRelief
|
slot |
Set whether the relief is visible.
- Parameters
-
visible visibility of the relief
Definition at line 873 of file MarbleWidget.cpp.
◆ setShowRivers
|
slot |
Set whether the rivers are visible.
- Parameters
-
visible visibility of the rivers
Definition at line 888 of file MarbleWidget.cpp.
◆ setShowRuntimeTrace
|
slot |
Set whether the runtime tracing for layers gets shown.
- Parameters
-
visible visibility of the runtime tracing
Definition at line 910 of file MarbleWidget.cpp.
◆ setShowScaleBar
|
slot |
Set whether the scale bar overlay is visible.
- Parameters
-
visible visibility of the scale bar
Definition at line 789 of file MarbleWidget.cpp.
◆ setShowSunShading
|
slot |
Set whether the night shadow is visible.
- Parameters
-
visible visibility of shadow
Definition at line 804 of file MarbleWidget.cpp.
◆ setShowTerrain
|
slot |
Set whether the terrain place mark overlay is visible.
- Parameters
-
visible visibility of the terrain place marks
Definition at line 863 of file MarbleWidget.cpp.
◆ setShowTileId
|
slot |
Set whether the is tile is visible NOTE: This is part of the transitional debug API and might be subject to changes until Marble 0.8.
- Parameters
-
visible visibility of the tile
Definition at line 960 of file MarbleWidget.cpp.
◆ setSubSolarPointIconVisible
|
slot |
Set whether the sun icon is shown in the sub solar point.
- Parameters
-
visible if the sun icon is shown in the sub solar point
Definition at line 822 of file MarbleWidget.cpp.
◆ setViewContext
|
slot |
◆ setVolatileTileCacheLimit
|
slot |
Set the limit of the volatile (in RAM) tile cache.
- Parameters
-
kiloBytes The limit in kilobytes.
Definition at line 983 of file MarbleWidget.cpp.
◆ setZoom
Zoom the view to a certain zoomlevel.
- Parameters
-
zoom the new zoom level. mode the FlyToMode that will be used.
The zoom level is an abstract value without physical interpretation. A zoom value around 1000 lets the viewer see all of the earth in the default window.
Definition at line 522 of file MarbleWidget.cpp.
◆ showAtmosphere()
bool Marble::MarbleWidget::showAtmosphere | ( | ) | const |
Return whether the atmospheric glow is visible.
- Returns
- The cloud cover visibility.
Definition at line 447 of file MarbleWidget.cpp.
◆ showBackground()
bool Marble::MarbleWidget::showBackground | ( | ) | const |
Definition at line 512 of file MarbleWidget.cpp.
◆ showBorders()
bool Marble::MarbleWidget::showBorders | ( | ) | const |
Return whether the borders are visible.
- Returns
- The border visibility.
Definition at line 492 of file MarbleWidget.cpp.
◆ showCities()
bool Marble::MarbleWidget::showCities | ( | ) | const |
Return whether the city place marks are visible.
- Returns
- The city place mark visibility.
Definition at line 467 of file MarbleWidget.cpp.
◆ showCityLights()
bool Marble::MarbleWidget::showCityLights | ( | ) | const |
Return whether the city lights are shown instead of the night shadow.
- Returns
- visibility of city lights
Definition at line 432 of file MarbleWidget.cpp.
◆ showClouds()
bool Marble::MarbleWidget::showClouds | ( | ) | const |
Return whether the cloud cover is visible.
- Returns
- The cloud cover visibility.
Definition at line 422 of file MarbleWidget.cpp.
◆ showCompass()
bool Marble::MarbleWidget::showCompass | ( | ) | const |
Return whether the compass bar is visible.
- Returns
- The compass visibility.
Definition at line 417 of file MarbleWidget.cpp.
◆ showCrosshairs()
bool Marble::MarbleWidget::showCrosshairs | ( | ) | const |
Return whether the crosshairs are visible.
- Returns
- The crosshairs' visibility.
Definition at line 452 of file MarbleWidget.cpp.
◆ showDebugBatchRender
|
slot |
Definition at line 935 of file MarbleWidget.cpp.
◆ showDebugPlacemarks
|
slot |
Definition at line 945 of file MarbleWidget.cpp.
◆ showDebugPolygons
|
slot |
Definition at line 925 of file MarbleWidget.cpp.
◆ showFrameRate()
bool Marble::MarbleWidget::showFrameRate | ( | ) | const |
Return whether the frame rate gets displayed.
- Returns
- the frame rates visibility
Definition at line 507 of file MarbleWidget.cpp.
◆ showGrid()
bool Marble::MarbleWidget::showGrid | ( | ) | const |
Return whether the coordinate grid is visible.
- Returns
- The coordinate grid visibility.
Definition at line 457 of file MarbleWidget.cpp.
◆ showIceLayer()
bool Marble::MarbleWidget::showIceLayer | ( | ) | const |
Return whether the ice layer is visible.
- Returns
- The ice layer visibility.
Definition at line 487 of file MarbleWidget.cpp.
◆ showLakes()
bool Marble::MarbleWidget::showLakes | ( | ) | const |
Return whether the lakes are visible.
- Returns
- The lakes' visibility.
Definition at line 502 of file MarbleWidget.cpp.
◆ showOtherPlaces()
bool Marble::MarbleWidget::showOtherPlaces | ( | ) | const |
Return whether other places are visible.
- Returns
- The visibility of other places.
Definition at line 477 of file MarbleWidget.cpp.
◆ showOverviewMap()
bool Marble::MarbleWidget::showOverviewMap | ( | ) | const |
Return whether the overview map is visible.
- Returns
- The overview map visibility.
Definition at line 407 of file MarbleWidget.cpp.
◆ showPlaces()
bool Marble::MarbleWidget::showPlaces | ( | ) | const |
Return whether the place marks are visible.
- Returns
- The place mark visibility.
Definition at line 462 of file MarbleWidget.cpp.
◆ showRelief()
bool Marble::MarbleWidget::showRelief | ( | ) | const |
Return whether the relief is visible.
- Returns
- The relief visibility.
Definition at line 482 of file MarbleWidget.cpp.
◆ showRivers()
bool Marble::MarbleWidget::showRivers | ( | ) | const |
Return whether the rivers are visible.
- Returns
- The rivers' visibility.
Definition at line 497 of file MarbleWidget.cpp.
◆ showRuntimeTrace
|
slot |
Definition at line 915 of file MarbleWidget.cpp.
◆ showScaleBar()
bool Marble::MarbleWidget::showScaleBar | ( | ) | const |
Return whether the scale bar is visible.
- Returns
- The scale bar visibility.
Definition at line 412 of file MarbleWidget.cpp.
◆ showSunShading()
bool Marble::MarbleWidget::showSunShading | ( | ) | const |
Return whether the night shadow is visible.
- Returns
- visibility of night shadow
Definition at line 427 of file MarbleWidget.cpp.
◆ showTerrain()
bool Marble::MarbleWidget::showTerrain | ( | ) | const |
Return whether the terrain place marks are visible.
- Returns
- The terrain place mark visibility.
Definition at line 472 of file MarbleWidget.cpp.
◆ styleBuilder()
const StyleBuilder * Marble::MarbleWidget::styleBuilder | ( | ) | const |
- Since
- 0.26.0
Definition at line 1218 of file MarbleWidget.cpp.
◆ textureLayer
|
slot |
Definition at line 366 of file MarbleWidget.cpp.
◆ themeChanged
|
signal |
Signal that the theme has changed.
- Parameters
-
theme Name of the new theme.
◆ tileZoomLevel()
int Marble::MarbleWidget::tileZoomLevel | ( | ) | const |
Definition at line 331 of file MarbleWidget.cpp.
◆ vectorTileLayer
|
slot |
Definition at line 371 of file MarbleWidget.cpp.
◆ viewContext()
ViewContext Marble::MarbleWidget::viewContext | ( | ) | const |
Retrieve the view context (i.e.
still or animated map)
Definition at line 1008 of file MarbleWidget.cpp.
◆ viewport() [1/2]
ViewportParams * Marble::MarbleWidget::viewport | ( | ) |
Definition at line 286 of file MarbleWidget.cpp.
◆ viewport() [2/2]
const ViewportParams * Marble::MarbleWidget::viewport | ( | ) | const |
Definition at line 291 of file MarbleWidget.cpp.
◆ visibleLatLonAltBoxChanged
|
signal |
This signal is emitted when the visible region of the map changes.
This typically happens when the user moves the map around or zooms.
◆ volatileTileCacheLimit()
quint64 Marble::MarbleWidget::volatileTileCacheLimit | ( | ) | const |
Returns the limit in kilobytes of the volatile (in RAM) tile cache.
- Returns
- the limit of volatile tile cache
Definition at line 517 of file MarbleWidget.cpp.
◆ whichFeatureAt()
QList< const GeoDataFeature * > Marble::MarbleWidget::whichFeatureAt | ( | const QPoint & | curpos | ) | const |
Definition at line 346 of file MarbleWidget.cpp.
◆ whichItemAt()
Returns all widgets of dataPlugins on the position curpos.
Definition at line 351 of file MarbleWidget.cpp.
◆ writePluginSettings()
void Marble::MarbleWidget::writePluginSettings | ( | QSettings & | settings | ) | const |
Writes the plugin settings in the passed QSettings.
You shouldn't use this in a KDE application as these use KConfig. Here you could use MarblePart which is handling this automatically.
- Parameters
-
settings The QSettings object to be used.
Definition at line 1116 of file MarbleWidget.cpp.
◆ zoom()
int Marble::MarbleWidget::zoom | ( | ) | const |
Return the current zoom amount.
Definition at line 326 of file MarbleWidget.cpp.
◆ zoomChanged
|
signal |
◆ zoomFromDistance()
qreal Marble::MarbleWidget::zoomFromDistance | ( | qreal | distance | ) | const |
Returns the zoom value (no unit) corresponding to the given camera distance (km)
Definition at line 1198 of file MarbleWidget.cpp.
◆ zoomIn
Zoom in by the amount zoomStep.
Definition at line 540 of file MarbleWidget.cpp.
◆ zoomOut
Zoom out by the amount zoomStep.
Definition at line 546 of file MarbleWidget.cpp.
◆ zoomView
- Deprecated
- To be removed soon. Please use setZoom instead. Same parameters.
Definition at line 528 of file MarbleWidget.cpp.
◆ zoomViewBy
Zoom the view by a certain step.
- Parameters
-
zoomStep the difference between the old zoom and the new mode the FlyToMode that will be used.
Definition at line 534 of file MarbleWidget.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2025 The KDE developers.
Generated on Fri May 2 2025 12:01:36 by doxygen 1.13.2 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.