Marble::RenderPlugin
#include <RenderPlugin.h>
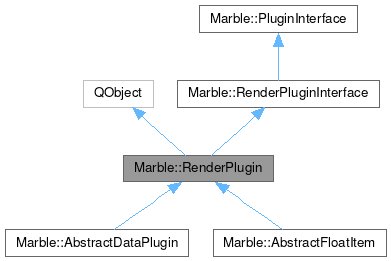
Public Types | |
enum | RenderType { UnknownRenderType , TopLevelRenderType , PanelRenderType , OnlineRenderType , ThemeRenderType } |
Properties | |
QString | description |
bool | enabled |
QString | name |
QString | nameId |
bool | userCheckable |
QString | version |
bool | visible |
![]() | |
objectName | |
Signals | |
void | actionGroupsChanged () |
void | enabledChanged (bool enable) |
void | repaintNeeded (const QRegion &dirtyRegion=QRegion()) |
void | settingsChanged (const QString &nameId) |
void | userCheckableChanged (bool isUserCheckable) |
void | visibilityChanged (bool visible, const QString &nameId) |
Public Slots | |
QAction * | action () const |
void | restoreDefaultSettings () |
void | setEnabled (bool enabled) |
bool | setSetting (const QString &key, const QVariant &value) |
QVariant | setting (const QString &key) const |
QStringList | settingKeys () const |
void | setUserCheckable (bool isUserCheckable) |
void | setVisible (bool visible) |
Public Member Functions | |
RenderPlugin (const MarbleModel *marbleModel) | |
virtual const QList< QActionGroup * > * | actionGroups () const |
bool | enabled () const |
virtual QString | guiString () const =0 |
bool | isUserCheckable () const |
const MarbleModel * | marbleModel () const |
virtual RenderPlugin * | newInstance (const MarbleModel *marbleModel) const =0 |
RenderState | renderState () const override |
virtual RenderType | renderType () const |
QString | runtimeTrace () const override |
virtual void | setSettings (const QHash< QString, QVariant > &settings) |
virtual QHash< QString, QVariant > | settings () const |
virtual const QList< QActionGroup * > * | toolbarActionGroups () const |
bool | visible () const |
![]() | |
QObject (QObject *parent) | |
QBindable< QString > | bindableObjectName () |
bool | blockSignals (bool block) |
const QObjectList & | children () const const |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) const const |
bool | disconnect (const QObject *receiver, const char *method) const const |
void | dumpObjectInfo () const const |
void | dumpObjectTree () const const |
QList< QByteArray > | dynamicPropertyNames () const const |
virtual bool | event (QEvent *e) |
T | findChild (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QRegularExpression &re, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (Qt::FindChildOptions options) const const |
bool | inherits (const char *className) const const |
void | installEventFilter (QObject *filterObj) |
bool | isQuickItemType () const const |
bool | isWidgetType () const const |
bool | isWindowType () const const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const const |
void | moveToThread (QThread *targetThread) |
QString | objectName () const const |
void | objectNameChanged (const QString &objectName) |
QObject * | parent () const const |
QVariant | property (const char *name) const const |
Q_CLASSINFO (Name, Value) | |
Q_EMIT Q_EMIT | |
Q_ENUM (...) | |
Q_ENUM_NS (...) | |
Q_ENUMS (...) | |
Q_FLAG (...) | |
Q_FLAG_NS (...) | |
Q_FLAGS (...) | |
Q_GADGET Q_GADGET | |
Q_GADGET_EXPORT (EXPORT_MACRO) | |
Q_INTERFACES (...) | |
Q_INVOKABLE Q_INVOKABLE | |
Q_MOC_INCLUDE Q_MOC_INCLUDE | |
Q_NAMESPACE Q_NAMESPACE | |
Q_NAMESPACE_EXPORT (EXPORT_MACRO) | |
Q_OBJECT Q_OBJECT | |
Q_PROPERTY (...) | |
Q_REVISION Q_REVISION | |
Q_SET_OBJECT_NAME (Object) | |
Q_SIGNAL Q_SIGNAL | |
Q_SIGNALS Q_SIGNALS | |
Q_SLOT Q_SLOT | |
Q_SLOTS Q_SLOTS | |
T | qobject_cast (const QObject *object) |
T | qobject_cast (QObject *object) |
QT_NO_NARROWING_CONVERSIONS_IN_CONNECT QT_NO_NARROWING_CONVERSIONS_IN_CONNECT | |
void | removeEventFilter (QObject *obj) |
void | setObjectName (const QString &name) |
void | setObjectName (QAnyStringView name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | setProperty (const char *name, QVariant &&value) |
bool | signalsBlocked () const const |
int | startTimer (int interval, Qt::TimerType timerType) |
int | startTimer (std::chrono::milliseconds interval, Qt::TimerType timerType) |
QThread * | thread () const const |
![]() | |
virtual QStringList | backendTypes () const =0 |
virtual void | initialize ()=0 |
virtual bool | isInitialized () const =0 |
virtual QString | renderPolicy () const =0 |
![]() | |
virtual QString | aboutDataText () const |
virtual QString | copyrightYears () const =0 |
virtual QString | description () const =0 |
virtual QIcon | icon () const =0 |
virtual QString | name () const =0 |
virtual QString | nameId () const =0 |
virtual QList< PluginAuthor > | pluginAuthors () const =0 |
virtual QString | version () const =0 |
Protected Member Functions | |
bool | eventFilter (QObject *, QEvent *) override |
![]() | |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const QMetaMethod &signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const QMetaMethod &signal) |
bool | isSignalConnected (const QMetaMethod &signal) const const |
int | receivers (const char *signal) const const |
QObject * | sender () const const |
int | senderSignalIndex () const const |
virtual void | timerEvent (QTimerEvent *event) |
Additional Inherited Members | |
![]() | |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *context, Functor functor, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, Functor functor) |
bool | disconnect (const QMetaObject::Connection &connection) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
bool | disconnect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
![]() | |
typedef | QObjectList |
Detailed Description
The abstract class that creates a renderable item.
Renderable Plugins can be used to extend Marble's functionality: They allow to draw stuff on top of the map / globe
Definition at line 37 of file RenderPlugin.h.
Member Enumeration Documentation
◆ RenderType
A Type of plugin.
Definition at line 53 of file RenderPlugin.h.
Property Documentation
◆ description
|
read |
Definition at line 44 of file RenderPlugin.h.
◆ enabled
|
readwrite |
Definition at line 45 of file RenderPlugin.h.
◆ name
|
read |
Definition at line 41 of file RenderPlugin.h.
◆ nameId
|
read |
Definition at line 42 of file RenderPlugin.h.
◆ userCheckable
|
readwrite |
Definition at line 47 of file RenderPlugin.h.
◆ version
|
read |
Definition at line 43 of file RenderPlugin.h.
◆ visible
|
readwrite |
Definition at line 46 of file RenderPlugin.h.
Constructor & Destructor Documentation
◆ RenderPlugin()
|
explicit |
Definition at line 51 of file RenderPlugin.cpp.
◆ ~RenderPlugin()
|
override |
Definition at line 63 of file RenderPlugin.cpp.
Member Function Documentation
◆ action
|
slot |
Plugin's menu action.
The action is checkable and controls the visibility of the plugin.
- Returns
- action, displayed in menu
Definition at line 73 of file RenderPlugin.cpp.
◆ actionGroups()
|
virtual |
Getting all actions.
This method is used by the main window to get all of the actions that this plugin defines. There is no guarantee where the main window will place the actions but it will generally be in a Menu. The returned QList should also contain all of the actions returned by
- See also
- toolbarActions().
- Returns
- a list of grouped actions
Definition at line 83 of file RenderPlugin.cpp.
◆ actionGroupsChanged
|
signal |
This signal is emitted if the actions that the plugin supports change in any way.
◆ enabled()
bool Marble::RenderPlugin::enabled | ( | ) | const |
is enabled
This method indicates enableability of the plugin
If plugin is enabled it going to be displayed in Marble Menu as active action which can be
- See also
- setUserCheckable
- Returns
- enableability of the plugin
- See also
- setEnabled
Definition at line 155 of file RenderPlugin.cpp.
◆ enabledChanged
|
signal |
This signal is emitted if the enabled property is changed with.
- See also
- setEnabled
◆ eventFilter()
Reimplemented from QObject.
Definition at line 201 of file RenderPlugin.cpp.
◆ guiString()
|
pure virtual |
String that should be displayed in GUI.
Using a "&" you can suggest key shortcuts
Example: "&Stars"
- Returns
- string for gui usage
◆ isUserCheckable()
bool Marble::RenderPlugin::isUserCheckable | ( | ) | const |
is user checkable
This method indicates user checkability of plugin's action displayed in application menu
Can control plugin visibility
- Warning
- User can do it only if
- See also
- enabled is true
- Returns
- checkability of the plugin
- See also
- setUserCheckable
Definition at line 165 of file RenderPlugin.cpp.
◆ marbleModel()
const MarbleModel * Marble::RenderPlugin::marbleModel | ( | ) | const |
Access to the MarbleModel.
Internal way to access the model of marble. Can be used to interact with the main application
- Returns
- marble model
- See also
- MarbleModel
Definition at line 68 of file RenderPlugin.cpp.
◆ newInstance()
|
pure virtual |
Creation a new instance of the plugin.
This method is used to create a new object of the current plugin using the marbleModel
given.
- Parameters
-
marbleModel base model
- Returns
- new instance of current plugin
- Note
- Typically this method is implemented with the help of the MARBLE_PLUGIN() macro.
◆ renderState()
|
override |
Definition at line 191 of file RenderPlugin.cpp.
◆ renderType()
|
virtual |
Render type of the plugin.
Function for returning the type of plugin this is for. This affects where in the menu tree the action() is placed.
- See also
- RenderType
- Returns
- : The type of render plugin this is
Reimplemented in Marble::AbstractDataPlugin, and Marble::AbstractFloatItem.
Definition at line 186 of file RenderPlugin.cpp.
◆ repaintNeeded
This signal is emitted if an update of the view is needed.
If available with the dirtyRegion
which is the region the view will change in. If dirtyRegion.isEmpty() returns true, the whole viewport has to be repainted.
◆ restoreDefaultSettings
|
slot |
Passes an empty set of settings to the plugin.
Well behaving plugins restore their settings to default values as a result of calling this method.
Definition at line 206 of file RenderPlugin.cpp.
◆ runtimeTrace()
|
override |
Definition at line 196 of file RenderPlugin.cpp.
◆ setEnabled
|
slot |
setting enabled
If enabled
= true, plugin will be enabled
If plugin is enabled it will be possible to show/hide it from menu (access from UI)
- Parameters
-
enabled plugin's enabled state
- See also
- enabled
Definition at line 124 of file RenderPlugin.cpp.
◆ setSetting
Change setting key's values.
- Parameters
-
key setting key value new value
This method applies value
for the key
- Returns
- successfully changed or not
Definition at line 216 of file RenderPlugin.cpp.
◆ setSettings()
Set the settings of the plugin.
Usually this is called at startup to restore saved settings.
- Parameters
-
new plugin's settings
- See also
- settings
Reimplemented in Marble::AbstractFloatItem.
Definition at line 180 of file RenderPlugin.cpp.
◆ setting
Getting setting value from the settings.
- Parameters
-
key setting's key index
This method should be used to get current value of key
in settings hash table
- Returns
- setting value
Definition at line 228 of file RenderPlugin.cpp.
◆ settingKeys
|
slot |
Full list of the settings keys.
This method should be used to get all possible settings' keys for the plugin's settings
- Returns
- list with the keys of settings
Definition at line 211 of file RenderPlugin.cpp.
◆ settings()
Settings of the plugin.
Settings is the map (hash table) of plugin's settings This method is called to determine the current settings of the plugin for serialization, e.g. when closing the application.
- Returns
- plugin's settings
- See also
- setSettings
Reimplemented in Marble::AbstractFloatItem.
Definition at line 170 of file RenderPlugin.cpp.
◆ settingsChanged
|
signal |
This signal is emitted if the settings of the RenderPlugin changed.
◆ setUserCheckable
|
slot |
setting user checkable
If isUserCheckable
= true, user will get an option to control visibility in application menu
- Parameters
-
isUserCheckable user checkability of the plugin
- See also
- isUserCheckable
Definition at line 146 of file RenderPlugin.cpp.
◆ setVisible
|
slot |
setting visible
If visible
= true, plugin will be visible
- Parameters
-
visible visibility of the plugin
- See also
- visible
Definition at line 136 of file RenderPlugin.cpp.
◆ toolbarActionGroups()
|
virtual |
Getting all actions which should be placed in the toolbar.
This method returns a subset of the actions returned by
- See also
- actions() which are intended to be placed in a more prominent place such as a toolbar above the Marble Widget. You are not guaranteed that they will be in an actual toolbar but they will be visible and discoverable
- Returns
- a list of grouped toolbar actions
Definition at line 88 of file RenderPlugin.cpp.
◆ userCheckableChanged
|
signal |
This signal is emitted if the user checkable property is changed with.
- See also
- setUserCheckable
◆ visibilityChanged
|
signal |
This signal is emitted if the visibility is changed with.
- See also
- setVisible
◆ visible()
bool Marble::RenderPlugin::visible | ( | ) | const |
is visible
This method indicates visibility of the plugin
If plugin is visible you can see it on the map/globe
- Returns
- visibility of the plugin
- See also
- setVisible
Definition at line 160 of file RenderPlugin.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2025 The KDE developers.
Generated on Fri May 2 2025 12:01:36 by doxygen 1.13.2 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.