Marble::MarbleMap
#include <MarbleMap.h>
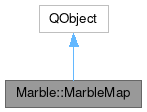
Signals | |
void | framesPerSecond (qreal fps) |
void | highlightedPlacemarksChanged (qreal, qreal, GeoDataCoordinates::Unit) |
void | mouseClickGeoPosition (qreal lon, qreal lat, GeoDataCoordinates::Unit) |
void | mouseMoveGeoPosition (const QString &geoPositionString) |
void | pluginSettingsChanged () |
void | projectionChanged (Projection) |
void | propertyValueChanged (const QString &name, bool value) |
void | radiusChanged (int radius) |
void | renderPluginInitialized (RenderPlugin *renderPlugin) |
void | renderStateChanged (const RenderState &state) |
void | renderStatusChanged (RenderStatus status) |
void | repaintNeeded (const QRegion &dirtyRegion=QRegion()) |
void | themeChanged (const QString &theme) |
void | tileLevelChanged (int level) |
void | viewContextChanged (ViewContext viewContext) |
void | visibleLatLonAltBoxChanged (const GeoDataLatLonAltBox &visibleLatLonAltBox) |
void | visibleRelationTypesChanged (GeoDataRelation::RelationTypes relationTypes) |
Public Slots | |
void | centerOn (const qreal lon, const qreal lat) |
void | clearVolatileTileCache () |
int | debugLevelTag () const |
void | downloadRegion (QList< TileCoordsPyramid > const &) |
void | highlightRouteRelation (qint64 osmId, bool enabled) |
bool | levelTagDebugModeEnabled () const |
void | notifyMouseClick (int x, int y) |
void | paint (GeoPainter &painter, const QRect &dirtyRect) |
void | reload () |
void | rotateBy (qreal deltaLon, qreal deltaLat) |
void | setCenterLatitude (qreal lat) |
void | setCenterLongitude (qreal lon) |
void | setDebugLevelTag (int level) |
void | setDefaultAngleUnit (AngleUnit angleUnit) |
void | setDefaultFont (const QFont &font) |
void | setHeading (qreal heading) |
void | setLevelTagDebugModeEnabled (bool visible) |
void | setLockToSubSolarPoint (bool visible) |
void | setMapThemeId (const QString &maptheme) |
void | setProjection (Projection projection) |
void | setPropertyValue (const QString &name, bool value) |
void | setRadius (int radius) |
void | setShowAtmosphere (bool visible) |
void | setShowBackground (bool visible) |
void | setShowBorders (bool visible) |
void | setShowCities (bool visible) |
void | setShowCityLights (bool visible) |
void | setShowClouds (bool visible) |
void | setShowCompass (bool visible) |
void | setShowCrosshairs (bool visible) |
void | setShowDebugBatchRender (bool visible) |
void | setShowDebugPlacemarks (bool visible) |
void | setShowDebugPolygons (bool visible) |
void | setShowFrameRate (bool visible) |
void | setShowGrid (bool visible) |
void | setShowIceLayer (bool visible) |
void | setShowLakes (bool visible) |
void | setShowOtherPlaces (bool visible) |
void | setShowOverviewMap (bool visible) |
void | setShowPlaces (bool visible) |
void | setShowRelief (bool visible) |
void | setShowRivers (bool visible) |
void | setShowRuntimeTrace (bool visible) |
void | setShowScaleBar (bool visible) |
void | setShowSunShading (bool visible) |
void | setShowTerrain (bool visible) |
void | setShowTileId (bool visible) |
void | setSubSolarPointIconVisible (bool visible) |
void | setVisibleRelationTypes (GeoDataRelation::RelationTypes relationTypes) |
void | setVolatileTileCacheLimit (quint64 kiloBytes) |
bool | showDebugBatchRender () const |
bool | showDebugPlacemarks () const |
bool | showDebugPolygons () const |
bool | showRuntimeTrace () const |
Public Member Functions | |
MarbleMap () | |
MarbleMap (MarbleModel *model) | |
void | addLayer (LayerInterface *layer) |
QString | addTextureLayer (GeoSceneTextureTileDataset *texture) |
qreal | centerLatitude () const |
qreal | centerLongitude () const |
QList< AbstractDataPlugin * > | dataPlugins () const |
AngleUnit | defaultAngleUnit () const |
QFont | defaultFont () const |
bool | discreteZoom () const |
AbstractFloatItem * | floatItem (const QString &nameId) const |
QList< AbstractFloatItem * > | floatItems () const |
bool | geoCoordinates (int x, int y, qreal &lon, qreal &lat, GeoDataCoordinates::Unit=GeoDataCoordinates::Degree) const |
bool | hasFeatureAt (const QPoint &) const |
qreal | heading () const |
int | height () const |
bool | isLockedToSubSolarPoint () const |
bool | isSubSolarPointIconVisible () const |
MapQuality | mapQuality () const |
MapQuality | mapQuality (ViewContext viewContext) const |
QString | mapThemeId () const |
int | maximumZoom () const |
int | minimumZoom () const |
MarbleModel * | model () const |
int | preferredRadiusCeil (int radius) const |
int | preferredRadiusFloor (int radius) const |
Projection | projection () const |
bool | propertyValue (const QString &name) const |
int | radius () const |
void | removeLayer (LayerInterface *layer) |
void | removeTextureLayer (const QString &key) |
QList< RenderPlugin * > | renderPlugins () const |
RenderState | renderState () const |
RenderStatus | renderStatus () const |
bool | screenCoordinates (qreal lon, qreal lat, qreal &x, qreal &y) const |
void | setMapQualityForViewContext (MapQuality qualityForViewContext, ViewContext viewContext) |
void | setSize (const QSize &size) |
void | setSize (int width, int height) |
void | setViewContext (ViewContext viewContext) |
bool | showAtmosphere () const |
bool | showBackground () const |
bool | showBorders () const |
bool | showCities () const |
bool | showCityLights () const |
bool | showClouds () const |
bool | showCompass () const |
bool | showCrosshairs () const |
bool | showFrameRate () const |
bool | showGrid () const |
bool | showIceLayer () const |
bool | showLakes () const |
bool | showOtherPlaces () const |
bool | showOverviewMap () const |
bool | showPlaces () const |
bool | showRelief () const |
bool | showRivers () const |
bool | showScaleBar () const |
bool | showSunShading () const |
bool | showTerrain () const |
QSize | size () const |
const StyleBuilder * | styleBuilder () const |
TextureLayer * | textureLayer () const |
int | tileZoomLevel () const |
VectorTileLayer * | vectorTileLayer () const |
ViewContext | viewContext () const |
ViewportParams * | viewport () |
const ViewportParams * | viewport () const |
GeoDataRelation::RelationTypes | visibleRelationTypes () const |
quint64 | volatileTileCacheLimit () const |
QList< const GeoDataFeature * > | whichFeatureAt (const QPoint &) const |
QList< AbstractDataPluginItem * > | whichItemAt (const QPoint &curpos) const |
int | width () const |
![]() | |
QObject (QObject *parent) | |
QBindable< QString > | bindableObjectName () |
bool | blockSignals (bool block) |
const QObjectList & | children () const const |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) const const |
bool | disconnect (const QObject *receiver, const char *method) const const |
void | dumpObjectInfo () const const |
void | dumpObjectTree () const const |
QList< QByteArray > | dynamicPropertyNames () const const |
virtual bool | event (QEvent *e) |
virtual bool | eventFilter (QObject *watched, QEvent *event) |
T | findChild (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QRegularExpression &re, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (Qt::FindChildOptions options) const const |
bool | inherits (const char *className) const const |
void | installEventFilter (QObject *filterObj) |
bool | isQuickItemType () const const |
bool | isWidgetType () const const |
bool | isWindowType () const const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const const |
void | moveToThread (QThread *targetThread) |
QString | objectName () const const |
void | objectNameChanged (const QString &objectName) |
QObject * | parent () const const |
QVariant | property (const char *name) const const |
Q_CLASSINFO (Name, Value) | |
Q_EMIT Q_EMIT | |
Q_ENUM (...) | |
Q_ENUM_NS (...) | |
Q_ENUMS (...) | |
Q_FLAG (...) | |
Q_FLAG_NS (...) | |
Q_FLAGS (...) | |
Q_GADGET Q_GADGET | |
Q_GADGET_EXPORT (EXPORT_MACRO) | |
Q_INTERFACES (...) | |
Q_INVOKABLE Q_INVOKABLE | |
Q_MOC_INCLUDE Q_MOC_INCLUDE | |
Q_NAMESPACE Q_NAMESPACE | |
Q_NAMESPACE_EXPORT (EXPORT_MACRO) | |
Q_OBJECT Q_OBJECT | |
Q_PROPERTY (...) | |
Q_REVISION Q_REVISION | |
Q_SET_OBJECT_NAME (Object) | |
Q_SIGNAL Q_SIGNAL | |
Q_SIGNALS Q_SIGNALS | |
Q_SLOT Q_SLOT | |
Q_SLOTS Q_SLOTS | |
T | qobject_cast (const QObject *object) |
T | qobject_cast (QObject *object) |
QT_NO_NARROWING_CONVERSIONS_IN_CONNECT QT_NO_NARROWING_CONVERSIONS_IN_CONNECT | |
void | removeEventFilter (QObject *obj) |
void | setObjectName (const QString &name) |
void | setObjectName (QAnyStringView name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | setProperty (const char *name, QVariant &&value) |
bool | signalsBlocked () const const |
int | startTimer (int interval, Qt::TimerType timerType) |
int | startTimer (std::chrono::milliseconds interval, Qt::TimerType timerType) |
QThread * | thread () const const |
Protected Member Functions | |
virtual void | customPaint (GeoPainter *painter) |
![]() | |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const QMetaMethod &signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const QMetaMethod &signal) |
bool | isSignalConnected (const QMetaMethod &signal) const const |
int | receivers (const char *signal) const const |
QObject * | sender () const const |
int | senderSignalIndex () const const |
virtual void | timerEvent (QTimerEvent *event) |
Additional Inherited Members | |
![]() | |
objectName | |
![]() | |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *context, Functor functor, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, Functor functor) |
bool | disconnect (const QMetaObject::Connection &connection) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
bool | disconnect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
![]() | |
typedef | QObjectList |
Detailed Description
A class that can paint a view of the earth.
FIXME: Change this description when we are done.
This class can paint a view of the earth or any other globe, depending on which dataset is used. It can be used to show the globe in a widget like MarbleWidget does, or on any other QPaintDevice.
The projection and other view parameters that control how MarbleMap paints the map is given through the class ViewParams. If the programmer wants to allow the user to control the map, he/she has to provide a way for the user to interact with it. An example of this can be seen in the class MarbleWidgetInputHandler, that lets the user control a MarbleWidget that uses MarbleMap internally.
The MarbleMap needs to be provided with a data model to work. This model is contained in the MarbleModel class. The widget can also construct its own model if none is given to the constructor. This data model contains 3 separate datatypes: tiles which provide the background, vectors which provide things like country borders and coastlines and placemarks which can show points of interest, such as cities, mountain tops or the poles.
- See also
- MarbleWidget
- MarbleModel
Definition at line 83 of file MarbleMap.h.
Constructor & Destructor Documentation
◆ MarbleMap() [1/2]
Marble::MarbleMap::MarbleMap | ( | ) |
Construct a new MarbleMap.
This constructor should be used when you will only use one MarbleMap. The widget will create its own MarbleModel when created.
Definition at line 304 of file MarbleMap.cpp.
◆ MarbleMap() [2/2]
|
explicit |
Construct a new MarbleMap.
- Parameters
-
model the data model for the widget.
This constructor should be used when you plan to use more than one MarbleMap for the same MarbleModel (not yet supported, but will be soon).
Definition at line 310 of file MarbleMap.cpp.
◆ ~MarbleMap()
|
override |
Definition at line 316 of file MarbleMap.cpp.
Member Function Documentation
◆ addLayer()
void Marble::MarbleMap::addLayer | ( | LayerInterface * | layer | ) |
Add a layer to be included in rendering.
Definition at line 1390 of file MarbleMap.cpp.
◆ addTextureLayer()
QString Marble::MarbleMap::addTextureLayer | ( | GeoSceneTextureTileDataset * | texture | ) |
Adds a texture sublayer.
- Returns
- Returns a key that identifies the texture sublayer
Definition at line 1410 of file MarbleMap.cpp.
◆ centerLatitude()
qreal Marble::MarbleMap::centerLatitude | ( | ) | const |
Return the latitude of the center point.
- Returns
- The latitude of the center point in degree.
Definition at line 449 of file MarbleMap.cpp.
◆ centerLongitude()
qreal Marble::MarbleMap::centerLongitude | ( | ) | const |
Return the longitude of the center point.
- Returns
- The longitude of the center point in degree.
Definition at line 462 of file MarbleMap.cpp.
◆ centerOn
|
slot |
Center the view on a geographical point.
- Parameters
-
lat an angle parallel to the latitude lines +90(N) - -90(S) lon an angle parallel to the longitude lines +180(W) - -180(E)
Definition at line 707 of file MarbleMap.cpp.
◆ clearVolatileTileCache
|
slot |
Definition at line 1310 of file MarbleMap.cpp.
◆ customPaint()
|
protectedvirtual |
Enables custom drawing onto the MarbleMap straight after.
the globe and before all other layers have been rendered.
- Parameters
-
painter
- Deprecated
- implement LayerInterface and add it using
addLayer()
Definition at line 850 of file MarbleMap.cpp.
◆ dataPlugins()
QList< AbstractDataPlugin * > Marble::MarbleMap::dataPlugins | ( | ) | const |
Returns a list of all DataPlugins on the layer.
- Returns
- the list of DataPlugins
Definition at line 1380 of file MarbleMap.cpp.
◆ debugLevelTag
|
slot |
Definition at line 1279 of file MarbleMap.cpp.
◆ defaultAngleUnit()
AngleUnit Marble::MarbleMap::defaultAngleUnit | ( | ) | const |
Definition at line 1323 of file MarbleMap.cpp.
◆ defaultFont()
QFont Marble::MarbleMap::defaultFont | ( | ) | const |
Definition at line 1347 of file MarbleMap.cpp.
◆ discreteZoom()
bool Marble::MarbleMap::discreteZoom | ( | ) | const |
Definition at line 486 of file MarbleMap.cpp.
◆ downloadRegion
|
slot |
Definition at line 505 of file MarbleMap.cpp.
◆ floatItem()
AbstractFloatItem * Marble::MarbleMap::floatItem | ( | const QString & | nameId | ) | const |
Returns a list of all FloatItems in the model.
- Returns
- the list of the floatItems
Definition at line 1368 of file MarbleMap.cpp.
◆ floatItems()
QList< AbstractFloatItem * > Marble::MarbleMap::floatItems | ( | ) | const |
Definition at line 1363 of file MarbleMap.cpp.
◆ geoCoordinates()
bool Marble::MarbleMap::geoCoordinates | ( | int | x, |
int | y, | ||
qreal & | lon, | ||
qreal & | lat, | ||
GeoDataCoordinates::Unit | unit = GeoDataCoordinates::Degree ) const |
Get the earth coordinates corresponding to a pixel in the map.
- Parameters
-
x the x coordinate of the pixel y the y coordinate of the pixel lon the longitude angle is returned through this parameter lat the latitude angle is returned through this parameter unit the angle units
- Returns
true
if the pixel (x, y) is within the globefalse
if the pixel (x, y) is outside the globe, i.e. in space.
Definition at line 748 of file MarbleMap.cpp.
◆ hasFeatureAt()
bool Marble::MarbleMap::hasFeatureAt | ( | const QPoint & | position | ) | const |
- Since
- 0.26.0
Definition at line 457 of file MarbleMap.cpp.
◆ heading()
qreal Marble::MarbleMap::heading | ( | ) | const |
Definition at line 1436 of file MarbleMap.cpp.
◆ height()
int Marble::MarbleMap::height | ( | ) | const |
Definition at line 411 of file MarbleMap.cpp.
◆ highlightRouteRelation
|
slot |
Definition at line 554 of file MarbleMap.cpp.
◆ isLockedToSubSolarPoint()
bool Marble::MarbleMap::isLockedToSubSolarPoint | ( | ) | const |
Return whether the globe is locked to the sub solar point.
- Returns
- if globe is locked to sub solar point
Definition at line 606 of file MarbleMap.cpp.
◆ isSubSolarPointIconVisible()
bool Marble::MarbleMap::isSubSolarPointIconVisible | ( | ) | const |
Return whether the sun icon is shown in the sub solar point.
- Returns
- visibility of the sun icon in the sub solar point
Definition at line 611 of file MarbleMap.cpp.
◆ levelTagDebugModeEnabled
|
slot |
Definition at line 1268 of file MarbleMap.cpp.
◆ mapQuality() [1/2]
MapQuality Marble::MarbleMap::mapQuality | ( | ) | const |
Return the current map quality.
Definition at line 361 of file MarbleMap.cpp.
◆ mapQuality() [2/2]
MapQuality Marble::MarbleMap::mapQuality | ( | ViewContext | viewContext | ) | const |
Definition at line 356 of file MarbleMap.cpp.
◆ mapThemeId()
QString Marble::MarbleMap::mapThemeId | ( | ) | const |
Get the ID of the current map theme To ensure that a unique identifier is being used the theme does NOT get represented by its name but the by relative location of the file that specifies the theme:
Example: maptheme = "earth/bluemarble/bluemarble.dgml"
Definition at line 855 of file MarbleMap.cpp.
◆ maximumZoom()
int Marble::MarbleMap::maximumZoom | ( | ) | const |
return the minimum zoom value for the current map theme.
Definition at line 478 of file MarbleMap.cpp.
◆ minimumZoom()
int Marble::MarbleMap::minimumZoom | ( | ) | const |
return the minimum zoom value for the current map theme.
Definition at line 470 of file MarbleMap.cpp.
◆ model()
MarbleModel * Marble::MarbleMap::model | ( | ) | const |
Return the model that this view shows.
Definition at line 333 of file MarbleMap.cpp.
◆ notifyMouseClick
|
slot |
used to notify about the position of the mouse click
Definition at line 1298 of file MarbleMap.cpp.
◆ paint
|
slot |
Paint the map using a give painter.
- Parameters
-
painter The painter to use. dirtyRect the rectangle that actually needs repainting.
Definition at line 808 of file MarbleMap.cpp.
◆ pluginSettingsChanged
|
signal |
This signal is emit when the settings of a plugin changed.
◆ preferredRadiusCeil()
int Marble::MarbleMap::preferredRadiusCeil | ( | int | radius | ) | const |
Definition at line 433 of file MarbleMap.cpp.
◆ preferredRadiusFloor()
int Marble::MarbleMap::preferredRadiusFloor | ( | int | radius | ) | const |
Definition at line 438 of file MarbleMap.cpp.
◆ projection()
Projection Marble::MarbleMap::projection | ( | ) | const |
Get the Projection used for the map.
- Returns
Spherical
a Globe-
Equirectangular
a flat map -
Mercator
another flat map
Definition at line 724 of file MarbleMap.cpp.
◆ propertyValue()
bool Marble::MarbleMap::propertyValue | ( | const QString & | name | ) | const |
Return the property value by name.
- Returns
- The property value (usually: visibility).
Definition at line 559 of file MarbleMap.cpp.
◆ radius()
int Marble::MarbleMap::radius | ( | ) | const |
Return the radius of the globe in pixels.
Definition at line 416 of file MarbleMap.cpp.
◆ reload
|
slot |
Reload the currently displayed map by reloading texture tiles from the Internet.
In the future this should be extended to all kinds of data which is used in the map.
Definition at line 499 of file MarbleMap.cpp.
◆ removeLayer()
void Marble::MarbleMap::removeLayer | ( | LayerInterface * | layer | ) |
Remove a layer from being included in rendering.
Definition at line 1395 of file MarbleMap.cpp.
◆ removeTextureLayer()
void Marble::MarbleMap::removeTextureLayer | ( | const QString & | key | ) |
Removes a texture sublayer.
- Parameters
-
key a key that was returned from corresponding addTextureLayer
Definition at line 1415 of file MarbleMap.cpp.
◆ renderPluginInitialized
|
signal |
Signal that a render item has been initialized.
◆ renderPlugins()
QList< RenderPlugin * > Marble::MarbleMap::renderPlugins | ( | ) | const |
Returns a list of all RenderPlugins in the model, this includes float items.
- Returns
- the list of RenderPlugins
Definition at line 1358 of file MarbleMap.cpp.
◆ renderState()
RenderState Marble::MarbleMap::renderState | ( | ) | const |
Definition at line 1405 of file MarbleMap.cpp.
◆ renderStatus()
RenderStatus Marble::MarbleMap::renderStatus | ( | ) | const |
Definition at line 1400 of file MarbleMap.cpp.
◆ renderStatusChanged
|
signal |
Emitted when the layer rendering status has changed.
- Parameters
-
status New render status
◆ repaintNeeded
This signal is emitted when the repaint of the view was requested.
If available with the dirtyRegion
which is the region the view will change in. If dirtyRegion.isEmpty() returns true, the whole viewport has to be repainted.
◆ rotateBy
|
slot |
Rotate the view by the two angles phi and theta.
- Parameters
-
deltaLon an angle that specifies the change in terms of longitude deltaLat an angle that specifies the change in terms of latitude
This function rotates the view by two angles, deltaLon ("theta") and deltaLat ("phi"). If we start at (0, 0), the result will be the exact equivalent of (lon, lat), otherwise the resulting angle will be the sum of the previous position and the two offsets.
Definition at line 702 of file MarbleMap.cpp.
◆ screenCoordinates()
bool Marble::MarbleMap::screenCoordinates | ( | qreal | lon, |
qreal | lat, | ||
qreal & | x, | ||
qreal & | y ) const |
Get the screen coordinates corresponding to geographical coordinates in the map.
- Parameters
-
lon the lon coordinate of the requested pixel position lat the lat coordinate of the requested pixel position x the x coordinate of the pixel is returned through this parameter y the y coordinate of the pixel is returned through this parameter
- Returns
true
if the geographical coordinates are visible on the screenfalse
if the geographical coordinates are not visible on the screen
Definition at line 743 of file MarbleMap.cpp.
◆ setCenterLatitude
|
slot |
Set the latitude for the center point.
- Parameters
-
lat the new value for the latitude in degree
Definition at line 714 of file MarbleMap.cpp.
◆ setCenterLongitude
|
slot |
Set the longitude for the center point.
- Parameters
-
lon the new value for the longitude in degree
Definition at line 719 of file MarbleMap.cpp.
◆ setDebugLevelTag
|
slot |
Definition at line 1273 of file MarbleMap.cpp.
◆ setDefaultAngleUnit
|
slot |
Definition at line 1334 of file MarbleMap.cpp.
◆ setDefaultFont
|
slot |
Definition at line 1352 of file MarbleMap.cpp.
◆ setHeading
|
slot |
Definition at line 1441 of file MarbleMap.cpp.
◆ setLevelTagDebugModeEnabled
|
slot |
Set whether to enter the debug mode for level tags.
- Parameters
-
visible visibility according to OSM level tags
Definition at line 1259 of file MarbleMap.cpp.
◆ setLockToSubSolarPoint
|
slot |
Set the globe locked to the sub solar point.
- Parameters
-
visible if globe is locked to the sub solar point
Definition at line 1122 of file MarbleMap.cpp.
◆ setMapQualityForViewContext()
void Marble::MarbleMap::setMapQualityForViewContext | ( | MapQuality | qualityForViewContext, |
ViewContext | viewContext ) |
Definition at line 348 of file MarbleMap.cpp.
◆ setMapThemeId
|
slot |
Set a new map theme.
- Parameters
-
maptheme The ID of the new maptheme. To ensure that a unique identifier is being used the theme does NOT get represented by its name but the by relative location of the file that specifies the theme:
Example: maptheme = "earth/bluemarble/bluemarble.dgml"
Definition at line 860 of file MarbleMap.cpp.
◆ setProjection
|
slot |
Set the Projection used for the map.
- Parameters
-
projection projection type (e.g. Spherical, Equirectangular, Mercator)
Definition at line 729 of file MarbleMap.cpp.
◆ setPropertyValue
|
slot |
Sets the value of a map theme property.
- Parameters
-
name name of the property value value of the property (usually: visibility)
Later on we might add a "setPropertyType and a QVariant if needed.
Definition at line 1048 of file MarbleMap.cpp.
◆ setRadius
|
slot |
Set the radius of the globe in pixels.
- Parameters
-
radius The new globe radius value in pixels.
Definition at line 421 of file MarbleMap.cpp.
◆ setShowAtmosphere
|
slot |
Set whether the atmospheric glow is visible.
- Parameters
-
visible visibility of the atmospheric glow
Definition at line 1080 of file MarbleMap.cpp.
◆ setShowBackground
|
slot |
Definition at line 1284 of file MarbleMap.cpp.
◆ setShowBorders
|
slot |
Set whether the borders visible.
- Parameters
-
visible visibility of the borders
Definition at line 1186 of file MarbleMap.cpp.
◆ setShowCities
|
slot |
Set whether the city place mark overlay is visible.
- Parameters
-
visible visibility of the city place marks
Definition at line 1161 of file MarbleMap.cpp.
◆ setShowCityLights
|
slot |
Set whether city lights instead of night shadow are visible.
- Parameters
-
visible visibility of city lights
Definition at line 1116 of file MarbleMap.cpp.
◆ setShowClouds
|
slot |
Set whether the cloud cover is visible.
- Parameters
-
visible visibility of the cloud cover
Definition at line 1104 of file MarbleMap.cpp.
◆ setShowCompass
|
slot |
Set whether the compass overlay is visible.
- Parameters
-
visible visibility of the compass
Definition at line 1075 of file MarbleMap.cpp.
◆ setShowCrosshairs
|
slot |
Set whether the crosshairs are visible.
- Parameters
-
visible visibility of the crosshairs
Definition at line 1092 of file MarbleMap.cpp.
◆ setShowDebugBatchRender
|
slot |
Set whether to enter the debug mode for visualizing batch rendering.
- Parameters
-
visible visibility of the batch rendering
Definition at line 1232 of file MarbleMap.cpp.
◆ setShowDebugPlacemarks
|
slot |
Set whether to enter the debug mode for placemark drawing.
- Parameters
-
visible visibility of the node debug mode
Definition at line 1246 of file MarbleMap.cpp.
◆ setShowDebugPolygons
|
slot |
Set whether to enter the debug mode for polygon node drawing.
- Parameters
-
visible visibility of the node debug mode
Definition at line 1219 of file MarbleMap.cpp.
◆ setShowFrameRate
|
slot |
Set whether the frame rate gets shown.
- Parameters
-
visible visibility of the frame rate
Definition at line 1201 of file MarbleMap.cpp.
◆ setShowGrid
|
slot |
Set whether the coordinate grid overlay is visible.
- Parameters
-
visible visibility of the coordinate grid
Definition at line 1151 of file MarbleMap.cpp.
◆ setShowIceLayer
|
slot |
Set whether the ice layer is visible.
- Parameters
-
visible visibility of the ice layer
Definition at line 1181 of file MarbleMap.cpp.
◆ setShowLakes
|
slot |
Set whether the lakes are visible.
- Parameters
-
visible visibility of the lakes
Definition at line 1196 of file MarbleMap.cpp.
◆ setShowOtherPlaces
|
slot |
Set whether the other places overlay is visible.
- Parameters
-
visible visibility of other places
Definition at line 1171 of file MarbleMap.cpp.
◆ setShowOverviewMap
|
slot |
Set whether the overview map overlay is visible.
- Parameters
-
visible visibility of the overview map
Definition at line 1065 of file MarbleMap.cpp.
◆ setShowPlaces
|
slot |
Set whether the place mark overlay is visible.
- Parameters
-
visible visibility of the place marks
Definition at line 1156 of file MarbleMap.cpp.
◆ setShowRelief
|
slot |
Set whether the relief is visible.
- Parameters
-
visible visibility of the relief
Definition at line 1176 of file MarbleMap.cpp.
◆ setShowRivers
|
slot |
Set whether the rivers are visible.
- Parameters
-
visible visibility of the rivers
Definition at line 1191 of file MarbleMap.cpp.
◆ setShowRuntimeTrace
|
slot |
Definition at line 1206 of file MarbleMap.cpp.
◆ setShowScaleBar
|
slot |
Set whether the scale bar overlay is visible.
- Parameters
-
visible visibility of the scale bar
Definition at line 1070 of file MarbleMap.cpp.
◆ setShowSunShading
|
slot |
Set whether the night shadow is visible.
- Parameters
-
visible visibility of shadow
Definition at line 1111 of file MarbleMap.cpp.
◆ setShowTerrain
|
slot |
Set whether the terrain place mark overlay is visible.
- Parameters
-
visible visibility of the terrain place marks
Definition at line 1166 of file MarbleMap.cpp.
◆ setShowTileId
|
slot |
Set whether the is tile is visible NOTE: This is part of the transitional debug API and might be subject to changes until Marble 0.8.
- Parameters
-
visible visibility of the tile
Definition at line 1146 of file MarbleMap.cpp.
◆ setSize() [1/2]
void Marble::MarbleMap::setSize | ( | const QSize & | size | ) |
Definition at line 394 of file MarbleMap.cpp.
◆ setSize() [2/2]
void Marble::MarbleMap::setSize | ( | int | width, |
int | height ) |
Definition at line 389 of file MarbleMap.cpp.
◆ setSubSolarPointIconVisible
|
slot |
Set whether the sun icon is shown in the sub solar point.
- Parameters
-
visible if the sun icon is shown in the sub solar point
Definition at line 1139 of file MarbleMap.cpp.
◆ setViewContext()
void Marble::MarbleMap::setViewContext | ( | ViewContext | viewContext | ) |
Definition at line 366 of file MarbleMap.cpp.
◆ setVisibleRelationTypes
|
slot |
Definition at line 1289 of file MarbleMap.cpp.
◆ setVolatileTileCacheLimit
|
slot |
Set the limit of the volatile (in RAM) tile cache.
- Parameters
-
kiloBytes The limit in kilobytes.
Definition at line 1317 of file MarbleMap.cpp.
◆ showAtmosphere()
bool Marble::MarbleMap::showAtmosphere | ( | ) | const |
Return whether the atmospheric glow is visible.
- Returns
- The cloud cover visibility.
Definition at line 616 of file MarbleMap.cpp.
◆ showBackground()
bool Marble::MarbleMap::showBackground | ( | ) | const |
Definition at line 687 of file MarbleMap.cpp.
◆ showBorders()
bool Marble::MarbleMap::showBorders | ( | ) | const |
Return whether the borders are visible.
- Returns
- The border visibility.
Definition at line 667 of file MarbleMap.cpp.
◆ showCities()
bool Marble::MarbleMap::showCities | ( | ) | const |
Return whether the city place marks are visible.
- Returns
- The city place mark visibility.
Definition at line 642 of file MarbleMap.cpp.
◆ showCityLights()
bool Marble::MarbleMap::showCityLights | ( | ) | const |
Return whether the city lights are shown instead of the night shadow.
- Returns
- visibility of city lights
Definition at line 601 of file MarbleMap.cpp.
◆ showClouds()
bool Marble::MarbleMap::showClouds | ( | ) | const |
Return whether the cloud cover is visible.
- Returns
- The cloud cover visibility.
Definition at line 591 of file MarbleMap.cpp.
◆ showCompass()
bool Marble::MarbleMap::showCompass | ( | ) | const |
Return whether the compass bar is visible.
- Returns
- The compass visibility.
Definition at line 581 of file MarbleMap.cpp.
◆ showCrosshairs()
bool Marble::MarbleMap::showCrosshairs | ( | ) | const |
Return whether the crosshairs are visible.
- Returns
- The crosshairs' visibility.
Definition at line 621 of file MarbleMap.cpp.
◆ showDebugBatchRender
|
slot |
Definition at line 1241 of file MarbleMap.cpp.
◆ showDebugPlacemarks
|
slot |
Definition at line 1254 of file MarbleMap.cpp.
◆ showDebugPolygons
|
slot |
Definition at line 1227 of file MarbleMap.cpp.
◆ showFrameRate()
bool Marble::MarbleMap::showFrameRate | ( | ) | const |
Return whether the frame rate gets displayed.
- Returns
- the frame rates visibility
Definition at line 682 of file MarbleMap.cpp.
◆ showGrid()
bool Marble::MarbleMap::showGrid | ( | ) | const |
Return whether the coordinate grid is visible.
- Returns
- The coordinate grid visibility.
Definition at line 586 of file MarbleMap.cpp.
◆ showIceLayer()
bool Marble::MarbleMap::showIceLayer | ( | ) | const |
Return whether the ice layer is visible.
- Returns
- The ice layer visibility.
Definition at line 662 of file MarbleMap.cpp.
◆ showLakes()
bool Marble::MarbleMap::showLakes | ( | ) | const |
Return whether the lakes are visible.
- Returns
- The lakes' visibility.
Definition at line 677 of file MarbleMap.cpp.
◆ showOtherPlaces()
bool Marble::MarbleMap::showOtherPlaces | ( | ) | const |
Return whether other places are visible.
- Returns
- The visibility of other places.
Definition at line 652 of file MarbleMap.cpp.
◆ showOverviewMap()
bool Marble::MarbleMap::showOverviewMap | ( | ) | const |
Return whether the overview map is visible.
- Returns
- The overview map visibility.
Definition at line 571 of file MarbleMap.cpp.
◆ showPlaces()
bool Marble::MarbleMap::showPlaces | ( | ) | const |
Return whether the place marks are visible.
- Returns
- The place mark visibility.
Definition at line 637 of file MarbleMap.cpp.
◆ showRelief()
bool Marble::MarbleMap::showRelief | ( | ) | const |
Return whether the relief is visible.
- Returns
- The relief visibility.
Definition at line 657 of file MarbleMap.cpp.
◆ showRivers()
bool Marble::MarbleMap::showRivers | ( | ) | const |
Return whether the rivers are visible.
- Returns
- The rivers' visibility.
Definition at line 672 of file MarbleMap.cpp.
◆ showRuntimeTrace
|
slot |
Definition at line 1214 of file MarbleMap.cpp.
◆ showScaleBar()
bool Marble::MarbleMap::showScaleBar | ( | ) | const |
Return whether the scale bar is visible.
- Returns
- The scale bar visibility.
Definition at line 576 of file MarbleMap.cpp.
◆ showSunShading()
bool Marble::MarbleMap::showSunShading | ( | ) | const |
Return whether the night shadow is visible.
- Returns
- visibility of night shadow
Definition at line 596 of file MarbleMap.cpp.
◆ showTerrain()
bool Marble::MarbleMap::showTerrain | ( | ) | const |
Return whether the terrain place marks are visible.
- Returns
- The terrain place mark visibility.
Definition at line 647 of file MarbleMap.cpp.
◆ size()
QSize Marble::MarbleMap::size | ( | ) | const |
Definition at line 401 of file MarbleMap.cpp.
◆ styleBuilder()
const StyleBuilder * Marble::MarbleMap::styleBuilder | ( | ) | const |
- Since
- 0.26.0
Definition at line 1431 of file MarbleMap.cpp.
◆ textureLayer()
TextureLayer * Marble::MarbleMap::textureLayer | ( | ) | const |
Definition at line 1421 of file MarbleMap.cpp.
◆ themeChanged
|
signal |
Signal that the theme has changed.
- Parameters
-
theme Name of the new theme.
◆ tileZoomLevel()
int Marble::MarbleMap::tileZoomLevel | ( | ) | const |
Definition at line 443 of file MarbleMap.cpp.
◆ vectorTileLayer()
VectorTileLayer * Marble::MarbleMap::vectorTileLayer | ( | ) | const |
Definition at line 1426 of file MarbleMap.cpp.
◆ viewContext()
ViewContext Marble::MarbleMap::viewContext | ( | ) | const |
Definition at line 384 of file MarbleMap.cpp.
◆ viewport() [1/2]
ViewportParams * Marble::MarbleMap::viewport | ( | ) |
Definition at line 338 of file MarbleMap.cpp.
◆ viewport() [2/2]
const ViewportParams * Marble::MarbleMap::viewport | ( | ) | const |
Definition at line 343 of file MarbleMap.cpp.
◆ visibleLatLonAltBoxChanged
|
signal |
This signal is emitted when the visible region of the map changes.
This typically happens when the user moves the map around or zooms.
◆ visibleRelationTypes()
GeoDataRelation::RelationTypes Marble::MarbleMap::visibleRelationTypes | ( | ) | const |
Definition at line 692 of file MarbleMap.cpp.
◆ volatileTileCacheLimit()
quint64 Marble::MarbleMap::volatileTileCacheLimit | ( | ) | const |
Returns the limit in kilobytes of the volatile (in RAM) tile cache.
- Returns
- the limit of volatile tile cache in kilobytes.
Definition at line 697 of file MarbleMap.cpp.
◆ whichFeatureAt()
QList< const GeoDataFeature * > Marble::MarbleMap::whichFeatureAt | ( | const QPoint & | curpos | ) | const |
Definition at line 494 of file MarbleMap.cpp.
◆ whichItemAt()
Returns all widgets of dataPlugins on the position curpos.
Definition at line 1385 of file MarbleMap.cpp.
◆ width()
int Marble::MarbleMap::width | ( | ) | const |
Definition at line 406 of file MarbleMap.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2025 The KDE developers.
Generated on Fri May 2 2025 12:01:36 by doxygen 1.13.2 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.