FMH::FileLoader
#include <fileloader.h>
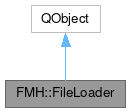
Signals | |
void | finished (FMH::MODEL_LIST items, QList< QUrl > urls) |
void | itemReady (FMH::MODEL item, QList< QUrl > urls) |
void | itemsReady (FMH::MODEL_LIST items, QList< QUrl > urls) |
Public Member Functions | |
FileLoader (QObject *parent=nullptr) | |
uint | batchCount () const |
void | requestPath (const QList< QUrl > &urls, const bool &recursive, const QStringList &nameFilters={}, const QDir::Filters &filters=QDir::Files, const uint &limit=99999) |
void | setBatchCount (const uint &count) |
![]() | |
QObject (QObject *parent) | |
QBindable< QString > | bindableObjectName () |
bool | blockSignals (bool block) |
const QObjectList & | children () const const |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) const const |
bool | disconnect (const QObject *receiver, const char *method) const const |
void | dumpObjectInfo () const const |
void | dumpObjectTree () const const |
QList< QByteArray > | dynamicPropertyNames () const const |
virtual bool | event (QEvent *e) |
virtual bool | eventFilter (QObject *watched, QEvent *event) |
T | findChild (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QRegularExpression &re, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (Qt::FindChildOptions options) const const |
bool | inherits (const char *className) const const |
void | installEventFilter (QObject *filterObj) |
bool | isQuickItemType () const const |
bool | isWidgetType () const const |
bool | isWindowType () const const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const const |
void | moveToThread (QThread *targetThread) |
QString | objectName () const const |
void | objectNameChanged (const QString &objectName) |
QObject * | parent () const const |
QVariant | property (const char *name) const const |
Q_CLASSINFO (Name, Value) | |
Q_EMIT Q_EMIT | |
Q_ENUM (...) | |
Q_ENUM_NS (...) | |
Q_ENUMS (...) | |
Q_FLAG (...) | |
Q_FLAG_NS (...) | |
Q_FLAGS (...) | |
Q_GADGET Q_GADGET | |
Q_GADGET_EXPORT (EXPORT_MACRO) | |
Q_INTERFACES (...) | |
Q_INVOKABLE Q_INVOKABLE | |
Q_MOC_INCLUDE Q_MOC_INCLUDE | |
Q_NAMESPACE Q_NAMESPACE | |
Q_NAMESPACE_EXPORT (EXPORT_MACRO) | |
Q_OBJECT Q_OBJECT | |
Q_PROPERTY (...) | |
Q_REVISION Q_REVISION | |
Q_SET_OBJECT_NAME (Object) | |
Q_SIGNAL Q_SIGNAL | |
Q_SIGNALS Q_SIGNALS | |
Q_SLOT Q_SLOT | |
Q_SLOTS Q_SLOTS | |
T | qobject_cast (const QObject *object) |
T | qobject_cast (QObject *object) |
QT_NO_NARROWING_CONVERSIONS_IN_CONNECT QT_NO_NARROWING_CONVERSIONS_IN_CONNECT | |
void | removeEventFilter (QObject *obj) |
void | setObjectName (const QString &name) |
void | setObjectName (QAnyStringView name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | setProperty (const char *name, QVariant &&value) |
bool | signalsBlocked () const const |
int | startTimer (int interval, Qt::TimerType timerType) |
int | startTimer (std::chrono::milliseconds interval, Qt::TimerType timerType) |
QThread * | thread () const const |
Static Public Attributes | |
static std::function< FMH::MODEL(const QUrl &url)> | informer = &FMStatic::getFileInfoModel |
Additional Inherited Members | |
![]() | |
objectName | |
![]() | |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *context, Functor functor, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, Functor functor) |
bool | disconnect (const QMetaObject::Connection &connection) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
bool | disconnect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
![]() | |
typedef | QObjectList |
![]() | |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const QMetaMethod &signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const QMetaMethod &signal) |
bool | isSignalConnected (const QMetaMethod &signal) const const |
int | receivers (const char *signal) const const |
QObject * | sender () const const |
int | senderSignalIndex () const const |
virtual void | timerEvent (QTimerEvent *event) |
Detailed Description
The FileLoader class asynchronously loads batches of files from a given list of local directories or tags, allowing to filter them by name, mime-types, and much more.
The execution of the file listing will be moved into a different thread, - so to retrieve the information you will depend on the exposed signals and the informer
callback function to give structure to the data.
- See also
- informer
The following code snippet demonstrates the usage, by listing all the content in the downloads and pictures local directories.
Definition at line 75 of file fileloader.h.
Constructor & Destructor Documentation
◆ FileLoader()
Creates a new instance, the execution will be moved to a different thread.
Definition at line 12 of file fileloader.cpp.
◆ ~FileLoader()
Definition at line 24 of file fileloader.cpp.
Member Function Documentation
◆ batchCount()
The amount of items which will be dispatched while iterating throughout all the given directories.
Definition at line 35 of file fileloader.cpp.
◆ finished
|
signal |
Emitted once the operation has completely finished retrieving all the existing files or reached the limit number of requested files.
- Parameters
-
items all of the retrieved items. The items data model is created using the informer
callback function.
- See also
- informer
- Parameters
-
urls the list of directories given for which the item were retrieved.
◆ itemReady
|
signal |
Emitted for every single item that becomes available.
- Parameters
-
item the packaged item, formed by the informer
callback function.urls the list of directories given for which the item were retrieved.
◆ itemsReady
|
signal |
Emitted when the batch of file items is ready.
- See also
- setBatchCount
- Parameters
-
items the packaged list of items, formed by the informer
callback function.urls the list of directories given for which the item were retrieved.
◆ requestPath()
Sends the request to start iterating throughout all the given location URLs, and with the given parameters.
- Parameters
-
urls the list of directories or locations to iterate. This operation only supports local directories and tags. recursive Whether the iteration should be done recursively and navigate sub-folder structures nameFilters a list of filtering strings, this can be used with regular expressions, for example *.jpg
to only list files ending with the given suffix. Dy default this is set to an empty array, so nothing will be filtered.filters the possible QDir filters. By default this is set to QDir::Files
.limit the limit of files to retrieve. By default this is is set to 99999
.
Definition at line 40 of file fileloader.cpp.
◆ setBatchCount()
Set the amount of items to be dispatched via the itemReady
signal.
This allows to dispatch item files which are ready and don't have to wait for the operation to finished completely, in case there are too many files to wait for.
- See also
- itemsReady
- Parameters
-
count the amount of items
Definition at line 30 of file fileloader.cpp.
Member Data Documentation
◆ informer
|
static |
A callback function which structures the retrieved file URLs, with the required information.
This callback function will receive the file URL, and expects a FMH::MODEL to be formed and returned. By default this informer callback function is set to FMStatic::getFileInfoModel
, which retrieves basic information about a file.
- See also
- FMStatic::getFileInfoModel
- Parameters
-
url the file URL retrieved
- Returns
- the formed data model based on the given file URL
Definition at line 116 of file fileloader.h.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2025 The KDE developers.
Generated on Fri Apr 25 2025 11:51:37 by doxygen 1.13.2 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.