FMStatic
#include <fmstatic.h>
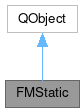
Classes | |
struct | PATH_CONTENT |
Public Types | |
enum | FILTER_TYPE : int { AUDIO , VIDEO , TEXT , IMAGE , DOCUMENT , COMPRESSED , FONT , NONE } |
enum | PATHTYPE_KEY : int { PLACES_PATH , REMOTE_PATH , DRIVES_PATH , REMOVABLE_PATH , TAGS_PATH , UNKNOWN_TYPE , APPS_PATH , TRASH_PATH , SEARCH_PATH , CLOUD_PATH , FISH_PATH , MTP_PATH , QUICK_PATH , BOOKMARKS_PATH , OTHER_PATH } |
Public Slots | |
static void | bookmark (const QUrl &url) |
static bool | checkFileType (const FMStatic::FILTER_TYPE &type, const QString &mimeTypeName) |
static bool | checkFileType (const int &type, const QString &mimeTypeName) |
static bool | copy (const QList< QUrl > &urls, const QUrl &destinationDir) |
static bool | createDir (const QUrl &path, const QString &name) |
static bool | createFile (const QUrl &path, const QString &name) |
static bool | createSymlink (const QUrl &path, const QUrl &where) |
static bool | cut (const QList< QUrl > &urls, const QUrl &where) |
static bool | cut (const QList< QUrl > &urls, const QUrl &where, const QString &name) |
static const QString | dirConfIcon (const QUrl &path) |
static void | emptyTrash () |
static QUrl | fileDir (const QUrl &path) |
static bool | fileExists (const QUrl &path) |
static FMH::MODEL_LIST | getDefaultPaths () |
static FMH::MODEL_LIST | getDevices () |
static const QVariantMap | getFileInfo (const QUrl &path) |
static const FMH::MODEL | getFileInfoModel (const QUrl &path) |
static const QString | getIconName (const QUrl &path) |
static const QString | getMime (const QUrl &path) |
static FMStatic::PATHTYPE_KEY | getPathType (const QUrl &url) |
static bool | group (const QList< QUrl > &urls, const QUrl &destinationDir, const QString &name) |
static QString | homePath () |
static bool | isCloud (const QUrl &path) |
static bool | isDefaultPath (const QString &path) |
static bool | isDir (const QUrl &path) |
static void | moveToTrash (const QList< QUrl > &urls) |
static QStringList | nameFilters (const int &type) |
static void | openLocation (const QStringList &urls) |
static void | openUrl (const QUrl &url) |
static FMH::MODEL_LIST | packItems (const QStringList &items, const QString &type) |
static QUrl | parentDir (const QUrl &path) |
static bool | removeDir (const QUrl &path) |
static bool | removeFiles (const QList< QUrl > &urls) |
static bool | rename (const QUrl &url, const QString &name) |
static FMH::MODEL_LIST | search (const QString &query, const QUrl &path, const bool &hidden=false, const bool &onlyDirs=false, const QStringList &filters=QStringList()) |
static void | setDirConf (const QUrl &path, const QString &group, const QString &key, const QVariant &value) |
Static Public Member Functions | |
static QStringList | getMimeTypeSuffixes (const FILTER_TYPE &type, QString(*cb)(QString)=nullptr) |
static QString | PathTypeLabel (const FMStatic::PATHTYPE_KEY &key) |
![]() | |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *context, Functor functor, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, Functor functor) |
bool | disconnect (const QMetaObject::Connection &connection) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
bool | disconnect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
Additional Inherited Members | |
![]() | |
objectName | |
![]() | |
QObject (QObject *parent) | |
QBindable< QString > | bindableObjectName () |
bool | blockSignals (bool block) |
const QObjectList & | children () const const |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) const const |
bool | disconnect (const QObject *receiver, const char *method) const const |
void | dumpObjectInfo () const const |
void | dumpObjectTree () const const |
QList< QByteArray > | dynamicPropertyNames () const const |
virtual bool | event (QEvent *e) |
virtual bool | eventFilter (QObject *watched, QEvent *event) |
T | findChild (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QRegularExpression &re, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (Qt::FindChildOptions options) const const |
bool | inherits (const char *className) const const |
void | installEventFilter (QObject *filterObj) |
bool | isQuickItemType () const const |
bool | isWidgetType () const const |
bool | isWindowType () const const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const const |
void | moveToThread (QThread *targetThread) |
QString | objectName () const const |
void | objectNameChanged (const QString &objectName) |
QObject * | parent () const const |
QVariant | property (const char *name) const const |
Q_CLASSINFO (Name, Value) | |
Q_EMIT Q_EMIT | |
Q_ENUM (...) | |
Q_ENUM_NS (...) | |
Q_ENUMS (...) | |
Q_FLAG (...) | |
Q_FLAG_NS (...) | |
Q_FLAGS (...) | |
Q_GADGET Q_GADGET | |
Q_GADGET_EXPORT (EXPORT_MACRO) | |
Q_INTERFACES (...) | |
Q_INVOKABLE Q_INVOKABLE | |
Q_MOC_INCLUDE Q_MOC_INCLUDE | |
Q_NAMESPACE Q_NAMESPACE | |
Q_NAMESPACE_EXPORT (EXPORT_MACRO) | |
Q_OBJECT Q_OBJECT | |
Q_PROPERTY (...) | |
Q_REVISION Q_REVISION | |
Q_SET_OBJECT_NAME (Object) | |
Q_SIGNAL Q_SIGNAL | |
Q_SIGNALS Q_SIGNALS | |
Q_SLOT Q_SLOT | |
Q_SLOTS Q_SLOTS | |
T | qobject_cast (const QObject *object) |
T | qobject_cast (QObject *object) |
QT_NO_NARROWING_CONVERSIONS_IN_CONNECT QT_NO_NARROWING_CONVERSIONS_IN_CONNECT | |
void | removeEventFilter (QObject *obj) |
void | setObjectName (const QString &name) |
void | setObjectName (QAnyStringView name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | setProperty (const char *name, QVariant &&value) |
bool | signalsBlocked () const const |
int | startTimer (int interval, Qt::TimerType timerType) |
int | startTimer (std::chrono::milliseconds interval, Qt::TimerType timerType) |
QThread * | thread () const const |
![]() | |
typedef | QObjectList |
![]() | |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const QMetaMethod &signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const QMetaMethod &signal) |
bool | isSignalConnected (const QMetaMethod &signal) const const |
int | receivers (const char *signal) const const |
QObject * | sender () const const |
int | senderSignalIndex () const const |
virtual void | timerEvent (QTimerEvent *event) |
Detailed Description
The FMStatic class is a group of static file management methods, this class has a constructor only as a way to register it to QML, however all methods in here are static.
Definition at line 29 of file fmstatic.h.
Member Enumeration Documentation
◆ FILTER_TYPE
enum FMStatic::FILTER_TYPE : int |
The common file types for filtering.
Definition at line 46 of file fmstatic.h.
◆ PATHTYPE_KEY
enum FMStatic::PATHTYPE_KEY : int |
The different location types supported. Most of them need of KDE KIO framework to be fully operational.
Enumerator | |
---|---|
PLACES_PATH | Local paths, such as the Downloads, Pictures, etc. |
REMOTE_PATH | Remote locations, such as servers accessed via SSH or FTP. |
DRIVES_PATH | Hard drives locations. |
REMOVABLE_PATH | Removable places, such as optic CDs, USB pen drives, etc. |
TAGS_PATH | A tag location. |
UNKNOWN_TYPE | Unknown location type. |
APPS_PATH | The applications location. Accessed with KIO via the |
TRASH_PATH | The trash location.
|
SEARCH_PATH | A search results. |
CLOUD_PATH | A remote cloud server path. |
FISH_PATH | A remote SHH or FTP.
|
MTP_PATH | MTP path. |
QUICK_PATH | The common standard paths. |
BOOKMARKS_PATH | A bookmarked location. |
OTHER_PATH | Any other path. |
Definition at line 258 of file fmstatic.h.
Member Function Documentation
◆ bookmark
|
staticslot |
Add a directory URL to the places bookmarks.
- Parameters
-
url the directory URL to be bookmarked
Definition at line 496 of file fmstatic.cpp.
◆ checkFileType [1/2]
|
staticslot |
Definition at line 491 of file fmstatic.cpp.
◆ checkFileType [2/2]
|
staticslot |
Checks if a mime-type belongs to a file type, for example, whether image/jpg
belongs to the type FMH::FILTER_TYPE
- Parameters
-
type FMH::FILTER_TYPE value mimeTypeName the mime type name
- Returns
- whether the type contains the given name
Definition at line 486 of file fmstatic.cpp.
◆ copy
Perform a copy of the files to the given destination.
- Parameters
-
urls list of URLs to be copy destinationDir destination
- Returns
- whether the operation has been successful
Definition at line 216 of file fmstatic.cpp.
◆ createDir
Creates a new directory given a base path and a name.
- Parameters
-
path base path where to create the new directory name the new directory name
- Returns
- whether the operation was successful
Definition at line 371 of file fmstatic.cpp.
◆ createFile
Creates a file given the base directory path and a file name.
- Parameters
-
path the base location path name the name of the new file to be created with the extension
- Returns
- whether the operation was successful
Definition at line 382 of file fmstatic.cpp.
◆ createSymlink
Creates a symbolic link to a given file URL.
- Parameters
-
path file to be linked where destination of the symbolic link
- Returns
- whether the operation was successful
Definition at line 394 of file fmstatic.cpp.
◆ cut [1/2]
Perform a move/cut of a list of files to a destination.
This function also moves the associated tags.
- Parameters
-
urls list of URLs to be moved where destination path
- Returns
- whether the operation has been successful
Definition at line 256 of file fmstatic.cpp.
◆ cut [2/2]
|
staticslot |
- See also
- cut
- Parameters
-
name new name of the directory where the files will be pasted
Definition at line 261 of file fmstatic.cpp.
◆ dirConfIcon
Return the icon name set in the directory .directory
conf file.
The passed path must be a local file URL.
- Parameters
-
path the directory location
- Returns
- the icon name
Definition at line 437 of file fmstatic.cpp.
◆ emptyTrash
|
staticslot |
Empty the trashcan.
Definition at line 333 of file fmstatic.cpp.
◆ fileDir
Given a file URL, return its parent directory.
- Note
- If the given path is a directory then returns the same path.
- Parameters
-
path file path URL
- Returns
- the directory URL
Definition at line 160 of file fmstatic.cpp.
◆ fileExists
|
staticslot |
Checks if a local file exists in the file system.
- Parameters
-
path file URL
- Returns
- whether the file path exists locally
Definition at line 155 of file fmstatic.cpp.
◆ getDefaultPaths
|
staticslot |
A model list of the default paths in most systems, such as Home, Pictures, Video, Downloads, Music and Documents folders.
- Returns
- the packaged model with information for each directory
Definition at line 74 of file fmstatic.cpp.
◆ getDevices
|
staticslot |
Devices mounted in the file system.
- Returns
- list of devices represented as a FMH::MODEL_LIST with information
Definition at line 110 of file fmstatic.cpp.
◆ getFileInfo
|
staticslot |
◆ getFileInfoModel
|
staticslot |
◆ getIconName
Returns the icon name for certain file.
The file path must be represented as a local file URL. It also looks into the directory conf file to get the directory custom icon.
- Note
- To get an abstract icon, use a template name, such as
test.jpg
, to get an icon for the JPG image type. The file does not need to exists.
- Parameters
-
path file path
- Returns
Definition at line 618 of file fmstatic.cpp.
◆ getMime
Get the mime type of the given file path.
- Parameters
-
path the file path
- Returns
- the mime-type string
Definition at line 506 of file fmstatic.cpp.
◆ getMimeTypeSuffixes()
|
inlinestatic |
Given a FILTER_TYPE and its associated mime-types, return a list of all the supported file extension suffixes.
- Parameters
-
type the FILTER_TYPE cb a callback function to modify the gathered suffix extension. This function will receive the supported suffix and it can return a new string of a modified suffix or the same one. This is optional.
- Returns
- the list of associated file extensions
Definition at line 191 of file fmstatic.h.
◆ getPathType
|
staticslot |
Given a file URL with a well defined scheme, get the PATHTYPE_KEY.
- See also
- PATHTYPE_KEY
- Parameters
-
url the file URL
- Returns
- the file PATHTYPE_KEY
Definition at line 646 of file fmstatic.cpp.
◆ group
|
staticslot |
Perform a move operation of the given files to a new destination.
- Parameters
-
urls list of URLs to be copied destinationDir destination name the name of the new directory where all the entries will be grouped/moved into
- Returns
- whether the operation has been successful
Definition at line 244 of file fmstatic.cpp.
◆ homePath
|
staticslot |
◆ isCloud
|
staticslot |
Whether a path is a URL server instead of a local file.
- Parameters
-
path
- Returns
Definition at line 150 of file fmstatic.cpp.
◆ isDefaultPath
|
staticslot |
Checks if a given path URL is a default path as found in the defaultPaths
method.
- Parameters
-
path the directory location path
- Returns
- whether is a default path
Definition at line 122 of file fmstatic.cpp.
◆ isDir
|
staticslot |
Whether a local file URL is a directory.
- Parameters
-
path file URL
- Returns
- is a directory
Definition at line 139 of file fmstatic.cpp.
◆ moveToTrash
Moves to the trashcan the provided file URLs.
The associated tags are kept in case the files are restored.
- Parameters
-
urls the file URLs
Definition at line 323 of file fmstatic.cpp.
◆ nameFilters
|
staticslot |
Given a filter type return a list of associated name filters, as their suffixes.
- Parameters
-
type the filter type to be mapped to a FMH::FILTER_TYPE
- See also
- FMH::FILTER_LIST
Definition at line 501 of file fmstatic.cpp.
◆ openLocation
|
staticslot |
Open the file URLs with the default file manager.
- Parameters
-
urls file or location URLs to be opened
Definition at line 423 of file fmstatic.cpp.
◆ openUrl
|
staticslot |
Given a URL it tries to open it using the default application associated to it.
- Parameters
-
url the file URL to be opened
Definition at line 406 of file fmstatic.cpp.
◆ packItems
|
staticslot |
Given a list of path URLs pack all the info of such files as a FMH::MODEL_LIST.
- Parameters
-
items list of local URLs type the type of the list of URLs, such as local, remote etc. This value is inserted with the key FMH::MODEL_KEY::TYPE
- Returns
Definition at line 57 of file fmstatic.cpp.
◆ parentDir
Given a file URL return its parent directory.
- Parameters
-
path the file URL
- Returns
- the parent directory URL if it exists otherwise returns the passed URL
Definition at line 127 of file fmstatic.cpp.
◆ PathTypeLabel()
|
static |
Given a PATHTYPE_KEY return a user friendly string.
- Warning
- This is a user visible and translatable string, so it should not be used as a key anywhere
- Parameters
-
key the PATHTYPE_KEY key
Definition at line 31 of file fmstatic.cpp.
◆ removeDir
|
staticslot |
Remove a directory recursively.
- Parameters
-
path directory URL to be removed
- Returns
- whether the operation has been sucessfull
Definition at line 341 of file fmstatic.cpp.
◆ removeFiles
List of files to be removed completely.
This function also removes the associated tags to the files.
- Parameters
-
urls file URLs to be removed
- Returns
- Whether the operation has been sucessfull
Definition at line 296 of file fmstatic.cpp.
◆ rename
Rename a file.
The associated tags will be updated.
- Parameters
-
url the file URL to be renamed name the new name of the file, not the new URL, for setting a new URl use cut instead.
- Returns
- whether the operation was successful.
Definition at line 366 of file fmstatic.cpp.
◆ search
|
staticslot |
Search for files in a path using name filters.
- Parameters
-
query the term query to be searched, such as ".qml"
or"music"
path the path where to perform or start the search hidden whether to search for hidden files onlyDirs whether to only search for directories and not files filters list of filter patterns such as {"*.qml"}
, it can use regular expressions.
- Returns
- the search results are returned as a FMH::MODEL_LIST
Definition at line 79 of file fmstatic.cpp.
◆ setDirConf
|
staticslot |
Write a configuration key-value entry to the directory conf file.
- Parameters
-
path directory path group the entry group name key the key name of the entry value the value of the entry
Definition at line 464 of file fmstatic.cpp.
Member Data Documentation
◆ AppsPath
|
inlinestatic |
◆ AUDIO_MIMETYPES
|
inlinestatic |
The list of supported audio formats, associated to FILTER_TYPE::AUDIO
Definition at line 51 of file fmstatic.h.
◆ CloudCachePath
◆ COMPRESSED_MIMETYPES
|
inlinestatic |
The list of supported archive formats, associated to FILTER_TYPE::COMPRESSED
Definition at line 154 of file fmstatic.h.
◆ ConfigPath
|
inlinestatic |
◆ DataPath
|
inlinestatic |
◆ defaultPaths
|
inlinestatic |
The internally defined quick standard locations.
Definition at line 447 of file fmstatic.h.
◆ DesktopPath
|
inlinestatic |
◆ DOCUMENT_MIMETYPES
|
inlinestatic |
The list of supported document formats, associated to FILTER_TYPE::DOCUMENT
Definition at line 143 of file fmstatic.h.
◆ DocumentsPath
|
inlinestatic |
Definition at line 440 of file fmstatic.h.
◆ DownloadsPath
|
inlinestatic |
Definition at line 439 of file fmstatic.h.
◆ FILTER_LIST
|
inlinestatic |
Convenient map set of file type extensions.
The values make use of the regex wildcard operator [*] meant for filtering a directory contents, for example. FILTER_LIST[FILTER_TYPE::AUDIO]
could possible return something alike ["*.mp3", "*.mp4", "*.mpeg", "*.wav"]
etc.
Definition at line 220 of file fmstatic.h.
◆ folderIcon
A mapping of the standard location to a icon name.
Definition at line 463 of file fmstatic.h.
◆ FONT_MIMETYPES
|
inlinestatic |
The list of supported font formats, associated to FILTER_TYPE::FONT
Definition at line 169 of file fmstatic.h.
◆ HomePath
|
inlinestatic |
◆ IMAGE_MIMETYPES
|
inlinestatic |
The list of supported image formats, associated to FILTER_TYPE::IMAGE
Definition at line 118 of file fmstatic.h.
◆ MusicPath
|
inlinestatic |
Definition at line 437 of file fmstatic.h.
◆ PATHTYPE_SCHEME
|
inlinestatic |
The map of the PATH_TYPE to its associated protocol scheme.
For example PATHTYPE_SCHEME[PATHTYPE_KEY::TRASH_PATH] = "trash"
, PATHTYPE_SCHEME[PATHTYPE_KEY::PLACES_PATH] = "file"
Definition at line 339 of file fmstatic.h.
◆ PATHTYPE_SCHEME_NAME
|
inlinestatic |
The protocol scheme mapped to its PATHTYPE_KEY.
Where PATHTYPE_SCHEME_NAME["file"] = FMH::PATHTYPE_KEY::PLACES_PATH
Definition at line 357 of file fmstatic.h.
◆ PATHTYPE_URI
|
inlinestatic |
Similar to PATHTYPE_SCHEME, but mapped with the complete scheme.
For example PATHTYPE_URIE[PATHTYPE_KEY::TRASH_PATH] = "trash://"
, PATHTYPE_URI[PLACES_PATH] = "file://"
Definition at line 375 of file fmstatic.h.
◆ PicturesPath
|
inlinestatic |
Definition at line 438 of file fmstatic.h.
◆ RootPath
|
inlinestatic |
◆ SUPPORTED_MIMETYPES
|
inlinestatic |
The map set of the supported mime types for the FM classes.
This structure maps the FILTER_TYPE
to the associated list of mime types.
- For example
SUPPORTED_MIMETYPES[FILTER_TYPE::AUDIO]
would return a list of mimetypes associated to the FILTER_TYPE::AUDIO, such as"audio/mpeg", "audio/mp4", "audio/flac", "audio/ogg", "audio/wav"
.
Definition at line 177 of file fmstatic.h.
◆ TEXT_MIMETYPES
|
inlinestatic |
The list of supported text formats, associated to FILTER_TYPE::TEXT
Definition at line 79 of file fmstatic.h.
◆ TrashPath
|
inlinestatic |
◆ VIDEO_MIMETYPES
|
inlinestatic |
The list of supported video formats, associated to FILTER_TYPE::VIDEO
Definition at line 61 of file fmstatic.h.
◆ VideosPath
|
inlinestatic |
Definition at line 441 of file fmstatic.h.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2025 The KDE developers.
Generated on Fri Apr 18 2025 12:07:49 by doxygen 1.13.2 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.