Konsole::Session
#include <Session.h>
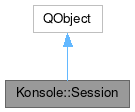
Public Types | |
enum | TabTitleContext { LocalTabTitle , RemoteTabTitle } |
enum | TitleRole { NameRole , DisplayedTitleRole } |
![]() | |
typedef | QObjectList |
Properties | |
QString | keyBindings |
QString | name |
int | processId |
QSize | size |
![]() | |
objectName | |
Signals | |
void | activity () |
void | bellRequest (const QString &message) |
void | changeBackgroundColorRequest (const QColor &) |
void | changeTabTextColorRequest (int) |
void | cursorChanged (Emulation::KeyboardCursorShape cursorShape, bool blinkingCursorEnabled) |
void | finished () |
void | flowControlEnabledChanged (bool enabled) |
void | openUrlRequest (const QString &url) |
void | profileChangeCommandReceived (const QString &text) |
void | profileChanged (const QString &profile) |
void | receivedData (const QString &text) |
void | resizeRequest (const QSize &size) |
void | silence () |
void | started () |
void | stateChanged (int state) |
void | titleChanged () |
Public Slots | |
void | close () |
void | run () |
void | runEmptyPTY () |
void | setUserTitle (int, const QString &caption) |
Public Member Functions | |
Session (QObject *parent=nullptr) | |
QStringList | arguments () const |
void | clearHistory () |
QString | currentDir () |
Emulation * | emulation () const |
QStringList | environment () const |
bool | flowControlEnabled () const |
int | foregroundProcessId () const |
QString | foregroundProcessName () |
int | getPtySlaveFd () const |
bool | hasDarkBackground () const |
const HistoryType & | historyType () const |
QString | iconName () const |
QString | iconText () const |
QString | initialWorkingDirectory () |
bool | isMonitorActivity () const |
bool | isMonitorSilence () const |
bool | isRunning () const |
bool | isTitleChanged () const |
QString | keyBindings () const |
QString | nameTitle () const |
int | processId () const |
QString | profileKey () const |
QString | program () const |
void | refresh () |
void | removeView (TerminalDisplay *widget) |
void | sendKeyEvent (QKeyEvent *e) const |
bool | sendSignal (int signal) |
void | sendText (const QString &text) const |
int | sessionId () const |
void | setAddToUtmp (bool) |
void | setArguments (const QStringList &arguments) |
void | setAutoClose (bool b) |
void | setCodec (QTextCodec *codec) const |
void | setDarkBackground (bool darkBackground) |
void | setEnvironment (const QStringList &environment) |
void | setFlowControlEnabled (bool enabled) |
void | setHistoryType (const HistoryType &type) |
void | setIconName (const QString &iconName) |
void | setIconText (const QString &iconText) |
void | setInitialWorkingDirectory (const QString &dir) |
void | setKeyBindings (const QString &id) |
void | setMonitorActivity (bool) |
void | setMonitorSilence (bool) |
void | setMonitorSilenceSeconds (int seconds) |
void | setProfileKey (const QString &profileKey) |
void | setProgram (const QString &program) |
void | setSize (const QSize &size) |
void | setTabTitleFormat (TabTitleContext context, const QString &format) |
void | setTitle (TitleRole role, const QString &title) |
void | setView (TerminalDisplay *widget) |
QSize | size () |
QString | tabTitleFormat (TabTitleContext context) const |
QString | title (TitleRole role) const |
QString | userTitle () const |
TerminalDisplay * | view () const |
![]() | |
QObject (QObject *parent) | |
QBindable< QString > | bindableObjectName () |
bool | blockSignals (bool block) |
const QObjectList & | children () const const |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) const const |
bool | disconnect (const QObject *receiver, const char *method) const const |
void | dumpObjectInfo () const const |
void | dumpObjectTree () const const |
QList< QByteArray > | dynamicPropertyNames () const const |
virtual bool | event (QEvent *e) |
virtual bool | eventFilter (QObject *watched, QEvent *event) |
T | findChild (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QRegularExpression &re, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (Qt::FindChildOptions options) const const |
bool | inherits (const char *className) const const |
void | installEventFilter (QObject *filterObj) |
bool | isQuickItemType () const const |
bool | isWidgetType () const const |
bool | isWindowType () const const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const const |
void | moveToThread (QThread *targetThread) |
QString | objectName () const const |
void | objectNameChanged (const QString &objectName) |
QObject * | parent () const const |
QVariant | property (const char *name) const const |
Q_CLASSINFO (Name, Value) | |
Q_EMIT Q_EMIT | |
Q_ENUM (...) | |
Q_ENUM_NS (...) | |
Q_ENUMS (...) | |
Q_FLAG (...) | |
Q_FLAG_NS (...) | |
Q_FLAGS (...) | |
Q_GADGET Q_GADGET | |
Q_GADGET_EXPORT (EXPORT_MACRO) | |
Q_INTERFACES (...) | |
Q_INVOKABLE Q_INVOKABLE | |
Q_MOC_INCLUDE Q_MOC_INCLUDE | |
Q_NAMESPACE Q_NAMESPACE | |
Q_NAMESPACE_EXPORT (EXPORT_MACRO) | |
Q_OBJECT Q_OBJECT | |
Q_PROPERTY (...) | |
Q_REVISION Q_REVISION | |
Q_SET_OBJECT_NAME (Object) | |
Q_SIGNAL Q_SIGNAL | |
Q_SIGNALS Q_SIGNALS | |
Q_SLOT Q_SLOT | |
Q_SLOTS Q_SLOTS | |
T | qobject_cast (const QObject *object) |
T | qobject_cast (QObject *object) |
QT_NO_NARROWING_CONVERSIONS_IN_CONNECT QT_NO_NARROWING_CONVERSIONS_IN_CONNECT | |
void | removeEventFilter (QObject *obj) |
void | setObjectName (const QString &name) |
void | setObjectName (QAnyStringView name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | setProperty (const char *name, QVariant &&value) |
bool | signalsBlocked () const const |
int | startTimer (int interval, Qt::TimerType timerType) |
int | startTimer (std::chrono::milliseconds interval, Qt::TimerType timerType) |
QThread * | thread () const const |
Additional Inherited Members | |
![]() | |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *context, Functor functor, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, Functor functor) |
bool | disconnect (const QMetaObject::Connection &connection) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
bool | disconnect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
![]() | |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const QMetaMethod &signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const QMetaMethod &signal) |
bool | isSignalConnected (const QMetaMethod &signal) const const |
int | receivers (const char *signal) const const |
QObject * | sender () const const |
int | senderSignalIndex () const const |
virtual void | timerEvent (QTimerEvent *event) |
Detailed Description
Represents a terminal session consisting of a pseudo-teletype and a terminal emulation.
The pseudo-teletype (or PTY) handles I/O between the terminal process and Konsole. The terminal emulation ( Emulation and subclasses ) processes the output stream from the PTY and produces a character image which is then shown on views connected to the session.
Each Session can be connected to one or more views by using the addView() method. The attached views can then display output from the program running in the terminal or send input to the program in the terminal in the form of keypresses and mouse activity.
Member Enumeration Documentation
◆ TabTitleContext
◆ TitleRole
Property Documentation
◆ keyBindings
◆ name
◆ processId
◆ size
Constructor & Destructor Documentation
◆ Session()
Session::Session | ( | QObject * | parent = nullptr | ) |
Constructs a new session.
To start the terminal process, call the run() method, after specifying the program and arguments using setProgram() and setArguments()
If no program or arguments are specified explicitly, the Session falls back to using the program specified in the SHELL environment variable.
Definition at line 52 of file Session.cpp.
Member Function Documentation
◆ arguments()
QStringList Session::arguments | ( | ) | const |
Returns the arguments passed to the shell process when run() is called.
Definition at line 647 of file Session.cpp.
◆ bellRequest
|
signal |
Emitted when a bell event occurs in the session.
◆ changeBackgroundColorRequest
|
signal |
Requests that the background color of views on this session should be changed.
◆ changeTabTextColorRequest
|
signal |
Requests that the color the text for any tabs associated with this session should be changed;.
TODO: Document what the parameter does
◆ clearHistory()
void Session::clearHistory | ( | ) |
Clears the history store used by this session.
Definition at line 642 of file Session.cpp.
◆ close
|
slot |
Closes the terminal session.
This sends a hangup signal (SIGHUP) to the terminal process and causes the done(Session*) signal to be emitted.
Definition at line 489 of file Session.cpp.
◆ currentDir()
QString Session::currentDir | ( | ) |
Returns the current working directory of the process.
Definition at line 762 of file Session.cpp.
◆ cursorChanged
|
signal |
Broker for Emulation::cursorChanged() signal.
◆ emulation()
Emulation * Session::emulation | ( | ) | const |
Returns the terminal emulation instance being used to encode / decode characters to / from the process.
Definition at line 549 of file Session.cpp.
◆ environment()
QStringList Session::environment | ( | ) | const |
Returns the environment of this session as a list of strings like VARIABLE=VALUE.
Definition at line 559 of file Session.cpp.
◆ finished
|
signal |
Emitted when the terminal process exits.
◆ flowControlEnabled()
bool Session::flowControlEnabled | ( | ) | const |
Returns whether flow control is enabled for this terminal session.
Definition at line 719 of file Session.cpp.
◆ flowControlEnabledChanged
|
signal |
Emitted when the flow control state changes.
- Parameters
-
enabled True if flow control is enabled or false otherwise.
◆ foregroundProcessId()
int Session::foregroundProcessId | ( | ) | const |
Returns the process id of the terminal's foreground process.
This is initially the same as processId() but can change as the user starts other programs inside the terminal.
Definition at line 743 of file Session.cpp.
◆ foregroundProcessName()
QString Session::foregroundProcessName | ( | ) |
Returns the name of the terminal's foreground process.
Definition at line 748 of file Session.cpp.
◆ getPtySlaveFd()
int Session::getPtySlaveFd | ( | ) | const |
Returns a pty slave file descriptor.
This can be used for display and control a remote terminal.
Definition at line 797 of file Session.cpp.
◆ hasDarkBackground()
bool Session::hasDarkBackground | ( | ) | const |
Returns true if the session has a dark background.
Definition at line 121 of file Session.cpp.
◆ historyType()
const HistoryType & Session::historyType | ( | ) | const |
Returns the type of history store used by this session.
Definition at line 637 of file Session.cpp.
◆ iconName()
QString Session::iconName | ( | ) | const |
Returns the name of the icon associated with this session.
Definition at line 617 of file Session.cpp.
◆ iconText()
QString Session::iconText | ( | ) | const |
Returns the text of the icon associated with this session.
Definition at line 622 of file Session.cpp.
◆ initialWorkingDirectory()
|
inline |
◆ isMonitorActivity()
bool Session::isMonitorActivity | ( | ) | const |
Returns true if monitoring for activity is enabled.
Definition at line 658 of file Session.cpp.
◆ isMonitorSilence()
bool Session::isMonitorSilence | ( | ) | const |
Returns true if monitoring for inactivity (silence) in the session is enabled.
Definition at line 663 of file Session.cpp.
◆ isRunning()
bool Session::isRunning | ( | ) | const |
Returns true if the session is currently running.
This will be true after run() has been called successfully.
Definition at line 125 of file Session.cpp.
◆ isTitleChanged()
bool Session::isTitleChanged | ( | ) | const |
Flag if the title/icon was changed by user/shell.
Definition at line 627 of file Session.cpp.
◆ keyBindings()
QString Session::keyBindings | ( | ) | const |
Returns the name of the key bindings used by this session.
Definition at line 554 of file Session.cpp.
◆ nameTitle()
|
inline |
◆ openUrlRequest
◆ processId()
int Session::processId | ( | ) | const |
Returns the process id of the terminal process.
This is the id used by the system API to refer to the process.
Definition at line 793 of file Session.cpp.
◆ profileChangeCommandReceived
|
signal |
Emitted when a profile change command is received from the terminal.
- Parameters
-
text The text of the command. This is a string of the form "PropertyName=Value;PropertyName=Value ..."
◆ profileChanged
|
signal |
Emitted when the session's profile has changed.
◆ profileKey()
QString Session::profileKey | ( | ) | const |
Returns the profile key associated with this session.
This can be passed to the SessionManager to obtain the current profile settings.
Definition at line 517 of file Session.cpp.
◆ program()
QString Session::program | ( | ) | const |
Returns the program name of the shell process started when run() is called.
Definition at line 652 of file Session.cpp.
◆ receivedData
|
signal |
Emitted when output is received from the terminal process.
◆ refresh()
void Session::refresh | ( | ) |
Attempts to get the shell program to redraw the current display area.
This can be used after clearing the screen, for example, to get the shell to redraw the prompt line.
Definition at line 456 of file Session.cpp.
◆ removeView()
void Session::removeView | ( | TerminalDisplay * | widget | ) |
Removes a view from this session.
When the last view is removed, the session will be closed automatically.
widget
will no longer display output from or send input to the terminal
Definition at line 197 of file Session.cpp.
◆ resizeRequest
|
signal |
TODO: Document me.
Emitted when the terminal process requests a change in the size of the terminal window.
- Parameters
-
size The requested window size in terms of lines and columns.
◆ run
|
slot |
Starts the terminal session.
This creates the terminal process and connects the teletype to it.
Definition at line 220 of file Session.cpp.
◆ runEmptyPTY
|
slot |
Starts the terminal session for "as is" PTY (without the direction a data to internal terminal process).
It can be used for control or display a remote/external terminal.
Definition at line 283 of file Session.cpp.
◆ sendKeyEvent()
void Session::sendKeyEvent | ( | QKeyEvent * | e | ) | const |
Definition at line 504 of file Session.cpp.
◆ sendSignal()
bool Session::sendSignal | ( | int | signal | ) |
Sends the specified signal
to the terminal process.
Definition at line 478 of file Session.cpp.
◆ sendText()
void Session::sendText | ( | const QString & | text | ) | const |
Sends text
to the current foreground terminal program.
Definition at line 499 of file Session.cpp.
◆ sessionId()
int Session::sessionId | ( | ) | const |
Returns the unique ID for this session.
Definition at line 569 of file Session.cpp.
◆ setAddToUtmp()
void Session::setAddToUtmp | ( | bool | set | ) |
Specifies whether a utmp entry should be created for the pty used by this session.
Definition at line 700 of file Session.cpp.
◆ setArguments()
void Session::setArguments | ( | const QStringList & | arguments | ) |
Sets the command line arguments which the session's program will be passed when run() is called.
Definition at line 143 of file Session.cpp.
◆ setAutoClose()
|
inline |
◆ setCodec()
void Session::setCodec | ( | QTextCodec * | codec | ) | const |
Sets the text codec used by this session's terminal emulation.
Definition at line 130 of file Session.cpp.
◆ setDarkBackground()
void Session::setDarkBackground | ( | bool | darkBackground | ) |
Sets whether the session has a dark background or not.
The session uses this information to set the COLORFGBG variable in the process's environment, which allows the programs running in the terminal to determine whether the background is light or dark and use appropriate colors by default.
This has no effect once the session is running.
Definition at line 117 of file Session.cpp.
◆ setEnvironment()
void Session::setEnvironment | ( | const QStringList & | environment | ) |
Sets the environment for this session.
environment
should be a list of strings like VARIABLE=VALUE
Definition at line 564 of file Session.cpp.
◆ setFlowControlEnabled()
void Session::setFlowControlEnabled | ( | bool | enabled | ) |
Sets whether flow control is enabled for this terminal session.
Definition at line 705 of file Session.cpp.
◆ setHistoryType()
void Session::setHistoryType | ( | const HistoryType & | type | ) |
Sets the type of history store used by this session.
Lines of output produced by the terminal are added to the history store. The type of history store used affects the number of lines which can be remembered before they are lost and the storage (in memory, on-disk etc.) used.
Definition at line 632 of file Session.cpp.
◆ setIconName()
void Session::setIconName | ( | const QString & | iconName | ) |
Sets the name of the icon associated with this session.
Definition at line 603 of file Session.cpp.
◆ setIconText()
void Session::setIconText | ( | const QString & | iconText | ) |
Sets the text of the icon associated with this session.
Definition at line 611 of file Session.cpp.
◆ setInitialWorkingDirectory()
void Session::setInitialWorkingDirectory | ( | const QString & | dir | ) |
Sets the initial working directory for the session when it is run This has no effect once the session has been started.
Definition at line 139 of file Session.cpp.
◆ setKeyBindings()
void Session::setKeyBindings | ( | const QString & | id | ) |
Sets the key bindings used by this session.
The bindings specify how input key sequences are translated into the character stream which is sent to the terminal.
- Parameters
-
id The name of the key bindings to use. The names of available key bindings can be determined using the KeyboardTranslatorManager class.
Definition at line 574 of file Session.cpp.
◆ setMonitorActivity()
void Session::setMonitorActivity | ( | bool | _monitor | ) |
Enables monitoring for activity in the session.
This will cause notifySessionState() to be emitted with the NOTIFYACTIVITY state flag when output is received from the terminal.
Definition at line 668 of file Session.cpp.
◆ setMonitorSilence()
void Session::setMonitorSilence | ( | bool | _monitor | ) |
Enables monitoring for silence in the session.
This will cause notifySessionState() to be emitted with the NOTIFYSILENCE state flag when output is not received from the terminal for a certain period of time, specified with setMonitorSilenceSeconds()
Definition at line 676 of file Session.cpp.
◆ setMonitorSilenceSeconds()
void Session::setMonitorSilenceSeconds | ( | int | seconds | ) |
Definition at line 692 of file Session.cpp.
◆ setProfileKey()
void Session::setProfileKey | ( | const QString & | profileKey | ) |
Sets the profile associated with this session.
- Parameters
-
profileKey A key which can be used to obtain the current profile settings from the SessionManager
Definition at line 511 of file Session.cpp.
◆ setProgram()
void Session::setProgram | ( | const QString & | program | ) |
Sets the program to be executed when run() is called.
Definition at line 135 of file Session.cpp.
◆ setSize()
void Session::setSize | ( | const QSize & | size | ) |
Emits a request to resize the session to accommodate the specified window size.
- Parameters
-
size The size in lines and columns to request.
Definition at line 735 of file Session.cpp.
◆ setTabTitleFormat()
void Session::setTabTitleFormat | ( | TabTitleContext | context, |
const QString & | format ) |
Sets the format used by this session for tab titles.
- Parameters
-
context The context whoose format should be set. format The tab title format. This may be a mixture of plain text and dynamic elements denoted by a '' character followed by a letter. (eg. d for directory). The dynamic elements available depend on the context
Definition at line 374 of file Session.cpp.
◆ setTitle()
Sets the session's title for the specified role
to title
.
Definition at line 579 of file Session.cpp.
◆ setUserTitle
|
slot |
Changes the session title or other customizable aspects of the terminal emulation display.
For a list of what may be changed see the Emulation::titleChanged() signal.
Definition at line 295 of file Session.cpp.
◆ setView()
void Session::setView | ( | TerminalDisplay * | widget | ) |
Adds a new view for this session.
The viewing widget will display the output from the terminal and input from the viewing widget (key presses, mouse activity etc.) will be sent to the terminal.
Views can be removed using removeView(). The session is automatically closed when the last view is removed.
Definition at line 153 of file Session.cpp.
◆ size()
QSize Session::size | ( | ) |
Returns the terminal session's window size in lines and columns.
Definition at line 730 of file Session.cpp.
◆ started
|
signal |
Emitted when the terminal process starts.
◆ stateChanged
|
signal |
Emitted when the activity state of this session changes.
- Parameters
-
state The new state of the session. This may be one of NOTIFYNORMAL, NOTIFYSILENCE or NOTIFYACTIVITY
◆ tabTitleFormat()
QString Session::tabTitleFormat | ( | TabTitleContext | context | ) | const |
Returns the format used by this session for tab titles.
Definition at line 382 of file Session.cpp.
◆ title()
Returns the session's title for the specified role
.
Definition at line 592 of file Session.cpp.
◆ titleChanged
|
signal |
Emitted when the session's title has changed.
◆ userTitle()
QString Session::userTitle | ( | ) | const |
Return the session title set by the user (ie.
the program running in the terminal), or an empty string if the user has not set a custom title
Definition at line 369 of file Session.cpp.
◆ view()
TerminalDisplay * Session::view | ( | ) | const |
Returns the view connected to this session.
Definition at line 148 of file Session.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2024 The KDE developers.
Generated on Fri Dec 20 2024 11:55:13 by doxygen 1.12.0 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.