Document
#include <Document.h>
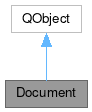
Public Slots | |
Node * | activeNode () const |
int | animationLength () |
QByteArray | annotation (const QString &type) |
QString | annotationDescription (const QString &type) const |
QStringList | annotationTypes () const |
qreal | audioLevel () const |
QList< QString > | audioTracks () const |
bool | autosave () |
QColor | backgroundColor () |
bool | batchmode () const |
QRect | bounds () const |
Document * | clone () const |
bool | close () |
QString | colorDepth () const |
QString | colorModel () const |
QString | colorProfile () const |
CloneLayer * | createCloneLayer (const QString &name, const Node *source) |
ColorizeMask * | createColorizeMask (const QString &name) |
FileLayer * | createFileLayer (const QString &name, const QString fileName, const QString scalingMethod, const QString scalingFilter="Bicubic") |
FillLayer * | createFillLayer (const QString &name, const QString generatorName, InfoObject &configuration, Selection &selection) |
FilterLayer * | createFilterLayer (const QString &name, Filter &filter, Selection &selection) |
FilterMask * | createFilterMask (const QString &name, Filter &filter, const Node *selection_source) |
FilterMask * | createFilterMask (const QString &name, Filter &filter, Selection &selection) |
GroupLayer * | createGroupLayer (const QString &name) |
Node * | createNode (const QString &name, const QString &nodeType) |
SelectionMask * | createSelectionMask (const QString &name) |
TransformMask * | createTransformMask (const QString &name) |
TransparencyMask * | createTransparencyMask (const QString &name) |
VectorLayer * | createVectorLayer (const QString &name) |
void | crop (int x, int y, int w, int h) |
int | currentTime () |
QString | documentInfo () const |
bool | exportImage (const QString &filename, const InfoObject &exportConfiguration) |
QString | fileName () const |
void | flatten () |
int | framesPerSecond () |
int | fullClipRangeEndTime () |
int | fullClipRangeStartTime () |
GridConfig * | gridConfig () |
GuidesConfig * | guidesConfig () |
Q_DECL_DEPRECATED bool | guidesLocked () const |
Q_DECL_DEPRECATED bool | guidesVisible () const |
int | height () const |
Q_DECL_DEPRECATED QList< qreal > | horizontalGuides () const |
bool | importAnimation (const QList< QString > &files, int firstFrame, int step) |
void | lock () |
bool | modified () const |
QString | name () const |
Node * | nodeByName (const QString &name) const |
Node * | nodeByUniqueID (const QUuid &id) const |
QByteArray | pixelData (int x, int y, int w, int h) const |
int | playBackEndTime () |
int | playBackStartTime () |
QImage | projection (int x=0, int y=0, int w=0, int h=0) const |
void | refreshProjection () |
void | removeAnnotation (const QString &type) |
void | resizeImage (int x, int y, int w, int h) |
int | resolution () const |
Node * | rootNode () const |
void | rotateImage (double radians) |
bool | save () |
bool | saveAs (const QString &filename) |
void | scaleImage (int w, int h, int xres, int yres, QString strategy) |
Selection * | selection () const |
void | setActiveNode (Node *value) |
void | setAnnotation (const QString &type, const QString &description, const QByteArray &annotation) |
void | setAudioLevel (const qreal level) |
bool | setAudioTracks (const QList< QString > files) const |
void | setAutosave (bool active) |
bool | setBackgroundColor (const QColor &color) |
void | setBatchmode (bool value) |
bool | setColorProfile (const QString &colorProfile) |
bool | setColorSpace (const QString &colorModel, const QString &colorDepth, const QString &colorProfile) |
void | setCurrentTime (int time) |
void | setDocumentInfo (const QString &document) |
void | setFileName (QString value) |
void | setFramesPerSecond (int fps) |
void | setFullClipRangeEndTime (int endTime) |
void | setFullClipRangeStartTime (int startTime) |
void | setGridConfig (GridConfig *gridConfig) |
void | setGuidesConfig (GuidesConfig *guidesConfig) |
Q_DECL_DEPRECATED void | setGuidesLocked (bool locked) |
Q_DECL_DEPRECATED void | setGuidesVisible (bool visible) |
void | setHeight (int value) |
Q_DECL_DEPRECATED void | setHorizontalGuides (const QList< qreal > &lines) |
void | setModified (bool modified) |
void | setName (QString value) |
void | setPlayBackRange (int start, int stop) |
void | setResolution (int value) |
void | setSelection (Selection *value) |
Q_DECL_DEPRECATED void | setVerticalGuides (const QList< qreal > &lines) |
void | setWidth (int value) |
void | setXOffset (int x) |
void | setXRes (double xRes) const |
void | setYOffset (int y) |
void | setYRes (double yRes) const |
void | shearImage (double angleX, double angleY) |
QImage | thumbnail (int w, int h) const |
QList< Node * > | topLevelNodes () const |
bool | tryBarrierLock () |
void | unlock () |
Q_DECL_DEPRECATED QList< qreal > | verticalGuides () const |
void | waitForDone () |
int | width () const |
int | xOffset () const |
double | xRes () const |
int | yOffset () const |
double | yRes () const |
Public Member Functions | |
Document (KisDocument *document, bool ownsDocument, QObject *parent=0) | |
bool | operator!= (const Document &other) const |
bool | operator== (const Document &other) const |
![]() | |
QObject (QObject *parent) | |
QBindable< QString > | bindableObjectName () |
bool | blockSignals (bool block) |
const QObjectList & | children () const const |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) const const |
bool | disconnect (const QObject *receiver, const char *method) const const |
void | dumpObjectInfo () const const |
void | dumpObjectTree () const const |
QList< QByteArray > | dynamicPropertyNames () const const |
virtual bool | event (QEvent *e) |
virtual bool | eventFilter (QObject *watched, QEvent *event) |
T | findChild (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QRegularExpression &re, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (Qt::FindChildOptions options) const const |
bool | inherits (const char *className) const const |
void | installEventFilter (QObject *filterObj) |
bool | isQuickItemType () const const |
bool | isWidgetType () const const |
bool | isWindowType () const const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const const |
void | moveToThread (QThread *targetThread) |
QString | objectName () const const |
void | objectNameChanged (const QString &objectName) |
QObject * | parent () const const |
QVariant | property (const char *name) const const |
Q_CLASSINFO (Name, Value) | |
Q_EMIT Q_EMIT | |
Q_ENUM (...) | |
Q_ENUM_NS (...) | |
Q_ENUMS (...) | |
Q_FLAG (...) | |
Q_FLAG_NS (...) | |
Q_FLAGS (...) | |
Q_GADGET Q_GADGET | |
Q_GADGET_EXPORT (EXPORT_MACRO) | |
Q_INTERFACES (...) | |
Q_INVOKABLE Q_INVOKABLE | |
Q_MOC_INCLUDE Q_MOC_INCLUDE | |
Q_NAMESPACE Q_NAMESPACE | |
Q_NAMESPACE_EXPORT (EXPORT_MACRO) | |
Q_OBJECT Q_OBJECT | |
Q_PROPERTY (...) | |
Q_REVISION Q_REVISION | |
Q_SET_OBJECT_NAME (Object) | |
Q_SIGNAL Q_SIGNAL | |
Q_SIGNALS Q_SIGNALS | |
Q_SLOT Q_SLOT | |
Q_SLOTS Q_SLOTS | |
T | qobject_cast (const QObject *object) |
T | qobject_cast (QObject *object) |
QT_NO_NARROWING_CONVERSIONS_IN_CONNECT QT_NO_NARROWING_CONVERSIONS_IN_CONNECT | |
void | removeEventFilter (QObject *obj) |
void | setObjectName (const QString &name) |
void | setObjectName (QAnyStringView name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | setProperty (const char *name, QVariant &&value) |
bool | signalsBlocked () const const |
int | startTimer (int interval, Qt::TimerType timerType) |
int | startTimer (std::chrono::milliseconds interval, Qt::TimerType timerType) |
QThread * | thread () const const |
Additional Inherited Members | |
![]() | |
typedef | QObjectList |
![]() | |
objectName | |
![]() | |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *context, Functor functor, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, Functor functor) |
bool | disconnect (const QMetaObject::Connection &connection) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
bool | disconnect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
![]() | |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const QMetaMethod &signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const QMetaMethod &signal) |
bool | isSignalConnected (const QMetaMethod &signal) const const |
int | receivers (const char *signal) const const |
QObject * | sender () const const |
int | senderSignalIndex () const const |
virtual void | timerEvent (QTimerEvent *event) |
Detailed Description
The Document class encapsulates a Krita Document/Image.
A Krita document is an Image with a filename. Libkis does not differentiate between a document and an image, like Krita does internally.
Definition at line 36 of file Document.h.
Constructor & Destructor Documentation
◆ Document()
|
explicit |
Definition at line 79 of file Document.cpp.
◆ ~Document()
|
override |
Definition at line 87 of file Document.cpp.
Member Function Documentation
◆ activeNode
|
slot |
activeNode retrieve the node that is currently active in the currently active window
- Returns
- the active node. If there is no active window, the first child node is returned.
Definition at line 118 of file Document.cpp.
◆ animationLength
|
slot |
get total frame range for animation
- Returns
- total frame range for animation
Definition at line 1051 of file Document.cpp.
◆ annotation
|
slot |
annotation the actual data for the annotation for this type.
It's a simple QByteArray, what's in it depends on the type of the annotation
- Parameters
-
type the type of the annotation
- Returns
- a bytearray, possibly empty if this type of annotation doesn't exist
Definition at line 1134 of file Document.cpp.
◆ annotationDescription
annotationDescription gets the pretty description for the current annotation
- Parameters
-
type the type of the annotation
- Returns
- a string that can be presented to the user
Definition at line 1127 of file Document.cpp.
◆ annotationTypes
|
slot |
annotationTypes returns the list of annotations present in the document.
Each annotation type is unique.
Definition at line 1100 of file Document.cpp.
◆ audioLevel
|
slot |
Return current audio level for document.
- Returns
- A value between 0.0 and 1.0 (1.0 = 100%)
Definition at line 1277 of file Document.cpp.
◆ audioTracks
Return a list of current audio tracks for document.
- Returns
- List of absolute path/file name of audio files
Definition at line 1287 of file Document.cpp.
◆ autosave
|
slot |
Return autosave status for document Notes:
- returned value is Autosave flag value Even if autosave is set to True, under condition Krita will not proceed to automatic save of document:
- autosave is globally deactivated
- document is read-only
- When autosave is set to False, Krita never execute automatic save for document
- Returns
- True if autosave is active, otherwise False
Definition at line 1165 of file Document.cpp.
◆ backgroundColor
|
slot |
backgroundColor returns the current background color of the document.
The color will also include the opacity.
- Returns
- QColor
Definition at line 236 of file Document.cpp.
◆ batchmode
|
slot |
Batchmode means that no actions on the document should show dialogs or popups.
- Returns
- true if the document is in batchmode.
Definition at line 106 of file Document.cpp.
◆ bounds
|
slot |
bounds return the bounds of the image
- Returns
- the bounds
Definition at line 967 of file Document.cpp.
◆ clone
|
slot |
clone create a shallow clone of this document.
- Returns
- a new Document that should be identical to this one in every respect.
We set ownsDocument to true, it will be reset automatically as soon as we create the first view for the document
Definition at line 891 of file Document.cpp.
◆ close
|
slot |
close Close the document: remove it from Krita's internal list of documents and close all views.
If the document is modified, you should save it first. There will be no prompt for saving.
After closing the document it becomes invalid.
- Returns
- true if the document is closed.
Definition at line 488 of file Document.cpp.
◆ colorDepth
|
slot |
colorDepth A string describing the color depth of the image:
- U8: unsigned 8 bits integer, the most common type
- U16: unsigned 16 bits integer
- F16: half, 16 bits floating point. Only available if Krita was built with OpenEXR
- F32: 32 bits floating point
- Returns
- the color depth.
Definition at line 192 of file Document.cpp.
◆ colorModel
|
slot |
colorModel retrieve the current color model of this document:
- A: Alpha mask
- RGBA: RGB with alpha channel (The actual order of channels is most often BGR!)
- XYZA: XYZ with alpha channel
- LABA: LAB with alpha channel
- CMYKA: CMYK with alpha channel
- GRAYA: Gray with alpha channel
- YCbCrA: YCbCr with alpha channel
- Returns
- the internal color model string.
Definition at line 198 of file Document.cpp.
◆ colorProfile
|
slot |
- Returns
- the name of the current color profile
Definition at line 204 of file Document.cpp.
◆ createCloneLayer
|
slot |
◆ createColorizeMask
|
slot |
createColorizeMask Creates a colorize mask, which can be used to color fill via keystrokes.
- Parameters
-
name - the name of the layer.
- Returns
- a TransparencyMask
Definition at line 792 of file Document.cpp.
◆ createFileLayer
|
slot |
createFileLayer returns a layer that shows an external image.
- Parameters
-
name name of the file layer. fileName the absolute filename of the file referenced. Symlinks will be resolved. scalingMethod how the dimensions of the file are interpreted can be either "None", "ImageToSize" or "ImageToPPI" scalingFilter filter used to scale the file can be "Bicubic", "Hermite", "NearestNeighbor", "Bilinear", "Bell", "BSpline", "Lanczos3", "Mitchell"
- Returns
- a FileLayer
Definition at line 672 of file Document.cpp.
◆ createFillLayer
|
slot |
createFillLayer creates a fill layer object, which is a layer
- Parameters
-
name generatorName - name of the generation filter. configuration - the configuration for the generation filter. selection - the selection.
- Returns
- a filllayer object.
Definition at line 690 of file Document.cpp.
◆ createFilterLayer
|
slot |
createFilterLayer creates a filter layer, which is a layer that represents a filter applied non-destructively.
- Parameters
-
name name of the filterLayer filter the filter that this filter layer will us. selection the selection.
- Returns
- a filter layer object.
Definition at line 681 of file Document.cpp.
◆ createFilterMask [1/2]
|
slot |
createFilterMask Creates a filter mask object that much like a filterlayer can apply a filter non-destructively.
- Parameters
-
name the name of the layer. filter the filter assigned. selection_source a node from which the selection should be initialized
- Returns
- a FilterMask
Definition at line 728 of file Document.cpp.
◆ createFilterMask [2/2]
|
slot |
createFilterMask Creates a filter mask object that much like a filterlayer can apply a filter non-destructively.
- Parameters
-
name the name of the layer. filter the filter assigned. selection the selection to be used by the filter mask
- Returns
- a FilterMask
Definition at line 750 of file Document.cpp.
◆ createGroupLayer
|
slot |
createGroupLayer Returns a grouplayer object.
Grouplayers are nodes that can have other layers as children and have the passthrough mode.
- Parameters
-
name the name of the layer.
- Returns
- a GroupLayer object.
Definition at line 663 of file Document.cpp.
◆ createNode
@brief createNode create a new node of the given type. The node is not added to the node hierarchy; you need to do that by finding the right parent node, getting its list of child nodes and adding the node in the right place, then calling Node::SetChildNodes @param name The name of the node @param nodeType The type of the node. Valid types are: <ul> <li>paintlayer <li>grouplayer <li>filelayer <li>filterlayer <li>filllayer <li>clonelayer <li>vectorlayer <li>transparencymask <li>filtermask <li>transformmask <li>selectionmask </ul> When relevant, the new Node will have the colorspace of the image by default; that can be changed with Node::setColorSpace. The settings and selections for relevant layer and mask types can also be set after the Node has been created.
- Returns
- the new Node.
Definition at line 615 of file Document.cpp.
◆ createSelectionMask
|
slot |
createSelectionMask Creates a selection mask, which can be used to store selections.
- Parameters
-
name - the name of the layer.
- Returns
- a SelectionMask
Definition at line 765 of file Document.cpp.
◆ createTransformMask
|
slot |
createTransformMask Creates a transform mask, which can be used to apply a transformation non-destructively.
- Parameters
-
name - the name of the layer mask.
- Returns
- a TransformMask
Definition at line 783 of file Document.cpp.
◆ createTransparencyMask
|
slot |
createTransparencyMask Creates a transparency mask, which can be used to assign transparency to regions.
- Parameters
-
name - the name of the layer.
- Returns
- a TransparencyMask
Definition at line 774 of file Document.cpp.
◆ createVectorLayer
|
slot |
createVectorLayer Creates a vector layer that can contain vector shapes.
- Parameters
-
name the name of this layer.
- Returns
- a VectorLayer.
Definition at line 719 of file Document.cpp.
◆ crop
|
slot |
crop the image to rectangle described by x
, y
, w
and h
- Parameters
-
x x coordinate of the top left corner y y coordinate of the top left corner w width h height
Definition at line 511 of file Document.cpp.
◆ currentTime
|
slot |
get current frame selected of animation
- Returns
- current frame selected of animation
Definition at line 1084 of file Document.cpp.
◆ documentInfo
|
slot |
documentInfo creates and XML document representing document and author information.
- Returns
- a string containing a valid XML document with the right information about the document and author. The DTD can be found here:
https://phabricator.kde.org/source/krita/browse/master/krita/dtd/
Definition at line 259 of file Document.cpp.
◆ exportImage
|
slot |
exportImage export the image, without changing its URL to the given path.
- Parameters
-
filename the full path to which the image is to be saved exportConfiguration a configuration object appropriate to the file format. An InfoObject will used to that configuration.
The supported formats have specific configurations that must be used when in batchmode. They are described below:
png
- alpha: bool (True or False)
- compression: int (1 to 9)
- forceSRGB: bool (True or False)
- indexed: bool (True or False)
- interlaced: bool (True or False)
- saveSRGBProfile: bool (True or False)
- transparencyFillcolor: rgb (Ex:[255,255,255])
jpeg
- baseline: bool (True or False)
- exif: bool (True or False)
- filters: bool (['ToolInfo', 'Anonymizer'])
- forceSRGB: bool (True or False)
- iptc: bool (True or False)
- is_sRGB: bool (True or False)
- optimize: bool (True or False)
- progressive: bool (True or False)
- quality: int (0 to 100)
- saveProfile: bool (True or False)
- smoothing: int (0 to 100)
- subsampling: int (0 to 3)
- transparencyFillcolor: rgb (Ex:[255,255,255])
- xmp: bool (True or False)
- Returns
- true if the export succeeded, false if it failed.
Definition at line 521 of file Document.cpp.
◆ fileName
|
slot |
- Returns
- the full path to the document, if it has been set.
Definition at line 277 of file Document.cpp.
◆ flatten
|
slot |
flatten all layers in the image
Definition at line 531 of file Document.cpp.
◆ framesPerSecond
|
slot |
frames per second of document
- Returns
- the fps of the document
Definition at line 1000 of file Document.cpp.
◆ fullClipRangeEndTime
|
slot |
get the full clip range end time
- Returns
- full clip range end time
Definition at line 1043 of file Document.cpp.
◆ fullClipRangeStartTime
|
slot |
get the full clip range start time
- Returns
- full clip range start time
Definition at line 1025 of file Document.cpp.
◆ gridConfig
|
slot |
Returns a GridConfig grid configuration for current document.
- Returns
- a GridConfig object with grid configuration
Definition at line 1263 of file Document.cpp.
◆ guidesConfig
|
slot |
Returns a GuidesConfig guides configuration for current document.
- Returns
- a GuidesConfig object with guides configuration
Definition at line 1170 of file Document.cpp.
◆ guidesLocked
|
slot |
DEPRECATED - use guidesConfig() instead Returns guide lockedness.
- Returns
- whether the guides are locked.
Definition at line 885 of file Document.cpp.
◆ guidesVisible
|
slot |
DEPRECATED - use guidesConfig() instead Returns guide visibility.
- Returns
- whether the guides are visible.
Definition at line 879 of file Document.cpp.
◆ height
|
slot |
- Returns
- the height of the image in pixels
Definition at line 291 of file Document.cpp.
◆ horizontalGuides
|
slot |
DEPRECATED - use guidesConfig() instead The horizontal guides.
- Returns
- a list of the horizontal positions of guides.
Definition at line 847 of file Document.cpp.
◆ importAnimation
Import an image sequence of files from a directory.
This will grab all images from the directory and import them with a potential offset (firstFrame) and step (images on 2s, 3s, etc)
- Returns
- whether the animation import was successful
Definition at line 985 of file Document.cpp.
◆ lock
|
slot |
[low-level] Lock the image without waiting for all the internal job queues are processed
WARNING: Don't use it unless you really know what you are doing! Use barrierLock() instead!
Waits for all the currently running internal jobs to complete and locks the image for writing. Please note that this function does not wait for all the internal queues to process, so there might be some non-finished actions pending. It means that you just postpone these actions until you unlock() the image back. Until then, then image might easily be frozen in some inconsistent state.
The only sane usage for this function is to lock the image for emergency processing, when some internal action or scheduler got hung up, and you just want to fetch some data from the image without races.
In all other cases, please use barrierLock() instead!
Definition at line 814 of file Document.cpp.
◆ modified
|
slot |
modified returns true if the document has unsaved modifications.
Definition at line 955 of file Document.cpp.
◆ name
|
slot |
- Returns
- the name of the document. This is the title field in the documentInfo
Definition at line 310 of file Document.cpp.
◆ nodeByName
nodeByName searches the node tree for a node with the given name and returns it
- Parameters
-
name the name of the node
- Returns
- the first node with the given name or 0 if no node is found
Definition at line 171 of file Document.cpp.
◆ nodeByUniqueID
nodeByUniqueID searches the node tree for a node with the given name and returns it.
- Parameters
-
uuid the unique id of the node
- Returns
- the node with the given unique id, or 0 if no node is found.
Definition at line 181 of file Document.cpp.
◆ operator!=()
bool Document::operator!= | ( | const Document & | other | ) | const |
Definition at line 101 of file Document.cpp.
◆ operator==()
bool Document::operator== | ( | const Document & | other | ) | const |
Definition at line 96 of file Document.cpp.
◆ pixelData
|
slot |
pixelData reads the given rectangle from the image projection and returns it as a byte array.
The pixel data starts top-left, and is ordered row-first.
The byte array can be interpreted as follows: 8 bits images have one byte per channel, and as many bytes as there are channels. 16 bits integer images have two bytes per channel, representing an unsigned short. 16 bits float images have two bytes per channel, representing a half, or 16 bits float. 32 bits float images have four bytes per channel, representing a float.
You can read outside the image boundaries; those pixels will be transparent black.
The order of channels is:
- Integer RGBA: Blue, Green, Red, Alpha
- Float RGBA: Red, Green, Blue, Alpha
- LabA: L, a, b, Alpha
- CMYKA: Cyan, Magenta, Yellow, Key, Alpha
- XYZA: X, Y, Z, A
- YCbCrA: Y, Cb, Cr, Alpha
The byte array is a copy of the original image data. In Python, you can use bytes, bytearray and the struct module to interpret the data and construct, for instance, a Pillow Image object.
- Parameters
-
x x position from where to start reading y y position from where to start reading w row length to read h number of rows to read
- Returns
- a QByteArray with the pixel data. The byte array may be empty.
Definition at line 474 of file Document.cpp.
◆ playBackEndTime
|
slot |
get end time of current playback
- Returns
- end time of current playback
Definition at line 1076 of file Document.cpp.
◆ playBackStartTime
|
slot |
get start time of current playback
- Returns
- start time of current playback
Definition at line 1068 of file Document.cpp.
◆ projection
|
slot |
projection creates a QImage from the rendered image or a cutout rectangle.
Definition at line 801 of file Document.cpp.
◆ refreshProjection
|
slot |
Starts a synchronous recomposition of the projection: everything will wait until the image is fully recomputed.
Definition at line 839 of file Document.cpp.
◆ removeAnnotation
|
slot |
removeAnnotation remove the specified annotation from the image
- Parameters
-
type the type defining the annotation
Definition at line 1154 of file Document.cpp.
◆ resizeImage
|
slot |
resizeImage resizes the canvas to the given left edge, top edge, width and height.
Note: This doesn't scale, use scale image for that.
- Parameters
-
x the new left edge y the new top edge w the new width h the new height
Definition at line 539 of file Document.cpp.
◆ resolution
|
slot |
- Returns
- the resolution in pixels per inch
Definition at line 323 of file Document.cpp.
◆ rootNode
|
slot |
rootNode the root node is the invisible group layer that contains the entire node hierarchy.
- Returns
- the root of the image
Definition at line 346 of file Document.cpp.
◆ rotateImage
|
slot |
rotateImage Rotate the image by the given radians.
- Parameters
-
radians the amount you wish to rotate the image in radians
Definition at line 570 of file Document.cpp.
◆ save
|
slot |
save the image to its currently set path.
The modified flag of the document will be reset
- Returns
- true if saving succeeded, false otherwise.
Definition at line 588 of file Document.cpp.
◆ saveAs
|
slot |
saveAs save the document under the filename
.
The document's filename will be reset to filename
.
- Parameters
-
filename the new filename (full path) for the document
- Returns
- true if saving succeeded, false otherwise.
Definition at line 599 of file Document.cpp.
◆ scaleImage
|
slot |
scaleImage
- Parameters
-
w the new width h the new height xres the new xres yres the new yres strategy the scaling strategy. There's several ones amongst these that aren't available in the regular UI. The list of filters is extensible and can be retrieved with Krita::filter - Hermite
- Bicubic - Adds pixels using the color of surrounding pixels. Produces smoother tonal gradations than Bilinear.
- Box - Replicate pixels in the image. Preserves all the original detail, but can produce jagged effects.
- Bilinear - Adds pixels averaging the color values of surrounding pixels. Produces medium quality results when the image is scaled from half to two times the original size.
- Bell
- BSpline
- Kanczos3 - Offers similar results than Bicubic, but maybe a little bit sharper. Can produce light and dark halos along strong edges.
- Mitchell
Definition at line 554 of file Document.cpp.
◆ selection
|
slot |
selection Create a Selection object around the global selection, if there is one.
- Returns
- the global selection or None if there is no global selection.
Definition at line 355 of file Document.cpp.
◆ setActiveNode
|
slot |
setActiveNode make the given node active in the currently active view and window
- Parameters
-
value the node to make active.
If we created any nodes via the same script, then dummies state may be not synchronized with the actual state of the nodes in the image. Hence, we should explicitly deliver the synchronized events before we try to manipulate with the GUI representation of nodes.
Definition at line 138 of file Document.cpp.
◆ setAnnotation
|
slot |
setAnnotation Add the given annotation to the document
- Parameters
-
type the unique type of the annotation description the user-visible description of the annotation annotation the annotation itself
Definition at line 1146 of file Document.cpp.
◆ setAudioLevel
|
slot |
Set current audio level for document.
- Parameters
-
level Audio volumne between 0.0 and 1.0 (1.0 = 100%)
Definition at line 1282 of file Document.cpp.
◆ setAudioTracks
Set a list of audio tracks for document Note: the function allows to add more than one file while from Krita's UI, importing a file will replace the complete list.
The reason why this method let the ability to provide more than one file is related to the internal's Krita method from KisDocument class: void KisDocument::setAudioTracks(QVector<QFileInfo> f)
- Parameters
-
files List of absolute path/file name of audio files
- Returns
- True if all files from list have been added, otherwise False (a file was not found)
Definition at line 1296 of file Document.cpp.
◆ setAutosave
|
slot |
Allow to activate/deactivate autosave for document When activated, it will use default Krita autosave settings It means that even when autosave is set to True, under condition Krita will not proceed to automatic save of document:
- autosave is globally deactivated
- document is read-only
Being able to deactivate autosave on a document can make sense when a plugin use internal document (document is not exposed in a view, only created for intenal process purposes)
- Parameters
-
active True to activate autosave
Definition at line 1160 of file Document.cpp.
◆ setBackgroundColor
|
slot |
setBackgroundColor sets the background color of the document.
It will trigger a projection update.
- Parameters
-
color A QColor. The color will be converted from sRGB.
- Returns
- bool
Definition at line 245 of file Document.cpp.
◆ setBatchmode
|
slot |
Set batchmode to value
.
If batchmode is true, then there should be no popups or dialogs shown to the user.
Definition at line 112 of file Document.cpp.
◆ setColorProfile
|
slot |
setColorProfile set the color profile of the image to the given profile.
The profile has to be registered with krita and be compatible with the current color model and depth; the image data is not converted.
- Parameters
-
colorProfile
- Returns
- false if the colorProfile name does not correspond to to a registered profile or if assigning the profile failed.
Definition at line 210 of file Document.cpp.
◆ setColorSpace
|
slot |
setColorSpace convert the nodes and the image to the given colorspace.
The conversion is done with Perceptual as intent, High Quality and No LCMS Optimizations as flags and no blackpoint compensation.
- Parameters
-
colorModel A string describing the color model of the image: - A: Alpha mask
- RGBA: RGB with alpha channel (The actual order of channels is most often BGR!)
- XYZA: XYZ with alpha channel
- LABA: LAB with alpha channel
- CMYKA: CMYK with alpha channel
- GRAYA: Gray with alpha channel
- YCbCrA: YCbCr with alpha channel
colorDepth A string describing the color depth of the image: - U8: unsigned 8 bits integer, the most common type
- U16: unsigned 16 bits integer
- F16: half, 16 bits floating point. Only available if Krita was built with OpenEXR
- F32: 32 bits floating point
colorProfile a valid color profile for this color model and color depth combination.
- Returns
- false the combination of these arguments does not correspond to a colorspace.
Definition at line 221 of file Document.cpp.
◆ setCurrentTime
|
slot |
set current time of document's animation
Definition at line 1092 of file Document.cpp.
◆ setDocumentInfo
|
slot |
setDocumentInfo set the Document information to the information contained in document
- Parameters
-
document A string containing a valid XML document that conforms to the document-info DTD that can be found here:
https://phabricator.kde.org/source/krita/browse/master/krita/dtd/
Definition at line 267 of file Document.cpp.
◆ setFileName
|
slot |
setFileName set the full path of the document to
- Parameters
-
value
Definition at line 283 of file Document.cpp.
◆ setFramesPerSecond
|
slot |
set frames per second of document
Definition at line 1008 of file Document.cpp.
◆ setFullClipRangeEndTime
|
slot |
set full clip range end time
Definition at line 1034 of file Document.cpp.
◆ setFullClipRangeStartTime
|
slot |
set start time of animation
Definition at line 1016 of file Document.cpp.
◆ setGridConfig
|
slot |
@brief Set grid configuration for current document @param gridConfig a GridConfig object to apply for grid configuration To modify/set grid property on a document
Definition at line 1270 of file Document.cpp.
◆ setGuidesConfig
|
slot |
@brief Set guides configuration for current document @param guidesConfig a GuidesConfig object to apply for guides configuration To modify/set guides property on a document
Definition at line 1225 of file Document.cpp.
◆ setGuidesLocked
|
slot |
DEPRECATED - use guidesConfig() instead set guides locked on this document.
- Parameters
-
locked whether or not to lock the guides on this document.
Definition at line 946 of file Document.cpp.
◆ setGuidesVisible
|
slot |
DEPRECATED - use guidesConfig() instead set guides visible on this document.
- Parameters
-
visible whether or not the guides are visible.
Definition at line 937 of file Document.cpp.
◆ setHeight
|
slot |
setHeight resize the document to
- Parameters
-
value height. This is a canvas resize, not a scale.
Definition at line 299 of file Document.cpp.
◆ setHorizontalGuides
|
slot |
DEPRECATED - use guidesConfig() instead replace all existing horizontal guides with the entries in the list.
- Parameters
-
lines a list of floats containing the new guides.
Definition at line 903 of file Document.cpp.
◆ setModified
|
slot |
setModified sets the modified status of the document
- Parameters
-
modified if true, the document is considered modified and closing it will ask for saving.
Definition at line 961 of file Document.cpp.
◆ setName
|
slot |
setName sets the name of the document to value
.
This is the title field in the documentInfo
Definition at line 316 of file Document.cpp.
◆ setPlayBackRange
|
slot |
set temporary playback range of document
Definition at line 1059 of file Document.cpp.
◆ setResolution
|
slot |
setResolution set the resolution of the image; this does not scale the image
- Parameters
-
value the resolution in pixels per inch
Definition at line 332 of file Document.cpp.
◆ setSelection
|
slot |
setSelection set or replace the global selection
- Parameters
-
value a valid selection object.
Definition at line 363 of file Document.cpp.
◆ setVerticalGuides
|
slot |
DEPRECATED - use guidesConfig() instead replace all existing horizontal guides with the entries in the list.
- Parameters
-
lines a list of floats containing the new guides.
Definition at line 920 of file Document.cpp.
◆ setWidth
|
slot |
setWidth resize the document to
- Parameters
-
value width. This is a canvas resize, not a scale.
Definition at line 384 of file Document.cpp.
◆ setXOffset
|
slot |
setXOffset sets the left edge of the canvas to x
.
Definition at line 403 of file Document.cpp.
◆ setXRes
|
slot |
setXRes set the horizontal resolution of the image to xRes in pixels per inch
Definition at line 440 of file Document.cpp.
◆ setYOffset
|
slot |
setYOffset sets the top edge of the canvas to y
.
Definition at line 422 of file Document.cpp.
◆ setYRes
|
slot |
setYRes set the vertical resolution of the image to yRes in pixels per inch
Definition at line 460 of file Document.cpp.
◆ shearImage
|
slot |
shearImage shear the whole image.
- Parameters
-
angleX the X-angle in degrees to shear by angleY the Y-angle in degrees to shear by
Definition at line 579 of file Document.cpp.
◆ thumbnail
|
slot |
thumbnail create a thumbnail of the given dimensions.
If the requested size is too big a null QImage is created.
- Returns
- a QImage representing the layer contents.
Definition at line 807 of file Document.cpp.
◆ topLevelNodes
toplevelNodes return a list with all top level nodes in the image graph
Definition at line 163 of file Document.cpp.
◆ tryBarrierLock
|
slot |
Tries to lock the image without waiting for the jobs to finish.
Same as barrierLock(), but doesn't block execution of the calling thread until all the background jobs are finished. Instead, in case of presence of unfinished jobs in the queue, it just returns false
- Returns
- whether the lock has been acquired
Definition at line 833 of file Document.cpp.
◆ unlock
|
slot |
Unlocks the image and starts/resumes all the pending internal jobs.
If the image has been locked for a non-readOnly access, then all the internal caches of the image (e.g. lod-planes) are reset and regeneration jobs are scheduled.
Definition at line 820 of file Document.cpp.
◆ verticalGuides
|
slot |
DEPRECATED - use guidesConfig() instead The vertical guide lines.
- Returns
- a list of vertical guides.
Definition at line 863 of file Document.cpp.
◆ waitForDone
|
slot |
Wait for all the internal image jobs to complete and return without locking the image.
This function is handy for tests or other synchronous actions, when one needs to wait for the result of his actions.
Definition at line 826 of file Document.cpp.
◆ width
|
slot |
- Returns
- the width of the image in pixels.
Definition at line 376 of file Document.cpp.
◆ xOffset
|
slot |
- Returns
- the left edge of the canvas in pixels.
Definition at line 395 of file Document.cpp.
◆ xRes
|
slot |
- Returns
- xRes the horizontal resolution of the image in pixels per inch
Definition at line 433 of file Document.cpp.
◆ yOffset
|
slot |
- Returns
- the top edge of the canvas in pixels.
Definition at line 414 of file Document.cpp.
◆ yRes
|
slot |
- Returns
- yRes the vertical resolution of the image in pixels per inch
Definition at line 453 of file Document.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2024 The KDE developers.
Generated on Mon Nov 18 2024 12:18:59 by doxygen 1.12.0 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.