ColorizeMask
#include <ColorizeMask.h>
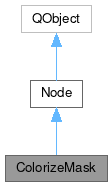
Public Slots | |
qreal | cleanUpAmount () const |
qreal | edgeDetectionSize () const |
bool | editKeyStrokes () const |
void | initializeKeyStrokeColors (QList< ManagedColor * > colors, int transparentIndex=-1) |
QByteArray | keyStrokePixelData (ManagedColor *color, int x, int y, int w, int h) const |
QList< ManagedColor * > | keyStrokesColors () const |
bool | limitToDeviceBounds () const |
void | removeKeyStroke (ManagedColor *color) |
void | resetCache () |
void | setCleanUpAmount (qreal value) |
void | setEdgeDetectionSize (qreal value) |
void | setEditKeyStrokes (bool enabled) |
bool | setKeyStrokePixelData (QByteArray value, ManagedColor *color, int x, int y, int w, int h) |
void | setLimitToDeviceBounds (bool value) |
void | setShowOutput (bool enabled) |
void | setUseEdgeDetection (bool value) |
bool | showOutput () const |
int | transparencyIndex () const |
virtual QString | type () const override |
void | updateMask (bool force=false) |
bool | useEdgeDetection () const |
![]() | |
bool | addChildNode (Node *child, Node *above) |
bool | alphaLocked () const |
bool | animated () const |
QString | blendingMode () const |
QRect | bounds () const |
QList< Channel * > | channels () const |
QList< Node * > | childNodes () const |
Node * | clone () const |
bool | collapsed () const |
QString | colorDepth () const |
int | colorLabel () const |
QString | colorModel () const |
QString | colorProfile () const |
void | cropNode (int x, int y, int w, int h) |
Node * | duplicate () |
void | enableAnimation () const |
QList< Node * > | findChildNodes (const QString &name=QString(), bool recursive=false, bool partialMatch=false, const QString &type=QString(), int colorLabelIndex=0) const |
bool | hasExtents () |
bool | hasKeyframeAtTime (int frameNumber) |
QIcon | icon () const |
int | index () const |
bool | inheritAlpha () const |
bool | isPinnedToTimeline () const |
QString | layerStyleToAsl () |
bool | locked () const |
Node * | mergeDown () |
void | move (int x, int y) |
QString | name () const |
int | opacity () const |
QString | paintAbility () |
void | paintEllipse (const QRectF &rect, const QString strokeStyle=PaintingResources::defaultStrokeStyle, const QString fillStyle=PaintingResources::defaultFillStyle) |
void | paintLine (const QPointF pointOne, const QPointF pointTwo, double pressureOne=1.0, double pressureTwo=1.0, const QString strokeStyle=PaintingResources::defaultStrokeStyle) |
void | paintPath (const QPainterPath &path, const QString strokeStyle=PaintingResources::defaultStrokeStyle, const QString fillStyle=PaintingResources::defaultFillStyle) |
void | paintPolygon (const QList< QPointF > points, const QString strokeStyle=PaintingResources::defaultStrokeStyle, const QString fillStyle=PaintingResources::defaultFillStyle) |
void | paintRectangle (const QRectF &rect, const QString strokeStyle=PaintingResources::defaultStrokeStyle, const QString fillStyle=PaintingResources::defaultFillStyle) |
Node * | parentNode () const |
QByteArray | pixelData (int x, int y, int w, int h) const |
QByteArray | pixelDataAtTime (int x, int y, int w, int h, int time) const |
QPoint | position () const |
QByteArray | projectionPixelData (int x, int y, int w, int h) const |
bool | remove () |
bool | removeChildNode (Node *child) |
void | rotateNode (double radians) |
bool | save (const QString &filename, double xRes, double yRes, const InfoObject &exportConfiguration, const QRect &exportRect=QRect()) |
void | scaleNode (QPointF origin, int width, int height, QString strategy) |
void | setAlphaLocked (bool value) |
void | setBlendingMode (QString value) |
void | setChildNodes (QList< Node * > nodes) |
void | setCollapsed (bool collapsed) |
void | setColorLabel (int index) |
bool | setColorProfile (const QString &colorProfile) |
bool | setColorSpace (const QString &colorModel, const QString &colorDepth, const QString &colorProfile) |
void | setInheritAlpha (bool value) |
bool | setLayerStyleFromAsl (const QString &asl) |
void | setLocked (bool value) |
void | setName (QString name) |
void | setOpacity (int value) |
void | setPinnedToTimeline (bool pinned) const |
bool | setPixelData (QByteArray value, int x, int y, int w, int h) |
void | setVisible (bool visible) |
void | shearNode (double angleX, double angleY) |
QImage | thumbnail (int w, int h) |
virtual QString | type () const |
QUuid | uniqueId () const |
bool | visible () const |
Public Member Functions | |
ColorizeMask (KisImageSP image, KisColorizeMaskSP mask, QObject *parent=0) | |
ColorizeMask (KisImageSP image, QString name, QObject *parent=0) | |
![]() | |
bool | operator!= (const Node &other) const |
bool | operator== (const Node &other) const |
![]() | |
QObject (QObject *parent) | |
QBindable< QString > | bindableObjectName () |
bool | blockSignals (bool block) |
const QObjectList & | children () const const |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) const const |
bool | disconnect (const QObject *receiver, const char *method) const const |
void | dumpObjectInfo () const const |
void | dumpObjectTree () const const |
QList< QByteArray > | dynamicPropertyNames () const const |
virtual bool | event (QEvent *e) |
virtual bool | eventFilter (QObject *watched, QEvent *event) |
T | findChild (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QRegularExpression &re, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (Qt::FindChildOptions options) const const |
bool | inherits (const char *className) const const |
void | installEventFilter (QObject *filterObj) |
bool | isQuickItemType () const const |
bool | isWidgetType () const const |
bool | isWindowType () const const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const const |
void | moveToThread (QThread *targetThread) |
QString | objectName () const const |
void | objectNameChanged (const QString &objectName) |
QObject * | parent () const const |
QVariant | property (const char *name) const const |
Q_CLASSINFO (Name, Value) | |
Q_EMIT Q_EMIT | |
Q_ENUM (...) | |
Q_ENUM_NS (...) | |
Q_ENUMS (...) | |
Q_FLAG (...) | |
Q_FLAG_NS (...) | |
Q_FLAGS (...) | |
Q_GADGET Q_GADGET | |
Q_GADGET_EXPORT (EXPORT_MACRO) | |
Q_INTERFACES (...) | |
Q_INVOKABLE Q_INVOKABLE | |
Q_MOC_INCLUDE Q_MOC_INCLUDE | |
Q_NAMESPACE Q_NAMESPACE | |
Q_NAMESPACE_EXPORT (EXPORT_MACRO) | |
Q_OBJECT Q_OBJECT | |
Q_PROPERTY (...) | |
Q_REVISION Q_REVISION | |
Q_SET_OBJECT_NAME (Object) | |
Q_SIGNAL Q_SIGNAL | |
Q_SIGNALS Q_SIGNALS | |
Q_SLOT Q_SLOT | |
Q_SLOTS Q_SLOTS | |
T | qobject_cast (const QObject *object) |
T | qobject_cast (QObject *object) |
QT_NO_NARROWING_CONVERSIONS_IN_CONNECT QT_NO_NARROWING_CONVERSIONS_IN_CONNECT | |
void | removeEventFilter (QObject *obj) |
void | setObjectName (const QString &name) |
void | setObjectName (QAnyStringView name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | setProperty (const char *name, QVariant &&value) |
bool | signalsBlocked () const const |
int | startTimer (int interval, Qt::TimerType timerType) |
int | startTimer (std::chrono::milliseconds interval, Qt::TimerType timerType) |
QThread * | thread () const const |
Additional Inherited Members | |
![]() | |
typedef | QObjectList |
![]() | |
objectName | |
![]() | |
static Node * | createNode (KisImageSP image, KisNodeSP node, QObject *parent=0) |
![]() | |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *context, Functor functor, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, Functor functor) |
bool | disconnect (const QMetaObject::Connection &connection) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
bool | disconnect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
![]() | |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const QMetaMethod &signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const QMetaMethod &signal) |
bool | isSignalConnected (const QMetaMethod &signal) const const |
int | receivers (const char *signal) const const |
QObject * | sender () const const |
int | senderSignalIndex () const const |
virtual void | timerEvent (QTimerEvent *event) |
Detailed Description
The ColorizeMask class A colorize mask is a mask type node that can be used to color in line art.
Definition at line 59 of file ColorizeMask.h.
Constructor & Destructor Documentation
◆ ColorizeMask() [1/2]
Definition at line 21 of file ColorizeMask.cpp.
◆ ColorizeMask() [2/2]
|
explicit |
Definition at line 27 of file ColorizeMask.cpp.
◆ ~ColorizeMask()
|
override |
Definition at line 32 of file ColorizeMask.cpp.
Member Function Documentation
◆ cleanUpAmount
|
slot |
cleanUpAmount
- Returns
- a float value of 0.0 to 100.0 representing the cleanup amount where 0.0 is no cleanup is done and 100.00 is most aggressive.
Definition at line 195 of file ColorizeMask.cpp.
◆ edgeDetectionSize
|
slot |
edgeDetectionSize
- Returns
- a float value of the edge detection size in pixels.
Definition at line 179 of file ColorizeMask.cpp.
◆ editKeyStrokes
|
slot |
editKeyStrokes Edit keystrokes mode allows the user to modify keystrokes on the active Colorize Mask.
- Returns
- true if edit keystrokes mode is enabled, false if disabled.
Definition at line 271 of file ColorizeMask.cpp.
◆ initializeKeyStrokeColors
|
slot |
initializeKeyStrokeColors Set the colors to use for the Colorize Mask's keystrokes.
- Parameters
-
colors a list of ManagedColor to use for the keystrokes. transparentIndex index of the color that should be marked as transparent.
This method is supposed to to initial initialization only!
It is necessary because the function also changes the color space and blending mode of the mask
TODO: implement a proper API that modifies key strokes of a colorize mask without breaking undo history
Definition at line 51 of file ColorizeMask.cpp.
◆ keyStrokePixelData
|
slot |
keyStrokePixelData reads the given rectangle from the keystroke image data and returns it as a byte array.
The pixel data starts top-left, and is ordered row-first.
- Parameters
-
color a ManagedColor to get keystrokes pixeldata from. x x position from where to start reading y y position from where to start reading w row length to read h number of rows to read
- Returns
- a QByteArray with the pixel data. The byte array may be empty.
Definition at line 105 of file ColorizeMask.cpp.
◆ keyStrokesColors
|
slot |
keyStrokesColors Colors used in the Colorize Mask's keystrokes.
- Returns
- a ManagedColor list containing the colors of keystrokes.
Definition at line 38 of file ColorizeMask.cpp.
◆ limitToDeviceBounds
|
slot |
limitToDeviceBounds
- Returns
- true if limit bounds is enabled, false if disabled.
Definition at line 211 of file ColorizeMask.cpp.
◆ removeKeyStroke
|
slot |
removeKeyStroke Remove a color from the Colorize Mask's keystrokes.
- Parameters
-
color a ManagedColor to be removed from the keystrokes.
Definition at line 96 of file ColorizeMask.cpp.
◆ resetCache
|
slot |
Definition at line 231 of file ColorizeMask.cpp.
◆ setCleanUpAmount
|
slot |
setCleanUpAmount This will attempt to handle messy strokes that overlap the line art where they shouldn't.
- Parameters
-
value a float value from 0.0 to 100.00 where 0.0 is no cleanup is done and 100.00 is most aggressive.
Definition at line 187 of file ColorizeMask.cpp.
◆ setEdgeDetectionSize
|
slot |
setEdgeDetectionSize Set the value to the thinnest line on the image.
- Parameters
-
value a float value of the edge size to detect in pixels.
Definition at line 171 of file ColorizeMask.cpp.
◆ setEditKeyStrokes
|
slot |
setEditKeyStrokes Toggle Colorize Mask's edit keystrokes mode.
- Parameters
-
enabled set true to enable edit keystrokes mode and false to disable it.
Definition at line 263 of file ColorizeMask.cpp.
◆ setKeyStrokePixelData
|
slot |
setKeyStrokePixelData writes the given bytes, of which there must be enough, into the keystroke, the keystroke's original pixels are overwritten
- Parameters
-
value the byte array representing the pixels. There must be enough bytes available. Krita will take the raw pointer from the QByteArray and start reading, not stopping before (number of channels * size of channel * w * h) bytes are read. color a ManagedColor to set keystrokes pixeldata for. x the x position to start writing from y the y position to start writing from w the width of each row h the number of rows to write
- Returns
- true if writing the pixeldata worked
Definition at line 130 of file ColorizeMask.cpp.
◆ setLimitToDeviceBounds
|
slot |
setLimitToDeviceBounds Limit the colorize mask to the combined layer bounds of the strokes and the line art it is filling.
This can speed up the use of the mask on complicated compositions, such as comic pages.
- Parameters
-
value set true to enabled limit bounds, false to disable.
Definition at line 203 of file ColorizeMask.cpp.
◆ setShowOutput
|
slot |
setShowOutput Toggle Colorize Mask's show output mode.
- Parameters
-
enabled set true to enable show coloring mode and false to disable it.
Definition at line 239 of file ColorizeMask.cpp.
◆ setUseEdgeDetection
|
slot |
setUseEdgeDetection Activate this for line art with large solid areas, for example shadows on an object.
- Parameters
-
value true to enable edge detection, false to disable.
Definition at line 155 of file ColorizeMask.cpp.
◆ showOutput
|
slot |
showOutput Show output mode allows the user to see the result of the Colorize Mask's algorithm.
- Returns
- true if edit show coloring mode is enabled, false if disabled.
Definition at line 247 of file ColorizeMask.cpp.
◆ transparencyIndex
|
slot |
transparencyIndex Index of the transparent color.
- Returns
- an integer containing the index of the current color marked as transparent.
Definition at line 88 of file ColorizeMask.cpp.
◆ type
|
overridevirtualslot |
type Krita has several types of nodes, split in layers and masks.
Group layers can contain other layers, any layer can contain masks.
- Returns
- colorizemask
If the Node object isn't wrapping a valid Krita layer or mask object, and empty string is returned.
Definition at line 287 of file ColorizeMask.cpp.
◆ updateMask
|
slot |
updateMask Process the Colorize Mask's keystrokes and generate a projection of the computed colors.
- Parameters
-
force force an update
Definition at line 219 of file ColorizeMask.cpp.
◆ useEdgeDetection
|
slot |
useEdgeDetection
- Returns
- true if Edge detection is enabled, false if disabled.
Definition at line 163 of file ColorizeMask.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2024 The KDE developers.
Generated on Mon Nov 18 2024 12:18:59 by doxygen 1.12.0 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.