Node
#include <Node.h>
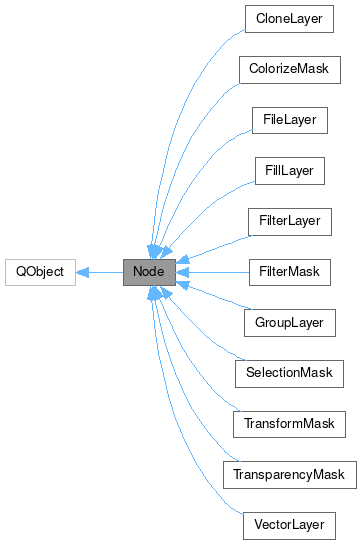
Public Slots | |
bool | addChildNode (Node *child, Node *above) |
bool | alphaLocked () const |
bool | animated () const |
QString | blendingMode () const |
QRect | bounds () const |
QList< Channel * > | channels () const |
QList< Node * > | childNodes () const |
Node * | clone () const |
bool | collapsed () const |
QString | colorDepth () const |
int | colorLabel () const |
QString | colorModel () const |
QString | colorProfile () const |
void | cropNode (int x, int y, int w, int h) |
Node * | duplicate () |
void | enableAnimation () const |
QList< Node * > | findChildNodes (const QString &name=QString(), bool recursive=false, bool partialMatch=false, const QString &type=QString(), int colorLabelIndex=0) const |
bool | hasExtents () |
bool | hasKeyframeAtTime (int frameNumber) |
QIcon | icon () const |
int | index () const |
bool | inheritAlpha () const |
bool | isPinnedToTimeline () const |
QString | layerStyleToAsl () |
bool | locked () const |
Node * | mergeDown () |
void | move (int x, int y) |
QString | name () const |
int | opacity () const |
QString | paintAbility () |
void | paintEllipse (const QRectF &rect, const QString strokeStyle=PaintingResources::defaultStrokeStyle, const QString fillStyle=PaintingResources::defaultFillStyle) |
void | paintLine (const QPointF pointOne, const QPointF pointTwo, double pressureOne=1.0, double pressureTwo=1.0, const QString strokeStyle=PaintingResources::defaultStrokeStyle) |
void | paintPath (const QPainterPath &path, const QString strokeStyle=PaintingResources::defaultStrokeStyle, const QString fillStyle=PaintingResources::defaultFillStyle) |
void | paintPolygon (const QList< QPointF > points, const QString strokeStyle=PaintingResources::defaultStrokeStyle, const QString fillStyle=PaintingResources::defaultFillStyle) |
void | paintRectangle (const QRectF &rect, const QString strokeStyle=PaintingResources::defaultStrokeStyle, const QString fillStyle=PaintingResources::defaultFillStyle) |
Node * | parentNode () const |
QByteArray | pixelData (int x, int y, int w, int h) const |
QByteArray | pixelDataAtTime (int x, int y, int w, int h, int time) const |
QPoint | position () const |
QByteArray | projectionPixelData (int x, int y, int w, int h) const |
bool | remove () |
bool | removeChildNode (Node *child) |
void | rotateNode (double radians) |
bool | save (const QString &filename, double xRes, double yRes, const InfoObject &exportConfiguration, const QRect &exportRect=QRect()) |
void | scaleNode (QPointF origin, int width, int height, QString strategy) |
void | setAlphaLocked (bool value) |
void | setBlendingMode (QString value) |
void | setChildNodes (QList< Node * > nodes) |
void | setCollapsed (bool collapsed) |
void | setColorLabel (int index) |
bool | setColorProfile (const QString &colorProfile) |
bool | setColorSpace (const QString &colorModel, const QString &colorDepth, const QString &colorProfile) |
void | setInheritAlpha (bool value) |
bool | setLayerStyleFromAsl (const QString &asl) |
void | setLocked (bool value) |
void | setName (QString name) |
void | setOpacity (int value) |
void | setPinnedToTimeline (bool pinned) const |
bool | setPixelData (QByteArray value, int x, int y, int w, int h) |
void | setVisible (bool visible) |
void | shearNode (double angleX, double angleY) |
QImage | thumbnail (int w, int h) |
virtual QString | type () const |
QUuid | uniqueId () const |
bool | visible () const |
Public Member Functions | |
bool | operator!= (const Node &other) const |
bool | operator== (const Node &other) const |
![]() | |
QObject (QObject *parent) | |
QBindable< QString > | bindableObjectName () |
bool | blockSignals (bool block) |
const QObjectList & | children () const const |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) const const |
bool | disconnect (const QObject *receiver, const char *method) const const |
void | dumpObjectInfo () const const |
void | dumpObjectTree () const const |
QList< QByteArray > | dynamicPropertyNames () const const |
virtual bool | event (QEvent *e) |
virtual bool | eventFilter (QObject *watched, QEvent *event) |
T | findChild (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QRegularExpression &re, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (Qt::FindChildOptions options) const const |
bool | inherits (const char *className) const const |
void | installEventFilter (QObject *filterObj) |
bool | isQuickItemType () const const |
bool | isWidgetType () const const |
bool | isWindowType () const const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const const |
void | moveToThread (QThread *targetThread) |
QString | objectName () const const |
void | objectNameChanged (const QString &objectName) |
QObject * | parent () const const |
QVariant | property (const char *name) const const |
Q_CLASSINFO (Name, Value) | |
Q_EMIT Q_EMIT | |
Q_ENUM (...) | |
Q_ENUM_NS (...) | |
Q_ENUMS (...) | |
Q_FLAG (...) | |
Q_FLAG_NS (...) | |
Q_FLAGS (...) | |
Q_GADGET Q_GADGET | |
Q_GADGET_EXPORT (EXPORT_MACRO) | |
Q_INTERFACES (...) | |
Q_INVOKABLE Q_INVOKABLE | |
Q_MOC_INCLUDE Q_MOC_INCLUDE | |
Q_NAMESPACE Q_NAMESPACE | |
Q_NAMESPACE_EXPORT (EXPORT_MACRO) | |
Q_OBJECT Q_OBJECT | |
Q_PROPERTY (...) | |
Q_REVISION Q_REVISION | |
Q_SET_OBJECT_NAME (Object) | |
Q_SIGNAL Q_SIGNAL | |
Q_SIGNALS Q_SIGNALS | |
Q_SLOT Q_SLOT | |
Q_SLOTS Q_SLOTS | |
T | qobject_cast (const QObject *object) |
T | qobject_cast (QObject *object) |
QT_NO_NARROWING_CONVERSIONS_IN_CONNECT QT_NO_NARROWING_CONVERSIONS_IN_CONNECT | |
void | removeEventFilter (QObject *obj) |
void | setObjectName (const QString &name) |
void | setObjectName (QAnyStringView name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | setProperty (const char *name, QVariant &&value) |
bool | signalsBlocked () const const |
int | startTimer (int interval, Qt::TimerType timerType) |
int | startTimer (std::chrono::milliseconds interval, Qt::TimerType timerType) |
QThread * | thread () const const |
Static Public Member Functions | |
static Node * | createNode (KisImageSP image, KisNodeSP node, QObject *parent=0) |
![]() | |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *context, Functor functor, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, Functor functor) |
bool | disconnect (const QMetaObject::Connection &connection) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
bool | disconnect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
Additional Inherited Members | |
![]() | |
typedef | QObjectList |
![]() | |
objectName | |
![]() | |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const QMetaMethod &signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const QMetaMethod &signal) |
bool | isSignalConnected (const QMetaMethod &signal) const const |
int | receivers (const char *signal) const const |
QObject * | sender () const const |
int | senderSignalIndex () const const |
virtual void | timerEvent (QTimerEvent *event) |
Detailed Description
Node represents a layer or mask in a Krita image's Node hierarchy.
Group layers can contain other layers and masks; layers can contain masks.
Constructor & Destructor Documentation
◆ ~Node()
Member Function Documentation
◆ addChildNode
◆ alphaLocked
|
slot |
◆ animated
|
slot |
◆ blendingMode
|
slot |
◆ bounds
|
slot |
◆ channels
channels creates a list of Channel objects that can be used individually to show or hide certain channels, and to retrieve the contents of each channel in a node separately.
Only layers have channels, masks do not, and calling channels on a Node that is a mask will return an empty list.
- Returns
- the list of channels ordered in by position of the channels in pixel position
◆ childNodes
◆ clone
|
slot |
◆ collapsed
|
slot |
◆ colorDepth
|
slot |
colorDepth A string describing the color depth of the image:
- U8: unsigned 8 bits integer, the most common type
- U16: unsigned 16 bits integer
- F16: half, 16 bits floating point. Only available if Krita was built with OpenEXR
- F32: 32 bits floating point
- Returns
- the color depth.
◆ colorLabel
|
slot |
◆ colorModel
|
slot |
colorModel retrieve the current color model of this document:
- A: Alpha mask
- RGBA: RGB with alpha channel (The actual order of channels is most often BGR!)
- XYZA: XYZ with alpha channel
- LABA: LAB with alpha channel
- CMYKA: CMYK with alpha channel
- GRAYA: Gray with alpha channel
- YCbCrA: YCbCr with alpha channel
- Returns
- the internal color model string.
◆ colorProfile
|
slot |
◆ createNode()
◆ cropNode
|
slot |
◆ duplicate
|
slot |
◆ enableAnimation
|
slot |
◆ findChildNodes
|
slot |
findChildNodes
- Parameters
-
name name of the child node to search for. Leaving this blank will return all nodes. recursive whether or not to search recursively. Defaults to false. partialMatch return if the name partially contains the string (case insensitive). Defaults to false. type filter returned nodes based on type colorLabelIndex filter returned nodes based on color label index
- Returns
- returns a list of child nodes and grand child nodes of the current node that match the search criteria.
◆ hasExtents
|
slot |
◆ hasKeyframeAtTime
|
slot |
◆ icon
|
slot |
◆ index
|
slot |
◆ inheritAlpha
|
slot |
◆ isPinnedToTimeline
|
slot |
◆ layerStyleToAsl
|
slot |
◆ locked
|
slot |
◆ mergeDown
|
slot |
◆ move
|
slot |
◆ name
|
slot |
◆ opacity
|
slot |
◆ operator!=()
◆ operator==()
◆ paintAbility
|
slot |
paintAbility can be used to determine whether this node can be painted on with the current brush preset.
- Returns
- QString, one of the following:
- VECTOR - This node is vector-based.
- CLONE - This node is a Clone Layer.
- PAINT - This node is paintable by the current brush preset.
- UNPAINTABLE - This node is not paintable, or a null preset is somehow selected./li>
- MYPAINTBRUSH_UNPAINTABLE - This node's non-RGBA colorspace cannot be painted on by the currently selected MyPaint brush.
◆ paintEllipse
|
slot |
paint an ellipse on the canvas.
Uses current brush preset
- Parameters
-
rect QRect with x, y, width, and height - None
- ForegroundColor
- BackgroundColor
fillStyle appearance of the fill, one of: - None
- ForegroundColor
- BackgroundColor
- Pattern
◆ paintLine
|
slot |
paint a line on the canvas.
Uses current brush preset
- Parameters
-
pointOne starting point pointTwo end point pressureOne starting pressure pressureTwo end pressure strokeStyle appearance of the outline, one of: - None - will use Foreground Color, since line would be invisible otherwise
- ForegroundColor
- BackgroundColor
◆ paintPath
|
slot |
paint a custom path on the canvas.
Uses current brush preset
- Parameters
-
path QPainterPath to determine path - None
- ForegroundColor
- BackgroundColor
fillStyle appearance of the fill, one of: - None
- ForegroundColor
- BackgroundColor
- Pattern
◆ paintPolygon
◆ paintRectangle
|
slot |
paint a rectangle on the canvas.
Uses current brush preset
- Parameters
-
rect QRect with x, y, width, and height strokeStyle appearance of the outline, one of: - None
- ForegroundColor
- BackgroundColor
fillStyle appearance of the fill, one of: - None
- ForegroundColor
- BackgroundColor
- Pattern
◆ parentNode
|
slot |
◆ pixelData
|
slot |
pixelData reads the given rectangle from the Node's paintable pixels, if those exist, and returns it as a byte array.
The pixel data starts top-left, and is ordered row-first.
The byte array can be interpreted as follows: 8 bits images have one byte per channel, and as many bytes as there are channels. 16 bits integer images have two bytes per channel, representing an unsigned short. 16 bits float images have two bytes per channel, representing a half, or 16 bits float. 32 bits float images have four bytes per channel, representing a float.
You can read outside the node boundaries; those pixels will be transparent black.
The order of channels is:
- Integer RGBA: Blue, Green, Red, Alpha
- Float RGBA: Red, Green, Blue, Alpha
- GrayA: Gray, Alpha
- Selection: selectedness
- LabA: L, a, b, Alpha
- CMYKA: Cyan, Magenta, Yellow, Key, Alpha
- XYZA: X, Y, Z, A
- YCbCrA: Y, Cb, Cr, Alpha
The byte array is a copy of the original node data. In Python, you can use bytes, bytearray and the struct module to interpret the data and construct, for instance, a Pillow Image object.
If you read the pixeldata of a mask, a filter or generator layer, you get the selection bytes, which is one channel with values in the range from 0..255.
If you want to change the pixels of a node you can write the pixels back after manipulation with setPixelData(). This will only succeed on nodes with writable pixel data, e.g not on groups or file layers.
- Parameters
-
x x position from where to start reading y y position from where to start reading w row length to read h number of rows to read
- Returns
- a QByteArray with the pixel data. The byte array may be empty.
◆ pixelDataAtTime
|
slot |
pixelDataAtTime a basic function to get pixeldata from an animated node at a given time.
- Parameters
-
x the position from the left to start reading. y the position from the top to start reader w the row length to read h the number of rows to read time the frame number
- Returns
- a QByteArray with the pixel data. The byte array may be empty.
◆ position
|
slot |
position returns the position of the paint device of this node.
The position is always 0,0 unless the layer has been moved. If you want to know the topleft position of the rectangle around the actual non-transparent pixels in the node, use bounds().
- Returns
- the top-left position of the node
◆ projectionPixelData
|
slot |
projectionPixelData reads the given rectangle from the Node's projection (that is, what the node looks like after all sub-Nodes (like layers in a group or masks on a layer) have been applied, and returns it as a byte array.
The pixel data starts top-left, and is ordered row-first.
The byte array can be interpreted as follows: 8 bits images have one byte per channel, and as many bytes as there are channels. 16 bits integer images have two bytes per channel, representing an unsigned short. 16 bits float images have two bytes per channel, representing a half, or 16 bits float. 32 bits float images have four bytes per channel, representing a float.
You can read outside the node boundaries; those pixels will be transparent black.
The order of channels is:
- Integer RGBA: Blue, Green, Red, Alpha
- Float RGBA: Red, Green, Blue, Alpha
- GrayA: Gray, Alpha
- Selection: selectedness
- LabA: L, a, b, Alpha
- CMYKA: Cyan, Magenta, Yellow, Key, Alpha
- XYZA: X, Y, Z, A
- YCbCrA: Y, Cb, Cr, Alpha
The byte array is a copy of the original node data. In Python, you can use bytes, bytearray and the struct module to interpret the data and construct, for instance, a Pillow Image object.
If you read the projection of a mask, you get the selection bytes, which is one channel with values in the range from 0..255.
If you want to change the pixels of a node you can write the pixels back after manipulation with setPixelData(). This will only succeed on nodes with writable pixel data, e.g not on groups or file layers.
- Parameters
-
x x position from where to start reading y y position from where to start reading w row length to read h number of rows to read
- Returns
- a QByteArray with the pixel data. The byte array may be empty.
◆ remove
|
slot |
◆ removeChildNode
|
slot |
◆ rotateNode
|
slot |
◆ save
|
slot |
save exports the given node with this filename.
The extension of the filename determines the filetype.
- Parameters
-
filename the filename including extension xRes the horizontal resolution in pixels per pt (there are 72 pts in an inch) yRes the horizontal resolution in pixels per pt (there are 72 pts in an inch) exportConfiguration a configuration object appropriate to the file format. exportRect the export bounds for saving a node as a QRect If exportRect
is empty, then save exactBounds() of the node. If you'd like to save the image- aligned area of the node, just pass image->bounds() there. See Document->exportImage for InfoObject details.
- Returns
- true if saving succeeded, false if it failed.
◆ scaleNode
scaleNode
- Parameters
-
origin the origin point width the width height the height strategy the scaling strategy. There's several ones amongst these that aren't available in the regular UI. - Hermite
- Bicubic - Adds pixels using the color of surrounding pixels. Produces smoother tonal gradations than Bilinear.
- Box - Replicate pixels in the image. Preserves all the original detail, but can produce jagged effects.
- Bilinear - Adds pixels averaging the color values of surrounding pixels. Produces medium quality results when the image is scaled from half to two times the original size.
- Bell
- BSpline
- Lanczos3 - Offers similar results than Bicubic, but maybe a little bit sharper. Can produce light and dark halos along strong edges.
- Mitchell
◆ setAlphaLocked
|
slot |
◆ setBlendingMode
|
slot |
◆ setChildNodes
◆ setCollapsed
|
slot |
◆ setColorLabel
|
slot |
◆ setColorProfile
|
slot |
setColorProfile set the color profile of the image to the given profile.
The profile has to be registered with krita and be compatible with the current color model and depth; the image data is not converted.
- Parameters
-
colorProfile
- Returns
- if assigning the color profile worked
◆ setColorSpace
|
slot |
setColorSpace convert the node to the given colorspace
- Parameters
-
colorModel A string describing the color model of the node: - A: Alpha mask
- RGBA: RGB with alpha channel (The actual order of channels is most often BGR!)
- XYZA: XYZ with alpha channel
- LABA: LAB with alpha channel
- CMYKA: CMYK with alpha channel
- GRAYA: Gray with alpha channel
- YCbCrA: YCbCr with alpha channel
colorDepth A string describing the color depth of the image: - U8: unsigned 8 bits integer, the most common type
- U16: unsigned 16 bits integer
- F16: half, 16 bits floating point. Only available if Krita was built with OpenEXR
- F32: 32 bits floating point
colorProfile a valid color profile for this color model and color depth combination.
◆ setInheritAlpha
|
slot |
◆ setLayerStyleFromAsl
|
slot |
◆ setLocked
|
slot |
◆ setName
|
slot |
◆ setOpacity
|
slot |
◆ setPinnedToTimeline
|
slot |
◆ setPixelData
|
slot |
setPixelData writes the given bytes, of which there must be enough, into the Node, if the Node has writable pixel data:
- paint layer: the layer's original pixels are overwritten
- filter layer, generator layer, any mask: the embedded selection's pixels are overwritten. Note: for these
File layers, Group layers, Clone layers cannot be written to. Calling setPixelData on those layer types will silently do nothing.
- Parameters
-
value the byte array representing the pixels. There must be enough bytes available. Krita will take the raw pointer from the QByteArray and start reading, not stopping before (number of channels * size of channel * w * h) bytes are read. x the x position to start writing from y the y position to start writing from w the width of each row h the number of rows to write
- Returns
- true if writing the pixeldata worked
◆ setVisible
|
slot |
◆ shearNode
|
slot |
◆ thumbnail
|
slot |
thumbnail create a thumbnail of the given dimensions.
The thumbnail is sized according to the layer dimensions, not the image dimensions. If the requested size is too big a null QImage is created. If the current node cannot generate a thumbnail, a transparent QImage of the requested size is generated.
- Returns
- a QImage representing the layer contents.
◆ type
|
virtualslot |
type Krita has several types of nodes, split in layers and masks.
Group layers can contain other layers, any layer can contain masks.
- Returns
- The type of the node. Valid types are:
- paintlayer
- grouplayer
- filelayer
- filterlayer
- filllayer
- clonelayer
- vectorlayer
- transparencymask
- filtermask
- transformmask
- selectionmask
- colorizemask
If the Node object isn't wrapping a valid Krita layer or mask object, and empty string is returned.
◆ uniqueId
|
slot |
◆ visible
|
slot |
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2024 The KDE developers.
Generated on Mon Nov 18 2024 12:18:59 by doxygen 1.12.0 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.