Channel
#include <Channel.h>
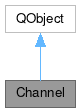
Public Member Functions | |
Channel (KisNodeSP node, KoChannelInfo *channel, QObject *parent=0) | |
QRect | bounds () const |
int | channelSize () const |
QString | name () const |
bool | operator!= (const Channel &other) const |
bool | operator== (const Channel &other) const |
QByteArray | pixelData (const QRect &rect) const |
int | position () const |
void | setPixelData (QByteArray value, const QRect &rect) |
void | setVisible (bool value) |
bool | visible () const |
![]() | |
QObject (QObject *parent) | |
QBindable< QString > | bindableObjectName () |
bool | blockSignals (bool block) |
const QObjectList & | children () const const |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) const const |
bool | disconnect (const QObject *receiver, const char *method) const const |
void | dumpObjectInfo () const const |
void | dumpObjectTree () const const |
QList< QByteArray > | dynamicPropertyNames () const const |
virtual bool | event (QEvent *e) |
virtual bool | eventFilter (QObject *watched, QEvent *event) |
T | findChild (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QRegularExpression &re, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (Qt::FindChildOptions options) const const |
bool | inherits (const char *className) const const |
void | installEventFilter (QObject *filterObj) |
bool | isQuickItemType () const const |
bool | isWidgetType () const const |
bool | isWindowType () const const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const const |
void | moveToThread (QThread *targetThread) |
QString | objectName () const const |
void | objectNameChanged (const QString &objectName) |
QObject * | parent () const const |
QVariant | property (const char *name) const const |
Q_CLASSINFO (Name, Value) | |
Q_EMIT Q_EMIT | |
Q_ENUM (...) | |
Q_ENUM_NS (...) | |
Q_ENUMS (...) | |
Q_FLAG (...) | |
Q_FLAG_NS (...) | |
Q_FLAGS (...) | |
Q_GADGET Q_GADGET | |
Q_GADGET_EXPORT (EXPORT_MACRO) | |
Q_INTERFACES (...) | |
Q_INVOKABLE Q_INVOKABLE | |
Q_MOC_INCLUDE Q_MOC_INCLUDE | |
Q_NAMESPACE Q_NAMESPACE | |
Q_NAMESPACE_EXPORT (EXPORT_MACRO) | |
Q_OBJECT Q_OBJECT | |
Q_PROPERTY (...) | |
Q_REVISION Q_REVISION | |
Q_SET_OBJECT_NAME (Object) | |
Q_SIGNAL Q_SIGNAL | |
Q_SIGNALS Q_SIGNALS | |
Q_SLOT Q_SLOT | |
Q_SLOTS Q_SLOTS | |
T | qobject_cast (const QObject *object) |
T | qobject_cast (QObject *object) |
QT_NO_NARROWING_CONVERSIONS_IN_CONNECT QT_NO_NARROWING_CONVERSIONS_IN_CONNECT | |
void | removeEventFilter (QObject *obj) |
void | setObjectName (const QString &name) |
void | setObjectName (QAnyStringView name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | setProperty (const char *name, QVariant &&value) |
bool | signalsBlocked () const const |
int | startTimer (int interval, Qt::TimerType timerType) |
int | startTimer (std::chrono::milliseconds interval, Qt::TimerType timerType) |
QThread * | thread () const const |
Additional Inherited Members | |
![]() | |
typedef | QObjectList |
![]() | |
objectName | |
![]() | |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *context, Functor functor, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, Functor functor) |
bool | disconnect (const QMetaObject::Connection &connection) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
bool | disconnect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
![]() | |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const QMetaMethod &signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const QMetaMethod &signal) |
bool | isSignalConnected (const QMetaMethod &signal) const const |
int | receivers (const char *signal) const const |
QObject * | sender () const const |
int | senderSignalIndex () const const |
virtual void | timerEvent (QTimerEvent *event) |
Detailed Description
A Channel represents a single channel in a Node.
Krita does not use channels to store local selections: these are strictly the color and alpha channels.
Constructor & Destructor Documentation
◆ Channel()
|
explicit |
Definition at line 32 of file Channel.cpp.
◆ ~Channel()
|
override |
Definition at line 40 of file Channel.cpp.
Member Function Documentation
◆ bounds()
QRect Channel::bounds | ( | ) | const |
- Returns
- the exact bounds of the channel. This can be smaller than the bounds of the Node this channel is part of.
Definition at line 114 of file Channel.cpp.
◆ channelSize()
int Channel::channelSize | ( | ) | const |
- Returns
- the number of bytes this channel takes
Definition at line 109 of file Channel.cpp.
◆ name()
QString Channel::name | ( | ) | const |
- Returns
- the name of the channel
Definition at line 99 of file Channel.cpp.
◆ operator!=()
bool Channel::operator!= | ( | const Channel & | other | ) | const |
Definition at line 52 of file Channel.cpp.
◆ operator==()
bool Channel::operator== | ( | const Channel & | other | ) | const |
Definition at line 46 of file Channel.cpp.
◆ pixelData()
QByteArray Channel::pixelData | ( | const QRect & | rect | ) | const |
Read the values of the channel into the a byte array for each pixel in the rect from the Node this channel is part of, and returns it.
Note that if Krita is built with OpenEXR and the Node has the 16 bits floating point channel depth type, Krita returns 32 bits float for every channel; the libkis scripting API does not support half.
Definition at line 152 of file Channel.cpp.
◆ position()
int Channel::position | ( | ) | const |
- Returns
- the position of the first byte of the channel in the pixel
Definition at line 104 of file Channel.cpp.
◆ setPixelData()
void Channel::setPixelData | ( | QByteArray | value, |
const QRect & | rect ) |
setPixelData writes the given data to the relevant channel in the Node.
This is only possible for Nodes that have a paintDevice, so nothing will happen when trying to write to e.g. a group layer.
Note that if Krita is built with OpenEXR and the Node has the 16 bits floating point channel depth type, Krita expects to be given a 4 byte, 32 bits float for every channel; the libkis scripting API does not support half.
- Parameters
-
value a byte array with exactly enough bytes. rect the rectangle to write the bytes into
Definition at line 196 of file Channel.cpp.
◆ setVisible()
void Channel::setVisible | ( | bool | value | ) |
setvisible set the visibility of the channel to the given value.
Definition at line 76 of file Channel.cpp.
◆ visible()
bool Channel::visible | ( | ) | const |
visible checks whether this channel is visible in the node
- Returns
- the status of this channel
Definition at line 58 of file Channel.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2025 The KDE developers.
Generated on Fri Jan 3 2025 11:57:35 by doxygen 1.12.0 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.