FileLayer
#include <FileLayer.h>
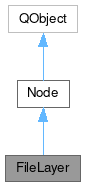
Public Slots | |
QString | path () const |
void | resetCache () |
QString | scalingFilter () const |
QString | scalingMethod () const |
void | setProperties (QString fileName, QString scalingMethod=QString("None"), QString scalingFilter=QString("Bicubic")) |
QString | type () const override |
![]() | |
bool | addChildNode (Node *child, Node *above) |
bool | alphaLocked () const |
bool | animated () const |
QString | blendingMode () const |
QRect | bounds () const |
QList< Channel * > | channels () const |
QList< Node * > | childNodes () const |
Node * | clone () const |
bool | collapsed () const |
QString | colorDepth () const |
int | colorLabel () const |
QString | colorModel () const |
QString | colorProfile () const |
void | cropNode (int x, int y, int w, int h) |
Node * | duplicate () |
void | enableAnimation () const |
QList< Node * > | findChildNodes (const QString &name=QString(), bool recursive=false, bool partialMatch=false, const QString &type=QString(), int colorLabelIndex=0) const |
bool | hasExtents () |
bool | hasKeyframeAtTime (int frameNumber) |
QIcon | icon () const |
int | index () const |
bool | inheritAlpha () const |
bool | isPinnedToTimeline () const |
QString | layerStyleToAsl () |
bool | locked () const |
Node * | mergeDown () |
void | move (int x, int y) |
QString | name () const |
int | opacity () const |
QString | paintAbility () |
void | paintEllipse (const QRectF &rect, const QString strokeStyle=PaintingResources::defaultStrokeStyle, const QString fillStyle=PaintingResources::defaultFillStyle) |
void | paintLine (const QPointF pointOne, const QPointF pointTwo, double pressureOne=1.0, double pressureTwo=1.0, const QString strokeStyle=PaintingResources::defaultStrokeStyle) |
void | paintPath (const QPainterPath &path, const QString strokeStyle=PaintingResources::defaultStrokeStyle, const QString fillStyle=PaintingResources::defaultFillStyle) |
void | paintPolygon (const QList< QPointF > points, const QString strokeStyle=PaintingResources::defaultStrokeStyle, const QString fillStyle=PaintingResources::defaultFillStyle) |
void | paintRectangle (const QRectF &rect, const QString strokeStyle=PaintingResources::defaultStrokeStyle, const QString fillStyle=PaintingResources::defaultFillStyle) |
Node * | parentNode () const |
QByteArray | pixelData (int x, int y, int w, int h) const |
QByteArray | pixelDataAtTime (int x, int y, int w, int h, int time) const |
QPoint | position () const |
QByteArray | projectionPixelData (int x, int y, int w, int h) const |
bool | remove () |
bool | removeChildNode (Node *child) |
void | rotateNode (double radians) |
bool | save (const QString &filename, double xRes, double yRes, const InfoObject &exportConfiguration, const QRect &exportRect=QRect()) |
void | scaleNode (QPointF origin, int width, int height, QString strategy) |
void | setAlphaLocked (bool value) |
void | setBlendingMode (QString value) |
void | setChildNodes (QList< Node * > nodes) |
void | setCollapsed (bool collapsed) |
void | setColorLabel (int index) |
bool | setColorProfile (const QString &colorProfile) |
bool | setColorSpace (const QString &colorModel, const QString &colorDepth, const QString &colorProfile) |
void | setInheritAlpha (bool value) |
bool | setLayerStyleFromAsl (const QString &asl) |
void | setLocked (bool value) |
void | setName (QString name) |
void | setOpacity (int value) |
void | setPinnedToTimeline (bool pinned) const |
bool | setPixelData (QByteArray value, int x, int y, int w, int h) |
void | setVisible (bool visible) |
void | shearNode (double angleX, double angleY) |
QImage | thumbnail (int w, int h) |
virtual QString | type () const |
QUuid | uniqueId () const |
bool | visible () const |
Public Member Functions | |
FileLayer (KisFileLayerSP layer, QObject *parent=0) | |
FileLayer (KisImageSP image, const QString name=QString(), const QString baseName=QString(), const QString fileName=QString(), const QString scalingMethod=QString(), const QString scalingFilter=QString(), QObject *parent=0) | |
![]() | |
bool | operator!= (const Node &other) const |
bool | operator== (const Node &other) const |
![]() | |
QObject (QObject *parent) | |
QBindable< QString > | bindableObjectName () |
bool | blockSignals (bool block) |
const QObjectList & | children () const const |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) const const |
bool | disconnect (const QObject *receiver, const char *method) const const |
void | dumpObjectInfo () const const |
void | dumpObjectTree () const const |
QList< QByteArray > | dynamicPropertyNames () const const |
virtual bool | event (QEvent *e) |
virtual bool | eventFilter (QObject *watched, QEvent *event) |
T | findChild (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QRegularExpression &re, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (Qt::FindChildOptions options) const const |
bool | inherits (const char *className) const const |
void | installEventFilter (QObject *filterObj) |
bool | isQuickItemType () const const |
bool | isWidgetType () const const |
bool | isWindowType () const const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const const |
void | moveToThread (QThread *targetThread) |
QString | objectName () const const |
void | objectNameChanged (const QString &objectName) |
QObject * | parent () const const |
QVariant | property (const char *name) const const |
Q_CLASSINFO (Name, Value) | |
Q_EMIT Q_EMIT | |
Q_ENUM (...) | |
Q_ENUM_NS (...) | |
Q_ENUMS (...) | |
Q_FLAG (...) | |
Q_FLAG_NS (...) | |
Q_FLAGS (...) | |
Q_GADGET Q_GADGET | |
Q_GADGET_EXPORT (EXPORT_MACRO) | |
Q_INTERFACES (...) | |
Q_INVOKABLE Q_INVOKABLE | |
Q_MOC_INCLUDE Q_MOC_INCLUDE | |
Q_NAMESPACE Q_NAMESPACE | |
Q_NAMESPACE_EXPORT (EXPORT_MACRO) | |
Q_OBJECT Q_OBJECT | |
Q_PROPERTY (...) | |
Q_REVISION Q_REVISION | |
Q_SET_OBJECT_NAME (Object) | |
Q_SIGNAL Q_SIGNAL | |
Q_SIGNALS Q_SIGNALS | |
Q_SLOT Q_SLOT | |
Q_SLOTS Q_SLOTS | |
T | qobject_cast (const QObject *object) |
T | qobject_cast (QObject *object) |
QT_NO_NARROWING_CONVERSIONS_IN_CONNECT QT_NO_NARROWING_CONVERSIONS_IN_CONNECT | |
void | removeEventFilter (QObject *obj) |
void | setObjectName (const QString &name) |
void | setObjectName (QAnyStringView name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | setProperty (const char *name, QVariant &&value) |
bool | signalsBlocked () const const |
int | startTimer (int interval, Qt::TimerType timerType) |
int | startTimer (std::chrono::milliseconds interval, Qt::TimerType timerType) |
QThread * | thread () const const |
Additional Inherited Members | |
![]() | |
objectName | |
![]() | |
static Node * | createNode (KisImageSP image, KisNodeSP node, QObject *parent=0) |
![]() | |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *context, Functor functor, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, Functor functor) |
bool | disconnect (const QMetaObject::Connection &connection) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
bool | disconnect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
![]() | |
typedef | QObjectList |
![]() | |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const QMetaMethod &signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const QMetaMethod &signal) |
bool | isSignalConnected (const QMetaMethod &signal) const const |
int | receivers (const char *signal) const const |
QObject * | sender () const const |
int | senderSignalIndex () const const |
virtual void | timerEvent (QTimerEvent *event) |
Detailed Description
The FileLayer class A file layer is a layer that can reference an external image and show said reference in the layer stack.
If the external image is updated, Krita will try to update the file layer image as well.
Definition at line 26 of file FileLayer.h.
Constructor & Destructor Documentation
◆ FileLayer() [1/2]
|
explicit |
Definition at line 12 of file FileLayer.cpp.
◆ FileLayer() [2/2]
|
explicit |
Definition at line 32 of file FileLayer.cpp.
◆ ~FileLayer()
|
override |
Definition at line 38 of file FileLayer.cpp.
Member Function Documentation
◆ path
|
slot |
path
- Returns
- A QString with the full path of the referenced image.
Definition at line 73 of file FileLayer.cpp.
◆ resetCache
|
slot |
makes the file layer to reload the connected image from disk
Definition at line 66 of file FileLayer.cpp.
◆ scalingFilter
|
slot |
scalingFilter returns the filter with which the file referenced is scaled.
Definition at line 95 of file FileLayer.cpp.
◆ scalingMethod
|
slot |
scalingMethod returns how the file referenced is scaled.
- Returns
- one of the following:
- None - The file is not scaled in any way.
- ToImageSize - The file is scaled to the full image size;
- ToImagePPI - The file is scaled by the PPI of the image. This keep the physical dimensions the same.
Definition at line 80 of file FileLayer.cpp.
◆ setProperties
|
slot |
setProperties Change the properties of the file layer.
- Parameters
-
fileName - A String containing the absolute file name. scalingMethod - a string with the scaling method, defaults to "None", other options are "ToImageSize" and "ToImagePPI" scalingFilter - a string with the scaling filter, defaults to "Bicubic", other options are "Hermite", "NearestNeighbor", "Bilinear", "Bell", "BSpline", "Lanczos3", "Mitchell"
Definition at line 48 of file FileLayer.cpp.
◆ type
|
overrideslot |
type Krita has several types of nodes, split in layers and masks.
Group layers can contain other layers, any layer can contain masks.
- Returns
- "filelayer"
Definition at line 43 of file FileLayer.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2025 The KDE developers.
Generated on Fri May 2 2025 12:05:08 by doxygen 1.13.2 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.