CSDButton
#include <csdcontrols.h>
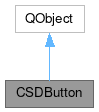
Public Types | |
enum | CSDButtonState { Normal , Hover , Pressed , Backdrop , Disabled } |
Properties | |
bool | isFocused |
QML_ELEMENTbool | isHovered |
bool | isMaximized |
bool | isPressed |
QUrl | source |
QString | style |
CSDButtonType | type |
![]() | |
objectName | |
Signals | |
void | isFocusedChanged () |
void | isHoveredChanged () |
void | isMaximizedChanged () |
void | isPressedChanged () |
void | sourceChanged () |
void | stateChanged () |
void | styleChanged () |
void | typeChanged () |
Public Slots | |
CSDButton::CSDButtonType | mapType (const QString &value) |
Public Member Functions | |
bool | isFocused () const |
bool | isHovered () const |
bool | isMaximized () const |
bool | isPressed () const |
Q_ENUM (CSDButtonState) enum CSDButtonType | |
Q_ENUM (CSDButtonType) explicit CSDButton(QObject *parent | |
void | setIsFocused (bool newIsFocused) |
void | setIsHovered (bool newIsHovered) |
void | setIsMaximized (bool newIsMaximized) |
void | setIsPressed (bool newIsPressed) |
void | setState (const CSDButtonState &state) |
void | setStyle (const QString &style) |
void | setType (CSDButton::CSDButtonType newType) |
QUrl | source () const |
CSDButtonState | state () const |
QString | style () const |
CSDButton::CSDButtonType | type () const |
![]() | |
QObject (QObject *parent) | |
QBindable< QString > | bindableObjectName () |
bool | blockSignals (bool block) |
const QObjectList & | children () const const |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) const const |
bool | disconnect (const QObject *receiver, const char *method) const const |
void | dumpObjectInfo () const const |
void | dumpObjectTree () const const |
QList< QByteArray > | dynamicPropertyNames () const const |
virtual bool | event (QEvent *e) |
virtual bool | eventFilter (QObject *watched, QEvent *event) |
T | findChild (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QRegularExpression &re, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (Qt::FindChildOptions options) const const |
bool | inherits (const char *className) const const |
void | installEventFilter (QObject *filterObj) |
bool | isQuickItemType () const const |
bool | isWidgetType () const const |
bool | isWindowType () const const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const const |
void | moveToThread (QThread *targetThread) |
QString | objectName () const const |
void | objectNameChanged (const QString &objectName) |
QObject * | parent () const const |
QVariant | property (const char *name) const const |
Q_CLASSINFO (Name, Value) | |
Q_EMIT Q_EMIT | |
Q_ENUM (...) | |
Q_ENUM_NS (...) | |
Q_ENUMS (...) | |
Q_FLAG (...) | |
Q_FLAG_NS (...) | |
Q_FLAGS (...) | |
Q_GADGET Q_GADGET | |
Q_GADGET_EXPORT (EXPORT_MACRO) | |
Q_INTERFACES (...) | |
Q_INVOKABLE Q_INVOKABLE | |
Q_MOC_INCLUDE Q_MOC_INCLUDE | |
Q_NAMESPACE Q_NAMESPACE | |
Q_NAMESPACE_EXPORT (EXPORT_MACRO) | |
Q_OBJECT Q_OBJECT | |
Q_PROPERTY (...) | |
Q_REVISION Q_REVISION | |
Q_SET_OBJECT_NAME (Object) | |
Q_SIGNAL Q_SIGNAL | |
Q_SIGNALS Q_SIGNALS | |
Q_SLOT Q_SLOT | |
Q_SLOTS Q_SLOTS | |
T | qobject_cast (const QObject *object) |
T | qobject_cast (QObject *object) |
QT_NO_NARROWING_CONVERSIONS_IN_CONNECT QT_NO_NARROWING_CONVERSIONS_IN_CONNECT | |
void | removeEventFilter (QObject *obj) |
void | setObjectName (const QString &name) |
void | setObjectName (QAnyStringView name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | setProperty (const char *name, QVariant &&value) |
bool | signalsBlocked () const const |
int | startTimer (int interval, Qt::TimerType timerType) |
int | startTimer (std::chrono::milliseconds interval, Qt::TimerType timerType) |
QThread * | thread () const const |
Additional Inherited Members | |
![]() | |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *context, Functor functor, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, Functor functor) |
bool | disconnect (const QMetaObject::Connection &connection) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
bool | disconnect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
![]() | |
typedef | QObjectList |
![]() | |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const QMetaMethod &signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const QMetaMethod &signal) |
bool | isSignalConnected (const QMetaMethod &signal) const const |
int | receivers (const char *signal) const const |
QObject * | sender () const const |
int | senderSignalIndex () const const |
virtual void | timerEvent (QTimerEvent *event) |
Detailed Description
An abstraction for a client-side-decoration button.
This class is exposed as the type CSDButton
to the QML engine, and it is used for creating the CSD window control themes.
CSDButton represents a button and its states. By reading the theme configuration, this class changes the images used as its state changes. The states need to be set manually.
Definition at line 19 of file csdcontrols.h.
Member Enumeration Documentation
◆ CSDButtonState
The states of a window control button.
Definition at line 72 of file csdcontrols.h.
Property Documentation
◆ isFocused
|
readwrite |
Whether the window is currently focused.
Uses the Normal
config entry to read the image file asset, if focused, other wise, the Backdrop
entry.
Definition at line 47 of file csdcontrols.h.
◆ isHovered
|
readwrite |
Whether the button is currently being hovered.
Uses the Hover
config entry to read the image file asset.
Definition at line 29 of file csdcontrols.h.
◆ isMaximized
|
readwrite |
Whether the window is currently maximized.
Uses the Restore
config section to read the image file assets.
Definition at line 35 of file csdcontrols.h.
◆ isPressed
|
readwrite |
Whether the button is currently being pressed.
Uses the Pressed
config entry to read the image file asset.
Definition at line 41 of file csdcontrols.h.
◆ source
|
read |
The source file path of the theme being used.
Definition at line 58 of file csdcontrols.h.
◆ style
|
readwrite |
The style to be used for picking up the image assets and config.
By default this will be set to the current preferred window controls style preference from MauiMan. However, this can be overridden to another existing style.
Definition at line 65 of file csdcontrols.h.
◆ type
|
readwrite |
Member Function Documentation
◆ isFocused()
bool CSDButton::isFocused | ( | ) | const |
Definition at line 323 of file csdcontrols.cpp.
◆ isHovered()
bool CSDButton::isHovered | ( | ) | const |
Definition at line 263 of file csdcontrols.cpp.
◆ isMaximized()
bool CSDButton::isMaximized | ( | ) | const |
Definition at line 283 of file csdcontrols.cpp.
◆ isPressed()
bool CSDButton::isPressed | ( | ) | const |
Definition at line 303 of file csdcontrols.cpp.
◆ mapType
|
slot |
Maps a based string value convention representing a button type to a CSDButton::CSDButtonType.
Usually each window control button is represented as a single letter, and the order of the window control buttons are an array of those string values.
An example would be the following array {"I", "A", "X"}
, which represents the following order: minimize, maximize, close
Definition at line 254 of file csdcontrols.cpp.
◆ Q_ENUM()
|
inline |
The possible types of supported window control buttons.
Closes the window surface
Minimizes/hides the window surface
Maximizes the window surface
Restores the window surface to the previous geometry it had before being maximized
Makes the window surface occupy the whole screen area
No button
Definition at line 98 of file csdcontrols.h.
◆ setIsFocused()
void CSDButton::setIsFocused | ( | bool | newIsFocused | ) |
Definition at line 328 of file csdcontrols.cpp.
◆ setIsHovered()
void CSDButton::setIsHovered | ( | bool | newIsHovered | ) |
Definition at line 268 of file csdcontrols.cpp.
◆ setIsMaximized()
void CSDButton::setIsMaximized | ( | bool | newIsMaximized | ) |
Definition at line 288 of file csdcontrols.cpp.
◆ setIsPressed()
void CSDButton::setIsPressed | ( | bool | newIsPressed | ) |
Definition at line 308 of file csdcontrols.cpp.
◆ setState()
void CSDButton::setState | ( | const CSDButtonState & | state | ) |
Definition at line 232 of file csdcontrols.cpp.
◆ setStyle()
void CSDButton::setStyle | ( | const QString & | style | ) |
Definition at line 138 of file csdcontrols.cpp.
◆ setType()
void CSDButton::setType | ( | CSDButton::CSDButtonType | newType | ) |
Definition at line 245 of file csdcontrols.cpp.
◆ source()
QUrl CSDButton::source | ( | ) | const |
Definition at line 154 of file csdcontrols.cpp.
◆ state()
CSDButton::CSDButtonState CSDButton::state | ( | ) | const |
Definition at line 178 of file csdcontrols.cpp.
◆ style()
QString CSDButton::style | ( | ) | const |
Definition at line 149 of file csdcontrols.cpp.
◆ type()
CSDButton::CSDButtonType CSDButton::type | ( | ) | const |
Definition at line 240 of file csdcontrols.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2025 The KDE developers.
Generated on Fri May 2 2025 11:57:11 by doxygen 1.13.2 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.