MD::PosCache
#include <poscache.h>
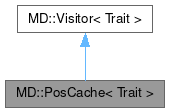
Public Types | |
using | Items = typename Trait::template Vector<Item<Trait> *> |
Public Member Functions | |
PosCache ()=default | |
~PosCache () override=default | |
Items | findFirstInCache (const MD::WithPosition &pos) const |
virtual void | initialize (std::shared_ptr< MD::Document< Trait > > doc) |
![]() | |
Visitor ()=default | |
virtual | ~Visitor ()=default |
void | process (std::shared_ptr< Document< Trait > > d) |
Protected Member Functions | |
void | findFirstInCache (const std::vector< details::PosRange< Trait > > &vec, const details::PosRange< Trait > &pos, Items &res) const |
details::PosRange< Trait > * | findInCache (std::vector< details::PosRange< Trait > > &vec, const details::PosRange< Trait > &pos) const |
void | insertInCache (const details::PosRange< Trait > &item, bool sort=false) |
void | onAddLineEnding () override |
void | onAnchor (Anchor< Trait > *) override |
void | onBlockquote (Blockquote< Trait > *b) override |
void | onCode (Code< Trait > *c) override |
void | onFootnote (Footnote< Trait > *f) override |
void | onFootnoteRef (FootnoteRef< Trait > *ref) override |
void | onHeading (Heading< Trait > *h) override |
void | onHorizontalLine (HorizontalLine< Trait > *l) override |
void | onImage (Image< Trait > *i) override |
void | onInlineCode (Code< Trait > *c) override |
void | onLineBreak (LineBreak< Trait > *) override |
void | onLink (Link< Trait > *l) override |
void | onList (List< Trait > *l) override |
void | onListItem (ListItem< Trait > *l, bool first) override |
void | onMath (Math< Trait > *m) override |
void | onParagraph (Paragraph< Trait > *p, bool wrap) override |
void | onRawHtml (RawHtml< Trait > *h) override |
virtual void | onReferenceLink (Link< Trait > *l) |
void | onTable (Table< Trait > *t) override |
void | onText (Text< Trait > *t) override |
void | onUserDefined (Item< Trait > *i) override |
![]() | |
virtual void | onTableCell (TableCell< Trait > *c) |
Protected Attributes | |
std::vector< details::PosRange< Trait > > | m_cache |
bool | m_skipInCache = false |
![]() | |
Trait::template Vector< typename Trait::String > | m_anchors |
std::shared_ptr< Document< Trait > > | m_doc |
Detailed Description
class MD::PosCache< Trait >
Cache of Markdown items to be accessed via position.
Definition at line 74 of file poscache.h.
Member Typedef Documentation
◆ Items
using MD::PosCache< Trait >::Items = typename Trait::template Vector<Item<Trait> *> |
Vector with items, where front is a top-level item, and back is most nested child.
Definition at line 101 of file poscache.h.
Constructor & Destructor Documentation
◆ PosCache()
|
default |
◆ ~PosCache()
|
overridedefault |
Member Function Documentation
◆ findFirstInCache() [1/2]
|
inline |
- Returns
- First occurense of Markdown item with all first children by the give position.
Definition at line 104 of file poscache.h.
◆ findFirstInCache() [2/2]
|
inlineprotected |
Find in cache items with the given position with all parents.
- Parameters
-
vec Cache. pos Position of sought-for item. res Reference to result of search.
Definition at line 140 of file poscache.h.
◆ findInCache()
|
inlineprotected |
Find in cache an item with the given position.
- Parameters
-
vec Cache of position. pos Position of sought-for item.
Definition at line 118 of file poscache.h.
◆ initialize()
|
inlinevirtual |
Initialize m_cache with the give document.
- Note
- Document should not be recursive.
Definition at line 82 of file poscache.h.
◆ insertInCache()
|
inlineprotected |
Insert in cache.
- Parameters
-
item Position for insertion. sort Should we sord when insert top-level item, or we can be sure that this item is last?
Definition at line 160 of file poscache.h.
◆ onAddLineEnding()
|
inlineoverrideprotectedvirtual |
For some generator it's important to keep line endings like they were in Markdown.
So onParagraph() method invokes this method when necessary to add line ending.
Implements MD::Visitor< Trait >.
Definition at line 208 of file poscache.h.
◆ onAnchor()
|
inlineoverrideprotectedvirtual |
Handle anchor.
- Parameters
-
a Anchor.
Implements MD::Visitor< Trait >.
Definition at line 369 of file poscache.h.
◆ onBlockquote()
|
inlineoverrideprotectedvirtual |
Cache blockquote.
Reimplemented from MD::Visitor< Trait >.
Definition at line 310 of file poscache.h.
◆ onCode()
|
inlineoverrideprotectedvirtual |
◆ onFootnote()
|
inlineoverrideprotectedvirtual |
◆ onFootnoteRef()
|
inlineoverrideprotectedvirtual |
Cache footnote reference.
Implements MD::Visitor< Trait >.
Definition at line 448 of file poscache.h.
◆ onHeading()
|
inlineoverrideprotectedvirtual |
◆ onHorizontalLine()
|
inlineoverrideprotectedvirtual |
◆ onImage()
|
inlineoverrideprotectedvirtual |
◆ onInlineCode()
|
inlineoverrideprotectedvirtual |
◆ onLineBreak()
|
inlineoverrideprotectedvirtual |
Handle line break.
- Parameters
-
b Linebreak.
Implements MD::Visitor< Trait >.
Definition at line 247 of file poscache.h.
◆ onLink()
|
inlineoverrideprotectedvirtual |
◆ onList()
|
inlineoverrideprotectedvirtual |
◆ onListItem()
|
inlineoverrideprotectedvirtual |
Cache list item.
Reimplemented from MD::Visitor< Trait >.
Definition at line 481 of file poscache.h.
◆ onMath()
|
inlineoverrideprotectedvirtual |
Cache LaTeX math expression.
Implements MD::Visitor< Trait >.
Definition at line 225 of file poscache.h.
◆ onParagraph()
|
inlineoverrideprotectedvirtual |
Cache paragraph.
Reimplemented from MD::Visitor< Trait >.
Definition at line 252 of file poscache.h.
◆ onRawHtml()
|
inlineoverrideprotectedvirtual |
◆ onReferenceLink()
|
inlineprotectedvirtual |
◆ onTable()
|
inlineoverrideprotectedvirtual |
◆ onText()
|
inlineoverrideprotectedvirtual |
◆ onUserDefined()
|
inlineoverrideprotectedvirtual |
Cache user defined item.
Reimplemented from MD::Visitor< Trait >.
Definition at line 191 of file poscache.h.
Member Data Documentation
◆ m_cache
|
protected |
Cache.
Definition at line 492 of file poscache.h.
◆ m_skipInCache
|
protected |
Skip adding in cache.
Definition at line 494 of file poscache.h.
The documentation for this class was generated from the following file:
Documentation copyright © 1996-2025 The KDE developers.
Generated on Fri May 2 2025 12:05:26 by doxygen 1.13.2 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.