Okular::RegularArea
Okular::RegularArea< NormalizedShape, Shape > Class Template Reference
#include <area.h>
Inheritance diagram for Okular::RegularArea< NormalizedShape, Shape >:
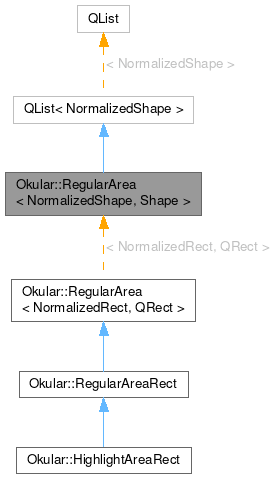
Public Member Functions | |
void | appendArea (const RegularArea< NormalizedShape, Shape > *area) |
void | appendShape (const NormalizedShape &shape, MergeSide side=MergeAll) |
bool | contains (const NormalizedShape &shape) const |
bool | contains (double x, double y) const |
QList< Shape > | geometry (int xScale, int yScale, int dx=0, int dy=0) const |
bool | intersects (const NormalizedShape &shape) const |
bool | intersects (const RegularArea< NormalizedShape, Shape > *area) const |
bool | isNull () const |
void | simplify () |
void | transform (const QTransform &matrix) |
![]() | |
QList (const QList< T > &other) | |
QList (InputIterator first, InputIterator last) | |
QList (QList< T > &&other) | |
QList (qsizetype size) | |
QList (qsizetype size, parameter_type value) | |
QList (std::initializer_list< T > args) | |
void | append (const QList< T > &value) |
void | append (parameter_type value) |
void | append (QList< T > &&value) |
void | append (rvalue_ref value) |
const_reference | at (qsizetype i) const const |
reference | back () |
const_reference | back () const const |
iterator | begin () |
const_iterator | begin () const const |
qsizetype | capacity () const const |
const_iterator | cbegin () const const |
const_iterator | cend () const const |
void | clear () |
const_iterator | constBegin () const const |
const_pointer | constData () const const |
const_iterator | constEnd () const const |
const T & | constFirst () const const |
const T & | constLast () const const |
bool | contains (const AT &value) const const |
qsizetype | count () const const |
qsizetype | count (const AT &value) const const |
const_reverse_iterator | crbegin () const const |
const_reverse_iterator | crend () const const |
pointer | data () |
const_pointer | data () const const |
iterator | emplace (const_iterator before, Args &&... args) |
iterator | emplace (qsizetype i, Args &&... args) |
reference | emplace_back (Args &&... args) |
reference | emplaceBack (Args &&... args) |
bool | empty () const const |
iterator | end () |
const_iterator | end () const const |
bool | endsWith (parameter_type value) const const |
iterator | erase (const_iterator begin, const_iterator end) |
iterator | erase (const_iterator pos) |
qsizetype | erase (QList< T > &list, const AT &t) |
qsizetype | erase_if (QList< T > &list, Predicate pred) |
QList< T > & | fill (parameter_type value, qsizetype size) |
T & | first () |
const T & | first () const const |
QList< T > | first (qsizetype n) const const |
reference | front () |
const_reference | front () const const |
qsizetype | indexOf (const AT &value, qsizetype from) const const |
iterator | insert (const_iterator before, parameter_type value) |
iterator | insert (const_iterator before, qsizetype count, parameter_type value) |
iterator | insert (const_iterator before, rvalue_ref value) |
iterator | insert (qsizetype i, parameter_type value) |
iterator | insert (qsizetype i, qsizetype count, parameter_type value) |
iterator | insert (qsizetype i, rvalue_ref value) |
bool | isEmpty () const const |
T & | last () |
const T & | last () const const |
QList< T > | last (qsizetype n) const const |
qsizetype | lastIndexOf (const AT &value, qsizetype from) const const |
qsizetype | length () const const |
QList< T > | mid (qsizetype pos, qsizetype length) const const |
void | move (qsizetype from, qsizetype to) |
bool | operator!= (const QList< T > &other) const const |
QList< T > | operator+ (const QList< T > &other) && |
QList< T > | operator+ (const QList< T > &other) const &const |
QList< T > | operator+ (QList< T > &&other) && |
QList< T > | operator+ (QList< T > &&other) const &const |
QList< T > & | operator+= (const QList< T > &other) |
QList< T > & | operator+= (parameter_type value) |
QList< T > & | operator+= (QList< T > &&other) |
QList< T > & | operator+= (rvalue_ref value) |
bool | operator< (const QList< T > &other) const const |
QList< T > & | operator<< (const QList< T > &other) |
QList< T > & | operator<< (parameter_type value) |
QDataStream & | operator<< (QDataStream &out, const QList< T > &list) |
QList< T > & | operator<< (QList< T > &&other) |
QList< T > & | operator<< (rvalue_ref value) |
bool | operator<= (const QList< T > &other) const const |
QList< T > & | operator= (const QList< T > &other) |
QList< T > & | operator= (QList< T > &&other) |
QList< T > & | operator= (std::initializer_list< T > args) |
bool | operator== (const QList< T > &other) const const |
bool | operator> (const QList< T > &other) const const |
bool | operator>= (const QList< T > &other) const const |
QDataStream & | operator>> (QDataStream &in, QList< T > &list) |
reference | operator[] (qsizetype i) |
const_reference | operator[] (qsizetype i) const const |
void | pop_back () |
void | pop_front () |
void | prepend (parameter_type value) |
void | prepend (rvalue_ref value) |
void | push_back (parameter_type value) |
void | push_back (rvalue_ref value) |
void | push_front (parameter_type value) |
void | push_front (rvalue_ref value) |
size_t | qHash (const QList< T > &key, size_t seed) |
reverse_iterator | rbegin () |
const_reverse_iterator | rbegin () const const |
void | remove (qsizetype i, qsizetype n) |
qsizetype | removeAll (const AT &t) |
void | removeAt (qsizetype i) |
void | removeFirst () |
qsizetype | removeIf (Predicate pred) |
void | removeLast () |
bool | removeOne (const AT &t) |
reverse_iterator | rend () |
const_reverse_iterator | rend () const const |
void | replace (qsizetype i, parameter_type value) |
void | replace (qsizetype i, rvalue_ref value) |
void | reserve (qsizetype size) |
void | resize (qsizetype size) |
void | resize (qsizetype size, parameter_type c) |
void | shrink_to_fit () |
qsizetype | size () const const |
QList< T > | sliced (qsizetype pos) const const |
QList< T > | sliced (qsizetype pos, qsizetype n) const const |
void | squeeze () |
bool | startsWith (parameter_type value) const const |
void | swap (QList< T > &other) |
void | swapItemsAt (qsizetype i, qsizetype j) |
T | takeAt (qsizetype i) |
value_type | takeFirst () |
value_type | takeLast () |
QList< T > | toList () const const |
QList< T > | toVector () const const |
T | value (qsizetype i) const const |
T | value (qsizetype i, parameter_type defaultValue) const const |
Additional Inherited Members | |
![]() | |
QList< T > | fromList (const QList< T > &list) |
QList< T > | fromVector (const QList< T > &list) |
![]() | |
typedef | const_pointer |
typedef | const_reference |
typedef | const_reverse_iterator |
typedef | ConstIterator |
typedef | difference_type |
typedef | Iterator |
typedef | parameter_type |
typedef | pointer |
typedef | reference |
typedef | reverse_iterator |
typedef | rvalue_ref |
typedef | size_type |
typedef | value_type |
Detailed Description
template<class NormalizedShape, class Shape>
class Okular::RegularArea< NormalizedShape, Shape >
class Okular::RegularArea< NormalizedShape, Shape >
An area with normalized coordinates, consisting of NormalizedShape objects.
This is a template class to describe an area which consists of multiple shapes of the same type, intersecting or non-intersecting. The coordinates are normalized, and can be mapped to a reference area of defined size. For more information about the normalized coordinate system, see NormalizedPoint.
Class NormalizedShape must have the following functions/operators defined:
- bool contains( double, double ), whether it contains the given NormalizedPoint
- bool intersects( NormalizedShape )
- bool isNull()
- Shape geometry( int, int ), which maps to the reference area
- operator|=( NormalizedShape ), which unites two NormalizedShape's
- See also
- RegularAreaRect, NormalizedPoint
Member Function Documentation
◆ appendArea()
template<class NormalizedShape, class Shape>
void Okular::RegularArea< NormalizedShape, Shape >::appendArea | ( | const RegularArea< NormalizedShape, Shape > * | area | ) |
◆ appendShape()
template<class NormalizedShape, class Shape>
void Okular::RegularArea< NormalizedShape, Shape >::appendShape | ( | const NormalizedShape & | shape, |
MergeSide | side = MergeAll ) |
◆ contains() [1/2]
template<class NormalizedShape, class Shape>
bool Okular::RegularArea< NormalizedShape, Shape >::contains | ( | const NormalizedShape & | shape | ) | const |
Returns whether this area contains a NormalizedShape object that equals shape
.
- Note
- The original NormalizedShape objects can be lost if simplify() was called.
◆ contains() [2/2]
template<class NormalizedShape, class Shape>
bool Okular::RegularArea< NormalizedShape, Shape >::contains | ( | double | x, |
double | y ) const |
◆ geometry()
template<class NormalizedShape, class Shape>
QList< Shape > Okular::RegularArea< NormalizedShape, Shape >::geometry | ( | int | xScale, |
int | yScale, | ||
int | dx = 0, | ||
int | dy = 0 ) const |
◆ intersects() [1/2]
template<class NormalizedShape, class Shape>
bool Okular::RegularArea< NormalizedShape, Shape >::intersects | ( | const NormalizedShape & | shape | ) | const |
◆ intersects() [2/2]
template<class NormalizedShape, class Shape>
bool Okular::RegularArea< NormalizedShape, Shape >::intersects | ( | const RegularArea< NormalizedShape, Shape > * | area | ) | const |
◆ isNull()
template<class NormalizedShape, class Shape>
bool Okular::RegularArea< NormalizedShape, Shape >::isNull | ( | ) | const |
◆ simplify()
template<class NormalizedShape, class Shape>
void Okular::RegularArea< NormalizedShape, Shape >::simplify | ( | ) |
◆ transform()
template<class NormalizedShape, class Shape>
void Okular::RegularArea< NormalizedShape, Shape >::transform | ( | const QTransform & | matrix | ) |
The documentation for this class was generated from the following file:
This file is part of the KDE documentation.
Documentation copyright © 1996-2025 The KDE developers.
Generated on Fri Apr 11 2025 11:55:12 by doxygen 1.13.2 written by Dimitri van Heesch, © 1997-2006
Documentation copyright © 1996-2025 The KDE developers.
Generated on Fri Apr 11 2025 11:55:12 by doxygen 1.13.2 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.