PerceptualColor::ColorDialog
#include <colordialog.h>
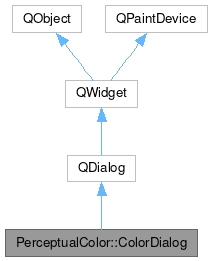
Public Types | |
typedef QColorDialog::ColorDialogOption | ColorDialogOption |
typedef QColorDialog::ColorDialogOptions | ColorDialogOptions |
enum class | DialogLayoutDimensions { ScreenSizeDependent , Collapsed , Expanded } |
![]() | |
enum | DialogCode |
![]() | |
enum | RenderFlag |
typedef | RenderFlags |
![]() | |
typedef | QObjectList |
![]() | |
enum | PaintDeviceMetric |
Signals | |
void | colorSelected (const QColor &color) |
void | currentColorChanged (const QColor &color) |
void | layoutDimensionsChanged (const PerceptualColor::ColorDialog::DialogLayoutDimensions newLayoutDimensions) |
void | optionsChanged (const PerceptualColor::ColorDialog::ColorDialogOptions newOptions) |
Public Slots | |
void | setCurrentColor (const QColor &color) |
void | setLayoutDimensions (const PerceptualColor::ColorDialog::DialogLayoutDimensions newLayoutDimensions) |
Q_INVOKABLE void | setOption (PerceptualColor::ColorDialog::ColorDialogOption option, bool on=true) |
void | setOptions (PerceptualColor::ColorDialog::ColorDialogOptions newOptions) |
Public Member Functions | |
Q_INVOKABLE | ColorDialog (const QColor &initial, QWidget *parent=nullptr) |
Q_INVOKABLE | ColorDialog (const QSharedPointer< PerceptualColor::RgbColorSpace > &colorSpace, const QColor &initial, QWidget *parent=nullptr) |
Q_INVOKABLE | ColorDialog (const QSharedPointer< PerceptualColor::RgbColorSpace > &colorSpace, QWidget *parent=nullptr) |
Q_INVOKABLE | ColorDialog (QWidget *parent=nullptr) |
virtual | ~ColorDialog () noexcept override |
QColor | currentColor () const |
ColorDialog::DialogLayoutDimensions | layoutDimensions () const |
virtual void | open () |
Q_INVOKABLE void | open (QObject *receiver, const char *member) |
ColorDialogOptions | options () const |
Q_INVOKABLE QColor | selectedColor () const |
virtual void | setVisible (bool visible) override |
Q_INVOKABLE bool | testOption (PerceptualColor::ColorDialog::ColorDialogOption option) const |
![]() | |
QDialog (QWidget *parent, Qt::WindowFlags f) | |
virtual void | accept () |
void | accepted () |
virtual int | exec () |
void | finished (int result) |
bool | isSizeGripEnabled () const const |
virtual QSize | minimumSizeHint () const const override |
virtual void | reject () |
void | rejected () |
int | result () const const |
void | setModal (bool modal) |
void | setResult (int i) |
void | setSizeGripEnabled (bool) |
virtual QSize | sizeHint () const const override |
![]() | |
QWidget (QWidget *parent, Qt::WindowFlags f) | |
bool | acceptDrops () const const |
QString | accessibleDescription () const const |
QString | accessibleName () const const |
QList< QAction * > | actions () const const |
void | activateWindow () |
QAction * | addAction (const QIcon &icon, const QString &text) |
QAction * | addAction (const QIcon &icon, const QString &text, Args &&... args) |
QAction * | addAction (const QIcon &icon, const QString &text, const QKeySequence &shortcut) |
QAction * | addAction (const QIcon &icon, const QString &text, const QKeySequence &shortcut, Args &&... args) |
QAction * | addAction (const QIcon &icon, const QString &text, const QKeySequence &shortcut, const QObject *receiver, const char *member, Qt::ConnectionType type) |
QAction * | addAction (const QIcon &icon, const QString &text, const QObject *receiver, const char *member, Qt::ConnectionType type) |
QAction * | addAction (const QString &text) |
QAction * | addAction (const QString &text, Args &&... args) |
QAction * | addAction (const QString &text, const QKeySequence &shortcut) |
QAction * | addAction (const QString &text, const QKeySequence &shortcut, Args &&... args) |
QAction * | addAction (const QString &text, const QKeySequence &shortcut, const QObject *receiver, const char *member, Qt::ConnectionType type) |
QAction * | addAction (const QString &text, const QObject *receiver, const char *member, Qt::ConnectionType type) |
void | addAction (QAction *action) |
void | addActions (const QList< QAction * > &actions) |
void | adjustSize () |
bool | autoFillBackground () const const |
QPalette::ColorRole | backgroundRole () const const |
QBackingStore * | backingStore () const const |
QSize | baseSize () const const |
QWidget * | childAt (const QPoint &p) const const |
QWidget * | childAt (int x, int y) const const |
QRect | childrenRect () const const |
QRegion | childrenRegion () const const |
void | clearFocus () |
void | clearMask () |
bool | close () |
QMargins | contentsMargins () const const |
QRect | contentsRect () const const |
Qt::ContextMenuPolicy | contextMenuPolicy () const const |
QCursor | cursor () const const |
void | customContextMenuRequested (const QPoint &pos) |
WId | effectiveWinId () const const |
void | ensurePolished () const const |
Qt::FocusPolicy | focusPolicy () const const |
QWidget * | focusProxy () const const |
QWidget * | focusWidget () const const |
const QFont & | font () const const |
QFontInfo | fontInfo () const const |
QFontMetrics | fontMetrics () const const |
QPalette::ColorRole | foregroundRole () const const |
QRect | frameGeometry () const const |
QSize | frameSize () const const |
const QRect & | geometry () const const |
QPixmap | grab (const QRect &rectangle) |
void | grabGesture (Qt::GestureType gesture, Qt::GestureFlags flags) |
void | grabKeyboard () |
void | grabMouse () |
void | grabMouse (const QCursor &cursor) |
int | grabShortcut (const QKeySequence &key, Qt::ShortcutContext context) |
QGraphicsEffect * | graphicsEffect () const const |
QGraphicsProxyWidget * | graphicsProxyWidget () const const |
bool | hasEditFocus () const const |
bool | hasFocus () const const |
virtual bool | hasHeightForWidth () const const |
bool | hasMouseTracking () const const |
bool | hasTabletTracking () const const |
int | height () const const |
virtual int | heightForWidth (int w) const const |
void | hide () |
Qt::InputMethodHints | inputMethodHints () const const |
virtual QVariant | inputMethodQuery (Qt::InputMethodQuery query) const const |
void | insertAction (QAction *before, QAction *action) |
void | insertActions (QAction *before, const QList< QAction * > &actions) |
bool | isActiveWindow () const const |
bool | isAncestorOf (const QWidget *child) const const |
bool | isEnabled () const const |
bool | isEnabledTo (const QWidget *ancestor) const const |
bool | isFullScreen () const const |
bool | isHidden () const const |
bool | isMaximized () const const |
bool | isMinimized () const const |
bool | isModal () const const |
bool | isTopLevel () const const |
bool | isVisible () const const |
bool | isVisibleTo (const QWidget *ancestor) const const |
bool | isWindow () const const |
bool | isWindowModified () const const |
QLayout * | layout () const const |
Qt::LayoutDirection | layoutDirection () const const |
QLocale | locale () const const |
void | lower () |
QPoint | mapFrom (const QWidget *parent, const QPoint &pos) const const |
QPointF | mapFrom (const QWidget *parent, const QPointF &pos) const const |
QPoint | mapFromGlobal (const QPoint &pos) const const |
QPointF | mapFromGlobal (const QPointF &pos) const const |
QPoint | mapFromParent (const QPoint &pos) const const |
QPointF | mapFromParent (const QPointF &pos) const const |
QPoint | mapTo (const QWidget *parent, const QPoint &pos) const const |
QPointF | mapTo (const QWidget *parent, const QPointF &pos) const const |
QPoint | mapToGlobal (const QPoint &pos) const const |
QPointF | mapToGlobal (const QPointF &pos) const const |
QPoint | mapToParent (const QPoint &pos) const const |
QPointF | mapToParent (const QPointF &pos) const const |
QRegion | mask () const const |
int | maximumHeight () const const |
QSize | maximumSize () const const |
int | maximumWidth () const const |
int | minimumHeight () const const |
QSize | minimumSize () const const |
int | minimumWidth () const const |
void | move (const QPoint &) |
void | move (int x, int y) |
QWidget * | nativeParentWidget () const const |
QWidget * | nextInFocusChain () const const |
QRect | normalGeometry () const const |
void | overrideWindowFlags (Qt::WindowFlags flags) |
virtual QPaintEngine * | paintEngine () const const override |
const QPalette & | palette () const const |
QWidget * | parentWidget () const const |
QPoint | pos () const const |
QWidget * | previousInFocusChain () const const |
QWIDGETSIZE_MAX QWIDGETSIZE_MAX | |
void | raise () |
QRect | rect () const const |
void | releaseKeyboard () |
void | releaseMouse () |
void | releaseShortcut (int id) |
void | removeAction (QAction *action) |
void | render (QPaintDevice *target, const QPoint &targetOffset, const QRegion &sourceRegion, RenderFlags renderFlags) |
void | render (QPainter *painter, const QPoint &targetOffset, const QRegion &sourceRegion, RenderFlags renderFlags) |
void | repaint () |
void | repaint (const QRect &rect) |
void | repaint (const QRegion &rgn) |
void | repaint (int x, int y, int w, int h) |
void | resize (const QSize &) |
void | resize (int w, int h) |
bool | restoreGeometry (const QByteArray &geometry) |
QByteArray | saveGeometry () const const |
QScreen * | screen () const const |
void | scroll (int dx, int dy) |
void | scroll (int dx, int dy, const QRect &r) |
void | setAcceptDrops (bool on) |
void | setAccessibleDescription (const QString &description) |
void | setAccessibleName (const QString &name) |
void | setAttribute (Qt::WidgetAttribute attribute, bool on) |
void | setAutoFillBackground (bool enabled) |
void | setBackgroundRole (QPalette::ColorRole role) |
void | setBaseSize (const QSize &) |
void | setBaseSize (int basew, int baseh) |
void | setContentsMargins (const QMargins &margins) |
void | setContentsMargins (int left, int top, int right, int bottom) |
void | setContextMenuPolicy (Qt::ContextMenuPolicy policy) |
void | setCursor (const QCursor &) |
void | setDisabled (bool disable) |
void | setEditFocus (bool enable) |
void | setEnabled (bool) |
void | setFixedHeight (int h) |
void | setFixedSize (const QSize &s) |
void | setFixedSize (int w, int h) |
void | setFixedWidth (int w) |
void | setFocus () |
void | setFocus (Qt::FocusReason reason) |
void | setFocusPolicy (Qt::FocusPolicy policy) |
void | setFocusProxy (QWidget *w) |
void | setFont (const QFont &) |
void | setForegroundRole (QPalette::ColorRole role) |
void | setGeometry (const QRect &) |
void | setGeometry (int x, int y, int w, int h) |
void | setGraphicsEffect (QGraphicsEffect *effect) |
void | setHidden (bool hidden) |
void | setInputMethodHints (Qt::InputMethodHints hints) |
void | setLayout (QLayout *layout) |
void | setLayoutDirection (Qt::LayoutDirection direction) |
void | setLocale (const QLocale &locale) |
void | setMask (const QBitmap &bitmap) |
void | setMask (const QRegion ®ion) |
void | setMaximumHeight (int maxh) |
void | setMaximumSize (const QSize &) |
void | setMaximumSize (int maxw, int maxh) |
void | setMaximumWidth (int maxw) |
void | setMinimumHeight (int minh) |
void | setMinimumSize (const QSize &) |
void | setMinimumSize (int minw, int minh) |
void | setMinimumWidth (int minw) |
void | setMouseTracking (bool enable) |
void | setPalette (const QPalette &) |
void | setParent (QWidget *parent) |
void | setParent (QWidget *parent, Qt::WindowFlags f) |
void | setScreen (QScreen *screen) |
void | setShortcutAutoRepeat (int id, bool enable) |
void | setShortcutEnabled (int id, bool enable) |
void | setSizeIncrement (const QSize &) |
void | setSizeIncrement (int w, int h) |
void | setSizePolicy (QSizePolicy) |
void | setSizePolicy (QSizePolicy::Policy horizontal, QSizePolicy::Policy vertical) |
void | setStatusTip (const QString &) |
void | setStyle (QStyle *style) |
void | setStyleSheet (const QString &styleSheet) |
void | setTabletTracking (bool enable) |
void | setToolTip (const QString &) |
void | setToolTipDuration (int msec) |
void | setUpdatesEnabled (bool enable) |
void | setupUi (QWidget *widget) |
void | setWhatsThis (const QString &) |
void | setWindowFilePath (const QString &filePath) |
void | setWindowFlag (Qt::WindowType flag, bool on) |
void | setWindowFlags (Qt::WindowFlags type) |
void | setWindowIcon (const QIcon &icon) |
void | setWindowIconText (const QString &) |
void | setWindowModality (Qt::WindowModality windowModality) |
void | setWindowModified (bool) |
void | setWindowOpacity (qreal level) |
void | setWindowRole (const QString &role) |
void | setWindowState (Qt::WindowStates windowState) |
void | setWindowTitle (const QString &) |
void | show () |
void | showFullScreen () |
void | showMaximized () |
void | showMinimized () |
void | showNormal () |
QSize | size () const const |
QSize | sizeIncrement () const const |
QSizePolicy | sizePolicy () const const |
void | stackUnder (QWidget *w) |
QString | statusTip () const const |
QStyle * | style () const const |
QString | styleSheet () const const |
bool | testAttribute (Qt::WidgetAttribute attribute) const const |
QString | toolTip () const const |
int | toolTipDuration () const const |
QWidget * | topLevelWidget () const const |
bool | underMouse () const const |
void | ungrabGesture (Qt::GestureType gesture) |
void | unsetCursor () |
void | unsetLayoutDirection () |
void | unsetLocale () |
void | update () |
void | update (const QRect &rect) |
void | update (const QRegion &rgn) |
void | update (int x, int y, int w, int h) |
void | updateGeometry () |
bool | updatesEnabled () const const |
QRegion | visibleRegion () const const |
QString | whatsThis () const const |
int | width () const const |
QWidget * | window () const const |
QString | windowFilePath () const const |
Qt::WindowFlags | windowFlags () const const |
QWindow * | windowHandle () const const |
QIcon | windowIcon () const const |
void | windowIconChanged (const QIcon &icon) |
QString | windowIconText () const const |
void | windowIconTextChanged (const QString &iconText) |
Qt::WindowModality | windowModality () const const |
qreal | windowOpacity () const const |
QString | windowRole () const const |
Qt::WindowStates | windowState () const const |
QString | windowTitle () const const |
void | windowTitleChanged (const QString &title) |
Qt::WindowType | windowType () const const |
WId | winId () const const |
int | x () const const |
int | y () const const |
![]() | |
QObject (QObject *parent) | |
QBindable< QString > | bindableObjectName () |
bool | blockSignals (bool block) |
const QObjectList & | children () const const |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) const const |
bool | disconnect (const QObject *receiver, const char *method) const const |
void | dumpObjectInfo () const const |
void | dumpObjectTree () const const |
QList< QByteArray > | dynamicPropertyNames () const const |
T | findChild (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QRegularExpression &re, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (Qt::FindChildOptions options) const const |
bool | inherits (const char *className) const const |
void | installEventFilter (QObject *filterObj) |
bool | isQuickItemType () const const |
bool | isWidgetType () const const |
bool | isWindowType () const const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const const |
void | moveToThread (QThread *targetThread) |
QString | objectName () const const |
void | objectNameChanged (const QString &objectName) |
QObject * | parent () const const |
QVariant | property (const char *name) const const |
Q_CLASSINFO (Name, Value) | |
Q_EMIT Q_EMIT | |
Q_ENUM (...) | |
Q_ENUM_NS (...) | |
Q_ENUMS (...) | |
Q_FLAG (...) | |
Q_FLAG_NS (...) | |
Q_FLAGS (...) | |
Q_GADGET Q_GADGET | |
Q_GADGET_EXPORT (EXPORT_MACRO) | |
Q_INTERFACES (...) | |
Q_INVOKABLE Q_INVOKABLE | |
Q_MOC_INCLUDE Q_MOC_INCLUDE | |
Q_NAMESPACE Q_NAMESPACE | |
Q_NAMESPACE_EXPORT (EXPORT_MACRO) | |
Q_OBJECT Q_OBJECT | |
Q_PROPERTY (...) | |
Q_REVISION Q_REVISION | |
Q_SET_OBJECT_NAME (Object) | |
Q_SIGNAL Q_SIGNAL | |
Q_SIGNALS Q_SIGNALS | |
Q_SLOT Q_SLOT | |
Q_SLOTS Q_SLOTS | |
T | qobject_cast (const QObject *object) |
T | qobject_cast (QObject *object) |
QT_NO_NARROWING_CONVERSIONS_IN_CONNECT QT_NO_NARROWING_CONVERSIONS_IN_CONNECT | |
void | removeEventFilter (QObject *obj) |
void | setObjectName (const QString &name) |
void | setObjectName (QAnyStringView name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | setProperty (const char *name, QVariant &&value) |
bool | signalsBlocked () const const |
int | startTimer (int interval, Qt::TimerType timerType) |
int | startTimer (std::chrono::milliseconds interval, Qt::TimerType timerType) |
QThread * | thread () const const |
![]() | |
int | colorCount () const const |
int | depth () const const |
qreal | devicePixelRatio () const const |
qreal | devicePixelRatioF () const const |
int | height () const const |
int | heightMM () const const |
int | logicalDpiX () const const |
int | logicalDpiY () const const |
bool | paintingActive () const const |
int | physicalDpiX () const const |
int | physicalDpiY () const const |
int | width () const const |
int | widthMM () const const |
Static Public Member Functions | |
static QColor | getColor (const QColor &initial=Qt::white, QWidget *parent=nullptr, const QString &title=QString(), ColorDialogOptions options=ColorDialogOptions()) |
static QColor | getColor (const QSharedPointer< PerceptualColor::RgbColorSpace > &colorSpace, const QColor &initial=Qt::white, QWidget *parent=nullptr, const QString &title=QString(), ColorDialogOptions options=ColorDialogOptions()) |
![]() | |
QWidget * | createWindowContainer (QWindow *window, QWidget *parent, Qt::WindowFlags flags) |
QWidget * | find (WId id) |
QWidget * | keyboardGrabber () |
QWidget * | mouseGrabber () |
void | setTabOrder (QWidget *first, QWidget *second) |
void | setTabOrder (std::initializer_list< QWidget * > widgets) |
![]() | |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *context, Functor functor, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, Functor functor) |
bool | disconnect (const QMetaObject::Connection &connection) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
bool | disconnect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
Protected Member Functions | |
virtual void | changeEvent (QEvent *event) override |
virtual void | done (int result) override |
virtual void | showEvent (QShowEvent *event) override |
![]() | |
virtual void | closeEvent (QCloseEvent *e) override |
virtual void | contextMenuEvent (QContextMenuEvent *e) override |
virtual bool | eventFilter (QObject *o, QEvent *e) override |
virtual void | keyPressEvent (QKeyEvent *e) override |
virtual void | resizeEvent (QResizeEvent *) override |
![]() | |
virtual void | actionEvent (QActionEvent *event) |
void | create (WId window, bool initializeWindow, bool destroyOldWindow) |
void | destroy (bool destroyWindow, bool destroySubWindows) |
virtual void | dragEnterEvent (QDragEnterEvent *event) |
virtual void | dragLeaveEvent (QDragLeaveEvent *event) |
virtual void | dragMoveEvent (QDragMoveEvent *event) |
virtual void | dropEvent (QDropEvent *event) |
virtual void | enterEvent (QEnterEvent *event) |
virtual bool | event (QEvent *event) override |
virtual void | focusInEvent (QFocusEvent *event) |
bool | focusNextChild () |
virtual bool | focusNextPrevChild (bool next) |
virtual void | focusOutEvent (QFocusEvent *event) |
bool | focusPreviousChild () |
virtual void | hideEvent (QHideEvent *event) |
virtual void | initPainter (QPainter *painter) const const override |
virtual void | inputMethodEvent (QInputMethodEvent *event) |
virtual void | keyReleaseEvent (QKeyEvent *event) |
virtual void | leaveEvent (QEvent *event) |
virtual int | metric (PaintDeviceMetric m) const const override |
virtual void | mouseDoubleClickEvent (QMouseEvent *event) |
virtual void | mouseMoveEvent (QMouseEvent *event) |
virtual void | mousePressEvent (QMouseEvent *event) |
virtual void | mouseReleaseEvent (QMouseEvent *event) |
virtual void | moveEvent (QMoveEvent *event) |
virtual bool | nativeEvent (const QByteArray &eventType, void *message, qintptr *result) |
virtual void | paintEvent (QPaintEvent *event) |
virtual void | tabletEvent (QTabletEvent *event) |
void | updateMicroFocus (Qt::InputMethodQuery query) |
virtual void | wheelEvent (QWheelEvent *event) |
![]() | |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const QMetaMethod &signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const QMetaMethod &signal) |
bool | isSignalConnected (const QMetaMethod &signal) const const |
int | receivers (const char *signal) const const |
QObject * | sender () const const |
int | senderSignalIndex () const const |
virtual void | timerEvent (QTimerEvent *event) |
![]() |
Additional Inherited Members | |
![]() | |
Accepted | |
Rejected | |
![]() | |
DrawChildren | |
DrawWindowBackground | |
IgnoreMask | |
![]() | |
PdmDepth | |
PdmDevicePixelRatio | |
PdmDevicePixelRatioScaled | |
PdmDpiX | |
PdmDpiY | |
PdmHeight | |
PdmHeightMM | |
PdmNumColors | |
PdmPhysicalDpiX | |
PdmPhysicalDpiY | |
PdmWidth | |
PdmWidthMM | |
Detailed Description
A perceptually uniform color picker dialog.
The color dialog’s function is to allow users to choose colors intuitively. For example, you might use this in a drawing program to allow the user to set the brush color.
At difference to QColorDialog, this dialog’s graphical components are perceptually uniform and therefore more intuitive. It’s internally based on the LCH color model, which does reflect the human perception much better than RGB or its transforms like HSV. At the same time, this dialog does not require the user itself to know anything about LCH at all, because the graphical representations is intuitive.
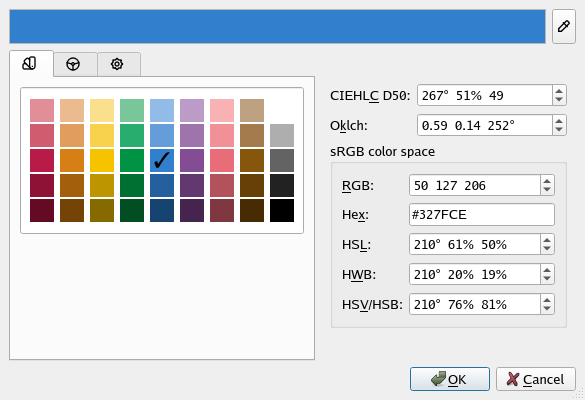
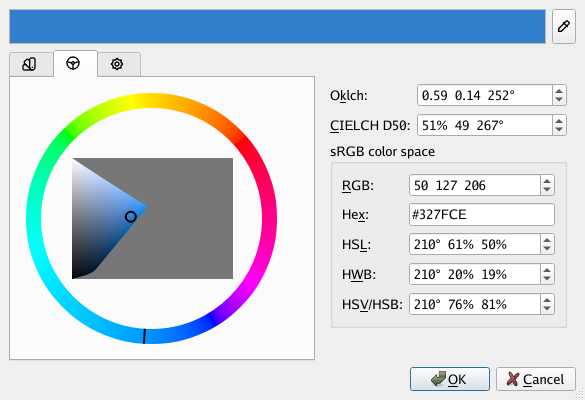
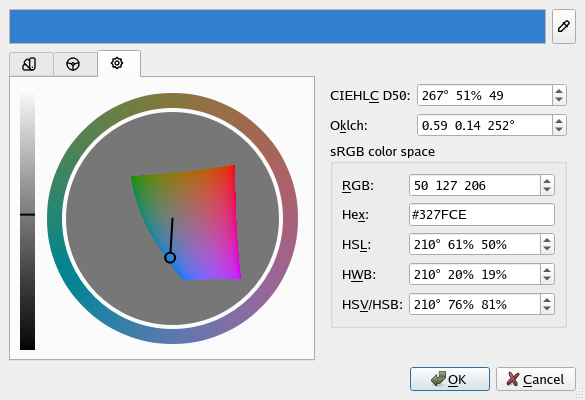
It is an mostly source-compatible replacement for QColorDialog. It also adds some extra functionality that is not available in QColorDialog.
Just as with QColorDialog, the static functions provide a modal color dialog. The static getColor() function shows the dialog, and allows the user to specify a color:
The function can also be used to let users choose a color with a level of transparency: pass the alpha channel option as an additional argument:
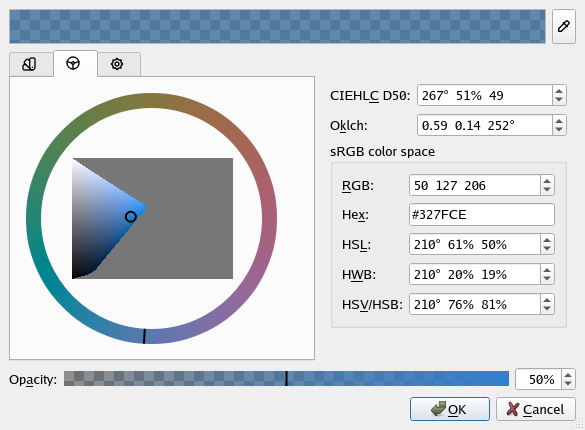
More features:
- A screen color picker is provided on many platforms.
- For a non-modal dialog, use the normal constructors of this class.
- The default window title is Select Color, and not the title of your application. It can of course be customized with
QWidget::setWindowTitle()
. The window title will not be updated onQEvent::LanguageChange
events. - At difference to the usual native platform color dialog, this dialog can be resized. That makes sense, because it allows to see better the gamut image. Therefore, this dialog is by default bigger than the usual native platform color dialog. You can of course customize the dialog size with QWidget::resize() or force a more space-saving layout through the layoutDimensions property.
- The ColorPatch that indicates the selected color is placed prominently at the top of the widget. That is also useful for touch screens as the ColorPatch will not be hidden by the hand of the user when the user is touching the above color selection widgets.
- This dialog uses icons. See High DPI support about how to enable support for high-DPI icons.
- Note
- The API of this class is mostly source-compatible to the API of QColorDialog. This is a list of incompatibilities:
- The constructors and also getColor() require a color space as argument.
- As this dialog does not provide functionality for custom colors and standard color, the corresponding static functions of QColorDialog are not available in this class.
- The option
ColorDialogOption::DontUseNativeDialog
will always remainfalse
(even if set explicitly), because it’s just the point of this library to provide an own, non-native dialog. - While the enum declaration ColorDialogOption itself is aliased here, this isn't possible for the enum values itself. Therefor, when working with KConfig Entry Options, you cannot use
ShowAlphaChannel
but you have to use the fully qualified identifier (eitherPerceptualColor::ColorDialog::ColorDialogOption::ShowAlphaChannel
orQColorDialog::ShowAlphaChannel
, at your option. - Calling setCurrentColor() with colors that are not
QColor::Spec::Rgb
will lead to an automatic conversion like QColorDialog does, but at difference to QColorDialog, it is done with more precision, therefor the resulting currentColor() might be slightly different. The same is true forQColor::Spec::Rgb
types with floating point precision: While QColorDialog would round to full integers, this dialog preserves the floating point precision. - When the default constructor is used, unlike QColorDialog, the default color is not guaranteed to be
Qt::white
.
Definition at line 295 of file colordialog.h.
Member Typedef Documentation
◆ ColorDialogOption
Local alias for QColorDialog::ColorDialogOption.
This type is declared as type to Qt’s type system via Q_DECLARE_METATYPE
. Depending on your use case (for example if you want to use for queued signal-slot connections), you might consider calling qRegisterMetaType()
for this type, once you have a QApplication object.
Definition at line 396 of file colordialog.h.
◆ ColorDialogOptions
Local alias for QColorDialog::ColorDialogOptions.
This type is declared as type to Qt’s type system via Q_DECLARE_METATYPE
. Depending on your use case (for example if you want to use for queued signal-slot connections), you might consider calling qRegisterMetaType()
for this type, once you have a QApplication object.
Definition at line 404 of file colordialog.h.
Member Enumeration Documentation
◆ DialogLayoutDimensions
|
strong |
Layout dimensions.
This enum is declared to the meta-object system with Q_ENUM
. This happens automatically. You do not need to make any manual calls.
This type is declared as type to Qt’s type system via Q_DECLARE_METATYPE
. Depending on your use case (for example if you want to use for queued signal-slot connections), you might consider calling qRegisterMetaType()
for this type, once you have a QApplication object.
Enumerator | |
---|---|
ScreenSizeDependent | Decide automatically between The decision is based on the screen size of the default screen of the widget (see |
Collapsed | Use the small, “collapsed“ layout of this dialog. |
Expanded | Use the large, “expanded” layout of this dialog.
|
Definition at line 415 of file colordialog.h.
Property Documentation
◆ currentColor
|
readwrite |
Currently selected color in the dialog.
- Invariant
- This property is provided as an RGB value.
QColor::isValid()
is alwaystrue
andQColor::spec()
is alwaysQColor::Spec::Rgb
. - The signal currentColorChanged() is emitted always and only when the value of this property changes.
- Note
- The setter setCurrentColor() does not accept all QColor values. See its documentation for details.
- See also
- READ currentColor() const
- WRITE setCurrentColor()
- NOTIFY currentColorChanged()
Definition at line 320 of file colordialog.h.
◆ layoutDimensions
|
readwrite |
Layout dimensions.
Defines if the dialog uses a rather collapsed (small) or a rather expanded (large) layout. In both cases, all elements are present. But for the collapsed variant, more elements are put in tab widgets, while for the expanded variant, more elements are visible at the same time.
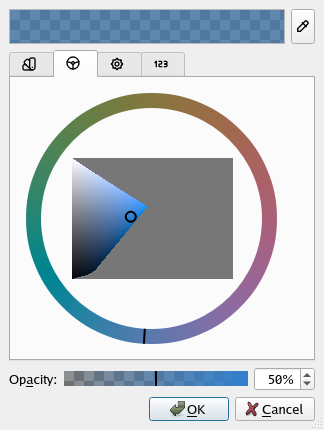
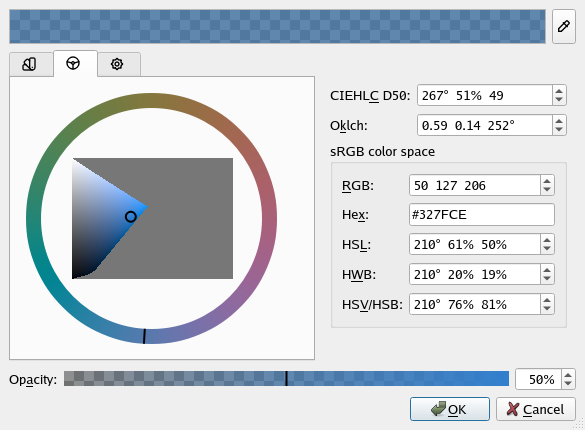
Default value:
When the layout dimension effectively changes, also the dialog size is adapted.
- See also
- DialogLayoutDimensions
- READ layoutDimensions() const
- WRITE setLayoutDimensions()
- NOTIFY layoutDimensionsChanged
Definition at line 352 of file colordialog.h.
◆ options
|
readwrite |
Various options that affect the look and feel of the dialog.
These are the same settings as for QColorDialog. For compatibility reasons, they are also of the same type: ColorDialogOptions
Option | Default value | Description |
---|---|---|
ShowAlphaChannel | false | Allow the user to select the alpha component of a color. |
NoButtons | false | Don't display OK and Cancel buttons. (Useful for “live dialogs”.) |
DontUseNativeDialog | true | Use Qt’s standard color dialog instead of the operating system native color dialog. |
- Invariant
- The option
ColorDialogOption::DontUseNativeDialog
will always betrue
because it’s just the point of this library to provide an own, non-native dialog. (If you setColorDialogOption::DontUseNativeDialog
explicitly tofalse
, this will silently be ignored, while the other options that you might have set, will be correctly applied.)
Example:
Or:
- Note
- At difference to QColorDialog, you need a fully qualified identifier for the enum values. The following code would therefore fail:
myDialog->setOption(ShowAlphaChannel, false);
- See also
- READ options() const
- testOption()
- WRITE setOptions()
- setOption()
- NOTIFY optionsChanged()
Definition at line 386 of file colordialog.h.
Constructor & Destructor Documentation
◆ ColorDialog() [1/4]
|
explicit |
Constructor.
- Parameters
-
parent pointer to the parent widget, if any
- Postcondition
- The currentColor property is set to a default value.
Definition at line 1294 of file colordialog.cpp.
◆ ColorDialog() [2/4]
|
explicit |
Constructor.
- Parameters
-
initial the initially chosen color of the dialog parent pointer to the parent widget, if any
- Postcondition
- The object is constructed and setCurrentColor() is called with initial. See setCurrentColor() for the modifications that will be applied before setting the current color. Especially, as this dialog is constructed by default without alpha support, the alpha channel of initial is ignored and a fully opaque color is used.
Definition at line 1312 of file colordialog.cpp.
◆ ColorDialog() [3/4]
|
explicit |
Constructor.
- Parameters
-
colorSpace The color space within which this widget should operate. Can be created with RgbColorSpaceFactory. parent pointer to the parent widget, if any
- Postcondition
- The currentColor property is set to a default value.
Definition at line 1331 of file colordialog.cpp.
◆ ColorDialog() [4/4]
|
explicit |
Constructor.
- Parameters
-
colorSpace The color space within which this widget should operate. Can be created with RgbColorSpaceFactory. initial the initially chosen color of the dialog parent pointer to the parent widget, if any
- Postcondition
- The object is constructed and setCurrentColor() is called with initial. See setCurrentColor() for the modifications that will be applied before setting the current color. Especially, as this dialog is constructed by default without alpha support, the alpha channel of initial is ignored and a fully opaque color is used.
Definition at line 1361 of file colordialog.cpp.
◆ ~ColorDialog()
|
overridevirtualnoexcept |
Destructor.
Definition at line 1375 of file colordialog.cpp.
Member Function Documentation
◆ changeEvent()
|
overrideprotectedvirtual |
Handle state changes.
Implements reaction on QEvent::LanguageChange
.
Reimplemented from base class.
- Parameters
-
event The event.
Reimplemented from QWidget.
Definition at line 2522 of file colordialog.cpp.
◆ colorSelected
|
signal |
This signal is emitted just after the user has clicked OK to select a color to use.
- Parameters
-
color the chosen color
◆ currentColor()
|
nodiscard |
Getter for property currentColor.
- Returns
- the property currentColor
Definition at line 1395 of file colordialog.cpp.
◆ currentColorChanged
|
signal |
Notify signal for property currentColor.
This signal is emitted whenever the “current color” changes in the dialog.
- Parameters
-
color the new “current color”
◆ done()
|
overrideprotectedvirtual |
Various updates when closing the dialog.
Reimplemented from base class.
- Parameters
-
result The result with which the dialog has been closed
Reimplemented from QDialog.
Definition at line 2371 of file colordialog.cpp.
◆ getColor() [1/2]
|
staticnodiscard |
Pops up a modal color dialog, lets the user choose a color, and returns that color.
- Parameters
-
initial initial value for currentColor() parent parent widget of the dialog (or 0 for no parent) title window title (or an empty string for the default window title) options the options() for customizing the look and feel of the dialog
- Returns
- selectedColor(): The color the user has selected; or an invalid color if the user has canceled the dialog.
Definition at line 2311 of file colordialog.cpp.
◆ getColor() [2/2]
|
staticnodiscard |
Pops up a modal color dialog, lets the user choose a color, and returns that color.
- Parameters
-
colorSpace The color space within which this widget should operate. initial initial value for currentColor() parent parent widget of the dialog (or 0 for no parent) title window title (or an empty string for the default window title) options the options() for customizing the look and feel of the dialog
- Returns
- selectedColor(): The color the user has selected; or an invalid color if the user has canceled the dialog.
Definition at line 2282 of file colordialog.cpp.
◆ layoutDimensions()
|
nodiscard |
Getter for property layoutDimensions.
- Returns
- the property layoutDimensions
Definition at line 2410 of file colordialog.cpp.
◆ layoutDimensionsChanged
|
signal |
Notify signal for property layoutDimensions.
- Parameters
-
newLayoutDimensions the new layout dimensions
◆ open() [1/2]
|
virtual |
Reimplemented from QDialog.
◆ open() [2/2]
void PerceptualColor::ColorDialog::open | ( | QObject * | receiver, |
const char * | member ) |
Opens the dialog and connects its colorSelected() signal to the slot specified by receiver and member.
The signal will be disconnected from the slot when the dialog is closed.
Example:
- Parameters
-
receiver the object that will receive the colorSelected() signal member the slot that will receive the colorSelected() signal
Definition at line 1449 of file colordialog.cpp.
◆ options()
|
nodiscard |
Getter for property KConfig Entry Options.
- Returns
- the current KConfig Entry Options
Definition at line 2208 of file colordialog.cpp.
◆ optionsChanged
|
signal |
Notify signal for property KConfig Entry Options.
- Parameters
-
newOptions the new options
◆ selectedColor()
|
nodiscard |
The color that was actually selected by the user.
At difference to the currentColor property, this function provides the color that was actually selected by the user by clicking the OK button or pressing the return key or another equivalent action.
This function most useful to get the actually selected color after that the dialog has been closed.
When a dialog that had been closed or hidden is shown again, this function returns to an invalid QColor().
- Returns
- Just after showing the dialog, the value is an invalid QColor. If the user selects a color by clicking the OK button or another equivalent action, the value is the selected color. If the user cancels the dialog (Cancel button, or by pressing the Escape key), the value remains an invalid QColor.
Definition at line 2337 of file colordialog.cpp.
◆ setCurrentColor
|
slot |
Setter for currentColor property.
- Parameters
-
color the new color
- Postcondition
- The property currentColor is adapted as follows:
- If color is not valid,
Qt::black
is used instead. - If color’s
QColor::Spec
is notQColor::Spec::Rgb
then it will be converted silently toQColor::Spec::Rgb
- The RGB part of currentColor will be the RGB part of
color
. - The alpha channel of currentColor will be the alpha channel of
color
if at the moment of the function call theQColorDialog::ColorDialogOption::ShowAlphaChannel
option is set. It will be fully opaque otherwise.
- If color is not valid,
Definition at line 1417 of file colordialog.cpp.
◆ setLayoutDimensions
|
slot |
Setter for property layoutDimensions.
- Parameters
-
newLayoutDimensions the new layout dimensions
Definition at line 2417 of file colordialog.cpp.
◆ setOption
|
slot |
Setter for KConfig Entry Options.
Sets a value for just one single option within KConfig Entry Options.
- Parameters
-
option the option to set on the new value of the option
Definition at line 2218 of file colordialog.cpp.
◆ setOptions
|
slot |
Setter for KConfig Entry Options.
- Parameters
-
newOptions the new options
- Postcondition
- All options of the widget have the same state (enabled/disabled) as in the given parameter.
Definition at line 2229 of file colordialog.cpp.
◆ setVisible()
|
overridevirtual |
Setter for property visible
Reimplemented from base class.
When a dialog, that wasn't formerly visible, gets visible, it’s selectedColor value is cleared.
- Parameters
-
visible holds whether or not the dialog should be visible
Reimplemented from QDialog.
Definition at line 2350 of file colordialog.cpp.
◆ showEvent()
|
overrideprotectedvirtual |
Handle show events.
Reimplemented from base class.
- Parameters
-
event The event.
Reimplemented from QDialog.
Definition at line 2574 of file colordialog.cpp.
◆ testOption()
|
nodiscard |
Getter for KConfig Entry Options.
Gets the value of just one single option within KConfig Entry Options.
- Parameters
-
option the requested option
- Returns
- the value of the requested option
Definition at line 2265 of file colordialog.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2024 The KDE developers.
Generated on Sat Dec 21 2024 16:57:18 by doxygen 1.12.0 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.