Phonon::Experimental::AudioDataOutput
#include <audiodataoutput.h>
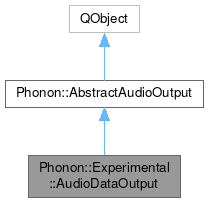
Public Types | |
enum | Channel { LeftChannel , RightChannel , CenterChannel , LeftSurroundChannel , RightSurroundChannel , SubwooferChannel } |
enum | Format { IntegerFormat = 1 , FloatFormat = 2 } |
Properties | |
int | dataSize |
Format | format |
![]() | |
objectName | |
Signals | |
void | dataReady (const QMap< Phonon::Experimental::AudioDataOutput::Channel, QVector< float > > &data) |
void | dataReady (const QMap< Phonon::Experimental::AudioDataOutput::Channel, QVector< qint16 > > &data) |
void | endOfMedia (int remainingSamples) |
Public Slots | |
void | setDataSize (int size) |
void | setFormat (Format format) |
Public Member Functions | |
int | dataSize () const |
Format | format () const |
int | sampleRate () const |
![]() | |
QObject (QObject *parent) | |
QBindable< QString > | bindableObjectName () |
bool | blockSignals (bool block) |
const QObjectList & | children () const const |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) const const |
bool | disconnect (const QObject *receiver, const char *method) const const |
void | dumpObjectInfo () const const |
void | dumpObjectTree () const const |
QList< QByteArray > | dynamicPropertyNames () const const |
virtual bool | event (QEvent *e) |
virtual bool | eventFilter (QObject *watched, QEvent *event) |
T | findChild (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QRegularExpression &re, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (Qt::FindChildOptions options) const const |
bool | inherits (const char *className) const const |
void | installEventFilter (QObject *filterObj) |
bool | isQuickItemType () const const |
bool | isWidgetType () const const |
bool | isWindowType () const const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const const |
void | moveToThread (QThread *targetThread) |
QString | objectName () const const |
void | objectNameChanged (const QString &objectName) |
QObject * | parent () const const |
QVariant | property (const char *name) const const |
Q_CLASSINFO (Name, Value) | |
Q_EMIT Q_EMIT | |
Q_ENUM (...) | |
Q_ENUM_NS (...) | |
Q_ENUMS (...) | |
Q_FLAG (...) | |
Q_FLAG_NS (...) | |
Q_FLAGS (...) | |
Q_GADGET Q_GADGET | |
Q_GADGET_EXPORT (EXPORT_MACRO) | |
Q_INTERFACES (...) | |
Q_INVOKABLE Q_INVOKABLE | |
Q_MOC_INCLUDE Q_MOC_INCLUDE | |
Q_NAMESPACE Q_NAMESPACE | |
Q_NAMESPACE_EXPORT (EXPORT_MACRO) | |
Q_OBJECT Q_OBJECT | |
Q_PROPERTY (...) | |
Q_REVISION Q_REVISION | |
Q_SET_OBJECT_NAME (Object) | |
Q_SIGNAL Q_SIGNAL | |
Q_SIGNALS Q_SIGNALS | |
Q_SLOT Q_SLOT | |
Q_SLOTS Q_SLOTS | |
T | qobject_cast (const QObject *object) |
T | qobject_cast (QObject *object) |
QT_NO_NARROWING_CONVERSIONS_IN_CONNECT QT_NO_NARROWING_CONVERSIONS_IN_CONNECT | |
void | removeEventFilter (QObject *obj) |
void | setObjectName (const QString &name) |
void | setObjectName (QAnyStringView name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | setProperty (const char *name, QVariant &&value) |
bool | signalsBlocked () const const |
int | startTimer (int interval, Qt::TimerType timerType) |
int | startTimer (std::chrono::milliseconds interval, Qt::TimerType timerType) |
QThread * | thread () const const |
Additional Inherited Members | |
![]() | |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *context, Functor functor, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, Functor functor) |
bool | disconnect (const QMetaObject::Connection &connection) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
bool | disconnect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
![]() | |
typedef | QObjectList |
![]() | |
AbstractAudioOutput (AbstractAudioOutputPrivate &dd, QObject *parent) | |
![]() | |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const QMetaMethod &signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const QMetaMethod &signal) |
bool | isSignalConnected (const QMetaMethod &signal) const const |
int | receivers (const char *signal) const const |
QObject * | sender () const const |
int | senderSignalIndex () const const |
virtual void | timerEvent (QTimerEvent *event) |
Detailed Description
This class gives you the audio data (for visualizations).
This class implements a special AbstractAudioOutput that gives your application the audio data. Don't expect realtime performance. But the latencies should be low enough to use the audio data for visualizations. You can also use the audio data for further processing (e.g. encoding and saving to a file).
The class supports different data formats. One of the most common formats is to read vectors of integers (which will only use 16 Bit), but you can also request floats which some backends use internally.
Definition at line 59 of file experimental/audiodataoutput.h.
Member Enumeration Documentation
◆ Channel
Specifies the channel the audio data belongs to.
Definition at line 71 of file experimental/audiodataoutput.h.
◆ Format
Used for telling the object whether you want 16 bit Integers or 32 bit floats.
- See also
- requestFormat
Definition at line 87 of file experimental/audiodataoutput.h.
Property Documentation
◆ dataSize
|
readwrite |
Definition at line 65 of file experimental/audiodataoutput.h.
◆ format
|
readwrite |
Definition at line 64 of file experimental/audiodataoutput.h.
Member Function Documentation
◆ dataReady [1/2]
|
signal |
Emitted whenever another dataSize number of samples are ready and format is set to FloatFormat.
If format is set to IntegerFormat the signal is not emitted at all.
- Parameters
-
data A mapping of Channel to a vector holding the audio data.
◆ dataReady [2/2]
|
signal |
Emitted whenever another dataSize number of samples are ready and format is set to IntegerFormat.
If format is set to FloatFormat the signal is not emitted at all.
- Parameters
-
data A mapping of Channel to a vector holding the audio data.
◆ dataSize()
int Phonon::Experimental::AudioDataOutput::dataSize | ( | ) | const |
Returns the currently used number of samples passed through the signal.
- See also
- setDataSize
◆ endOfMedia
|
signal |
This signal is emitted before the last dataReady signal of a media is emitted.
If, for example, the playback of a media file has finished and the last audio data of that file is going to be passed with the next dataReady signal, and only the 28 first samples of the data vector are from that media file endOfMedia will be emitted right before dataReady with remainingSamples
= 28.
- Parameters
-
remainingSamples The number of samples in the next dataReady vector that belong to the media that was playing to this point.
◆ format()
Format Phonon::Experimental::AudioDataOutput::format | ( | ) | const |
Returns the currently used format.
- See also
- setFormat
◆ sampleRate()
int Phonon::Experimental::AudioDataOutput::sampleRate | ( | ) | const |
Returns the sample rate in Hz.
Common sample rates are 44100 Hz and 48000 Hz. AudioDataOutput will not do any sample rate conversion for you. If you need to convert the sample rate you might want to take a look at libsamplerate. For visualizations it is often enough to do simple interpolation or even drop/duplicate samples.
- Returns
- The sample rate as reported by the backend. If the backend is unavailable -1 is returned.
◆ setDataSize
|
slot |
Sets the number of samples to be passed in one signal emission.
Defaults to 512 samples per emitted signal.
- Parameters
-
size the number of samples
◆ setFormat
|
slot |
Requests the dataformat you'd like to receive.
Only one of the signals of this class will be emitted when new data is ready.
The default format is IntegerFormat.
- See also
- format()
The documentation for this class was generated from the following file:
Documentation copyright © 1996-2025 The KDE developers.
Generated on Fri May 2 2025 12:04:30 by doxygen 1.13.2 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.