Phonon::MediaController
#include <phonon/MediaController>
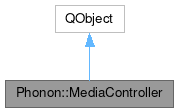
Public Types | |
enum | Feature { Angles = 1 , Chapters = 2 , Navigations = 3 , Titles = 4 , Subtitles = 5 , AudioChannels = 6 } |
typedef QFlags< Feature > | Features |
enum | NavigationMenu { RootMenu , TitleMenu , AudioMenu , SubtitleMenu , ChapterMenu , AngleMenu } |
Signals | |
void | angleChanged (int angleNumber) |
void | availableAnglesChanged (int availableAngles) |
void | availableAudioChannelsChanged () |
void | availableChaptersChanged (int availableChapters) |
void | availableMenusChanged (QList< NavigationMenu > menus) |
void | availableSubtitlesChanged () |
void | availableTitlesChanged (int availableTitles) |
void | chapterChanged (int chapterNumber) |
void | titleChanged (int titleNumber) |
Public Slots | |
void | nextTitle () |
void | previousTitle () |
void | setAutoplayTitles (bool) |
void | setCurrentAngle (int angleNumber) |
void | setCurrentChapter (int chapterNumber) |
void | setCurrentTitle (int titleNumber) |
Public Member Functions | |
MediaController (MediaObject *parent) | |
bool | autoplayTitles () const |
int | availableAngles () const |
QList< Phonon::AudioChannelDescription > | availableAudioChannels () const |
int | availableChapters () const |
QList< NavigationMenu > | availableMenus () const |
QList< SubtitleDescription > | availableSubtitles () const |
int | availableTitles () const |
int | currentAngle () const |
AudioChannelDescription | currentAudioChannel () const |
int | currentChapter () const |
SubtitleDescription | currentSubtitle () const |
int | currentTitle () const |
void | setCurrentAudioChannel (const Phonon::AudioChannelDescription &stream) |
void | setCurrentMenu (NavigationMenu menu) |
void | setCurrentSubtitle (const Phonon::SubtitleDescription &stream) |
void | setCurrentSubtitle (const QUrl &url) |
void | setSubtitleAutodetect (bool enable) |
void | setSubtitleEncoding (const QString &encoding) |
void | setSubtitleFont (const QFont &font) |
bool | subtitleAutodetect () const |
QString | subtitleEncoding () const |
QFont | subtitleFont () const |
Features | supportedFeatures () const |
![]() | |
QObject (QObject *parent) | |
QBindable< QString > | bindableObjectName () |
bool | blockSignals (bool block) |
const QObjectList & | children () const const |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) const const |
bool | disconnect (const QObject *receiver, const char *method) const const |
void | dumpObjectInfo () const const |
void | dumpObjectTree () const const |
QList< QByteArray > | dynamicPropertyNames () const const |
virtual bool | event (QEvent *e) |
virtual bool | eventFilter (QObject *watched, QEvent *event) |
T | findChild (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QRegularExpression &re, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (Qt::FindChildOptions options) const const |
bool | inherits (const char *className) const const |
void | installEventFilter (QObject *filterObj) |
bool | isQuickItemType () const const |
bool | isWidgetType () const const |
bool | isWindowType () const const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const const |
void | moveToThread (QThread *targetThread) |
QString | objectName () const const |
void | objectNameChanged (const QString &objectName) |
QObject * | parent () const const |
QVariant | property (const char *name) const const |
Q_CLASSINFO (Name, Value) | |
Q_EMIT Q_EMIT | |
Q_ENUM (...) | |
Q_ENUM_NS (...) | |
Q_ENUMS (...) | |
Q_FLAG (...) | |
Q_FLAG_NS (...) | |
Q_FLAGS (...) | |
Q_GADGET Q_GADGET | |
Q_GADGET_EXPORT (EXPORT_MACRO) | |
Q_INTERFACES (...) | |
Q_INVOKABLE Q_INVOKABLE | |
Q_MOC_INCLUDE Q_MOC_INCLUDE | |
Q_NAMESPACE Q_NAMESPACE | |
Q_NAMESPACE_EXPORT (EXPORT_MACRO) | |
Q_OBJECT Q_OBJECT | |
Q_PROPERTY (...) | |
Q_REVISION Q_REVISION | |
Q_SET_OBJECT_NAME (Object) | |
Q_SIGNAL Q_SIGNAL | |
Q_SIGNALS Q_SIGNALS | |
Q_SLOT Q_SLOT | |
Q_SLOTS Q_SLOTS | |
T | qobject_cast (const QObject *object) |
T | qobject_cast (QObject *object) |
QT_NO_NARROWING_CONVERSIONS_IN_CONNECT QT_NO_NARROWING_CONVERSIONS_IN_CONNECT | |
void | removeEventFilter (QObject *obj) |
void | setObjectName (const QString &name) |
void | setObjectName (QAnyStringView name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | setProperty (const char *name, QVariant &&value) |
bool | signalsBlocked () const const |
int | startTimer (int interval, Qt::TimerType timerType) |
int | startTimer (std::chrono::milliseconds interval, Qt::TimerType timerType) |
QThread * | thread () const const |
Static Public Member Functions | |
static QString | navigationMenuToString (NavigationMenu menu) |
![]() | |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *context, Functor functor, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, Functor functor) |
bool | disconnect (const QMetaObject::Connection &connection) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
bool | disconnect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
Protected Attributes | |
MediaControllerPrivate *const | d |
Additional Inherited Members | |
![]() | |
objectName | |
![]() | |
typedef | QObjectList |
![]() | |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const QMetaMethod &signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const QMetaMethod &signal) |
bool | isSignalConnected (const QMetaMethod &signal) const const |
int | receivers (const char *signal) const const |
QObject * | sender () const const |
int | senderSignalIndex () const const |
virtual void | timerEvent (QTimerEvent *event) |
Detailed Description
Controls optional features of a media file/device like title, chapter, angle.
Definition at line 47 of file mediacontroller.h.
Member Typedef Documentation
◆ Features
typedef QFlags< Feature > Phonon::MediaController::Features |
Definition at line 84 of file mediacontroller.h.
Member Enumeration Documentation
◆ Feature
Definition at line 52 of file mediacontroller.h.
◆ NavigationMenu
Enumerator | |
---|---|
TitleMenu | < Root/main menu. |
AudioMenu | < Title Menu to access different titles on the media source. The title menu is usually where one would select the episode of a TV series DVD. It can be equal to the main menu but does not need to be. |
SubtitleMenu | < Audio menu for language (and sometimes also subtitle) settings etc. |
ChapterMenu | < Subtitle menu. Usually this represents the same menu as AudioMenu or is not present at all (in which case subtitle settings are probably also in the AudioMenu). |
AngleMenu | < Chapter menu for chapter selection. < Angle menu. Rarely supported on any media source. |
Definition at line 86 of file mediacontroller.h.
Constructor & Destructor Documentation
◆ MediaController()
Phonon::MediaController::MediaController | ( | MediaObject * | parent | ) |
Definition at line 52 of file mediacontroller.cpp.
◆ ~MediaController()
|
override |
Definition at line 73 of file mediacontroller.cpp.
Member Function Documentation
◆ autoplayTitles()
bool Phonon::MediaController::autoplayTitles | ( | ) | const |
Definition at line 217 of file mediacontroller.cpp.
◆ availableAngles()
int Phonon::MediaController::availableAngles | ( | ) | const |
Definition at line 112 of file mediacontroller.cpp.
◆ availableAudioChannels()
QList< AudioChannelDescription > Phonon::MediaController::availableAudioChannels | ( | ) | const |
Returns the audio streams that can be selected by the user.
The strings can directly be used in the user interface.
- See also
- selectedAudioChannel
- setCurrentAudioChannel
Definition at line 306 of file mediacontroller.cpp.
◆ availableChapters()
int Phonon::MediaController::availableChapters | ( | ) | const |
Definition at line 135 of file mediacontroller.cpp.
◆ availableMenus()
QList< MediaController::NavigationMenu > Phonon::MediaController::availableMenus | ( | ) | const |
Get the list of currently available menus for the present media source.
The list is always ordered by occurrence in the NavgiationMenu enum. Should you wish to use a different order in your application you will have to make appropriate changes.
- Returns
- list of available menus (supported by backend and media source).
- See also
- navigationMenuToString()
Definition at line 177 of file mediacontroller.cpp.
◆ availableMenusChanged
|
signal |
The available menus changed, this for example emitted when Phonon switches from a media source without menus to one with menus (e.g.
a DVD).
- Parameters
-
menus is a list of all currently available menus, you should update GUI representations of the available menus with the new set.
◆ availableSubtitles()
QList< SubtitleDescription > Phonon::MediaController::availableSubtitles | ( | ) | const |
Returns the subtitle streams that can be selected by the user.
The strings can directly be used in the user interface.
- See also
- selectedSubtitle
- setCurrentSubtitle
Definition at line 315 of file mediacontroller.cpp.
◆ availableTitles()
int Phonon::MediaController::availableTitles | ( | ) | const |
Definition at line 196 of file mediacontroller.cpp.
◆ currentAngle()
int Phonon::MediaController::currentAngle | ( | ) | const |
Definition at line 119 of file mediacontroller.cpp.
◆ currentAudioChannel()
AudioChannelDescription Phonon::MediaController::currentAudioChannel | ( | ) | const |
Returns the selected audio stream.
Definition at line 243 of file mediacontroller.cpp.
◆ currentChapter()
int Phonon::MediaController::currentChapter | ( | ) | const |
Definition at line 142 of file mediacontroller.cpp.
◆ currentSubtitle()
SubtitleDescription Phonon::MediaController::currentSubtitle | ( | ) | const |
Returns the selected subtitle stream.
- See also
- availableSubtitles
- setCurrentSubtitle
Definition at line 299 of file mediacontroller.cpp.
◆ currentTitle()
int Phonon::MediaController::currentTitle | ( | ) | const |
Definition at line 203 of file mediacontroller.cpp.
◆ navigationMenuToString()
|
static |
Translates a NavigationMenu enum to a string you can use in your GUI.
Please note that keyboard shortucts will not be present in the returned String, therefore it is probably not a good idea to use this function if you are providing keyboard shortcuts for every other clickable.
Please note that RootMenu has the string representation "Main Menu" as root is a rather technical term when talking about menus.
Example:
- Returns
- the QString representation of the menu
Definition at line 158 of file mediacontroller.cpp.
◆ nextTitle
|
slot |
Skips to the next title.
If it was playing before the title change it will start playback on the next title if autoplayTitles is enabled.
Definition at line 231 of file mediacontroller.cpp.
◆ previousTitle
|
slot |
Skips to the previous title.
If it was playing before the title change it will start playback on the previous title if autoplayTitles is enabled.
Definition at line 236 of file mediacontroller.cpp.
◆ setAutoplayTitles
|
slot |
Definition at line 224 of file mediacontroller.cpp.
◆ setCurrentAngle
|
slot |
Definition at line 126 of file mediacontroller.cpp.
◆ setCurrentAudioChannel()
void Phonon::MediaController::setCurrentAudioChannel | ( | const Phonon::AudioChannelDescription & | stream | ) |
Selects an audio stream from the media.
Some media formats allow multiple audio streams to be stored in the same file. Normally only one should be played back.
- Parameters
-
stream Description of an audio stream
Definition at line 325 of file mediacontroller.cpp.
◆ setCurrentChapter
|
slot |
Definition at line 149 of file mediacontroller.cpp.
◆ setCurrentMenu()
void Phonon::MediaController::setCurrentMenu | ( | NavigationMenu | menu | ) |
Switches to a menu (e.g.
on a DVD).
- See also
- availableMenus()
Definition at line 188 of file mediacontroller.cpp.
◆ setCurrentSubtitle() [1/2]
void Phonon::MediaController::setCurrentSubtitle | ( | const Phonon::SubtitleDescription & | stream | ) |
Selects a subtitle stream from the media.
Some media formats allow multiple subtitle streams to be stored in the same file. Normally only one should be displayed.
- Parameters
-
stream description of a subtitle stream
Definition at line 332 of file mediacontroller.cpp.
◆ setCurrentSubtitle() [2/2]
void Phonon::MediaController::setCurrentSubtitle | ( | const QUrl & | url | ) |
Selects a subtitle file as subtitle source for the media.
- Note
- The file will also be added to the model of SubtitleDescriptions, so you do not need special handling in the UI.
Definition at line 339 of file mediacontroller.cpp.
◆ setCurrentTitle
|
slot |
Skips to the given title titleNumber
.
If it was playing before the title change it will start playback on the new title if autoplayTitles is enabled.
Definition at line 210 of file mediacontroller.cpp.
◆ setSubtitleAutodetect()
void Phonon::MediaController::setSubtitleAutodetect | ( | bool | enable | ) |
Sets/Unsets subtitles autodetection.
Detection is attempted when moving the MediaObject into Playing state. In order to enable/disable autodetection it must be set before play() is called. Whether a MediaSource is set on the MediaObject does not matter, and once detection is set it will remain set that way for this exact combination of MediaController and MediaObject.
- Note
- The subtitle autodetection may only be changed in states other than Playing | Buffering | Paused.
- See also
- subtitleAutodetect
- Since
- 4.7.0
Definition at line 257 of file mediacontroller.cpp.
◆ setSubtitleEncoding()
void Phonon::MediaController::setSubtitleEncoding | ( | const QString & | encoding | ) |
Selects the current encoding used to render subtitles.
The encoding name must respect the Link text IANA character-sets encoding file If no encoding is explicitly set, it defaults to UTF-8.
- Note
- The subtitle encoding may only be changed in states other than Playing | Buffering | Paused.
- Decoding support may vary between backends.
- See also
- subtitleEncoding
- Since
- 4.7.0
Definition at line 271 of file mediacontroller.cpp.
◆ setSubtitleFont()
void Phonon::MediaController::setSubtitleFont | ( | const QFont & | font | ) |
Selects the current font used to render subtitles.
If no font is explicitly set, the system default font is used.
- Note
- The subtitle font may only be changed in states other than Playing | Buffering | Paused.
- Non-system fonts can not be used. In particular adding fonts manually to the QFontDatabase will not make them available as render fonts.
- See also
- subtitleFont
- Since
- 4.7.0
Definition at line 285 of file mediacontroller.cpp.
◆ subtitleAutodetect()
bool Phonon::MediaController::subtitleAutodetect | ( | ) | const |
Subtitle auto-detection transparently tries to find a subtitle file for the current MediaSource and will automatically select a possible match.
Detected subtitles are added to the regular subtitle descriptions, allowing the user to deactivate it manually or switch to another detected file.
Matching method depends on the backend in use and may either be driven by a backend or even subsystem implementation. Consequently different backends may not give the same results. At any rate all algorithms are supposed to give as accurate as possible matches. Should you require reproducible matching across backends you should deactivate auto-detection entirely and instead do the lookup yourself and set the desired file using setCurrentSubtitle(QUrl); the file will still be added to the subtitledescriptions model.
- Note
- Auto-detection is always activate so long as the backend supports it.
- Returns
true
if subtitles are autodetected, otherwisefalse
is returned.
- See also
- setSubtitleAutodetect
- Since
- 4.7.0
Definition at line 250 of file mediacontroller.cpp.
◆ subtitleEncoding()
QString Phonon::MediaController::subtitleEncoding | ( | ) | const |
Returns the encoding used to render subtitles.
- See also
- setSubtitleEncoding
- Since
- 4.7.0
Definition at line 264 of file mediacontroller.cpp.
◆ subtitleFont()
QFont Phonon::MediaController::subtitleFont | ( | ) | const |
Returns the font used to render subtitles.
- See also
- setSubtitleFont
- QApplication::setFont
- Since
- 4.7.0
Definition at line 292 of file mediacontroller.cpp.
◆ supportedFeatures()
MediaController::Features Phonon::MediaController::supportedFeatures | ( | ) | const |
Definition at line 82 of file mediacontroller.cpp.
Member Data Documentation
◆ d
|
protected |
Definition at line 372 of file mediacontroller.h.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2024 The KDE developers.
Generated on Fri Jul 26 2024 11:50:46 by doxygen 1.11.0 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.