ContainmentItem
#include <containmentitem.h>
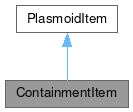
Properties | |
bool | loading |
WallpaperItem * | wallpaper |
![]() | |
QRect | availableScreenRect |
QVariantList | availableScreenRegion |
bool | hideOnWindowDeactivate |
int | screen |
QRect | screenGeometry |
QQuickItem * | toolTipItem |
QString | toolTipMainText |
QString | toolTipSubText |
int | toolTipTextFormat |
Signals | |
void | actionsChanged () |
void | appletsChanged () |
void | drawWallpaperChanged () |
void | editModeChanged () |
void | isLoadingChanged () |
void | wallpaperItemChanged () |
![]() | |
void | availableScreenRectChanged () |
void | availableScreenRegionChanged () |
void | contextualActionsAboutToShow () |
void | contextualActionsChanged () |
void | externalData (const QString &mimetype, const QVariant &data) |
void | hideOnWindowDeactivateChanged () |
void | screenChanged () |
void | screenGeometryChanged () |
void | toolTipItemChanged () |
void | toolTipMainTextChanged () |
void | toolTipSubTextChanged () |
void | toolTipTextFormatChanged () |
Protected Member Functions | |
void | addAppletActions (QMenu *desktopMenu, Plasma::Applet *applet, QEvent *event) |
void | addContainmentActions (QMenu *desktopMenu, QEvent *event) |
void | init () override |
bool | isLoading () const |
void | itemChange (ItemChange change, const ItemChangeData &value) override |
void | keyPressEvent (QKeyEvent *event) override |
void | loadWallpaper () |
void | mousePressEvent (QMouseEvent *event) override |
void | mouseReleaseEvent (QMouseEvent *event) override |
void | wheelEvent (QWheelEvent *event) override |
![]() | |
bool | event (QEvent *event) override |
bool | eventFilter (QObject *watched, QEvent *event) override |
void | init () override |
Detailed Description
This class is exposed to containments QML as the attached property Plasmoid.
Import Statement
- Version
- 2.0
Definition at line 35 of file containmentitem.h.
Property Documentation
◆ loading
|
read |
True if the UI is still loading, for instance a desktop which doesn't have its wallpaper yet.
Definition at line 44 of file containmentitem.h.
◆ wallpaper
|
read |
Definition at line 39 of file containmentitem.h.
Constructor & Destructor Documentation
◆ ContainmentItem()
ContainmentItem::ContainmentItem | ( | QQuickItem * | parent = nullptr | ) |
Definition at line 48 of file containmentitem.cpp.
Member Function Documentation
◆ addAppletActions()
|
protected |
Definition at line 966 of file containmentitem.cpp.
◆ addContainmentActions()
Definition at line 1011 of file containmentitem.cpp.
◆ adjustToAvailableScreenRegion()
QPointF ContainmentItem::adjustToAvailableScreenRegion | ( | int | x, |
int | y, | ||
int | w, | ||
int | h ) const |
Given a geometry, it adjusts it moving it completely inside of the boundaries of availableScreenRegion.
- Returns
- the toLeft point of the rectangle
Definition at line 212 of file containmentitem.cpp.
◆ classBegin()
|
overridevirtual |
Reimplemented from QQuickItem.
Definition at line 56 of file containmentitem.cpp.
◆ containment()
|
inline |
Definition at line 52 of file containmentitem.h.
◆ containmentItemAt()
QObject * ContainmentItem::containmentItemAt | ( | int | x, |
int | y ) |
Search for a containment at those coordinates.
the coordinates are passed as local coordinates of this containment
Definition at line 158 of file containmentitem.cpp.
◆ init()
|
overrideprotected |
Definition at line 71 of file containmentitem.cpp.
◆ isLoading()
|
protected |
Definition at line 1055 of file containmentitem.cpp.
◆ itemChange()
|
overrideprotectedvirtual |
Reimplemented from QQuickItem.
Definition at line 1060 of file containmentitem.cpp.
◆ itemFor()
PlasmaQuick::AppletQuickItem * ContainmentItem::itemFor | ( | Plasma::Applet * | applet | ) | const |
Returns the corresponding PlasmoidItem of one of its applets.
Definition at line 128 of file containmentitem.cpp.
◆ keyPressEvent()
|
overrideprotectedvirtual |
Reimplemented from QQuickItem.
Definition at line 946 of file containmentitem.cpp.
◆ loadWallpaper()
|
protected |
Definition at line 720 of file containmentitem.cpp.
◆ mapFromApplet()
QPointF ContainmentItem::mapFromApplet | ( | Plasma::Applet * | applet, |
int | x, | ||
int | y ) |
Map coordinates from relative to the given applet to relative to this containment.
Definition at line 182 of file containmentitem.cpp.
◆ mapToApplet()
QPointF ContainmentItem::mapToApplet | ( | Plasma::Applet * | applet, |
int | x, | ||
int | y ) |
Map coordinates from relative to this containment to relative to the given applet.
Definition at line 196 of file containmentitem.cpp.
◆ mousePressEvent()
|
overrideprotectedvirtual |
Reimplemented from QQuickItem.
Definition at line 777 of file containmentitem.cpp.
◆ mouseReleaseEvent()
|
overrideprotectedvirtual |
Reimplemented from QQuickItem.
Definition at line 772 of file containmentitem.cpp.
◆ openContextMenu()
void ContainmentItem::openContextMenu | ( | const QPointF & | globalPos | ) |
Opens the context menu of the Corona.
- Parameters
-
globalPos menu position in the global coordinate system
- Since
- 5.102
Definition at line 324 of file containmentitem.cpp.
◆ processMimeData() [1/2]
void ContainmentItem::processMimeData | ( | QMimeData * | data, |
int | x, | ||
int | y, | ||
KIO::DropJob * | dropJob = nullptr ) |
Process the mime data arrived to a particular coordinate, either with a drag and drop or paste with middle mouse button.
Definition at line 344 of file containmentitem.cpp.
◆ processMimeData() [2/2]
void ContainmentItem::processMimeData | ( | QObject * | data, |
int | x, | ||
int | y, | ||
KIO::DropJob * | dropJob = nullptr ) |
Process the mime data arrived to a particular coordinate, either with a drag and drop or paste with middle mouse button.
Definition at line 334 of file containmentitem.cpp.
◆ wallpaperItem()
|
inline |
Definition at line 57 of file containmentitem.h.
◆ wheelEvent()
|
overrideprotectedvirtual |
Reimplemented from QQuickItem.
Definition at line 918 of file containmentitem.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2025 The KDE developers.
Generated on Fri May 2 2025 11:55:47 by doxygen 1.13.2 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.