Plasma::Theme
#include <Plasma/Theme>
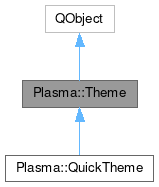
Public Types | |
enum | ColorGroup { NormalColorGroup = 0 , ButtonColorGroup = 1 , ViewColorGroup = 2 , ComplementaryColorGroup = 3 , HeaderColorGroup , ToolTipColorGroup } |
enum | ColorRole { TextColor = 0 , BackgroundColor = 1 , HighlightColor = 2 , HoverColor = 3 , FocusColor = 4 , LinkColor = 5 , VisitedLinkColor = 6 , HighlightedTextColor = 7 , PositiveTextColor = 8 , NeutralTextColor = 9 , NegativeTextColor = 10 , DisabledTextColor = 11 } |
Properties | |
qreal | backgroundContrast |
qreal | backgroundIntensity |
qreal | backgroundSaturation |
QFont | defaultFont |
QPalette | palette |
QFont | smallestFont |
QString | themeName |
bool | useGlobalSettings |
QString | wallpaperPath |
![]() | |
objectName | |
Signals | |
void | defaultFontChanged () |
void | smallestFontChanged () |
void | themeChanged () |
Public Member Functions | |
Theme (const QString &themeName, QObject *parent=nullptr) | |
Theme (QObject *parent=nullptr) | |
bool | adaptiveTransparencyEnabled () const |
qreal | backgroundContrast () const |
bool | backgroundContrastEnabled () const |
qreal | backgroundIntensity () const |
QString | backgroundPath (const QString &image) const |
qreal | backgroundSaturation () const |
bool | blurBehindEnabled () const |
QColor | color (ColorRole role, ColorGroup group=NormalColorGroup) const |
KSharedConfigPtr | colorScheme () const |
bool | currentThemeHasImage (const QString &name) const |
QFont | defaultFont () const |
QString | imagePath (const QString &name) const |
KPluginMetaData | metadata () const |
Q_INVOKABLE QSizeF | mSize (const QFont &font=QGuiApplication::font()) const |
QPalette | palette () const |
void | setThemeName (const QString &themeName) |
void | setUseGlobalSettings (bool useGlobal) |
QFont | smallestFont () const |
QString | themeName () const |
bool | useGlobalSettings () const |
QString | wallpaperPath (const QSize &size=QSize()) const |
Q_INVOKABLE QString | wallpaperPathForSize (int width=-1, int height=-1) const |
![]() | |
QObject (QObject *parent) | |
QBindable< QString > | bindableObjectName () |
bool | blockSignals (bool block) |
const QObjectList & | children () const const |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) const const |
bool | disconnect (const QObject *receiver, const char *method) const const |
void | dumpObjectInfo () const const |
void | dumpObjectTree () const const |
QList< QByteArray > | dynamicPropertyNames () const const |
virtual bool | event (QEvent *e) |
virtual bool | eventFilter (QObject *watched, QEvent *event) |
T | findChild (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QRegularExpression &re, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (Qt::FindChildOptions options) const const |
bool | inherits (const char *className) const const |
void | installEventFilter (QObject *filterObj) |
bool | isQuickItemType () const const |
bool | isWidgetType () const const |
bool | isWindowType () const const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const const |
void | moveToThread (QThread *targetThread) |
QString | objectName () const const |
void | objectNameChanged (const QString &objectName) |
QObject * | parent () const const |
QVariant | property (const char *name) const const |
Q_CLASSINFO (Name, Value) | |
Q_EMIT Q_EMIT | |
Q_ENUM (...) | |
Q_ENUM_NS (...) | |
Q_ENUMS (...) | |
Q_FLAG (...) | |
Q_FLAG_NS (...) | |
Q_FLAGS (...) | |
Q_GADGET Q_GADGET | |
Q_GADGET_EXPORT (EXPORT_MACRO) | |
Q_INTERFACES (...) | |
Q_INVOKABLE Q_INVOKABLE | |
Q_MOC_INCLUDE Q_MOC_INCLUDE | |
Q_NAMESPACE Q_NAMESPACE | |
Q_NAMESPACE_EXPORT (EXPORT_MACRO) | |
Q_OBJECT Q_OBJECT | |
Q_PROPERTY (...) | |
Q_REVISION Q_REVISION | |
Q_SET_OBJECT_NAME (Object) | |
Q_SIGNAL Q_SIGNAL | |
Q_SIGNALS Q_SIGNALS | |
Q_SLOT Q_SLOT | |
Q_SLOTS Q_SLOTS | |
T | qobject_cast (const QObject *object) |
T | qobject_cast (QObject *object) |
QT_NO_NARROWING_CONVERSIONS_IN_CONNECT QT_NO_NARROWING_CONVERSIONS_IN_CONNECT | |
void | removeEventFilter (QObject *obj) |
void | setObjectName (const QString &name) |
void | setObjectName (QAnyStringView name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | setProperty (const char *name, QVariant &&value) |
bool | signalsBlocked () const const |
int | startTimer (int interval, Qt::TimerType timerType) |
int | startTimer (std::chrono::milliseconds interval, Qt::TimerType timerType) |
QThread * | thread () const const |
Static Public Member Functions | |
static KSharedConfigPtr | globalColorScheme () |
static QPalette | globalPalette () |
![]() | |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *context, Functor functor, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, Functor functor) |
bool | disconnect (const QMetaObject::Connection &connection) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
bool | disconnect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
Additional Inherited Members | |
![]() | |
typedef | QObjectList |
![]() | |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const QMetaMethod &signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const QMetaMethod &signal) |
bool | isSignalConnected (const QMetaMethod &signal) const const |
int | receivers (const char *signal) const const |
QObject * | sender () const const |
int | senderSignalIndex () const const |
virtual void | timerEvent (QTimerEvent *event) |
Detailed Description
Interface to the Plasma theme.
Plasma::Theme provides access to a common and standardized set of graphic elements stored in SVG format. This allows artists to create single packages of SVGs that will affect the look and feel of all workspace components.
Plasma::Svg uses Plasma::Theme internally to locate and load the appropriate SVG data. Alternatively, Plasma::Theme can be used directly to retrieve file system paths to SVGs by name.
Member Enumeration Documentation
◆ ColorGroup
◆ ColorRole
Property Documentation
◆ backgroundContrast
◆ backgroundIntensity
◆ backgroundSaturation
◆ defaultFont
◆ palette
◆ smallestFont
◆ themeName
◆ useGlobalSettings
◆ wallpaperPath
Constructor & Destructor Documentation
◆ Theme() [1/2]
|
explicit |
◆ Theme() [2/2]
◆ ~Theme()
Member Function Documentation
◆ adaptiveTransparencyEnabled()
bool Plasma::Theme::adaptiveTransparencyEnabled | ( | ) | const |
This method allows Plasma to enable and disable the adaptive transparency option of the panel, which allows user to decide whether the panel should be always transparent, always opaque or only opaque when a window is maximized.
The configuration in the metadata.desktop file of the theme could look like this (for a lighter background):
- Returns
- Whether or not to enable the adaptive transparency
- Since
- 5.20
◆ backgroundContrast()
qreal Plasma::Theme::backgroundContrast | ( | ) | const |
This method allows Plasma to set a background contrast effect for a given theme, improving readability.
The value is read from the "contrast" key in the "ContrastEffect" group in the Theme's metadata file.
- Returns
- The contrast provided to the contrasteffect
- Since
- 5.0
- See also
- backgroundContrastEnabled
◆ backgroundContrastEnabled()
bool Plasma::Theme::backgroundContrastEnabled | ( | ) | const |
This method allows Plasma to enable and disable the background contrast effect for a given theme, improving readability.
The value is read from the "enabled" key in the "ContrastEffect" group in the Theme's metadata file. The configuration in the metadata.desktop file of the theme could look like this (for a lighter background):
- Returns
- Whether or not to enable the contrasteffect
- Since
- 5.0
◆ backgroundIntensity()
qreal Plasma::Theme::backgroundIntensity | ( | ) | const |
This method allows Plasma to set a background contrast effect for a given theme, improving readability.
The value is read from the "intensity" key in the "ContrastEffect" group in the Theme's metadata file.
- Returns
- The intensity provided to the contrasteffect
- Since
- 5.0
- See also
- backgroundContrastEnabled
◆ backgroundPath()
◆ backgroundSaturation()
qreal Plasma::Theme::backgroundSaturation | ( | ) | const |
This method allows Plasma to set a background contrast effect for a given theme, improving readability.
The value is read from the "saturation" key in the "ContrastEffect" group in the Theme's metadata file.
- Returns
- The saturation provided to the contrasteffect
- Since
- 5.0
- See also
- backgroundContrastEnabled
◆ blurBehindEnabled()
bool Plasma::Theme::blurBehindEnabled | ( | ) | const |
This method allows Plasma to enable and disable the blurring of what is behind the background for a given theme.
The value is read from the "enabled" key in the "BlurBehindEffect" group in the Theme's metadata file. Default is true
.
The configuration in the metadata.desktop file of the theme could look like this:
- Returns
- Whether or not to enable blurring of what is behind
- Since
- 5.57
◆ color()
◆ colorScheme()
KSharedConfigPtr Plasma::Theme::colorScheme | ( | ) | const |
Returns the color scheme configurationthat goes along this theme.
This can be used with KStatefulBrush and KColorScheme to determine the proper colours to use along with the visual elements in this theme.
◆ currentThemeHasImage()
bool Plasma::Theme::currentThemeHasImage | ( | const QString & | name | ) | const |
◆ defaultFont()
QFont Plasma::Theme::defaultFont | ( | ) | const |
◆ defaultFontChanged
|
signal |
Notifier for change of defaultFont property.
◆ globalColorScheme()
|
static |
◆ globalPalette()
◆ imagePath()
◆ metadata()
KPluginMetaData Plasma::Theme::metadata | ( | ) | const |
◆ mSize()
QSizeF Plasma::Theme::mSize | ( | const QFont & | font = QGuiApplication::font() | ) | const |
Returns the size of the letter "M" as rendered on the screen with the given font.
This values gives you a base size that:
- scales dependent on the DPI of the screen
- Scales with the default font as set by the user You can use it like this in QML Items: This allows you to dynamically scale elements of your user interface with different font settings and different physical outputs (with different DPI).
- Parameters
-
font The font to use for the metrics.
- Returns
- The size of the letter "M" as rendered on the screen with the given font.
- Since
- 5.0
◆ palette()
QPalette Plasma::Theme::palette | ( | ) | const |
◆ setThemeName()
void Plasma::Theme::setThemeName | ( | const QString & | themeName | ) |
◆ setUseGlobalSettings()
void Plasma::Theme::setUseGlobalSettings | ( | bool | useGlobal | ) |
◆ smallestFont()
QFont Plasma::Theme::smallestFont | ( | ) | const |
◆ smallestFontChanged
|
signal |
Notifier for change of smallestFont property.
◆ themeChanged
|
signal |
Emitted when the user changes the theme.
Stylesheet usage, colors, etc. should be updated at this point. However, SVGs should not be repainted in response to this signal; connect to Svg::repaintNeeded() instead for that, as Svg objects need repainting not only when themeChanged() is emitted; moreover Svg objects connect to and respond appropriately to themeChanged() internally, emitting Svg::repaintNeeded() at an appropriate time.
◆ themeName()
QString Plasma::Theme::themeName | ( | ) | const |
◆ useGlobalSettings()
bool Plasma::Theme::useGlobalSettings | ( | ) | const |
◆ wallpaperPath()
◆ wallpaperPathForSize()
QString Plasma::Theme::wallpaperPathForSize | ( | int | width = -1, |
int | height = -1 ) const |
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2025 The KDE developers.
Generated on Fri May 2 2025 11:55:47 by doxygen 1.13.2 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.