PolkitQt1::Authority
#include <Authority>
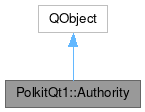
Public Types | |
enum | AuthorizationFlag { None = 0x00 , AllowUserInteraction = 0x01 } |
typedef QFlags< AuthorizationFlag > | AuthorizationFlags |
enum | ErrorCode { E_None = 0x00 , E_GetAuthority = 0x01 , E_CheckFailed = 0x02 , E_WrongSubject = 0x03 , E_UnknownResult = 0x04 , E_EnumFailed = 0x05 , E_RegisterFailed = 0x06 , E_UnregisterFailed = 0x07 , E_CookieOrIdentityEmpty = 0x08 , E_AgentResponseFailed = 0x09 , E_RevokeFailed = 0x0A } |
enum | Result { Unknown = 0x00 , Yes = 0x01 , No = 0x02 , Challenge = 0x03 } |
Signals | |
void | authenticationAgentResponseFinished (bool) |
void | checkAuthorizationFinished (PolkitQt1::Authority::Result) |
void | configChanged () |
void | consoleKitDBChanged () |
void | enumerateActionsFinished (PolkitQt1::ActionDescription::List) |
void | enumerateTemporaryAuthorizationsFinished (PolkitQt1::TemporaryAuthorization::List) |
void | registerAuthenticationAgentFinished (bool) |
void | revokeTemporaryAuthorizationFinished (bool) |
void | revokeTemporaryAuthorizationsFinished (bool) |
void | unregisterAuthenticationAgentFinished (bool) |
Static Public Member Functions | |
static Authority * | instance (PolkitAuthority *authority=nullptr) |
![]() | |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *context, Functor functor, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, Functor functor) |
bool | disconnect (const QMetaObject::Connection &connection) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
bool | disconnect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
Additional Inherited Members | |
![]() | |
objectName | |
![]() | |
typedef | QObjectList |
![]() | |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const QMetaMethod &signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const QMetaMethod &signal) |
bool | isSignalConnected (const QMetaMethod &signal) const const |
int | receivers (const char *signal) const const |
QObject * | sender () const const |
int | senderSignalIndex () const const |
virtual void | timerEvent (QTimerEvent *event) |
Detailed Description
Convenience class for Qt/KDE applications.
This class is a singleton that provides makes easy the usage of PolKitAuthority. It emits configChanged() whenever PolicyKit files change (e.g. the PolicyKit.conf or .policy files) or when ConsoleKit reports activities changes.
- Note
- This class is a singleton, its constructor is private. Call Authority::instance() to get an instance of the Authority object. Do not delete Authority::instance(), cleanup will be done automatically.
Definition at line 55 of file polkitqt1-authority.h.
Member Typedef Documentation
◆ AuthorizationFlags
Definition at line 82 of file polkitqt1-authority.h.
Member Enumeration Documentation
◆ AuthorizationFlag
Definition at line 72 of file polkitqt1-authority.h.
◆ ErrorCode
Error codes for the authority class.
Enumerator | |
---|---|
E_None | No error occurred. |
E_GetAuthority | Authority cannot be obtained. |
E_CheckFailed | Authority check failed. |
E_WrongSubject | Wrong or empty subject was given. |
E_UnknownResult | Action returned unknown result. |
E_EnumFailed | Enumerating actions failed. |
E_RegisterFailed | Registration of authentication agent failed. |
E_UnregisterFailed | Unregistration of authentication agent failed. |
E_CookieOrIdentityEmpty | Cookie or polkitqt1-identity.handled to the action is empty. |
E_AgentResponseFailed | Response of auth agent failed. |
E_RevokeFailed | Revoke temporary authorizations failed. |
Definition at line 85 of file polkitqt1-authority.h.
◆ Result
Enumerator | |
---|---|
Unknown | Result unknown. |
Yes | The subject is authorized for the specified action. |
No | The subject is not authorized for the specified action. |
Challenge | The subject is authorized if more information is provided. |
Definition at line 60 of file polkitqt1-authority.h.
Constructor & Destructor Documentation
◆ ~Authority()
|
override |
Definition at line 161 of file polkitqt1-authority.cpp.
Member Function Documentation
◆ authenticationAgentResponse()
void PolkitQt1::Authority::authenticationAgentResponse | ( | const QString & | cookie, |
const Identity & | identity ) |
Provide response that identity
successfully authenticated for the authentication request identified by cookie
.
- See also
- authenticationAgentResponseSync Synchronous version of this method.
- authenticationAgentResponseFinished Signal that is emitted when this method finishes.
- authenticationAgentResponseCancel Use it to cancel execution of this method.
- Parameters
-
cookie The cookie passed to the authentication agent from the authority. identity The identity that was authenticated.
Definition at line 677 of file polkitqt1-authority.cpp.
◆ authenticationAgentResponseCancel()
void PolkitQt1::Authority::authenticationAgentResponseCancel | ( | ) |
This method can be used to cancel the authenticationAgentResponseAsync method.
Definition at line 714 of file polkitqt1-authority.cpp.
◆ authenticationAgentResponseFinished
|
signal |
This signal is emitted when asynchronous method authenticationAgentResponse finishes.
The argument is true
if authority acknowledged the call, false
if error is set.
◆ authenticationAgentResponseSync()
bool PolkitQt1::Authority::authenticationAgentResponseSync | ( | const QString & | cookie, |
const PolkitQt1::Identity & | identity ) |
Provide response that identity
successfully authenticated for the authentication request identified by cookie
.
- See also
- authenticationAgentResponse Asynchronous version of this method.
- Parameters
-
cookie The cookie passed to the authentication agent from the authority. identity The identity that was authenticated.
- Returns
true
if authority acknowledged the call,false
if error is set.
Definition at line 650 of file polkitqt1-authority.cpp.
◆ checkAuthorization()
void PolkitQt1::Authority::checkAuthorization | ( | const QString & | actionId, |
const Subject & | subject, | ||
AuthorizationFlags | flags ) |
This function should be used by mechanisms (e.g.: helper applications).
It returns the action should be carried out, so if the caller was actually authorized to perform it. The result is in form of a Result, so that you can have more control over the whole process, and detect an eventual error. Most of the times you simply want to check if the result is == to Result::Yes
, if you don't have specific needs.
It is CRITICAL that you call this function and check what it returns before doing anything in your helper, since otherwise you could be actually performing an action from an unknown or unauthorized caller.
When operation is finished, signal checkAuthorizationFinish is emitted with result of authorization check in its parameter.
- See also
- checkAuthorizationSync Synchronous version of this method.
- checkAuthorizationFinished Signal that is emitted when this method finishes.
- checkAuthorizationCancel Use it to cancel execution of this method.
- Parameters
-
actionId the Id of the action in question subject subject that the action is authorized for (e.g. unix process) flags flags that influences the authorization checking
Definition at line 411 of file polkitqt1-authority.cpp.
◆ checkAuthorizationCancel()
void PolkitQt1::Authority::checkAuthorizationCancel | ( | ) |
This method can be used to cancel last authorization check.
Definition at line 441 of file polkitqt1-authority.cpp.
◆ checkAuthorizationFinished
|
signal |
This signal is emitted when asynchronous method checkAuthorization finishes.
The argument is the result of authorization.
◆ checkAuthorizationSync()
Authority::Result PolkitQt1::Authority::checkAuthorizationSync | ( | const QString & | actionId, |
const Subject & | subject, | ||
AuthorizationFlags | flags ) |
Synchronous version of the checkAuthorization method.
- Parameters
-
actionId the Id of the action in question subject subject that the action is authorized for (e.g. unix process) flags flags that influences the authorization checking
- See also
- checkAuthorization Asynchronous version of this method.
Definition at line 380 of file polkitqt1-authority.cpp.
◆ checkAuthorizationSyncWithDetails()
Authority::Result PolkitQt1::Authority::checkAuthorizationSyncWithDetails | ( | const QString & | actionId, |
const Subject & | subject, | ||
AuthorizationFlags | flags, | ||
const DetailsMap & | details ) |
This function does the same as checkAuthorizationSync(const QString&, const Subject&, AuthorizationFlags), but also accepts a DetailsMap parameter.
- See also
- checkAuthorization(const QString&, const Subject&, AuthorizationFlags, const DetailsMap&) for a description of the details parameter.
- checkAuthorizationSync(const QString&, const Subject, AuthorizationFlags) Base version of this method.
Definition at line 336 of file polkitqt1-authority.cpp.
◆ checkAuthorizationWithDetails()
void PolkitQt1::Authority::checkAuthorizationWithDetails | ( | const QString & | actionId, |
const Subject & | subject, | ||
AuthorizationFlags | flags, | ||
const DetailsMap & | details ) |
This function does the same as checkAuthorization(const QString&, const Subject&, AuthorizationFlags), but also accepts a DetailsMap parameter.
This map can contain key/value pairs that, for example, are used to expand placeholders in polkit authentication messages that are formatted like "Authentication required to access $(device)". For this example, when a key "device" exists in the map, the "$(device)" will be replaced by the corresponding value in the map.
- See also
- checkAuthorization(const QString&, const Subject&, AuthorizationFlags) Base version of this method.
Definition at line 385 of file polkitqt1-authority.cpp.
◆ clearError()
void PolkitQt1::Authority::clearError | ( | ) |
Use this method to clear the error message.
Definition at line 303 of file polkitqt1-authority.cpp.
◆ configChanged
|
signal |
This signal will be emitted when a configuration file gets changed (e.g.
/etc/PolicyKit/PolicyKit.conf or .policy files). Connect to this signal if you want to track down actions.
◆ consoleKitDBChanged
|
signal |
This signal is emitted when ConsoleKit configuration changes.
This might happen when a session becomes active or inactive.
If you want to track your actions directly you should connect to this signal, as this might change the return value PolicyKit will give you.
◆ enumerateActions()
void PolkitQt1::Authority::enumerateActions | ( | ) |
Asynchronously retrieves all registered actions.
When operation is finished, signal checkAuthorizationFinish is emitted with result of authorization check in its parameter.
- See also
- enumerateActionsSync Synchronous version of this method.
- enumerateActionsFinished Signal that is emitted when this method finishes.
- enumerateActionsCancel Use it to cancel execution of this method.
Definition at line 469 of file polkitqt1-authority.cpp.
◆ enumerateActionsCancel()
void PolkitQt1::Authority::enumerateActionsCancel | ( | ) |
This method can be used to cancel enumeration of actions.
Definition at line 499 of file polkitqt1-authority.cpp.
◆ enumerateActionsFinished
|
signal |
This signal is emitted when asynchronous method enumerateActions finishes.
The argument is the list of all Action IDs.
◆ enumerateActionsSync()
ActionDescription::List PolkitQt1::Authority::enumerateActionsSync | ( | ) |
Synchronously retrieves all registered actions.
- See also
- enumerateActions Asynchronous version of this method.
- Returns
- a list of Action IDs
Definition at line 448 of file polkitqt1-authority.cpp.
◆ enumerateTemporaryAuthorizations()
void PolkitQt1::Authority::enumerateTemporaryAuthorizations | ( | const Subject & | subject | ) |
Retrieves all temporary action that applies to subject
.
- See also
- enumerateTemporaryAuthorizationsSync Synchronous version of this method.
- enumerateTemporaryAuthorizationsFinished Signal that is emitted when this method finishes.
- enumerateTemporaryAuthorizationsCancel Use it to cancel execution of this method.
- Parameters
-
subject the subject to get temporary authorizations for
◆ enumerateTemporaryAuthorizationsCancel()
void PolkitQt1::Authority::enumerateTemporaryAuthorizationsCancel | ( | ) |
This method can be used to cancel the enumerateTemporaryAuthorizationsAsync method.
Definition at line 775 of file polkitqt1-authority.cpp.
◆ enumerateTemporaryAuthorizationsFinished
|
signal |
This signal is emitted when asynchronous method enumerateTemporaryAuthorizations finishes.
The argument is list of all temporary authorizations.
- Note
- Free all TemporaryAuthorization objects using
delete
operator.
◆ enumerateTemporaryAuthorizationsSync()
TemporaryAuthorization::List PolkitQt1::Authority::enumerateTemporaryAuthorizationsSync | ( | const Subject & | subject | ) |
Retrieves all temporary action that applies to subject
.
- See also
- enumerateTemporaryAuthorizations Asynchronous version of this method.
- Parameters
-
subject the subject to get temporary authorizations for
- Note
- Free all TemporaryAuthorization objects using
delete
operator.
- Returns
- List of all temporary authorizations
Definition at line 721 of file polkitqt1-authority.cpp.
◆ errorDetails()
const QString PolkitQt1::Authority::errorDetails | ( | ) | const |
Get detail information about error that occurred.
- Returns
- detail message
Definition at line 294 of file polkitqt1-authority.cpp.
◆ hasError()
bool PolkitQt1::Authority::hasError | ( | ) | const |
You should always call this method after every action.
No action will be allowed if the object is in error state. Use clearError() to clear the error message.
- See also
- lastError
- clearError
- Returns
true
if an error occurred,false
if the library is ready
Definition at line 284 of file polkitqt1-authority.cpp.
◆ instance()
|
static |
Returns the instance of Authority.
Returns the current instance of Authority. Call this function whenever you need to access the Authority class.
- Note
- Authority is a singleton. Memory is handled by polkit-qt, so you just need to call this function to get a working instance of Authority. Don't delete the object after having used it.
- Parameters
-
authority use this if you want to set an explicit PolkitAuthority. If you don't know what this implies, simply ignore the parameter. In case you want to use it, be sure of streaming it the first time you call this function, otherwise it will have no effect.
- Returns
- The current authority instance
Definition at line 34 of file polkitqt1-authority.cpp.
◆ lastError()
Authority::ErrorCode PolkitQt1::Authority::lastError | ( | ) | const |
- Returns
- the code of last error
Definition at line 289 of file polkitqt1-authority.cpp.
◆ polkitAuthority()
PolkitAuthority * PolkitQt1::Authority::polkitAuthority | ( | ) | const |
Returns the current instance of PolkitAuthority.
If you are handling it through Polkit-qt (which is quite likely, since you are calling this function), DO NOT use any PolicyKit API's specific method that modifies the instance on it, unless you're completely aware of what you're doing and of the possible consequencies. Use this instance only to gather information.
- Returns
- the current PolkitAuthority instance
Definition at line 331 of file polkitqt1-authority.cpp.
◆ registerAuthenticationAgent()
void PolkitQt1::Authority::registerAuthenticationAgent | ( | const Subject & | subject, |
const QString & | locale, | ||
const QString & | objectPath ) |
Registers an authentication agent.
- See also
- registerAuthenticationAgentSync Synchronous version of this method.
- registerAuthenticationAgentFinished Signal that is emitted when this method finishes.
- registerAuthenticationAgentCancel Use it to cancel execution of this method.
- Parameters
-
subject caller subject locale the locale of the authentication agent objectPath the object path for the authentication agent
Definition at line 533 of file polkitqt1-authority.cpp.
◆ registerAuthenticationAgentCancel()
void PolkitQt1::Authority::registerAuthenticationAgentCancel | ( | ) |
This method can be used to cancel the registration of the authentication agent.
Definition at line 571 of file polkitqt1-authority.cpp.
◆ registerAuthenticationAgentFinished
|
signal |
This signal is emitted when asynchronous method registerAuthenticationAgent finishes.
The argument is true
if the Authentication agent has been successfully registered false
if the Authentication agent registration failed
◆ registerAuthenticationAgentSync()
bool PolkitQt1::Authority::registerAuthenticationAgentSync | ( | const Subject & | subject, |
const QString & | locale, | ||
const QString & | objectPath ) |
Registers an authentication agent.
- See also
- registerAuthenticationAgent Asynchronous version of this method.
- Parameters
-
subject caller subject locale the locale of the authentication agent objectPath the object path for the authentication agent
- Returns
true
if the Authentication agent has been successfully registeredfalse
if the Authentication agent registration failed
Definition at line 506 of file polkitqt1-authority.cpp.
◆ revokeTemporaryAuthorization()
void PolkitQt1::Authority::revokeTemporaryAuthorization | ( | const QString & | id | ) |
Revokes temporary authorization by id
.
- See also
- revokeTemporaryAuthorizationSync Synchronous version of this method.
- revokeTemporaryAuthorizationFinished Signal that is emitted when this method finishes.
- revokeTemporaryAuthorizationCancel Use it to cancel execution of this method.
- Parameters
-
id the identifier of the temporary authorization
Definition at line 862 of file polkitqt1-authority.cpp.
◆ revokeTemporaryAuthorizationCancel()
void PolkitQt1::Authority::revokeTemporaryAuthorizationCancel | ( | ) |
This method can be used to cancel the method revokeTemporaryAuthorizationAsync.
Definition at line 895 of file polkitqt1-authority.cpp.
◆ revokeTemporaryAuthorizationFinished
|
signal |
This signal is emitted when asynchronous method revokeTemporaryAuthorization finishes.
- Returns
true
if the temporary authorization was revokedfalse
if the revoking failed
◆ revokeTemporaryAuthorizations()
void PolkitQt1::Authority::revokeTemporaryAuthorizations | ( | const Subject & | subject | ) |
Revokes all temporary authorizations that applies to subject
.
- See also
- revokeTemporaryAuthorizationsSync Synchronous version of this method.
- revokeTemporaryAuthorizationsFinished Signal that is emitted when this method finishes.
- revokeTemporaryAuthorizationsCancel Use it to cancel execution of this method.
- Parameters
-
subject the subject to revoke temporary authorizations from
Definition at line 802 of file polkitqt1-authority.cpp.
◆ revokeTemporaryAuthorizationsCancel()
void PolkitQt1::Authority::revokeTemporaryAuthorizationsCancel | ( | ) |
This method can be used to cancel the method revokeTemporaryAuthorizationsAsync.
Definition at line 835 of file polkitqt1-authority.cpp.
◆ revokeTemporaryAuthorizationsFinished
|
signal |
This signal is emitted when asynchronous method revokeTemporaryAuthorizations finishes.
The argument is true
if all temporary authorizations were revoked false
if the revoking failed
◆ revokeTemporaryAuthorizationsSync()
bool PolkitQt1::Authority::revokeTemporaryAuthorizationsSync | ( | const Subject & | subject | ) |
Revokes all temporary authorizations that applies to subject
.
- See also
- revokeTemporaryAuthorizations Asynchronous version of this method.
- Parameters
-
subject the subject to revoke temporary authorizations from
- Returns
true
if all temporary authorization were revokedfalse
if the revoking failed
Definition at line 782 of file polkitqt1-authority.cpp.
◆ revokeTemporaryAuthorizationSync()
bool PolkitQt1::Authority::revokeTemporaryAuthorizationSync | ( | const QString & | id | ) |
Revokes temporary authorization by id
.
- See also
- revokeTemporaryAuthorization Asynchronous version of this method.
- Parameters
-
id the identifier of the temporary authorization
- Returns
true
if the temporary authorization was revokedfalse
if the revoking failed
Definition at line 842 of file polkitqt1-authority.cpp.
◆ unregisterAuthenticationAgent()
void PolkitQt1::Authority::unregisterAuthenticationAgent | ( | const Subject & | subject, |
const QString & | objectPath ) |
Unregisters an Authentication agent.
- See also
- unregisterAuthenticationAgentSync Synchronous version of this method.
- unregisterAuthenticationAgentFinished Signal that is emitted when this method finishes.
- unregisterAuthenticationAgentCancel Use it to cancel execution of this method.
- Parameters
-
subject caller subject objectPath the object path for the Authentication agent
- Returns
true
if the Authentication agent has been successfully unregisteredfalse
if the Authentication agent unregistration failed
Definition at line 606 of file polkitqt1-authority.cpp.
◆ unregisterAuthenticationAgentCancel()
void PolkitQt1::Authority::unregisterAuthenticationAgentCancel | ( | ) |
This method can be used to cancel the unregistration of the authentication agent.
Definition at line 643 of file polkitqt1-authority.cpp.
◆ unregisterAuthenticationAgentFinished
|
signal |
This signal is emitted when asynchronous method unregisterAuthenticationAgent finishes.
The argument is true
if the Authentication agent has been successfully unregistered false
if the Authentication agent unregistration failed
◆ unregisterAuthenticationAgentSync()
bool PolkitQt1::Authority::unregisterAuthenticationAgentSync | ( | const Subject & | subject, |
const QString & | objectPath ) |
Unregisters an Authentication agent.
- See also
- unregisterAuthenticationAgent Asynchronous version of this method.
- Parameters
-
subject caller subject objectPath the object path for the Authentication agent
- Returns
true
if the Authentication agent has been successfully unregisteredfalse
if the Authentication agent unregistration failed
Definition at line 578 of file polkitqt1-authority.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2025 The KDE developers.
Generated on Fri Apr 11 2025 11:47:37 by doxygen 1.13.2 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.