PolkitQt1::Gui::Action
#include <Action>
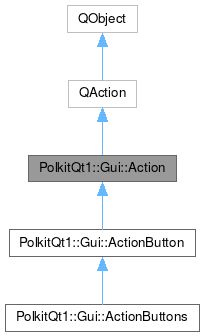
Public Types | |
enum | State { None = 0 , SelfBlocked = 1 , Yes = 2 , No = 4 , Auth = 8 , All = 512 } |
typedef QFlags< State > | States |
![]() | |
enum | ActionEvent |
enum | MenuRole |
enum | Priority |
Signals | |
void | authorized () |
void | dataChanged () |
Public Slots | |
bool | activate () |
void | revoke () |
void | setChecked (bool checked) |
Public Member Functions | |
Action (const QString &actionId=QString(), QObject *parent=nullptr) | |
QString | actionId () const |
QIcon | icon (State state=None) const |
bool | is (const QString &actionId) const |
bool | isAllowed () const |
bool | isEnabled (State state=None) const |
bool | isVisible (State state=None) const |
void | setEnabled (bool enabled, States states=All) |
void | setIcon (const QIcon &icon, States states=All) |
void | setPolkitAction (const QString &actionId) |
void | setTargetPID (qint64 pid) |
void | setText (const QString &text, States states=All) |
void | setToolTip (const QString &toolTip, States states=All) |
void | setVisible (bool visible, States states=All) |
void | setWhatsThis (const QString &whatsThis, States states=All) |
qint64 | targetPID () const |
QString | text (State state=None) const |
QString | toolTip (State state=None) const |
QString | whatsThis (State state=None) const |
![]() | |
QAction (const QIcon &icon, const QString &text, QObject *parent) | |
QAction (const QString &text, QObject *parent) | |
QAction (QObject *parent) | |
QActionGroup * | actionGroup () const const |
void | activate (ActionEvent event) |
QList< QGraphicsWidget * > | associatedGraphicsWidgets () const const |
QList< QObject * > | associatedObjects () const const |
QList< QWidget * > | associatedWidgets () const const |
bool | autoRepeat () const const |
void | changed () |
void | checkableChanged (bool checkable) |
QVariant | data () const const |
void | enabledChanged (bool enabled) |
QFont | font () const const |
void | hover () |
void | hovered () |
QIcon | icon () const const |
QString | iconText () const const |
bool | isCheckable () const const |
bool | isChecked () const const |
bool | isEnabled () const const |
bool | isIconVisibleInMenu () const const |
bool | isSeparator () const const |
bool | isShortcutVisibleInContextMenu () const const |
bool | isVisible () const const |
QMenu * | menu () const const |
MenuRole | menuRole () const const |
QWidget * | parentWidget () const const |
Priority | priority () const const |
void | resetEnabled () |
void | setActionGroup (QActionGroup *group) |
void | setAutoRepeat (bool) |
void | setCheckable (bool) |
void | setChecked (bool) |
void | setData (const QVariant &data) |
void | setDisabled (bool b) |
void | setEnabled (bool) |
void | setFont (const QFont &font) |
void | setIcon (const QIcon &icon) |
void | setIconText (const QString &text) |
void | setIconVisibleInMenu (bool visible) |
void | setMenu (QMenu *menu) |
void | setMenuRole (MenuRole menuRole) |
void | setPriority (Priority priority) |
void | setSeparator (bool b) |
void | setShortcut (const QKeySequence &shortcut) |
void | setShortcutContext (Qt::ShortcutContext context) |
void | setShortcuts (const QList< QKeySequence > &shortcuts) |
void | setShortcuts (QKeySequence::StandardKey key) |
void | setShortcutVisibleInContextMenu (bool show) |
void | setStatusTip (const QString &statusTip) |
void | setText (const QString &text) |
void | setToolTip (const QString &tip) |
void | setVisible (bool) |
void | setWhatsThis (const QString &what) |
QKeySequence | shortcut () const const |
Qt::ShortcutContext | shortcutContext () const const |
QList< QKeySequence > | shortcuts () const const |
bool | showStatusText (QObject *object) |
QString | statusTip () const const |
QString | text () const const |
void | toggle () |
void | toggled (bool checked) |
QString | toolTip () const const |
void | trigger () |
void | triggered (bool checked) |
void | visibleChanged () |
QString | whatsThis () const const |
![]() | |
QObject (QObject *parent) | |
QBindable< QString > | bindableObjectName () |
bool | blockSignals (bool block) |
const QObjectList & | children () const const |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) const const |
bool | disconnect (const QObject *receiver, const char *method) const const |
void | dumpObjectInfo () const const |
void | dumpObjectTree () const const |
QList< QByteArray > | dynamicPropertyNames () const const |
virtual bool | eventFilter (QObject *watched, QEvent *event) |
T | findChild (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QRegularExpression &re, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (Qt::FindChildOptions options) const const |
bool | inherits (const char *className) const const |
void | installEventFilter (QObject *filterObj) |
bool | isQuickItemType () const const |
bool | isWidgetType () const const |
bool | isWindowType () const const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const const |
void | moveToThread (QThread *targetThread) |
QString | objectName () const const |
void | objectNameChanged (const QString &objectName) |
QObject * | parent () const const |
QVariant | property (const char *name) const const |
Q_CLASSINFO (Name, Value) | |
Q_EMIT Q_EMIT | |
Q_ENUM (...) | |
Q_ENUM_NS (...) | |
Q_ENUMS (...) | |
Q_FLAG (...) | |
Q_FLAG_NS (...) | |
Q_FLAGS (...) | |
Q_GADGET Q_GADGET | |
Q_GADGET_EXPORT (EXPORT_MACRO) | |
Q_INTERFACES (...) | |
Q_INVOKABLE Q_INVOKABLE | |
Q_MOC_INCLUDE Q_MOC_INCLUDE | |
Q_NAMESPACE Q_NAMESPACE | |
Q_NAMESPACE_EXPORT (EXPORT_MACRO) | |
Q_OBJECT Q_OBJECT | |
Q_PROPERTY (...) | |
Q_REVISION Q_REVISION | |
Q_SET_OBJECT_NAME (Object) | |
Q_SIGNAL Q_SIGNAL | |
Q_SIGNALS Q_SIGNALS | |
Q_SLOT Q_SLOT | |
Q_SLOTS Q_SLOTS | |
T | qobject_cast (const QObject *object) |
T | qobject_cast (QObject *object) |
QT_NO_NARROWING_CONVERSIONS_IN_CONNECT QT_NO_NARROWING_CONVERSIONS_IN_CONNECT | |
void | removeEventFilter (QObject *obj) |
void | setObjectName (const QString &name) |
void | setObjectName (QAnyStringView name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | setProperty (const char *name, QVariant &&value) |
bool | signalsBlocked () const const |
int | startTimer (int interval, Qt::TimerType timerType) |
int | startTimer (std::chrono::milliseconds interval, Qt::TimerType timerType) |
QThread * | thread () const const |
Additional Inherited Members | |
![]() | |
autoRepeat | |
checkable | |
checked | |
enabled | |
font | |
icon | |
iconText | |
iconVisibleInMenu | |
menuRole | |
priority | |
shortcut | |
shortcutContext | |
shortcutVisibleInContextMenu | |
statusTip | |
text | |
toolTip | |
visible | |
whatsThis | |
![]() | |
objectName | |
![]() | |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *context, Functor functor, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, Functor functor) |
bool | disconnect (const QMetaObject::Connection &connection) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
bool | disconnect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
![]() | |
AboutQtRole | |
AboutRole | |
ApplicationSpecificRole | |
HighPriority | |
Hover | |
LowPriority | |
NormalPriority | |
NoRole | |
PreferencesRole | |
QuitRole | |
TextHeuristicRole | |
Trigger | |
![]() | |
typedef | QObjectList |
![]() | |
virtual bool | event (QEvent *e) override |
![]() | |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const QMetaMethod &signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const QMetaMethod &signal) |
bool | isSignalConnected (const QMetaMethod &signal) const const |
int | receivers (const char *signal) const const |
QObject * | sender () const const |
int | senderSignalIndex () const const |
virtual void | timerEvent (QTimerEvent *event) |
Detailed Description
Class used to manage actions.
This class is an interface around PolicyKit Actions. By using this class, you are able to track the result of a given action.
Most of the times, you would want to use this class combined with a QAbstractButton. In this case, you can use the more comfortable ActionButton class that manages button's properties update for you.
- See also
- ActionButton
Definition at line 40 of file polkitqt1-gui-action.h.
Member Typedef Documentation
◆ States
typedef QFlags< State > PolkitQt1::Gui::Action::States |
Definition at line 59 of file polkitqt1-gui-action.h.
Member Enumeration Documentation
◆ State
enum PolkitQt1::Gui::Action::State |
Definition at line 46 of file polkitqt1-gui-action.h.
Constructor & Destructor Documentation
◆ Action()
Constructs a new Action item.
- Parameters
-
actionId the PolicyKit action Id (e.g.: org.freedesktop.policykit.read) parent the object parent
Definition at line 92 of file polkitqt1-gui-action.cpp.
◆ ~Action()
|
override |
Definition at line 107 of file polkitqt1-gui-action.cpp.
Member Function Documentation
◆ actionId()
QString Action::actionId | ( | ) | const |
Returns the current action ID.
- Returns
- The action ID
Definition at line 493 of file polkitqt1-gui-action.cpp.
◆ activate
|
slot |
Use this slot if you want to activate the action.
authorized() will be emitted if the action gets authorized.
- Returns
true
if the caller can do the action
- See also
- authorized()
Definition at line 112 of file polkitqt1-gui-action.cpp.
◆ authorized
|
signal |
Emitted when using this class as a proxy for a given action, It's only emitted if the activate() slot is called and the auth permits the action.
- See also
- activate()
◆ dataChanged
|
signal |
Emitted when the PolicyKit result (PolKitResult) for the given action or the internal data changes (i.e.
the user called one of the set methods). You should connect to this signal if you want to track these changes.
◆ icon()
Gets the icon of the action when it is in the specified state.
- Note
- Passing None will return the current value
- Parameters
-
state The state to be checked
- Returns
- The icon shown when the action is in the specified state
Definition at line 388 of file polkitqt1-gui-action.cpp.
◆ is()
bool Action::is | ( | const QString & | actionId | ) | const |
This method compares a PolicyKit action Id with the current one of the object.
- See also
- actionId()
- Parameters
-
actionId the action Id to compare
- Returns
true
if the actionId is the same as this object's one
Definition at line 244 of file polkitqt1-gui-action.cpp.
◆ isAllowed()
bool Action::isAllowed | ( | ) | const |
This method can be used to check the if the current action can be performed (i.e.
PolKitResult is YES).
- Note
- This method does not call the authentication dialog, use activate() instead
- Returns
true
if the action can be performed
Definition at line 239 of file polkitqt1-gui-action.cpp.
◆ isEnabled()
bool Action::isEnabled | ( | State | state = None | ) | const |
Gets whether the action is enabled or not when it is in the specified state.
- Note
- Passing None will return the current value
- Parameters
-
state The state to be checked
- Returns
- Whether the action is enabled or not in the specified state
Definition at line 426 of file polkitqt1-gui-action.cpp.
◆ isVisible()
bool Action::isVisible | ( | State | state = None | ) | const |
Gets whether the action is visible or not when it is in the specified state.
- Note
- Passing None will return the current value
- Parameters
-
state The state to be checked
- Returns
- Whether the action is visible or not in the specified state
Definition at line 464 of file polkitqt1-gui-action.cpp.
◆ revoke
|
slot |
This method can be used to revoke the authorization obtained for this action.
Definition at line 249 of file polkitqt1-gui-action.cpp.
◆ setChecked
|
slot |
Defines the checked state.
The opposite state will trigger authentication for this actions. For example, if you set this to true
, when the action's checked state will become false
, the authentication will be triggered.
- Parameters
-
checked the new checked state
Definition at line 139 of file polkitqt1-gui-action.cpp.
◆ setEnabled()
void Action::setEnabled | ( | bool | enabled, |
States | states = All ) |
Sets whether the current action is enabled or not.
This will be shown only in the states specified in the states
parameter.
- Parameters
-
enabled whether the Action will be enabled or not states the states of the Polkit action on which the setting will be applied
Definition at line 406 of file polkitqt1-gui-action.cpp.
◆ setIcon()
Sets the icon for the current action.
This will be shown only in the states specified in the states
parameter.
- Note
- You need to pass a QIcon here. You can easily create one from a Pixmap, or pass a KIcon
- Parameters
-
icon the new icon for the action states the states of the Polkit action on which the setting will be applied
Definition at line 368 of file polkitqt1-gui-action.cpp.
◆ setPolkitAction()
void Action::setPolkitAction | ( | const QString & | actionId | ) |
Changes the action being tracked.
- Parameters
-
actionId The new action ID
Definition at line 482 of file polkitqt1-gui-action.cpp.
◆ setTargetPID()
void Action::setTargetPID | ( | qint64 | pid | ) |
This function sets the process id of the target that should receive the authorization.
Set this to 0 to set the current process as the target.
- Parameters
-
pid The target process id; 0 if it is the current process
Definition at line 231 of file polkitqt1-gui-action.cpp.
◆ setText()
Sets the text for the current action.
This will be shown only in the states specified in the states
parameter.
- Parameters
-
text the new text for the action states the states of the Polkit action on which the setting will be applied
Definition at line 254 of file polkitqt1-gui-action.cpp.
◆ setToolTip()
Sets the tooltip for the current action.
This will be shown only in the states specified in the states
parameter.
- Parameters
-
toolTip the new tooltip for the action states the states of the Polkit action on which the setting will be applied
Definition at line 292 of file polkitqt1-gui-action.cpp.
◆ setVisible()
void Action::setVisible | ( | bool | visible, |
States | states = All ) |
Sets whether the current action is visible or not.
This will be applied only in the states specified in the states
parameter.
- Parameters
-
visible visibility of the action states the states of the Polkit action on which the setting will be applied
Definition at line 444 of file polkitqt1-gui-action.cpp.
◆ setWhatsThis()
Sets the whatsthis for the current action.
This will be shown only in the states specified in the states
parameter.
- Parameters
-
whatsThis the new whatsthis for the action states the states of the Polkit action on which the setting will be applied
Definition at line 330 of file polkitqt1-gui-action.cpp.
◆ targetPID()
qint64 Action::targetPID | ( | ) | const |
- See also
- setTargetPID
Definition at line 222 of file polkitqt1-gui-action.cpp.
◆ text()
Gets the text of the action when it is in the specified state.
- Note
- Passing None will return the current value
- Parameters
-
state The state to be checked
- Returns
- The text shown when the action is in the specified state
◆ toolTip()
Gets the tooltip of the action when it is in the specified state.
- Note
- Passing None will return the current value
- Parameters
-
state The state to be checked
- Returns
- The tooltip shown when the action is in the specified state
Definition at line 312 of file polkitqt1-gui-action.cpp.
◆ whatsThis()
Gets the whatsThis of the action when it is in the specified state.
- Parameters
-
state The state to be checked
- Returns
- The whatsThis shown when the action is in the specified state
Definition at line 350 of file polkitqt1-gui-action.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2025 The KDE developers.
Generated on Fri May 2 2025 11:58:04 by doxygen 1.13.2 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.