PolkitQt1::Gui::ActionButton
#include <ActionButton>
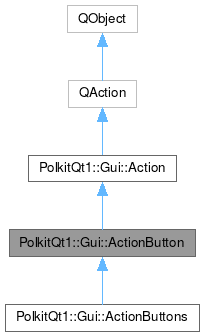
Signals | |
void | clicked (QAbstractButton *button, bool checked=false) |
![]() | |
void | authorized () |
void | dataChanged () |
Public Slots | |
bool | activate () |
![]() | |
bool | activate () |
void | revoke () |
void | setChecked (bool checked) |
Public Member Functions | |
ActionButton (QAbstractButton *button, const QString &actionId=QString(), QObject *parent=nullptr) | |
QAbstractButton * | button () const |
void | setButton (QAbstractButton *button) |
![]() | |
Action (const QString &actionId=QString(), QObject *parent=nullptr) | |
QString | actionId () const |
QIcon | icon (State state=None) const |
bool | is (const QString &actionId) const |
bool | isAllowed () const |
bool | isEnabled (State state=None) const |
bool | isVisible (State state=None) const |
void | setEnabled (bool enabled, States states=All) |
void | setIcon (const QIcon &icon, States states=All) |
void | setPolkitAction (const QString &actionId) |
void | setTargetPID (qint64 pid) |
void | setText (const QString &text, States states=All) |
void | setToolTip (const QString &toolTip, States states=All) |
void | setVisible (bool visible, States states=All) |
void | setWhatsThis (const QString &whatsThis, States states=All) |
qint64 | targetPID () const |
QString | text (State state=None) const |
QString | toolTip (State state=None) const |
QString | whatsThis (State state=None) const |
![]() | |
QAction (const QIcon &icon, const QString &text, QObject *parent) | |
QAction (const QString &text, QObject *parent) | |
QAction (QObject *parent) | |
QActionGroup * | actionGroup () const const |
void | activate (ActionEvent event) |
QList< QGraphicsWidget * > | associatedGraphicsWidgets () const const |
QList< QObject * > | associatedObjects () const const |
QList< QWidget * > | associatedWidgets () const const |
bool | autoRepeat () const const |
void | changed () |
void | checkableChanged (bool checkable) |
QVariant | data () const const |
void | enabledChanged (bool enabled) |
QFont | font () const const |
void | hover () |
void | hovered () |
QIcon | icon () const const |
QString | iconText () const const |
bool | isCheckable () const const |
bool | isChecked () const const |
bool | isEnabled () const const |
bool | isIconVisibleInMenu () const const |
bool | isSeparator () const const |
bool | isShortcutVisibleInContextMenu () const const |
bool | isVisible () const const |
QMenu * | menu () const const |
MenuRole | menuRole () const const |
QWidget * | parentWidget () const const |
Priority | priority () const const |
void | resetEnabled () |
void | setActionGroup (QActionGroup *group) |
void | setAutoRepeat (bool) |
void | setCheckable (bool) |
void | setChecked (bool) |
void | setData (const QVariant &data) |
void | setDisabled (bool b) |
void | setEnabled (bool) |
void | setFont (const QFont &font) |
void | setIcon (const QIcon &icon) |
void | setIconText (const QString &text) |
void | setIconVisibleInMenu (bool visible) |
void | setMenu (QMenu *menu) |
void | setMenuRole (MenuRole menuRole) |
void | setPriority (Priority priority) |
void | setSeparator (bool b) |
void | setShortcut (const QKeySequence &shortcut) |
void | setShortcutContext (Qt::ShortcutContext context) |
void | setShortcuts (const QList< QKeySequence > &shortcuts) |
void | setShortcuts (QKeySequence::StandardKey key) |
void | setShortcutVisibleInContextMenu (bool show) |
void | setStatusTip (const QString &statusTip) |
void | setText (const QString &text) |
void | setToolTip (const QString &tip) |
void | setVisible (bool) |
void | setWhatsThis (const QString &what) |
QKeySequence | shortcut () const const |
Qt::ShortcutContext | shortcutContext () const const |
QList< QKeySequence > | shortcuts () const const |
bool | showStatusText (QObject *object) |
QString | statusTip () const const |
QString | text () const const |
void | toggle () |
void | toggled (bool checked) |
QString | toolTip () const const |
void | trigger () |
void | triggered (bool checked) |
void | visibleChanged () |
QString | whatsThis () const const |
![]() | |
QObject (QObject *parent) | |
QBindable< QString > | bindableObjectName () |
bool | blockSignals (bool block) |
const QObjectList & | children () const const |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) const const |
bool | disconnect (const QObject *receiver, const char *method) const const |
void | dumpObjectInfo () const const |
void | dumpObjectTree () const const |
QList< QByteArray > | dynamicPropertyNames () const const |
virtual bool | eventFilter (QObject *watched, QEvent *event) |
T | findChild (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QRegularExpression &re, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (Qt::FindChildOptions options) const const |
bool | inherits (const char *className) const const |
void | installEventFilter (QObject *filterObj) |
bool | isQuickItemType () const const |
bool | isWidgetType () const const |
bool | isWindowType () const const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const const |
void | moveToThread (QThread *targetThread) |
QString | objectName () const const |
void | objectNameChanged (const QString &objectName) |
QObject * | parent () const const |
QVariant | property (const char *name) const const |
Q_CLASSINFO (Name, Value) | |
Q_EMIT Q_EMIT | |
Q_ENUM (...) | |
Q_ENUM_NS (...) | |
Q_ENUMS (...) | |
Q_FLAG (...) | |
Q_FLAG_NS (...) | |
Q_FLAGS (...) | |
Q_GADGET Q_GADGET | |
Q_GADGET_EXPORT (EXPORT_MACRO) | |
Q_INTERFACES (...) | |
Q_INVOKABLE Q_INVOKABLE | |
Q_MOC_INCLUDE Q_MOC_INCLUDE | |
Q_NAMESPACE Q_NAMESPACE | |
Q_NAMESPACE_EXPORT (EXPORT_MACRO) | |
Q_OBJECT Q_OBJECT | |
Q_PROPERTY (...) | |
Q_REVISION Q_REVISION | |
Q_SET_OBJECT_NAME (Object) | |
Q_SIGNAL Q_SIGNAL | |
Q_SIGNALS Q_SIGNALS | |
Q_SLOT Q_SLOT | |
Q_SLOTS Q_SLOTS | |
T | qobject_cast (const QObject *object) |
T | qobject_cast (QObject *object) |
QT_NO_NARROWING_CONVERSIONS_IN_CONNECT QT_NO_NARROWING_CONVERSIONS_IN_CONNECT | |
void | removeEventFilter (QObject *obj) |
void | setObjectName (const QString &name) |
void | setObjectName (QAnyStringView name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | setProperty (const char *name, QVariant &&value) |
bool | signalsBlocked () const const |
int | startTimer (int interval, Qt::TimerType timerType) |
int | startTimer (std::chrono::milliseconds interval, Qt::TimerType timerType) |
QThread * | thread () const const |
Protected Member Functions | |
ActionButton (ActionButtonPrivate &dd, const QString &actionId, QObject *parent=nullptr) | |
![]() | |
virtual bool | event (QEvent *e) override |
![]() | |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const QMetaMethod &signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const QMetaMethod &signal) |
bool | isSignalConnected (const QMetaMethod &signal) const const |
int | receivers (const char *signal) const const |
QObject * | sender () const const |
int | senderSignalIndex () const const |
virtual void | timerEvent (QTimerEvent *event) |
Protected Attributes | |
ActionButtonPrivate *const | d_ptr |
Additional Inherited Members | |
![]() | |
enum | State { None = 0 , SelfBlocked = 1 , Yes = 2 , No = 4 , Auth = 8 , All = 512 } |
typedef QFlags< State > | States |
![]() | |
enum | ActionEvent |
enum | MenuRole |
enum | Priority |
![]() | |
autoRepeat | |
checkable | |
checked | |
enabled | |
font | |
icon | |
iconText | |
iconVisibleInMenu | |
menuRole | |
priority | |
shortcut | |
shortcutContext | |
shortcutVisibleInContextMenu | |
statusTip | |
text | |
toolTip | |
visible | |
whatsThis | |
![]() | |
objectName | |
![]() | |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *context, Functor functor, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, Functor functor) |
bool | disconnect (const QMetaObject::Connection &connection) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
bool | disconnect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
![]() | |
AboutQtRole | |
AboutRole | |
ApplicationSpecificRole | |
HighPriority | |
Hover | |
LowPriority | |
NormalPriority | |
NoRole | |
PreferencesRole | |
QuitRole | |
TextHeuristicRole | |
Trigger | |
![]() | |
typedef | QObjectList |
Detailed Description
Class used to hold and update a QAbstractButton.
This class allows you to associate QAbstractButtons (i.e. QPushButton) to a PolicyKit Action. It will update the button properties according to the PolicyKit Action automatically.
- Note
- You should connect the activated() signal to receive a notification when the user clicked the button and gets permission to perform the given action. If you set 'noEnabled' to
true
it will be emitted when PolKitResult is NO.
Definition at line 39 of file polkitqt1-gui-actionbutton.h.
Constructor & Destructor Documentation
◆ ActionButton() [1/2]
|
explicit |
Constructs a new ActionButton.
You need to pass this constructor an existing QAbstractButton, whose properties will be modified according to the underlying Action object. As ActionButton inherits from Action, you can define your button's behavior right through this wrapper.
- See also
- Action
- Parameters
-
button the QAbstractButton to associate to this ActionButton actionId the action Id to create the underlying Action parent the parent object
Definition at line 19 of file polkitqt1-gui-actionbutton.cpp.
◆ ~ActionButton()
|
override |
Definition at line 38 of file polkitqt1-gui-actionbutton.cpp.
◆ ActionButton() [2/2]
|
protected |
Definition at line 29 of file polkitqt1-gui-actionbutton.cpp.
Member Function Documentation
◆ activate
|
slot |
Connect clicked() signals to this slot.
This should be manually done, as in some cases we might want to manually call this. Calling this will emit authorized().
- Note
- This slot is reentrant which is likely to only be a problem if you are creating an interface to setup PolicyKit policies.
- If you have a checkbox, connect to its' clicked() signal to avoid an infinite loop as this function internally calls setChecked(). You can always use the clicked(bool) signal in this class to connect to here.
- Warning
- if you use this class take care to not call Action::activate otherwise your checkable buttons won't be properly updated.
Definition at line 66 of file polkitqt1-gui-actionbutton.cpp.
◆ button()
QAbstractButton * PolkitQt1::Gui::ActionButton::button | ( | ) | const |
Returns the current button.
- Returns
- the button currently associated with the underlying action
Definition at line 135 of file polkitqt1-gui-actionbutton.cpp.
◆ clicked
|
signal |
Emitted when the abstract button clicked(bool) signal is emitted.
This allows you to use qobject_cast<ActionButton *>(sender()) in a slot connected to this signal and call activate() on it.
- Note
- you will normally want to connect this signal to the activate slot.
- Parameters
-
button the button that has been clicked checked the checked state, if applicable. Otherwise false
◆ setButton()
void PolkitQt1::Gui::ActionButton::setButton | ( | QAbstractButton * | button | ) |
Sets the button associated to the underlying action.
- Note
- If you are calling this function, you're probably changing the button the action is referring to. If this is the case, please note that Polkit-Qt does not handle the previous button's memory, so you should take care of deleting it yourself (if needed). You can retrieve it by using button()
- See also
- button
- Parameters
-
button the new button associated with the underlying action
Definition at line 87 of file polkitqt1-gui-actionbutton.cpp.
Member Data Documentation
◆ d_ptr
|
protected |
Definition at line 120 of file polkitqt1-gui-actionbutton.h.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2024 The KDE developers.
Generated on Fri Jul 26 2024 11:51:42 by doxygen 1.11.0 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.