KBookmarkManager
#include <KBookmarkManager>
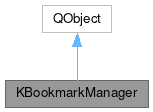
Signals | |
void | changed (const QString &groupAddress) |
void | error (const QString &errorMessage) |
Public Member Functions | |
KBookmarkManager (const QString &bookmarksFile, QObject *parent=nullptr) | |
~KBookmarkManager () override | |
void | emitChanged () |
void | emitChanged (const KBookmarkGroup &group) |
KBookmark | findByAddress (const QString &address) |
QDomDocument | internalDocument () const |
QString | path () const |
KBookmarkGroup | root () const |
bool | save (bool toolbarCache=true) const |
bool | saveAs (const QString &filename, bool toolbarCache=true) const |
KBookmarkGroup | toolbar () |
bool | updateAccessMetadata (const QString &url) |
![]() | |
QObject (QObject *parent) | |
QBindable< QString > | bindableObjectName () |
bool | blockSignals (bool block) |
const QObjectList & | children () const const |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) const const |
bool | disconnect (const QObject *receiver, const char *method) const const |
void | dumpObjectInfo () const const |
void | dumpObjectTree () const const |
QList< QByteArray > | dynamicPropertyNames () const const |
virtual bool | event (QEvent *e) |
virtual bool | eventFilter (QObject *watched, QEvent *event) |
T | findChild (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QRegularExpression &re, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (Qt::FindChildOptions options) const const |
bool | inherits (const char *className) const const |
void | installEventFilter (QObject *filterObj) |
bool | isQuickItemType () const const |
bool | isWidgetType () const const |
bool | isWindowType () const const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const const |
void | moveToThread (QThread *targetThread) |
QString | objectName () const const |
void | objectNameChanged (const QString &objectName) |
QObject * | parent () const const |
QVariant | property (const char *name) const const |
Q_CLASSINFO (Name, Value) | |
Q_EMIT Q_EMIT | |
Q_ENUM (...) | |
Q_ENUM_NS (...) | |
Q_ENUMS (...) | |
Q_FLAG (...) | |
Q_FLAG_NS (...) | |
Q_FLAGS (...) | |
Q_GADGET Q_GADGET | |
Q_GADGET_EXPORT (EXPORT_MACRO) | |
Q_INTERFACES (...) | |
Q_INVOKABLE Q_INVOKABLE | |
Q_MOC_INCLUDE Q_MOC_INCLUDE | |
Q_NAMESPACE Q_NAMESPACE | |
Q_NAMESPACE_EXPORT (EXPORT_MACRO) | |
Q_OBJECT Q_OBJECT | |
Q_PROPERTY (...) | |
Q_REVISION Q_REVISION | |
Q_SET_OBJECT_NAME (Object) | |
Q_SIGNAL Q_SIGNAL | |
Q_SIGNALS Q_SIGNALS | |
Q_SLOT Q_SLOT | |
Q_SLOTS Q_SLOTS | |
T | qobject_cast (const QObject *object) |
T | qobject_cast (QObject *object) |
QT_NO_NARROWING_CONVERSIONS_IN_CONNECT QT_NO_NARROWING_CONVERSIONS_IN_CONNECT | |
void | removeEventFilter (QObject *obj) |
void | setObjectName (const QString &name) |
void | setObjectName (QAnyStringView name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | setProperty (const char *name, QVariant &&value) |
bool | signalsBlocked () const const |
int | startTimer (int interval, Qt::TimerType timerType) |
int | startTimer (std::chrono::milliseconds interval, Qt::TimerType timerType) |
QThread * | thread () const const |
Additional Inherited Members | |
![]() | |
objectName | |
![]() | |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *context, Functor functor, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, Functor functor) |
bool | disconnect (const QMetaObject::Connection &connection) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
bool | disconnect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
![]() | |
typedef | QObjectList |
![]() | |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const QMetaMethod &signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const QMetaMethod &signal) |
bool | isSignalConnected (const QMetaMethod &signal) const const |
int | receivers (const char *signal) const const |
QObject * | sender () const const |
int | senderSignalIndex () const const |
virtual void | timerEvent (QTimerEvent *event) |
Detailed Description
This class implements the reading/writing of bookmarks in XML.
The bookmarks file is read and written using the XBEL standard (http://pyxml.sourceforge.net/topics/xbel/)
A sample file looks like this :
Definition at line 47 of file kbookmarkmanager.h.
Constructor & Destructor Documentation
◆ KBookmarkManager()
|
explicit |
Create a KBookmarkManager responsible for the given bookmarksFile
.
The manager watches the file for change detection.
- Parameters
-
bookmarksFile full path to the bookmarks file, Use ~/.kde/share/apps/konqueror/bookmarks.xml for the konqueror bookmarks
- Since
- 6.0
Definition at line 127 of file kbookmarkmanager.cpp.
◆ ~KBookmarkManager()
|
override |
Destructor.
Definition at line 162 of file kbookmarkmanager.cpp.
Member Function Documentation
◆ changed
|
signal |
Signals that the group (or any of its children) with the address groupAddress
(e.g.
"/4/5") has been modified. connect to this
◆ emitChanged() [1/2]
void KBookmarkManager::emitChanged | ( | ) |
Saves the bookmark file and notifies everyone.
Definition at line 360 of file kbookmarkmanager.cpp.
◆ emitChanged() [2/2]
void KBookmarkManager::emitChanged | ( | const KBookmarkGroup & | group | ) |
Saves the bookmark file and notifies everyone.
- Parameters
-
group the parent of all changed bookmarks
Definition at line 365 of file kbookmarkmanager.cpp.
◆ error
|
signal |
Emitted when an error occurs.
Contains the translated error message.
- Since
- 4.6
◆ findByAddress()
- Returns
- the bookmark designated by
address
- Parameters
-
address the address belonging to the bookmark you're looking for tolerate when true tries to find the most tolerable bookmark position
- See also
- KBookmark::address
Definition at line 329 of file kbookmarkmanager.cpp.
◆ internalDocument()
QDomDocument KBookmarkManager::internalDocument | ( | ) | const |
Definition at line 166 of file kbookmarkmanager.cpp.
◆ path()
QString KBookmarkManager::path | ( | ) | const |
This will return the path that this manager is using to read the bookmarks.
- Returns
- the path containing the bookmarks
Definition at line 280 of file kbookmarkmanager.cpp.
◆ root()
KBookmarkGroup KBookmarkManager::root | ( | ) | const |
This will return the root bookmark.
It is used to iterate through the bookmarks manually. It is mostly used internally.
- Returns
- the root (top-level) bookmark
Definition at line 285 of file kbookmarkmanager.cpp.
◆ save()
bool KBookmarkManager::save | ( | bool | toolbarCache = true | ) | const |
Save the bookmarks to an XML file on disk.
You should use emitChanged() instead of this function, it saves and notifies everyone that the file has changed. Only use this if you don't want the emitChanged signal.
- Parameters
-
toolbarCache iff true save a cache of the toolbar folder, too
- Returns
- true if saving was successful
Definition at line 217 of file kbookmarkmanager.cpp.
◆ saveAs()
bool KBookmarkManager::saveAs | ( | const QString & | filename, |
bool | toolbarCache = true ) const |
Save the bookmarks to the given XML file on disk.
- Parameters
-
filename full path to the desired bookmarks file location toolbarCache iff true save a cache of the toolbar folder, too
- Returns
- true if saving was successful
Definition at line 222 of file kbookmarkmanager.cpp.
◆ toolbar()
KBookmarkGroup KBookmarkManager::toolbar | ( | ) |
This returns the root of the toolbar menu.
In the XML, this is the group with the attribute toolbar=yes
- Returns
- the toolbar group
Definition at line 290 of file kbookmarkmanager.cpp.
◆ updateAccessMetadata()
bool KBookmarkManager::updateAccessMetadata | ( | const QString & | url | ) |
Update access time stamps for a given url.
- Parameters
-
url the viewed url
- Returns
- true if any metadata was modified (bookmarks file is not saved automatically)
Definition at line 376 of file kbookmarkmanager.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2025 The KDE developers.
Generated on Fri May 2 2025 11:55:16 by doxygen 1.13.2 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.