KQuickConfigModule
#include <KQuickConfigModule>
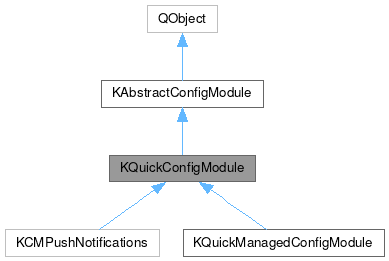
Properties | |
int | columnWidth |
int | currentIndex |
int | depth |
QQuickItem * | mainUi |
![]() | |
KAbstractConfigModule::Buttons | buttons |
bool | defaultsIndicatorsVisible |
QString | description |
QString | name |
bool | needsAuthorization |
bool | needsSave |
bool | representsDefaults |
![]() | |
objectName | |
Signals | |
void | columnWidthChanged (int width) |
void | currentIndexChanged (int index) |
void | depthChanged (int index) |
void | mainUiReady () |
void | pagePushed (QQuickItem *page) |
void | pageRemoved () |
Public Slots | |
void | pop () |
void | push (const QString &fileName, const QVariantMap &initialProperties=QVariantMap()) |
void | push (QQuickItem *item) |
QQuickItem * | takeLast () |
Public Member Functions | |
~KQuickConfigModule () override | |
int | columnWidth () const |
int | currentIndex () const |
int | depth () const |
std::shared_ptr< QQmlEngine > | engine () const |
QString | errorString () const |
QQuickItem * | mainUi () |
void | setColumnWidth (int width) |
void | setCurrentIndex (int index) |
QQuickItem * | subPage (int index) const |
![]() | |
KAbstractConfigModule (QObject *parent, const KPluginMetaData &metaData) | |
Q_SIGNAL void | activationRequested (const QVariantList &args) |
QString | authActionName () const |
Q_SIGNAL void | authActionNameChanged () |
Buttons | buttons () const |
Q_SIGNAL void | buttonsChanged () |
virtual void | defaults () |
bool | defaultsIndicatorsVisible () const |
Q_SIGNAL void | defaultsIndicatorsVisibleChanged () |
QString | description () const |
virtual void | load () |
KPluginMetaData | metaData () const |
QString | name () const |
bool | needsAuthorization () const |
bool | needsSave () const |
Q_SIGNAL void | needsSaveChanged () |
bool | representsDefaults () const |
Q_SIGNAL void | representsDefaultsChanged () |
virtual void | save () |
void | setAuthActionName (const QString &action) |
void | setButtons (const Buttons btn) |
void | setDefaultsIndicatorsVisible (bool visible) |
void | setNeedsSave (bool needs) |
void | setRepresentsDefaults (bool defaults) |
![]() | |
QObject (QObject *parent) | |
QBindable< QString > | bindableObjectName () |
bool | blockSignals (bool block) |
const QObjectList & | children () const const |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) const const |
bool | disconnect (const QObject *receiver, const char *method) const const |
void | dumpObjectInfo () const const |
void | dumpObjectTree () const const |
QList< QByteArray > | dynamicPropertyNames () const const |
virtual bool | event (QEvent *e) |
virtual bool | eventFilter (QObject *watched, QEvent *event) |
T | findChild (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QRegularExpression &re, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (Qt::FindChildOptions options) const const |
bool | inherits (const char *className) const const |
void | installEventFilter (QObject *filterObj) |
bool | isQuickItemType () const const |
bool | isWidgetType () const const |
bool | isWindowType () const const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const const |
void | moveToThread (QThread *targetThread) |
QString | objectName () const const |
void | objectNameChanged (const QString &objectName) |
QObject * | parent () const const |
QVariant | property (const char *name) const const |
Q_CLASSINFO (Name, Value) | |
Q_EMIT Q_EMIT | |
Q_ENUM (...) | |
Q_ENUM_NS (...) | |
Q_ENUMS (...) | |
Q_FLAG (...) | |
Q_FLAG_NS (...) | |
Q_FLAGS (...) | |
Q_GADGET Q_GADGET | |
Q_GADGET_EXPORT (EXPORT_MACRO) | |
Q_INTERFACES (...) | |
Q_INVOKABLE Q_INVOKABLE | |
Q_MOC_INCLUDE Q_MOC_INCLUDE | |
Q_NAMESPACE Q_NAMESPACE | |
Q_NAMESPACE_EXPORT (EXPORT_MACRO) | |
Q_OBJECT Q_OBJECT | |
Q_PROPERTY (...) | |
Q_REVISION Q_REVISION | |
Q_SET_OBJECT_NAME (Object) | |
Q_SIGNAL Q_SIGNAL | |
Q_SIGNALS Q_SIGNALS | |
Q_SLOT Q_SLOT | |
Q_SLOTS Q_SLOTS | |
T | qobject_cast (const QObject *object) |
T | qobject_cast (QObject *object) |
QT_NO_NARROWING_CONVERSIONS_IN_CONNECT QT_NO_NARROWING_CONVERSIONS_IN_CONNECT | |
void | removeEventFilter (QObject *obj) |
void | setObjectName (const QString &name) |
void | setObjectName (QAnyStringView name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | setProperty (const char *name, QVariant &&value) |
bool | signalsBlocked () const const |
int | startTimer (int interval, Qt::TimerType timerType) |
int | startTimer (std::chrono::milliseconds interval, Qt::TimerType timerType) |
QThread * | thread () const const |
Static Public Member Functions | |
static KQuickConfigModule * | qmlAttachedProperties (QObject *object) |
![]() | |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *context, Functor functor, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, Functor functor) |
bool | disconnect (const QMetaObject::Connection &connection) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
bool | disconnect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
Protected Member Functions | |
KQuickConfigModule (QObject *parent, const KPluginMetaData &metaData) | |
![]() | |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const QMetaMethod &signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const QMetaMethod &signal) |
bool | isSignalConnected (const QMetaMethod &signal) const const |
int | receivers (const char *signal) const const |
QObject * | sender () const const |
int | senderSignalIndex () const const |
virtual void | timerEvent (QTimerEvent *event) |
Additional Inherited Members | |
![]() | |
enum | Button { NoAdditionalButton = 0 , Help = 1 , Default = 2 , Apply = 4 , Export = 8 } |
typedef QFlags< Button > | Buttons |
![]() | |
typedef | QObjectList |
Detailed Description
The base class for QtQuick configuration modules.
Configuration modules are realized as plugins that are dynamically loaded.
All the necessary glue logic and the GUI bells and whistles are provided by the control center and must not concern the module author.
To write a config module, you have to create a C++ plugin and an accompanying QML user interface.
To allow KCMUtils to load your ConfigModule subclass, you must create a KPluginFactory implementation.
The constructor of the ConfigModule then looks like this:
The QML part must be in the KPackage format, installed under share/kpackage/kcms.
- See also
- KPackage::Package
The package must have the same name as the plugin filename, to be installed by CMake with the command:
The "packagedir" is the subdirectory in the source tree where the package sources are located, and "kcm_yourconfigmodule" is id of the plugin. Finally "kcms" is the literal string "kcms", so that the package is installed as a configuration module (and not some other kind of package).
The QML part can access all the properties of ConfigModule (together with the properties defined in its subclass) by accessing to the global object "kcm", or with the import of "org.kde.kcmutils" the ConfigModule attached property.
See https://develop.kde.org/docs/features/configuration/kcm/ for more detailed documentation.
- Since
- 6.0
Definition at line 105 of file kquickconfigmodule.h.
Property Documentation
◆ columnWidth
|
readwrite |
Definition at line 110 of file kquickconfigmodule.h.
◆ currentIndex
|
readwrite |
Definition at line 112 of file kquickconfigmodule.h.
◆ depth
|
read |
Definition at line 111 of file kquickconfigmodule.h.
◆ mainUi
|
read |
Definition at line 109 of file kquickconfigmodule.h.
Constructor & Destructor Documentation
◆ ~KQuickConfigModule()
|
override |
Destroys the module.
Definition at line 72 of file kquickconfigmodule.cpp.
◆ KQuickConfigModule()
|
explicitprotected |
Base class for all QtQuick config modules.
Use KQuickConfigModuleLoader to instantiate this class
- Note
- do not emit changed signals here, since they are not yet connected to any slot.
Definition at line 61 of file kquickconfigmodule.cpp.
Member Function Documentation
◆ columnWidth()
int KQuickConfigModule::columnWidth | ( | ) | const |
returns the width the kcm wants in column mode.
If a columnWidth is valid ( > 0 ) and less than the systemsettings' view width, more than one will be visible at once, and the first page will be a sidebar to the last page pushed. As default, this is -1 which will make the shell always show only one page at a time.
Definition at line 214 of file kquickconfigmodule.cpp.
◆ columnWidthChanged
|
signal |
Emitted when the wanted column width of the kcm changes.
◆ currentIndex()
int KQuickConfigModule::currentIndex | ( | ) | const |
- Returns
- the index of the page this kcm should display
Definition at line 245 of file kquickconfigmodule.cpp.
◆ currentIndexChanged
|
signal |
Emitted when the current page changed.
◆ depth()
int KQuickConfigModule::depth | ( | ) | const |
- Returns
- how many pages this kcm has. It is guaranteed to be at least 1 (the main ui) plus how many times a new page has been pushed without pop
Definition at line 229 of file kquickconfigmodule.cpp.
◆ depthChanged
|
signal |
Emitted when the number of pages changed.
◆ engine()
std::shared_ptr< QQmlEngine > KQuickConfigModule::engine | ( | ) | const |
- Returns
- the qml engine that built the main config UI
Definition at line 250 of file kquickconfigmodule.cpp.
◆ errorString()
QString KQuickConfigModule::errorString | ( | ) | const |
The error string in case the mainUi failed to load.
Definition at line 255 of file kquickconfigmodule.cpp.
◆ mainUi()
QQuickItem * KQuickConfigModule::mainUi | ( | ) |
Load the main UI for this configuration module.
It's a QQuickItem coming from the QML package named the same as the KAboutData's component name for this config module.
Normally, ui/main.qml will be loaded from the qrc baked into the plugin. However, if the PLASMA_PLATFORM environmental variable is set, the module will try to load a platform-specific QML file as its mainUi starting point.
For example:
environment has set export PLASMA_PLATFORM=phone:handset
The module will try to find main_phone.qml, then main_handset.qml, then main.qml. The first file that is found will be used as mainUi. If none is found and main.qml doesn't exist, the module is broken and an error will be displayed.
- Returns
- The main UI for this configuration module. It's a QQuickItem coming from
◆ mainUiReady
|
signal |
Emitted when the main Ui has loaded successfully and mainUi()
is available.
◆ pagePushed
|
signal |
Emitted when a new sub page is pushed.
◆ pageRemoved
|
signal |
Emitted when a sub page is popped.
◆ pop
|
slot |
pop the last page of the KCM hierarchy, the page is destroyed
Definition at line 195 of file kquickconfigmodule.cpp.
◆ push [1/2]
|
slot |
Push a new sub page in the KCM hierarchy: pages will be seen as a Kirigami PageRow.
Definition at line 155 of file kquickconfigmodule.cpp.
◆ push [2/2]
|
slot |
Definition at line 182 of file kquickconfigmodule.cpp.
◆ qmlAttachedProperties()
|
static |
Definition at line 83 of file kquickconfigmodule.cpp.
◆ setColumnWidth()
void KQuickConfigModule::setColumnWidth | ( | int | width | ) |
Sets the column width we want.
Definition at line 219 of file kquickconfigmodule.cpp.
◆ setCurrentIndex()
void KQuickConfigModule::setCurrentIndex | ( | int | index | ) |
Sets the current page index this kcm should display.
Definition at line 234 of file kquickconfigmodule.cpp.
◆ subPage()
QQuickItem * KQuickConfigModule::subPage | ( | int | index | ) | const |
Definition at line 260 of file kquickconfigmodule.cpp.
◆ takeLast
|
slot |
remove and return the last page of the KCM hierarchy: the popped page won't be deleted, it's the caller's responsibility to manage the lifetime of the returned item
- Returns
- the last page if any, nullptr otherwise
Definition at line 202 of file kquickconfigmodule.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2025 The KDE developers.
Generated on Fri May 2 2025 12:00:43 by doxygen 1.13.2 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.