KCoreAddons
#include <kuser.h>
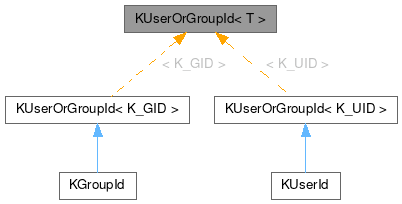
Public Types | |
typedef T | NativeType |
Public Member Functions | |
bool | isValid () const |
bool | isValid () const |
NativeType | nativeId () const |
void * | nativeId () const |
bool | operator!= (const KUserOrGroupId &other) const |
bool | operator!= (const KUserOrGroupId< void * > &other) const |
bool | operator== (const KUserOrGroupId &other) const |
bool | operator== (const KUserOrGroupId< void * > &other) const |
QString | toString () const |
QString | toString () const |
Protected Member Functions | |
KUserOrGroupId () | |
KUserOrGroupId (const KUserOrGroupId< T > &other) | |
KUserOrGroupId (const KUserOrGroupId< void * > &other) | |
KUserOrGroupId (NativeType nativeId) | |
KUserOrGroupId (void *nativeId) | |
KUserOrGroupId & | operator= (const KUserOrGroupId< T > &other) |
KUserOrGroupId< void * > & | operator= (const KUserOrGroupId< void * > &other) |
Detailed Description
struct KUserOrGroupId< T >
A platform independent user or group ID.
This struct is required since Windows does not have an integer uid_t/gid_t type but instead uses an opaque binary blob (SID) which must free allocated memory. On UNIX this is simply a uid_t/gid_t and all operations are inline, so there is no runtime overhead over using the uid_t/gid_t directly. On Windows this is an implicitly shared class that frees the underlying SID once no more references remain.
Unlike KUser/KUserGroup this does not query additional information, it is simply an abstraction over the native user/group ID type. If more information is necessary, a KUser or KUserGroup instance can be constructed from this ID
Member Typedef Documentation
◆ NativeType
typedef T KUserOrGroupId< T >::NativeType |
Constructor & Destructor Documentation
◆ KUserOrGroupId() [1/6]
|
inlineprotected |
Creates an invalid KUserOrGroupId.
◆ KUserOrGroupId() [2/6]
|
explicitprotected |
Creates a KUserOrGroupId from a native user/group ID.
On windows this will not take ownership over the passed SID, a copy will be created instead.
◆ KUserOrGroupId() [3/6]
|
inlineprotected |
◆ ~KUserOrGroupId() [1/2]
|
inlineprotected |
◆ KUserOrGroupId() [4/6]
|
protected |
Definition at line 737 of file kuser_win.cpp.
◆ ~KUserOrGroupId() [2/2]
|
protected |
Definition at line 742 of file kuser_win.cpp.
◆ KUserOrGroupId() [5/6]
|
protected |
Definition at line 747 of file kuser_win.cpp.
◆ KUserOrGroupId() [6/6]
|
protected |
Definition at line 760 of file kuser_win.cpp.
Member Function Documentation
◆ isValid() [1/2]
|
inline |
◆ isValid() [2/2]
bool KUserOrGroupId< void * >::isValid | ( | ) | const |
Definition at line 766 of file kuser_win.cpp.
◆ nativeId() [1/2]
|
inline |
- Returns
- A user/group ID that can be used in operating system specific functions
- Note
- On Windows the returned pointer will be freed once the last KUserOrGroupId referencing this user/group ID is deleted. Make sure that the KUserOrGroupId object remains valid as long as the native pointer is needed.
◆ nativeId() [2/2]
void * KUserOrGroupId< void * >::nativeId | ( | ) | const |
Definition at line 772 of file kuser_win.cpp.
◆ operator!=() [1/2]
|
inline |
- Returns
- whether this KUserOrGroupId is not equal to
other
◆ operator!=() [2/2]
bool KUserOrGroupId< void * >::operator!= | ( | const KUserOrGroupId< void * > & | other | ) | const |
Definition at line 793 of file kuser_win.cpp.
◆ operator=() [1/2]
|
inlineprotected |
◆ operator=() [2/2]
|
inlineprotected |
Definition at line 753 of file kuser_win.cpp.
◆ operator==() [1/2]
|
inline |
- Returns
- whether this KUserOrGroupId is equal to
other
◆ operator==() [2/2]
bool KUserOrGroupId< void * >::operator== | ( | const KUserOrGroupId< void * > & | other | ) | const |
Definition at line 781 of file kuser_win.cpp.
◆ toString() [1/2]
|
inline |
◆ toString() [2/2]
QString KUserOrGroupId< void * >::toString | ( | ) | const |
Definition at line 799 of file kuser_win.cpp.
The documentation for this struct was generated from the following file:
Documentation copyright © 1996-2025 The KDE developers.
Generated on Fri May 2 2025 11:53:00 by doxygen 1.13.2 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.