KModifierKeyInfo
#include <KModifierKeyInfo>
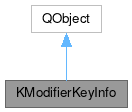
Signals | |
void | buttonPressed (Qt::MouseButton button, bool pressed) |
void | keyAdded (Qt::Key key) |
void | keyLatched (Qt::Key key, bool latched) |
void | keyLocked (Qt::Key key, bool locked) |
void | keyPressed (Qt::Key key, bool pressed) |
void | keyRemoved (Qt::Key key) |
Public Member Functions | |
KModifierKeyInfo (QObject *parent=nullptr) | |
~KModifierKeyInfo () override | |
bool | isButtonPressed (Qt::MouseButton button) const |
bool | isKeyLatched (Qt::Key key) const |
bool | isKeyLocked (Qt::Key key) const |
bool | isKeyPressed (Qt::Key key) const |
const QList< Qt::Key > | knownKeys () const |
bool | knowsKey (Qt::Key key) const |
bool | setKeyLatched (Qt::Key key, bool latched) |
bool | setKeyLocked (Qt::Key key, bool locked) |
![]() | |
QObject (QObject *parent) | |
QBindable< QString > | bindableObjectName () |
bool | blockSignals (bool block) |
const QObjectList & | children () const const |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) const const |
bool | disconnect (const QObject *receiver, const char *method) const const |
void | dumpObjectInfo () const const |
void | dumpObjectTree () const const |
QList< QByteArray > | dynamicPropertyNames () const const |
virtual bool | event (QEvent *e) |
virtual bool | eventFilter (QObject *watched, QEvent *event) |
T | findChild (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QRegularExpression &re, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (Qt::FindChildOptions options) const const |
bool | inherits (const char *className) const const |
void | installEventFilter (QObject *filterObj) |
bool | isQuickItemType () const const |
bool | isWidgetType () const const |
bool | isWindowType () const const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const const |
void | moveToThread (QThread *targetThread) |
QString | objectName () const const |
void | objectNameChanged (const QString &objectName) |
QObject * | parent () const const |
QVariant | property (const char *name) const const |
Q_CLASSINFO (Name, Value) | |
Q_EMIT Q_EMIT | |
Q_ENUM (...) | |
Q_ENUM_NS (...) | |
Q_ENUMS (...) | |
Q_FLAG (...) | |
Q_FLAG_NS (...) | |
Q_FLAGS (...) | |
Q_GADGET Q_GADGET | |
Q_GADGET_EXPORT (EXPORT_MACRO) | |
Q_INTERFACES (...) | |
Q_INVOKABLE Q_INVOKABLE | |
Q_MOC_INCLUDE Q_MOC_INCLUDE | |
Q_NAMESPACE Q_NAMESPACE | |
Q_NAMESPACE_EXPORT (EXPORT_MACRO) | |
Q_OBJECT Q_OBJECT | |
Q_PROPERTY (...) | |
Q_REVISION Q_REVISION | |
Q_SET_OBJECT_NAME (Object) | |
Q_SIGNAL Q_SIGNAL | |
Q_SIGNALS Q_SIGNALS | |
Q_SLOT Q_SLOT | |
Q_SLOTS Q_SLOTS | |
T | qobject_cast (const QObject *object) |
T | qobject_cast (QObject *object) |
QT_NO_NARROWING_CONVERSIONS_IN_CONNECT QT_NO_NARROWING_CONVERSIONS_IN_CONNECT | |
void | removeEventFilter (QObject *obj) |
void | setObjectName (const QString &name) |
void | setObjectName (QAnyStringView name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | setProperty (const char *name, QVariant &&value) |
bool | signalsBlocked () const const |
int | startTimer (int interval, Qt::TimerType timerType) |
int | startTimer (std::chrono::milliseconds interval, Qt::TimerType timerType) |
QThread * | thread () const const |
Additional Inherited Members | |
![]() | |
objectName | |
![]() | |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *context, Functor functor, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, Functor functor) |
bool | disconnect (const QMetaObject::Connection &connection) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
bool | disconnect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
![]() | |
typedef | QObjectList |
![]() | |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const QMetaMethod &signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const QMetaMethod &signal) |
bool | isSignalConnected (const QMetaMethod &signal) const const |
int | receivers (const char *signal) const const |
QObject * | sender () const const |
int | senderSignalIndex () const const |
virtual void | timerEvent (QTimerEvent *event) |
Detailed Description
Get information about the state of the keyboard's modifier keys.
This class provides cross-platform information about the state of the keyboard's modifier keys and the mouse buttons and allows to change the state as well.
It recognizes two states a key can be in:
- locked: eg. caps-locked (a.k.a. toggled)
- latched the key is temporarily locked but will be unlocked upon the next keypress.
An application can either query the states synchronously (isKeyLatched(), isKeyLocked()) or connect to KModifierKeyInfo's signals to be notified about changes (keyLatched(), keyLocked()).
Definition at line 35 of file kmodifierkeyinfo.h.
Constructor & Destructor Documentation
◆ KModifierKeyInfo()
|
explicit |
Default constructor.
Definition at line 41 of file kmodifierkeyinfo.cpp.
◆ ~KModifierKeyInfo()
|
override |
Destructor.
Definition at line 53 of file kmodifierkeyinfo.cpp.
Member Function Documentation
◆ buttonPressed
|
signal |
This signal is emitted whenever the pressed state of a mouse button changes (mouse button press or release).
- Parameters
-
button The mouse button that changed state pressed true if the mouse button is now pressed, false if is released.
◆ isButtonPressed()
bool KModifierKeyInfo::isButtonPressed | ( | Qt::MouseButton | button | ) | const |
Synchronously check if a mouse button is pressed.
- Parameters
-
button The mouse button to check
- Returns
- true if the mouse button is pressed, false if the mouse button is not pressed or its state is unknown.
Definition at line 92 of file kmodifierkeyinfo.cpp.
◆ isKeyLatched()
bool KModifierKeyInfo::isKeyLatched | ( | Qt::Key | key | ) | const |
Synchronously check if a key is latched.
- Parameters
-
key The key to check
- Returns
- true if the key is latched, false if the key is not latched or unknown.
- See also
- isKeyPressed,
- isKeyLocked,
- keyLatched
Definition at line 72 of file kmodifierkeyinfo.cpp.
◆ isKeyLocked()
bool KModifierKeyInfo::isKeyLocked | ( | Qt::Key | key | ) | const |
Synchronously check if a key is locked.
- Parameters
-
key The key to check
- Returns
- true if the key is locked, false if the key is not locked or unknown.
- See also
- isKeyPressed,
- isKeyLatched,
- keyLocked
Definition at line 82 of file kmodifierkeyinfo.cpp.
◆ isKeyPressed()
bool KModifierKeyInfo::isKeyPressed | ( | Qt::Key | key | ) | const |
Synchronously check if a key is pressed.
- Parameters
-
key The key to check
- Returns
- true if the key is pressed, false if the key is not pressed or unknown.
- See also
- isKeyLatched,
- isKeyLocked,
- keyPressed
Definition at line 67 of file kmodifierkeyinfo.cpp.
◆ keyAdded
|
signal |
This signal is emitted whenever a new modifier is found due to the keyboard mapping changing.
- Parameters
-
key The key that was discovered
◆ keyLatched
|
signal |
This signal is emitted whenever the latched state of a key changes.
- Parameters
-
key The key that changed state latched true if the key is now latched, false if it isn't
◆ keyLocked
|
signal |
This signal is emitted whenever the locked state of a key changes.
- Parameters
-
key The key that changed state locked true if the key is now locked, false if it isn't
◆ keyPressed
|
signal |
This signal is emitted whenever the pressed state of a key changes (key press or key release).
- Parameters
-
key The key that changed state pressed true if the key is now pressed, false if is released.
◆ keyRemoved
|
signal |
This signal is emitted whenever a previously known modifier no longer exists due to the keyboard mapping changing.
- Parameters
-
key The key that vanished
◆ knownKeys()
Get a list of known keys.
- Returns
- A list of known keys of which states will be reported.
Definition at line 62 of file kmodifierkeyinfo.cpp.
◆ knowsKey()
bool KModifierKeyInfo::knowsKey | ( | Qt::Key | key | ) | const |
Check if a key is known by the underlying window system and can be queried.
- Parameters
-
key The key to check
- Returns
- true if the key is available, false if it is unknown
Definition at line 57 of file kmodifierkeyinfo.cpp.
◆ setKeyLatched()
bool KModifierKeyInfo::setKeyLatched | ( | Qt::Key | key, |
bool | latched ) |
Set the latched state of a key.
- Parameters
-
key The key to latch latched true to latch the key, false to unlatch it.
- Returns
- false if the key is unknown. true doesn't guarantee you the operation worked.
Definition at line 77 of file kmodifierkeyinfo.cpp.
◆ setKeyLocked()
bool KModifierKeyInfo::setKeyLocked | ( | Qt::Key | key, |
bool | locked ) |
Set the locked state of a key.
- Parameters
-
key The key to lock latched true to lock the key, false to unlock it.
- Returns
- false if the key is unknown. true doesn't guarantee you the operation worked.
Definition at line 87 of file kmodifierkeyinfo.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2025 The KDE developers.
Generated on Fri Apr 18 2025 12:15:35 by doxygen 1.13.2 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.