KSharedPixmapCacheMixin
#include <kimagecache.h>
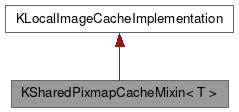
Public Member Functions | |
KSharedPixmapCacheMixin (const QString &cacheName, unsigned defaultCacheSize, unsigned expectedItemSize=0) | |
void | clear () |
bool | findImage (const QString &key, QImage *destination) const |
bool | findPixmap (const QString &key, QPixmap *destination) const |
bool | insertImage (const QString &key, const QImage &image) |
bool | insertPixmap (const QString &key, const QPixmap &pixmap) |
QDateTime | lastModifiedTime () const |
int | pixmapCacheLimit () const |
bool | pixmapCaching () const |
void | setPixmapCacheLimit (int size) |
void | setPixmapCaching (bool enable) |
Detailed Description
class KSharedPixmapCacheMixin< T >
A simple wrapping layer over KSharedDataCache to support caching images and pixmaps.
This class can be used to share images between different processes, which is useful when it is known that such images will be used across many processes, or when creating the image is expensive.
In addition, the class also supports caching QPixmaps in a single process using the setPixmapCaching() function.
Tips for use: If you already have QPixmaps that you intend to use, and you do not need access to the actual image data, then try to store and retrieve QPixmaps for use.
On the other hand, if you will need to store and retrieve actual image data (to modify the image after retrieval for instance) then you should use QImage to save the conversion cost from QPixmap to QImage.
KSharedPixmapCacheMixin is a subclass of KSharedDataCache, so all of the methods that can be used with KSharedDataCache can be used with KSharedPixmapCacheMixin, with the exception of KSharedDataCache::insert() and KSharedDataCache::find().
- Since
- 4.5
Definition at line 57 of file kimagecache.h.
Constructor & Destructor Documentation
◆ KSharedPixmapCacheMixin()
|
inline |
Constructs an image cache, named by cacheName
, with a default size of defaultCacheSize
.
- Parameters
-
cacheName Name of the cache to use. defaultCacheSize The default size, in bytes, of the cache. The actual on-disk size will be slightly larger. If the cache already exists, it will not be resized. If it is required to resize the cache then use the deleteCache() function to remove that cache first. expectedItemSize The expected general size of the items to be added to the image cache, in bytes. Use 0 if you just want a default item size.
Definition at line 73 of file kimagecache.h.
Member Function Documentation
◆ clear()
|
inline |
Removes all entries from the cache.
In addition any cached pixmaps (as per setPixmapCaching()) are also removed.
Definition at line 176 of file kimagecache.h.
◆ findImage()
|
inline |
Copies the cached image identified by key
to destination
.
If no such image exists destination
is unchanged.
- Returns
- true if the image identified by
key
existed, false otherwise.
Definition at line 158 of file kimagecache.h.
◆ findPixmap()
|
inline |
Copies the cached pixmap identified by key
to destination
.
If no such pixmap exists destination
is unchanged.
- Returns
- true if the pixmap identified by
key
existed, false otherwise.
- See also
- setPixmapCaching()
Definition at line 131 of file kimagecache.h.
◆ insertImage()
|
inline |
Inserts the image
into the shared cache, accessible with key
.
This variant is preferred over insertPixmap() if your source data is already a QImage, if it is essential that the image be in shared memory (such as for SVG icons which have a high render time), or if it will need to be in QImage form after it is retrieved from the cache.
- Parameters
-
key Name to access image
with.image The image to add to the shared cache.
- Returns
- true if the image was successfully cached, false otherwise.
Definition at line 114 of file kimagecache.h.
◆ insertPixmap()
|
inline |
Inserts the pixmap given by pixmap
to the cache, accessible with key
.
The pixmap must be converted to a QImage in order to be stored into shared memory. In order to prevent unnecessary conversions from taking place pixmap
will also be cached (but not in shared memory) and would be accessible using findPixmap() if pixmap caching is enabled.
- Parameters
-
key Name to access pixmap
with.pixmap The pixmap to add to the cache.
- Returns
- true if the pixmap was successfully cached, false otherwise.
- See also
- setPixmapCaching()
Definition at line 92 of file kimagecache.h.
◆ lastModifiedTime()
QDateTime KLocalImageCacheImplementation::lastModifiedTime | ( | ) | const |
- Returns
- The time that an image or pixmap was last inserted into a cache.
Definition at line 35 of file klocalimagecacheimpl.cpp.
◆ pixmapCacheLimit()
int KLocalImageCacheImplementation::pixmapCacheLimit | ( | ) | const |
- Returns
- The highest memory size in bytes to be used by cached pixmaps.
- Since
- 4.6
Definition at line 40 of file klocalimagecacheimpl.cpp.
◆ pixmapCaching()
bool KLocalImageCacheImplementation::pixmapCaching | ( | ) | const |
- Returns
- if QPixmaps added with insertPixmap() will be stored in a local pixmap cache as well as the shared image cache. The default is to cache pixmaps locally.
Definition at line 37 of file klocalimagecacheimpl.cpp.
◆ setPixmapCacheLimit()
void KLocalImageCacheImplementation::setPixmapCacheLimit | ( | int | size | ) |
Sets the highest memory size the pixmap cache should use.
- Parameters
-
size The size in bytes
- Since
- 4.6
Definition at line 41 of file klocalimagecacheimpl.cpp.
◆ setPixmapCaching()
void KLocalImageCacheImplementation::setPixmapCaching | ( | bool | enable | ) |
Enables or disables local pixmap caching.
If it is anticipated that a pixmap will be frequently needed then this can actually save memory overall since the X server or graphics card will not have to store duplicate copies of the same image.
- Parameters
-
enable Enables pixmap caching if true, disables otherwise.
Definition at line 38 of file klocalimagecacheimpl.cpp.
The documentation for this class was generated from the following file:
Documentation copyright © 1996-2025 The KDE developers.
Generated on Fri Apr 18 2025 12:15:35 by doxygen 1.13.2 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.