KIO::JobUiDelegate
#include <KIO/JobUiDelegate>
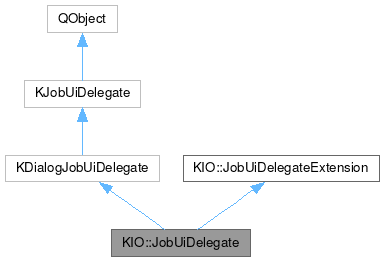
Public Member Functions | |
~JobUiDelegate () override | |
bool | askDeleteConfirmation (const QList< QUrl > &urls, DeletionType deletionType, ConfirmationType confirmationType) override |
ClipboardUpdater * | createClipboardUpdater (Job *job, ClipboardUpdaterMode mode) override |
void | setWindow (QWidget *window) override |
void | updateUrlInClipboard (const QUrl &src, const QUrl &dest) override |
![]() | |
KDialogJobUiDelegate (KJobUiDelegate::Flags flags, QWidget *window) | |
bool | setJob (KJob *job) override |
void | updateUserTimestamp (unsigned long time) |
unsigned long | userTimestamp () const |
QWidget * | window () const |
![]() | |
KJobUiDelegate (Flags flags={KJobUiDelegate::AutoHandlingDisabled}) | |
bool | isAutoErrorHandlingEnabled () const |
bool | isAutoWarningHandlingEnabled () const |
void | setAutoErrorHandlingEnabled (bool enable) |
void | setAutoWarningHandlingEnabled (bool enable) |
virtual void | showErrorMessage () |
![]() | |
QObject (QObject *parent) | |
QBindable< QString > | bindableObjectName () |
bool | blockSignals (bool block) |
const QObjectList & | children () const const |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) const const |
bool | disconnect (const QObject *receiver, const char *method) const const |
void | dumpObjectInfo () const const |
void | dumpObjectTree () const const |
QList< QByteArray > | dynamicPropertyNames () const const |
virtual bool | event (QEvent *e) |
virtual bool | eventFilter (QObject *watched, QEvent *event) |
T | findChild (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QRegularExpression &re, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (Qt::FindChildOptions options) const const |
bool | inherits (const char *className) const const |
void | installEventFilter (QObject *filterObj) |
bool | isQuickItemType () const const |
bool | isWidgetType () const const |
bool | isWindowType () const const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const const |
void | moveToThread (QThread *targetThread) |
QString | objectName () const const |
void | objectNameChanged (const QString &objectName) |
QObject * | parent () const const |
QVariant | property (const char *name) const const |
Q_CLASSINFO (Name, Value) | |
Q_EMIT Q_EMIT | |
Q_ENUM (...) | |
Q_ENUM_NS (...) | |
Q_ENUMS (...) | |
Q_FLAG (...) | |
Q_FLAG_NS (...) | |
Q_FLAGS (...) | |
Q_GADGET Q_GADGET | |
Q_GADGET_EXPORT (EXPORT_MACRO) | |
Q_INTERFACES (...) | |
Q_INVOKABLE Q_INVOKABLE | |
Q_MOC_INCLUDE Q_MOC_INCLUDE | |
Q_NAMESPACE Q_NAMESPACE | |
Q_NAMESPACE_EXPORT (EXPORT_MACRO) | |
Q_OBJECT Q_OBJECT | |
Q_PROPERTY (...) | |
Q_REVISION Q_REVISION | |
Q_SET_OBJECT_NAME (Object) | |
Q_SIGNAL Q_SIGNAL | |
Q_SIGNALS Q_SIGNALS | |
Q_SLOT Q_SLOT | |
Q_SLOTS Q_SLOTS | |
T | qobject_cast (const QObject *object) |
T | qobject_cast (QObject *object) |
QT_NO_NARROWING_CONVERSIONS_IN_CONNECT QT_NO_NARROWING_CONVERSIONS_IN_CONNECT | |
void | removeEventFilter (QObject *obj) |
void | setObjectName (const QString &name) |
void | setObjectName (QAnyStringView name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | setProperty (const char *name, QVariant &&value) |
bool | signalsBlocked () const const |
int | startTimer (int interval, Qt::TimerType timerType) |
int | startTimer (std::chrono::milliseconds interval, Qt::TimerType timerType) |
QThread * | thread () const const |
![]() |
Static Public Member Functions | |
static void | unregisterWindow (QWidget *window) |
![]() | |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *context, Functor functor, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, Functor functor) |
bool | disconnect (const QMetaObject::Connection &connection) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
bool | disconnect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
Protected Member Functions | |
JobUiDelegate (KJobUiDelegate::Flags flags=AutoHandlingDisabled, QWidget *window=nullptr, const QList< QObject * > &ifaces={}) | |
![]() | |
KJob * | job () const |
![]() | |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const QMetaMethod &signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const QMetaMethod &signal) |
bool | isSignalConnected (const QMetaMethod &signal) const const |
int | receivers (const char *signal) const const |
QObject * | sender () const const |
int | senderSignalIndex () const const |
virtual void | timerEvent (QTimerEvent *event) |
![]() | |
JobUiDelegateExtension () | |
virtual | ~JobUiDelegateExtension () |
Additional Inherited Members | |
![]() | |
enum | Flag |
typedef QFlags< Flag > | Flags |
![]() | |
typedef | QObjectList |
![]() | |
enum | ClipboardUpdaterMode { UpdateContent , OverwriteContent , RemoveContent } |
enum | ConfirmationType { DefaultConfirmation , ForceConfirmation } |
enum | DeletionType { Delete , Trash , EmptyTrash } |
![]() | |
objectName | |
![]() | |
AutoErrorHandlingEnabled | |
AutoHandlingDisabled | |
AutoHandlingEnabled | |
AutoWarningHandlingEnabled | |
Detailed Description
A UI delegate tuned to be used with KIO Jobs.
Constructor & Destructor Documentation
◆ JobUiDelegate()
|
explicitprotected |
Constructs a new KIO Job UI delegate.
- Parameters
-
flags allows to enable automatic error/warning handling window the window associated with this delegate, see setWindow. ifaces Interface instances such as OpenWithHandlerInterface to replace the default interfaces
- Since
- 5.98
Definition at line 319 of file jobuidelegate.cpp.
◆ ~JobUiDelegate()
Member Function Documentation
◆ askDeleteConfirmation()
|
overridevirtual |
Ask for confirmation before deleting/trashing urls
.
Note that this method is not called automatically by KIO jobs. It's the application's responsibility to ask the user for confirmation before calling KIO::del() or KIO::trash().
- Parameters
-
urls the urls about to be deleted/trashed deletionType the type of deletion (Delete for real deletion, Trash otherwise) confirmation see ConfirmationType. Normally set to DefaultConfirmation. Note: the window passed to setWindow is used as the parent for the message box.
- Returns
- true if confirmed
Implements KIO::JobUiDelegateExtension.
Definition at line 178 of file jobuidelegate.cpp.
◆ createClipboardUpdater()
|
overridevirtual |
Creates a clipboard updater.
Reimplemented from KIO::JobUiDelegateExtension.
Definition at line 304 of file jobuidelegate.cpp.
◆ setWindow()
|
overridevirtual |
Associate this job with a window given by window
.
- Parameters
-
window the window to associate to
- See also
- window()
Reimplemented from KDialogJobUiDelegate.
Definition at line 153 of file jobuidelegate.cpp.
◆ unregisterWindow()
|
static |
Unregister the given window from kded.
This is normally done automatically when the window is destroyed.
This method is useful for instance when keeping a hidden window around to make it faster to reuse later.
- Since
- 5.2
Definition at line 173 of file jobuidelegate.cpp.
◆ updateUrlInClipboard()
|
overridevirtual |
Update URL in clipboard, if present.
Reimplemented from KIO::JobUiDelegateExtension.
Definition at line 312 of file jobuidelegate.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2024 The KDE developers.
Generated on Fri Nov 29 2024 11:50:33 by doxygen 1.12.0 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.