KIO::JobUiDelegateExtension
#include <KIO/JobUiDelegateExtension>
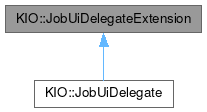
Public Types | |
enum | ClipboardUpdaterMode { UpdateContent , OverwriteContent , RemoveContent } |
enum | ConfirmationType { DefaultConfirmation , ForceConfirmation } |
enum | DeletionType { Delete , Trash , EmptyTrash } |
Public Member Functions | |
virtual bool | askDeleteConfirmation (const QList< QUrl > &urls, DeletionType deletionType, ConfirmationType confirmationType)=0 |
virtual ClipboardUpdater * | createClipboardUpdater (Job *job, ClipboardUpdaterMode mode) |
virtual void | updateUrlInClipboard (const QUrl &src, const QUrl &dest) |
Protected Member Functions | |
JobUiDelegateExtension () | |
virtual | ~JobUiDelegateExtension () |
Detailed Description
An abstract class defining interaction with users from KIO jobs:
- asking for confirmation before deleting files or directories
- Since
- 5.0
Member Enumeration Documentation
◆ ClipboardUpdaterMode
enum KIO::JobUiDelegateExtension::ClipboardUpdaterMode |
Definition at line 179 of file jobuidelegateextension.h.
◆ ConfirmationType
ForceConfirmation: always ask the user for confirmation DefaultConfirmation: don't ask the user if he/she said "don't ask again".
Used by askDeleteConfirmation.
Definition at line 161 of file jobuidelegateextension.h.
◆ DeletionType
The type of deletion: real deletion, moving the files to the trash or emptying the trash Used by askDeleteConfirmation.
Definition at line 150 of file jobuidelegateextension.h.
Constructor & Destructor Documentation
◆ JobUiDelegateExtension()
|
protected |
Constructor.
Definition at line 12 of file jobuidelegateextension.cpp.
◆ ~JobUiDelegateExtension()
|
protectedvirtual |
Destructor.
Definition at line 17 of file jobuidelegateextension.cpp.
Member Function Documentation
◆ askDeleteConfirmation()
|
pure virtual |
Ask for confirmation before deleting/trashing urls
.
Note that this method is not called automatically by KIO jobs. It's the application's responsibility to ask the user for confirmation before calling KIO::del() or KIO::trash().
- Parameters
-
urls the urls about to be deleted/trashed deletionType the type of deletion (Delete for real deletion, Trash otherwise) confirmationType see ConfirmationType. Normally set to DefaultConfirmation. Note: the window passed to setWindow is used as the parent for the message box.
- Returns
- true if confirmed
Implemented in KIO::JobUiDelegate.
◆ createClipboardUpdater()
|
virtual |
Creates a clipboard updater as a child of the given job.
Reimplemented in KIO::JobUiDelegate.
Definition at line 21 of file jobuidelegateextension.cpp.
◆ updateUrlInClipboard()
|
virtual |
Update URL in clipboard, if present.
Reimplemented in KIO::JobUiDelegate.
Definition at line 26 of file jobuidelegateextension.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2025 The KDE developers.
Generated on Fri Jan 3 2025 11:56:16 by doxygen 1.12.0 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.