ColumnView
#include <columnview.h>
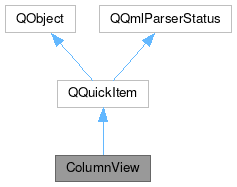
Public Types | |
enum | ColumnResizeMode { FixedColumns = 0 , DynamicColumns , SingleColumn } |
![]() | |
enum | Flag |
typedef | Flags |
enum | ItemChange |
enum | TransformOrigin |
![]() | |
typedef | QObjectList |
Properties | |
bool | acceptsMouse |
qreal | bottomPadding |
QML_ELEMENTColumnResizeMode | columnResizeMode |
qreal | columnWidth |
QQmlListProperty< QQuickItem > | contentChildren |
QQmlListProperty< QObject > | contentData |
QQuickItem * | contentItem |
qreal | contentWidth |
qreal | contentX |
int | count |
int | currentIndex |
QQuickItem * | currentItem |
bool | dragging |
bool | interactive |
QQuickItem * | leadingVisibleItem |
bool | moving |
int | scrollDuration |
bool | separatorVisible |
qreal | topPadding |
QQuickItem * | trailingVisibleItem |
QList< QQuickItem * > | visibleItems |
![]() | |
activeFocus | |
activeFocusOnTab | |
antialiasing | |
baselineOffset | |
childrenRect | |
clip | |
containmentMask | |
enabled | |
focus | |
height | |
implicitHeight | |
implicitWidth | |
opacity | |
parent | |
rotation | |
scale | |
smooth | |
state | |
transformOrigin | |
visible | |
width | |
x | |
y | |
z | |
![]() | |
objectName | |
Signals | |
void | acceptsMouseChanged () |
void | bottomPaddingChanged () |
void | columnResizeModeChanged () |
void | columnWidthChanged () |
void | contentChildrenChanged () |
void | contentWidthChanged () |
void | contentXChanged () |
void | countChanged () |
void | currentIndexChanged () |
void | currentItemChanged () |
void | draggingChanged () |
void | interactiveChanged () |
void | itemInserted (int position, QQuickItem *item) |
void | itemRemoved (QQuickItem *item) |
void | leadingVisibleItemChanged () |
void | movingChanged () |
void | scrollDurationChanged () |
void | separatorVisibleChanged () |
void | topPaddingChanged () |
void | trailingVisibleItemChanged () |
void | visibleItemsChanged () |
Public Slots | |
void | addItem (QQuickItem *item) |
void | clear () |
bool | containsItem (QQuickItem *item) |
void | insertItem (int pos, QQuickItem *item) |
QQuickItem * | itemAt (qreal x, qreal y) |
void | moveItem (int from, int to) |
Q_INVOKABLE QQuickItem * | removeItem (const QVariant &item) |
void | replaceItem (int pos, QQuickItem *item) |
Public Member Functions | |
ColumnView (QQuickItem *parent=nullptr) | |
bool | acceptsMouse () const |
qreal | bottomPadding () const |
ColumnResizeMode | columnResizeMode () const |
qreal | columnWidth () const |
QQmlListProperty< QQuickItem > | contentChildren () |
QQmlListProperty< QObject > | contentData () |
QQuickItem * | contentItem () const |
qreal | contentWidth () const |
qreal | contentX () const |
int | count () const |
int | currentIndex () const |
QQuickItem * | currentItem () |
bool | dragging () const |
bool | interactive () const |
QQuickItem * | leadingVisibleItem () const |
bool | moving () const |
Q_INVOKABLE QQuickItem * | pop () |
Q_INVOKABLE QQuickItem * | pop (const QVariant &item) |
QQuickItem * | pop (int index) |
QQuickItem * | pop (QQuickItem *item) |
QQuickItem * | removeItem (int index) |
QQuickItem * | removeItem (QQuickItem *item) |
int | scrollDuration () const |
bool | separatorVisible () const |
void | setAcceptsMouse (bool accepts) |
void | setBottomPadding (qreal padding) |
void | setColumnResizeMode (ColumnResizeMode mode) |
void | setColumnWidth (qreal width) |
void | setContentX (qreal x) const |
void | setCurrentIndex (int index) |
void | setInteractive (bool interactive) |
void | setScrollDuration (int duration) |
void | setSeparatorVisible (bool visible) |
void | setTopPadding (qreal padding) |
qreal | topPadding () const |
QQuickItem * | trailingVisibleItem () const |
QList< QQuickItem * > | visibleItems () const |
![]() | |
QQuickItem (QQuickItem *parent) | |
Qt::MouseButtons | acceptedMouseButtons () const const |
bool | acceptHoverEvents () const const |
bool | acceptTouchEvents () const const |
void | activeFocusChanged (bool) |
bool | activeFocusOnTab () const const |
void | activeFocusOnTabChanged (bool) |
bool | antialiasing () const const |
void | antialiasingChanged (bool) |
qreal | baselineOffset () const const |
void | baselineOffsetChanged (qreal) |
QBindable< qreal > | bindableHeight () |
QBindable< qreal > | bindableWidth () |
QBindable< qreal > | bindableX () |
QBindable< qreal > | bindableY () |
virtual QRectF | boundingRect () const const |
QQuickItem * | childAt (qreal x, qreal y) const const |
QList< QQuickItem * > | childItems () const const |
QRectF | childrenRect () |
void | childrenRectChanged (const QRectF &) |
bool | clip () const const |
void | clipChanged (bool) |
virtual QRectF | clipRect () const const |
QObject * | containmentMask () const const |
void | containmentMaskChanged () |
virtual bool | contains (const QPointF &point) const const |
QCursor | cursor () const const |
void | dumpItemTree () const const |
void | enabledChanged () |
void | ensurePolished () |
bool | filtersChildMouseEvents () const const |
Flags | flags () const const |
void | focusChanged (bool) |
void | forceActiveFocus () |
void | forceActiveFocus (Qt::FocusReason reason) |
void | grabMouse () |
QSharedPointer< QQuickItemGrabResult > | grabToImage (const QSize &targetSize) |
void | grabTouchPoints (const QList< int > &ids) |
bool | hasActiveFocus () const const |
bool | hasFocus () const const |
qreal | height () const const |
void | heightChanged () |
qreal | implicitHeight () const const |
void | implicitHeightChanged () |
qreal | implicitWidth () const const |
void | implicitWidthChanged () |
virtual QVariant | inputMethodQuery (Qt::InputMethodQuery query) const const |
bool | isAncestorOf (const QQuickItem *child) const const |
bool | isEnabled () const const |
bool | isFocusScope () const const |
virtual bool | isTextureProvider () const const |
bool | isVisible () const const |
bool | keepMouseGrab () const const |
bool | keepTouchGrab () const const |
QPointF | mapFromGlobal (const QPointF &point) const const |
QPointF | mapFromItem (const QQuickItem *item, const QPointF &point) const const |
QPointF | mapFromScene (const QPointF &point) const const |
QRectF | mapRectFromItem (const QQuickItem *item, const QRectF &rect) const const |
QRectF | mapRectFromScene (const QRectF &rect) const const |
QRectF | mapRectToItem (const QQuickItem *item, const QRectF &rect) const const |
QRectF | mapRectToScene (const QRectF &rect) const const |
QPointF | mapToGlobal (const QPointF &point) const const |
QPointF | mapToItem (const QQuickItem *item, const QPointF &point) const const |
QPointF | mapToScene (const QPointF &point) const const |
QQuickItem * | nextItemInFocusChain (bool forward) |
qreal | opacity () const const |
void | opacityChanged () |
void | parentChanged (QQuickItem *) |
QQuickItem * | parentItem () const const |
void | polish () |
void | resetAntialiasing () |
void | resetHeight () |
void | resetWidth () |
qreal | rotation () const const |
void | rotationChanged () |
qreal | scale () const const |
void | scaleChanged () |
QQuickItem * | scopedFocusItem () const const |
void | setAcceptedMouseButtons (Qt::MouseButtons buttons) |
void | setAcceptHoverEvents (bool enabled) |
void | setAcceptTouchEvents (bool enabled) |
void | setActiveFocusOnTab (bool) |
void | setAntialiasing (bool) |
void | setBaselineOffset (qreal) |
void | setClip (bool) |
void | setContainmentMask (QObject *mask) |
void | setCursor (const QCursor &cursor) |
void | setEnabled (bool) |
void | setFiltersChildMouseEvents (bool filter) |
void | setFlag (Flag flag, bool enabled) |
void | setFlags (Flags flags) |
void | setFocus (bool focus, Qt::FocusReason reason) |
void | setFocus (bool) |
void | setHeight (qreal) |
void | setImplicitHeight (qreal) |
void | setImplicitWidth (qreal) |
void | setKeepMouseGrab (bool keep) |
void | setKeepTouchGrab (bool keep) |
void | setOpacity (qreal) |
void | setParentItem (QQuickItem *parent) |
void | setRotation (qreal) |
void | setScale (qreal) |
void | setSize (const QSizeF &size) |
void | setSmooth (bool) |
void | setState (const QString &) |
void | setTransformOrigin (TransformOrigin) |
void | setVisible (bool) |
void | setWidth (qreal) |
void | setX (qreal) |
void | setY (qreal) |
void | setZ (qreal) |
QSizeF | size () const const |
bool | smooth () const const |
void | smoothChanged (bool) |
void | stackAfter (const QQuickItem *sibling) |
void | stackBefore (const QQuickItem *sibling) |
QString | state () const const |
void | stateChanged (const QString &) |
virtual QSGTextureProvider * | textureProvider () const const |
TransformOrigin | transformOrigin () const const |
void | transformOriginChanged (TransformOrigin) |
void | ungrabMouse () |
void | ungrabTouchPoints () |
void | unsetCursor () |
void | update () |
QQuickItem * | viewportItem () const const |
void | visibleChanged () |
qreal | width () const const |
void | widthChanged () |
QQuickWindow * | window () const const |
void | windowChanged (QQuickWindow *window) |
qreal | x () const const |
void | xChanged () |
qreal | y () const const |
void | yChanged () |
qreal | z () const const |
void | zChanged () |
![]() | |
QObject (QObject *parent) | |
QBindable< QString > | bindableObjectName () |
bool | blockSignals (bool block) |
const QObjectList & | children () const const |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) const const |
bool | disconnect (const QObject *receiver, const char *method) const const |
void | dumpObjectInfo () const const |
void | dumpObjectTree () const const |
QList< QByteArray > | dynamicPropertyNames () const const |
virtual bool | eventFilter (QObject *watched, QEvent *event) |
T | findChild (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QRegularExpression &re, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (Qt::FindChildOptions options) const const |
bool | inherits (const char *className) const const |
void | installEventFilter (QObject *filterObj) |
bool | isQuickItemType () const const |
bool | isWidgetType () const const |
bool | isWindowType () const const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const const |
void | moveToThread (QThread *targetThread) |
QString | objectName () const const |
void | objectNameChanged (const QString &objectName) |
QObject * | parent () const const |
QVariant | property (const char *name) const const |
Q_CLASSINFO (Name, Value) | |
Q_EMIT Q_EMIT | |
Q_ENUM (...) | |
Q_ENUM_NS (...) | |
Q_ENUMS (...) | |
Q_FLAG (...) | |
Q_FLAG_NS (...) | |
Q_FLAGS (...) | |
Q_GADGET Q_GADGET | |
Q_GADGET_EXPORT (EXPORT_MACRO) | |
Q_INTERFACES (...) | |
Q_INVOKABLE Q_INVOKABLE | |
Q_MOC_INCLUDE Q_MOC_INCLUDE | |
Q_NAMESPACE Q_NAMESPACE | |
Q_NAMESPACE_EXPORT (EXPORT_MACRO) | |
Q_OBJECT Q_OBJECT | |
Q_PROPERTY (...) | |
Q_REVISION Q_REVISION | |
Q_SET_OBJECT_NAME (Object) | |
Q_SIGNAL Q_SIGNAL | |
Q_SIGNALS Q_SIGNALS | |
Q_SLOT Q_SLOT | |
Q_SLOTS Q_SLOTS | |
T | qobject_cast (const QObject *object) |
T | qobject_cast (QObject *object) |
QT_NO_NARROWING_CONVERSIONS_IN_CONNECT QT_NO_NARROWING_CONVERSIONS_IN_CONNECT | |
void | removeEventFilter (QObject *obj) |
void | setObjectName (const QString &name) |
void | setObjectName (QAnyStringView name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | setProperty (const char *name, QVariant &&value) |
bool | signalsBlocked () const const |
int | startTimer (int interval, Qt::TimerType timerType) |
int | startTimer (std::chrono::milliseconds interval, Qt::TimerType timerType) |
QThread * | thread () const const |
![]() |
Static Public Member Functions | |
static ColumnViewAttached * | qmlAttachedProperties (QObject *object) |
![]() | |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *context, Functor functor, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, Functor functor) |
bool | disconnect (const QMetaObject::Connection &connection) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
bool | disconnect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
Protected Member Functions | |
bool | childMouseEventFilter (QQuickItem *item, QEvent *event) override |
void | classBegin () override |
void | componentComplete () override |
void | geometryChange (const QRectF &newGeometry, const QRectF &oldGeometry) override |
void | itemChange (QQuickItem::ItemChange change, const QQuickItem::ItemChangeData &value) override |
void | mouseMoveEvent (QMouseEvent *event) override |
void | mousePressEvent (QMouseEvent *event) override |
void | mouseReleaseEvent (QMouseEvent *event) override |
void | mouseUngrabEvent () override |
void | updatePolish () override |
![]() | |
virtual void | dragEnterEvent (QDragEnterEvent *event) |
virtual void | dragLeaveEvent (QDragLeaveEvent *event) |
virtual void | dragMoveEvent (QDragMoveEvent *event) |
virtual void | dropEvent (QDropEvent *event) |
virtual bool | event (QEvent *ev) override |
virtual void | focusInEvent (QFocusEvent *) |
virtual void | focusOutEvent (QFocusEvent *) |
bool | heightValid () const const |
virtual void | hoverEnterEvent (QHoverEvent *event) |
virtual void | hoverLeaveEvent (QHoverEvent *event) |
virtual void | hoverMoveEvent (QHoverEvent *event) |
virtual void | inputMethodEvent (QInputMethodEvent *event) |
bool | isComponentComplete () const const |
virtual void | keyPressEvent (QKeyEvent *event) |
virtual void | keyReleaseEvent (QKeyEvent *event) |
virtual void | mouseDoubleClickEvent (QMouseEvent *event) |
virtual void | releaseResources () |
virtual void | touchEvent (QTouchEvent *event) |
virtual void | touchUngrabEvent () |
void | updateInputMethod (Qt::InputMethodQueries queries) |
virtual QSGNode * | updatePaintNode (QSGNode *oldNode, UpdatePaintNodeData *updatePaintNodeData) |
virtual void | wheelEvent (QWheelEvent *event) |
bool | widthValid () const const |
![]() | |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const QMetaMethod &signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const QMetaMethod &signal) |
bool | isSignalConnected (const QMetaMethod &signal) const const |
int | receivers (const char *signal) const const |
QObject * | sender () const const |
int | senderSignalIndex () const const |
virtual void | timerEvent (QTimerEvent *event) |
Detailed Description
ColumnView is a container that lays out items horizontally in a row, when not all items fit in the ColumnView, it will behave like a Flickable and will be a scrollable view which shows only a determined number of columns.
The columns can either all have the same fixed size (recommended), size themselves with implicitWidth, or automatically expand to take all the available width: by default the last column will always be the expanding one. Items inside the ColumnView can access info of the view and set layouting hints via the ColumnView attached property.
This is the base for the implementation of PageRow
- Since
- 2.7
Definition at line 160 of file columnview.h.
Member Enumeration Documentation
◆ ColumnResizeMode
enum ColumnView::ColumnResizeMode |
Definition at line 278 of file columnview.h.
Property Documentation
◆ acceptsMouse
|
readwrite |
True if the contents can be dragged also with mouse besides touch.
Definition at line 264 of file columnview.h.
◆ bottomPadding
|
readwrite |
The padding this will have at the bottom.
Definition at line 218 of file columnview.h.
◆ columnResizeMode
|
readwrite |
The strategy to follow while automatically resizing the columns, the enum can have the following values:
- FixedColumns: every column is fixed at the same width of the columnWidth property
- DynamicColumns: columns take their width from their implicitWidth
- SingleColumn: only one column at a time is shown, as wide as the viewport, eventual reservedSpace on the column's attached property is ignored The default is FixedColumns.
Definition at line 174 of file columnview.h.
◆ columnWidth
|
readwrite |
The width of all columns when columnResizeMode is FixedColumns.
Definition at line 179 of file columnview.h.
◆ contentChildren
|
read |
Every column item the view contains.
Definition at line 270 of file columnview.h.
◆ contentData
|
read |
every item declared inside the view, both visual and non-visual items
Definition at line 274 of file columnview.h.
◆ contentItem
|
read |
The main content item of this view: it's the parent of the column items.
Definition at line 198 of file columnview.h.
◆ contentWidth
|
read |
The compound width of all columns in the view.
Definition at line 208 of file columnview.h.
◆ contentX
|
readwrite |
The value of the horizontal scroll of the view, in pixels.
Definition at line 203 of file columnview.h.
◆ count
|
read |
How many columns this view containsItem.
Definition at line 183 of file columnview.h.
◆ currentIndex
|
readwrite |
The position of the currently active column.
The current column will also have keyboard focus
Definition at line 188 of file columnview.h.
◆ currentItem
|
read |
The currently active column.
The current column will also have keyboard focus
Definition at line 193 of file columnview.h.
◆ dragging
|
read |
True when the user is dragging around with touch gestures the view contents.
Definition at line 249 of file columnview.h.
◆ interactive
|
readwrite |
True if it supports moving the contents by dragging.
Definition at line 259 of file columnview.h.
◆ leadingVisibleItem
|
read |
The first of visibleItems provided from convenience.
Definition at line 238 of file columnview.h.
◆ moving
|
read |
True both when the user is dragging around with touch gestures the view contents or the view is animating.
Definition at line 254 of file columnview.h.
◆ scrollDuration
|
readwrite |
The duration for scrolling animations.
Definition at line 223 of file columnview.h.
◆ separatorVisible
|
readwrite |
True if columns should be visually separated by a separator line.
Definition at line 228 of file columnview.h.
◆ topPadding
|
readwrite |
The padding this will have at the top.
Definition at line 213 of file columnview.h.
◆ trailingVisibleItem
|
read |
The last of visibleItems provided from convenience.
Definition at line 243 of file columnview.h.
◆ visibleItems
|
read |
The list of all visible column items that are at least partially in the viewport at any given moment.
Definition at line 233 of file columnview.h.
Constructor & Destructor Documentation
◆ ColumnView()
ColumnView::ColumnView | ( | QQuickItem * | parent = nullptr | ) |
Definition at line 966 of file columnview.cpp.
◆ ~ColumnView()
|
override |
Definition at line 996 of file columnview.cpp.
Member Function Documentation
◆ acceptsMouse()
bool ColumnView::acceptsMouse | ( | ) | const |
Definition at line 1247 of file columnview.cpp.
◆ addItem
|
slot |
Pushes a new item at the end of the view.
- Parameters
-
item the new item which will be reparented and managed
Definition at line 1273 of file columnview.cpp.
◆ bottomPadding()
qreal ColumnView::bottomPadding | ( | ) | const |
Definition at line 1142 of file columnview.cpp.
◆ childMouseEventFilter()
|
overrideprotectedvirtual |
Reimplemented from QQuickItem.
Definition at line 1538 of file columnview.cpp.
◆ classBegin()
|
overrideprotectedvirtual |
Reimplemented from QQuickItem.
Definition at line 1802 of file columnview.cpp.
◆ clear
|
slot |
Removes every item in the view.
Items will be reparented to their old parent. If they have JavaScript ownership and they didn't have an old parent, they will be destroyed
Definition at line 1500 of file columnview.cpp.
◆ columnResizeMode()
ColumnView::ColumnResizeMode ColumnView::columnResizeMode | ( | ) | const |
Definition at line 1000 of file columnview.cpp.
◆ columnWidth()
qreal ColumnView::columnWidth | ( | ) | const |
Definition at line 1020 of file columnview.cpp.
◆ componentComplete()
|
overrideprotectedvirtual |
Reimplemented from QQuickItem.
Definition at line 1823 of file columnview.cpp.
◆ containsItem
|
slot |
- Returns
- true if the view contains the given item
Definition at line 1512 of file columnview.cpp.
◆ contentItem()
QQuickItem * ColumnView::contentItem | ( | ) | const |
Definition at line 1158 of file columnview.cpp.
◆ contentWidth()
qreal ColumnView::contentWidth | ( | ) | const |
Definition at line 1206 of file columnview.cpp.
◆ contentX()
qreal ColumnView::contentX | ( | ) | const |
Definition at line 1211 of file columnview.cpp.
◆ count()
int ColumnView::count | ( | ) | const |
Definition at line 1121 of file columnview.cpp.
◆ currentIndex()
int ColumnView::currentIndex | ( | ) | const |
Definition at line 1040 of file columnview.cpp.
◆ dragging()
bool ColumnView::dragging | ( | ) | const |
Definition at line 1196 of file columnview.cpp.
◆ geometryChange()
|
overrideprotectedvirtual |
Reimplemented from QQuickItem.
Definition at line 1527 of file columnview.cpp.
◆ insertItem
|
slot |
Inserts a new item in the view at a given position.
The current Item will not be changed, currentIndex will be adjusted accordingly if needed to keep the same current item.
- Parameters
-
pos the position we want the new item to be inserted in item the new item which will be reparented and managed
Definition at line 1278 of file columnview.cpp.
◆ interactive()
bool ColumnView::interactive | ( | ) | const |
Definition at line 1221 of file columnview.cpp.
◆ itemAt
|
slot |
Returns the visible item containing the point x, y in content coordinates.
If there is no item at the point specified, or the item is not visible null is returned.
Definition at line 1517 of file columnview.cpp.
◆ itemChange()
|
overrideprotectedvirtual |
Reimplemented from QQuickItem.
Definition at line 1834 of file columnview.cpp.
◆ itemInserted
|
signal |
A new item has been inserted.
- Parameters
-
position where the page has been inserted item a pointer to the new item
◆ itemRemoved
|
signal |
An item has just been removed from the view.
- Parameters
-
item a pointer to the item that has just been removed
◆ leadingVisibleItem()
QQuickItem * ColumnView::leadingVisibleItem | ( | ) | const |
Definition at line 1103 of file columnview.cpp.
◆ mouseMoveEvent()
|
overrideprotectedvirtual |
Reimplemented from QQuickItem.
Definition at line 1724 of file columnview.cpp.
◆ mousePressEvent()
|
overrideprotectedvirtual |
Reimplemented from QQuickItem.
Definition at line 1700 of file columnview.cpp.
◆ mouseReleaseEvent()
|
overrideprotectedvirtual |
Reimplemented from QQuickItem.
Definition at line 1755 of file columnview.cpp.
◆ mouseUngrabEvent()
|
overrideprotectedvirtual |
Reimplemented from QQuickItem.
Definition at line 1785 of file columnview.cpp.
◆ moveItem
|
slot |
Move an item inside the view.
The currentIndex property may be changed in order to keep currentItem the same.
- Parameters
-
from the old position to the new position
Definition at line 1386 of file columnview.cpp.
◆ moving()
bool ColumnView::moving | ( | ) | const |
Definition at line 1201 of file columnview.cpp.
◆ pop() [1/4]
QQuickItem * ColumnView::pop | ( | ) |
This method removes the last item from the view and returns it.
This method calls removeItem() on the last item.
- See also
- removeItem()
- Returns
- the removed item
Definition at line 1492 of file columnview.cpp.
◆ pop() [2/4]
QQuickItem * ColumnView::pop | ( | const QVariant & | item | ) |
This method removes all the items after the specified item or index from the view and returns the last item that was removed.
Note that if the passed value is neither of the values said below, it will return a nullptr.
- Parameters
-
item the item to remove. It can be an item, index or not defined in which case it will pop the last item.
Definition at line 1461 of file columnview.cpp.
◆ pop() [3/4]
QQuickItem * ColumnView::pop | ( | int | index | ) |
This method removes all the items after the specified position from the view and returns the last item that was removed.
It starts iterating from the last item to the first item calling removeItem() for each of them until it reaches the specified position.
- See also
- removeItem()
- Parameters
-
the position where the iteration should stop at
- Returns
- the last item that has been removed
Definition at line 1482 of file columnview.cpp.
◆ pop() [4/4]
QQuickItem * ColumnView::pop | ( | QQuickItem * | item | ) |
This method removes all the items after the specified item from the view and returns the last item that was removed.
- See also
- removeItem()
- Parameters
-
the item where the iteration should stop at
- Returns
- the last item that has been removed
Definition at line 1472 of file columnview.cpp.
◆ qmlAttachedProperties()
|
static |
Definition at line 1522 of file columnview.cpp.
◆ removeItem [1/3]
|
slot |
This method removes an item from the view.
If the argument is a number, this method dispatches to removeItem(int index) to remove an item by its index. Otherwise the argument should be the item itself to be removed itself, and this method will dispatch to removeItem(QQuickItem *item).
- See also
- removeItem(QQuickItem *item)
- Parameters
-
index the index of the item which should be removed, or the item itself
- Returns
- the removed item
Definition at line 1450 of file columnview.cpp.
◆ removeItem() [2/3]
QQuickItem * ColumnView::removeItem | ( | int | index | ) |
This method removes an item at a given index from the view.
This method calls removeItem(QQuickItem *item) to remove the item at the specified index.
- See also
- removeItem(QQuickItem *item)
- Parameters
-
index the index of the item which should be removed
- Returns
- the removed item
Definition at line 1441 of file columnview.cpp.
◆ removeItem() [3/3]
QQuickItem * ColumnView::removeItem | ( | QQuickItem * | item | ) |
This method removes the specified item from the view.
Items will be reparented to their old parent. If they have JavaScript ownership and they didn't have an old parent, they will be destroyed. CurrentIndex may be changed in order to keep the same currentItem
- Parameters
-
item pointer to the item to remove
- Returns
- the removed item
Definition at line 1411 of file columnview.cpp.
◆ replaceItem
|
slot |
Replaces an item in the view at a given position with a new item.
The current Item and currentIndex will not be changed.
- Parameters
-
pos the position we want the new item to be placed in item the new item which will be reparented and managed
Definition at line 1320 of file columnview.cpp.
◆ scrollDuration()
int ColumnView::scrollDuration | ( | ) | const |
Definition at line 1163 of file columnview.cpp.
◆ separatorVisible()
bool ColumnView::separatorVisible | ( | ) | const |
Definition at line 1180 of file columnview.cpp.
◆ setAcceptsMouse()
void ColumnView::setAcceptsMouse | ( | bool | accepts | ) |
Definition at line 1252 of file columnview.cpp.
◆ setBottomPadding()
void ColumnView::setBottomPadding | ( | qreal | padding | ) |
Definition at line 1147 of file columnview.cpp.
◆ setColumnResizeMode()
void ColumnView::setColumnResizeMode | ( | ColumnResizeMode | mode | ) |
Definition at line 1005 of file columnview.cpp.
◆ setColumnWidth()
void ColumnView::setColumnWidth | ( | qreal | width | ) |
Definition at line 1025 of file columnview.cpp.
◆ setContentX()
void ColumnView::setContentX | ( | qreal | x | ) | const |
Definition at line 1216 of file columnview.cpp.
◆ setCurrentIndex()
void ColumnView::setCurrentIndex | ( | int | index | ) |
Definition at line 1045 of file columnview.cpp.
◆ setInteractive()
void ColumnView::setInteractive | ( | bool | interactive | ) |
Definition at line 1226 of file columnview.cpp.
◆ setScrollDuration()
void ColumnView::setScrollDuration | ( | int | duration | ) |
Definition at line 1168 of file columnview.cpp.
◆ setSeparatorVisible()
void ColumnView::setSeparatorVisible | ( | bool | visible | ) |
Definition at line 1185 of file columnview.cpp.
◆ setTopPadding()
void ColumnView::setTopPadding | ( | qreal | padding | ) |
Definition at line 1131 of file columnview.cpp.
◆ topPadding()
qreal ColumnView::topPadding | ( | ) | const |
Definition at line 1126 of file columnview.cpp.
◆ trailingVisibleItem()
QQuickItem * ColumnView::trailingVisibleItem | ( | ) | const |
Definition at line 1112 of file columnview.cpp.
◆ updatePolish()
|
overrideprotectedvirtual |
Reimplemented from QQuickItem.
Definition at line 1829 of file columnview.cpp.
◆ visibleItems()
QList< QQuickItem * > ColumnView::visibleItems | ( | ) | const |
Definition at line 1098 of file columnview.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2025 The KDE developers.
Generated on Fri Jan 3 2025 11:48:03 by doxygen 1.12.0 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.