FormLayoutAttached
#include <formlayoutattached.h>
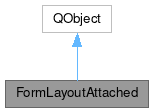
Properties | |
QQuickItem * | buddyFor |
bool | isSection |
QString | label |
int | labelAlignment |
![]() | |
objectName | |
Signals | |
void | buddyForChanged () |
void | isSectionChanged () |
void | labelAlignmentChanged () |
void | labelChanged () |
Public Member Functions | |
FormLayoutAttached (QObject *parent=nullptr) | |
QQuickItem * | buddyFor () const |
bool | isSection () const |
QString | label () const |
int | labelAlignment () const |
void | setBuddyFor (QQuickItem *aBuddyFor) |
void | setIsSection (bool section) |
void | setLabel (const QString &text) |
void | setLabelAlignment (int alignment) |
![]() | |
QObject (QObject *parent) | |
QBindable< QString > | bindableObjectName () |
bool | blockSignals (bool block) |
const QObjectList & | children () const const |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) const const |
bool | disconnect (const QObject *receiver, const char *method) const const |
void | dumpObjectInfo () const const |
void | dumpObjectTree () const const |
QList< QByteArray > | dynamicPropertyNames () const const |
virtual bool | event (QEvent *e) |
virtual bool | eventFilter (QObject *watched, QEvent *event) |
T | findChild (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QRegularExpression &re, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (Qt::FindChildOptions options) const const |
bool | inherits (const char *className) const const |
void | installEventFilter (QObject *filterObj) |
bool | isQuickItemType () const const |
bool | isWidgetType () const const |
bool | isWindowType () const const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const const |
void | moveToThread (QThread *targetThread) |
QString | objectName () const const |
void | objectNameChanged (const QString &objectName) |
QObject * | parent () const const |
QVariant | property (const char *name) const const |
Q_CLASSINFO (Name, Value) | |
Q_EMIT Q_EMIT | |
Q_ENUM (...) | |
Q_ENUM_NS (...) | |
Q_ENUMS (...) | |
Q_FLAG (...) | |
Q_FLAG_NS (...) | |
Q_FLAGS (...) | |
Q_GADGET Q_GADGET | |
Q_GADGET_EXPORT (EXPORT_MACRO) | |
Q_INTERFACES (...) | |
Q_INVOKABLE Q_INVOKABLE | |
Q_MOC_INCLUDE Q_MOC_INCLUDE | |
Q_NAMESPACE Q_NAMESPACE | |
Q_NAMESPACE_EXPORT (EXPORT_MACRO) | |
Q_OBJECT Q_OBJECT | |
Q_PROPERTY (...) | |
Q_REVISION Q_REVISION | |
Q_SET_OBJECT_NAME (Object) | |
Q_SIGNAL Q_SIGNAL | |
Q_SIGNALS Q_SIGNALS | |
Q_SLOT Q_SLOT | |
Q_SLOTS Q_SLOTS | |
T | qobject_cast (const QObject *object) |
T | qobject_cast (QObject *object) |
QT_NO_NARROWING_CONVERSIONS_IN_CONNECT QT_NO_NARROWING_CONVERSIONS_IN_CONNECT | |
void | removeEventFilter (QObject *obj) |
void | setObjectName (const QString &name) |
void | setObjectName (QAnyStringView name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | setProperty (const char *name, QVariant &&value) |
bool | signalsBlocked () const const |
int | startTimer (int interval, Qt::TimerType timerType) |
int | startTimer (std::chrono::milliseconds interval, Qt::TimerType timerType) |
QThread * | thread () const const |
Static Public Member Functions | |
static FormLayoutAttached * | qmlAttachedProperties (QObject *object) |
![]() | |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *context, Functor functor, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, Functor functor) |
bool | disconnect (const QMetaObject::Connection &connection) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
bool | disconnect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
Additional Inherited Members | |
![]() | |
typedef | QObjectList |
![]() | |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const QMetaMethod &signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const QMetaMethod &signal) |
bool | isSignalConnected (const QMetaMethod &signal) const const |
int | receivers (const char *signal) const const |
QObject * | sender () const const |
int | senderSignalIndex () const const |
virtual void | timerEvent (QTimerEvent *event) |
Detailed Description
This attached property contains the information for decorating a org::kde::kirigami::FormLayout:
It contains the text labels of fields and information about sections.
Some of its properties can be used with other Layout types.
- See also
- org::kde::kirigami::FormLayout
- Since
- 2.3
Definition at line 36 of file formlayoutattached.h.
Property Documentation
◆ buddyFor
|
readwrite |
This property can only be used in conjunction with a Kirigami.FormData.label, often in a layout that is a child of a org::kde::kirigami::FormLayout.
It then turns the item specified into a "buddy" of the label, making it work as if it were a child of the org::kde::kirigami::FormLayout.
A buddy item is useful for instance when the label has a keyboard accelerator, which when triggered provides active keyboard focus to the buddy item.
By default buddy is the item that Kirigami.FormData is attached to. Custom buddy can only be a direct child of that item; nested components are not supported at the moment.
Definition at line 131 of file formlayoutattached.h.
◆ isSection
|
readwrite |
If true, the child item of a org::kde::kirigami::FormLayout becomes a section separator, and may have different looks:
- To make it just a space between two fields, just put an empty item with FormData.isSection:
- To make it a space with a section title:
- To make it a space with a section title and a separator line: Kirigami.FormData.label: "Label:"}Kirigami.Separator {Kirigami.FormData.label: "Section Title"Kirigami.FormData.isSection: true}Kirigami.FormData.label: "Label:"}
- See also
- org::kde::kirigami::FormLayout
Definition at line 95 of file formlayoutattached.h.
◆ label
|
readwrite |
The label for a org::kde::kirigami::FormLayout field.
Definition at line 45 of file formlayoutattached.h.
◆ labelAlignment
|
readwrite |
The alignment for the label of a org::kde::kirigami::FormLayout field.
Definition at line 49 of file formlayoutattached.h.
Constructor & Destructor Documentation
◆ FormLayoutAttached()
|
explicit |
Definition at line 13 of file formlayoutattached.cpp.
◆ ~FormLayoutAttached()
|
override |
Definition at line 22 of file formlayoutattached.cpp.
Member Function Documentation
◆ buddyFor()
QQuickItem * FormLayoutAttached::buddyFor | ( | ) | const |
Definition at line 71 of file formlayoutattached.cpp.
◆ isSection()
bool FormLayoutAttached::isSection | ( | ) | const |
Definition at line 66 of file formlayoutattached.cpp.
◆ label()
QString FormLayoutAttached::label | ( | ) | const |
Definition at line 36 of file formlayoutattached.cpp.
◆ labelAlignment()
int FormLayoutAttached::labelAlignment | ( | ) | const |
Definition at line 51 of file formlayoutattached.cpp.
◆ qmlAttachedProperties()
|
static |
Definition at line 116 of file formlayoutattached.cpp.
◆ setBuddyFor()
void FormLayoutAttached::setBuddyFor | ( | QQuickItem * | aBuddyFor | ) |
Definition at line 76 of file formlayoutattached.cpp.
◆ setIsSection()
void FormLayoutAttached::setIsSection | ( | bool | section | ) |
Definition at line 56 of file formlayoutattached.cpp.
◆ setLabel()
void FormLayoutAttached::setLabel | ( | const QString & | text | ) |
Definition at line 26 of file formlayoutattached.cpp.
◆ setLabelAlignment()
void FormLayoutAttached::setLabelAlignment | ( | int | alignment | ) |
Definition at line 41 of file formlayoutattached.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2025 The KDE developers.
Generated on Fri Jan 3 2025 11:48:03 by doxygen 1.12.0 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.